Enhancing Python Performance: Key Strategies for Speed
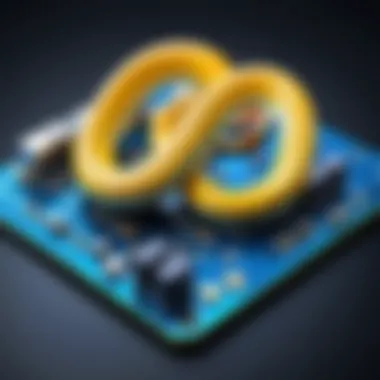
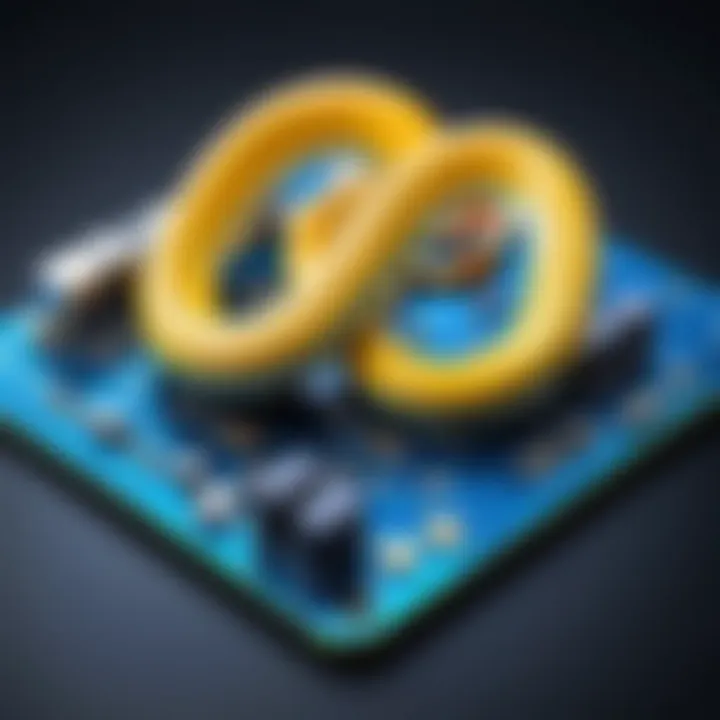
Intro
In the world of programming, Python has carved a niche for itself, celebrated for its readability and versatility. However, despite its strengths, performance issues can creep in, hampering applications ranging from simple scripts to complex machine learning models. Thus, understanding how to optimize Python code for performance is not just a nice-to-have; it is a necessity.
For developers and data scientists, this journey into optimization involves recognizing common bottlenecks, diving into built-in libraries that enhance efficiency, and adopting best coding practices. While Python might not always be the fastest language on the block, a thoughtful approach can lead to significant speed gains, enabling applications to handle heavy workloads with ease.
In this guide, we will cover not only the various strategies to fine-tune performance but also dive into real-world case studies that showcase successful implementations. By the end, readers will have a toolkit filled with actionable insights pertinent to their work, making them more adept in their respective fields.
Understanding Python Performance
When it comes to writing Python code, understanding performance is not just a minor detail—it’s the backbone of effective programming. High performance in Python can lead to smoother applications, faster data processing, and an overall better user experience. In this section, we will unpack the intricacies of Python's performance and provide insights into why it matters for developers, data scientists, and tech enthusiasts.
The contemporary digital landscape thrives on speed and efficiency. For developers, the necessity to optimize performance cannot be overstated. When lines of code run sluggishly, it reflects negatively on everything from app usability to server response times. For instance, a delay of even seconds can translate into lost sales for an e-commerce platform or a frustrating experience for a user navigating a data-heavy application. Therefore, grasping the nature of performance within Python isn’t merely beneficial; it's essential.
The Nature of Python Execution
Python is an interpreted language, which means that each line of code is executed in real time by an interpreter, rather than being compiled into machine language beforehand, as languages like C or C++ do. This characteristic plays a significant role in performance. The main advantage of this approach is flexibility and ease of debugging. Want to test a function without running the entire program? Python makes that a walk in the park. However, this ease comes with a trade-off: execution speeds can lag.
One of the notable aspects of Python is its dynamic typing feature. This adds a layer of convenience but can also slow things down. Each time a variable's type is checked at runtime, it consumes resources that could otherwise be utilized for executing the program efficiently. Therefore, understanding how Python's execution model functions can steer developers toward making informed choices, which can vastly improve execution speed.
Common Performance Misconceptions
A few myths float around regarding Python's performance that often lead developers astray. Firstly, some people believe that because Python is slower than statically-typed, compiled languages like C, it is unsuitable for high-performance applications. This couldn’t be further from the truth. Python shines in many domains—data analysis, web development, and automation are all areas where it excels. While it may not be the fastest, the right strategies can bring Python’s performance to the forefront of project requirements.
Another misconception involves the use of built-in data types. Many think that opting for lists is always the best choice due to their versatility. However, for fixed-size collections, tuples can actually provide a performance boost due to their immutable nature, leading to faster processing.
Furthermore, some developers believe higher-level abstraction means automatic efficiency. While abstraction helps in writing cleaner code, it doesn't inherently optimize performance. Misusing abstractions can lead to bloated code that runs like molasses in January.
Understanding these frameworks helps clear the fog around Python’s performance. Adopting a mindful approach to coding in Python can lead to surprising gains, making it not just a good language for many tasks but a powerful tool when performance is paramount.
We will delve further into specific techniques and tools later in this article, but grasping these foundational concepts sets the stage for optimizing your Python programs effectively. Will you join us in this journey towards unleashing the full potential of Python?
Remember: It's not always about the language you use; it's about how you implement it.
Identifying Performance Bottlenecks
In the realm of optimizing Python performance, shedding light on performance bottlenecks is crucial. These bottlenecks can be the proverbial spanners in the works, choking the flow of efficient code execution. Identifying where exactly the slowdowns occur allows developers to focus their optimization efforts where it matters most. Even the most elegantly designed algorithms can falter under the pressure of inefficient execution, making it vital to understand the various intricacies that contribute to sluggish performance.
Understanding how bottlenecks manifest helps in crafting more efficient code. Addressing these points not only improves application response times but also enhances the overall user experience. It’s like tuning a finely crafted instrument to ensure it plays in harmony rather than discord.
Using Profiling Tools
Profiling is an essential process for identifying how and where bottlenecks arise. Various tools can be used to profile Python code, each with its strengths. Knowing which tool to use is half the battle.
cProfile
When it comes to Python profiling, cProfile stands out as a top choice. This built-in profiler offers a simple yet effective means to assess how time is distributed across your functions. One significant advantage of cProfile is its ability to give a comprehensive report on function call frequencies and timings, which makes it easier to pinpoint not just the slowest components but also the most frequently called ones.
The unique aspect of cProfile is its cumulative time analysis, which can uncover areas where function calls, even if fast on their own, pile up and contribute to overall sluggishness. If you’re aiming for efficient code execution, cProfile is definitely a beneficial tool to start with, though it can produce a vast amount of data that might seem overwhelming without proper filtering and understanding.
line_profiler
For developers needing a more granular view of their code's performance, line_profiler is invaluable. Unlike cProfile, which aggregates metrics at the function level, line_profiler drills down to the line level, providing insights into which specific lines of code within a given function are causing delays. This feature is particularly useful for spotting inefficient loops or overly complex operations that might otherwise fly under the radar.
However, one must consider the overhead of adding line profiling to their code execution. Activating line_profiler might introduce a slight performance hit during profiling, so it is best utilized during development and testing phases rather than in production environments. Its detailed granularity, nonetheless, offers incomparable benefits in optimizing hot paths in the code.
memory_profiler
In performance optimization, memory usage often plays an underappreciated role, and that's where memory_profiler fits into this discussion. This tool provides insights into memory consumption alongside execution time, revealing if your slowdowns are due to excessive memory usage. It allows developers to monitor memory footprints line by line similar to how we discuss time with line_profiler.
An essential feature of memory_profiler is its ease of use; it integrates seamlessly with Jupyter notebooks and scripts. Nonetheless, developers should remain cautious, as measuring memory can be resource-intensive. If not monitored carefully, this could potentially skew results. Conversely, knowing where memory spikes occur can be as instrumental in optimization as understanding time bottlenecks.
Common Bottlenecks Analysis
After employing the right profiling tools, the next step is dissecting what sorts of bottlenecks are commonly encountered in Python code.
Time Complexity
When discussing the speed of algorithms, time complexity frequently comes into play. It refers to the computational time required as a function of the input size. Recognizing how an algorithm’s time complexity grows relative to its input is essential for predicting performance under varying conditions.
A common time complexity metric is Big O notation, which provides an upper limit on time consumption. For instance, an O(n) algorithm is more manageable as input grows than an O(n^2) algorithm. Being aware of time complexities when developing algorithms allows for direct comparison and, importantly, helps in selecting the right approach for scaling applications effectively.
However, it's also crucial to note that theoretical time complexity does not always translate to practical performance. Factors such as constant factors, underlying hardware, and even the Python interpreter can influence runtime, necessitating empirical testing alongside theoretical calculations.
Space Complexity
Treading alongside time complexity is space complexity, a crucial measure of memory use in relation to inputs. Space complexity evaluates the total amount of memory that an algorithm requires, covering both the input space and any auxiliary space utilized during execution. Particularly in environments with constrained resources, minimally invasive memory usage can mean the difference between success and failure.
Just like with time complexity, space complexity can be expressed using Big O notation. This gives developers a straightforward way to assess how their applications will scale with increasing data sizes. Furthermore, understanding both space and time complexity helps developers make informed decisions about trade-offs in optimization. Efficiently using memory often translates to a need for fewer resources, leading to faster execution and a more fulfilling user experience.
Optimizing Code Structure
When it comes to enhancing the performance of Python code, the structure of your code plays a significant role. The way we organize our functions and data types directly influences execution speed and memory usage. A well-thought-out structure not only enhances readability but also minimizes overhead, which can lead to considerable speed gains during run time. Simply put, an optimized code structure can be a game changer in the overall performance of your Python applications.

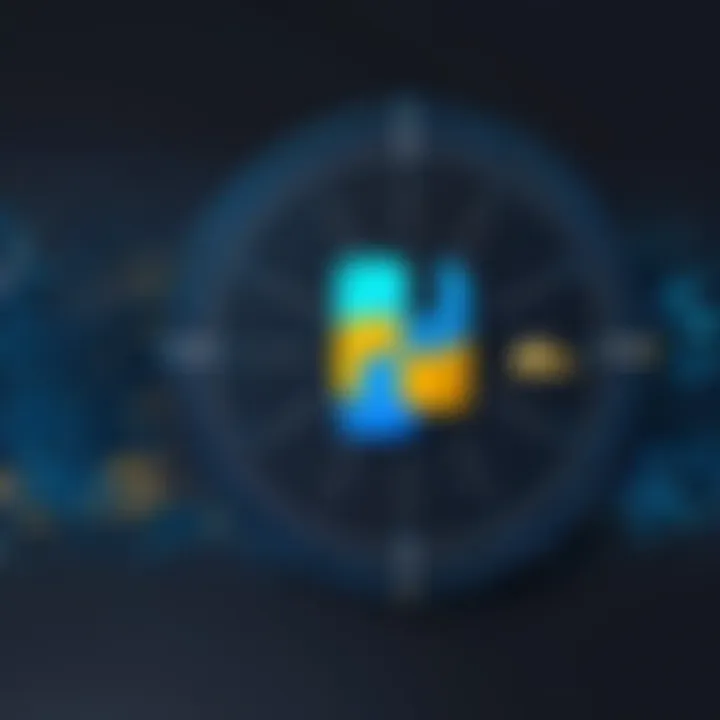
Efficient Data Structures
Data structures are the backbone of any programming language, and Python is no different. Selecting the right data structure can lead to noticeable improvements in efficiency.
Lists vs Tuples
One of the first things to understand is the difference between lists and tuples. Both are reserved for storing collections of items, but their performance characteristics vary. Lists are mutable, meaning you can change their content without creating a new instance. Tuples, on the flip side, are immutable. This immutability makes tuples lighter and faster, especially for read-heavy operations.
Key Characteristic: Tuples can be more memory-efficient than lists.
Using tuples can be a beneficial choice when the dataset is fixed, allowing optimizations during execution. Moreover, the immutable nature of tuples lets Python optimize memory allocation, in contrast with the overhead required for dynamically resizing lists.
However, if your operations include frequent inserts or updates, lists may be the proper go-to due to their flexibility despite the added overhead.
Dictionaries and Sets
Dictionaries and sets likewise contribute to optimizing code structure. A dictionary allows for key-value pair storage and excels in situations where quick lookups are necessary. Sets, meanwhile, hold unique elements and also offer fast membership testing.
Key Characteristic: Both structures utilize hash tables to maintain their data.
By employing a dictionary, developers can significantly minimize the time complexity of lookups, making them a popular choice for large datasets. Sets eliminate duplicates, making them advantageous in scenarios where data integrity is paramount.
However, the trade-off lies in the memory consumption, as these structures can be more demanding than lists and tuples.
Function Design Considerations
Effective function design is just as vital as selecting the right data structures. The manner in which functions are composed can drastically affect performance.
Reducing Function Calls
One interesting strategy to increase performance is to minimize the number of function calls. Each time a function is called, it incurs overhead that can slow down execution, especially if the calls happen within loops. Thus, reducing these calls can yield a noticeable performance boost.
For instance, consolidating multiple operations into a single function can reduce the overhead associated with context switching and stack manipulation. However, it’s crucial to strike a balance; overly complex functions can also reduce readability and maintainability.
Utilizing Generators
Generators offer a unique way to manage data efficiently. By yielding results one at a time and maintaining the state, generators help in handling large datasets without consuming a lot of memory. When faced with extensive iterations, shifting from traditional lists to generators can lead to significant performance gains.
In environments where resources are limited or performance is critical, utilizing generators not only keeps memory consumption in check but also enables progressive processing of data. Still, it's worth noting that generators can introduce complexity, particularly when debugging, as the state is not as easily iterable as traditional lists.
By optimizing your code structure through appropriate data structures and function design, you can pave the way for more efficient and faster Python applications.
Leveraging Built-in Libraries
When it comes to enhancing Python performance, leveraging built-in libraries stands as a cornerstone of effective optimization strategies. These libraries are not just tools; they provide a treasure trove of functionalities, enabling developers to write quicker and more efficient code. The importance of utilizing these libraries lies in their ability to harness underlying efficiencies that often elude the average Python programmer. By integrating libraries designed for specific tasks, substantial performance improvements can be realized without having to reinvent the wheel.
NumPy for Numerical Operations
One of the standout libraries in the Python ecosystem is NumPy. When dealing with numerical computations, NumPy shines brightly thanks to its array-oriented architecture. At its core, it allows for efficient storage and manipulation of large datasets. Using NumPy arrays can lead to performance gains because they are implemented in C and optimized for speed.
For example, simple operations on a traditional Python list can become cumbersome and time-consuming as the size increases. In contrast, NumPy allows element-wise operations to be performed in one go, making it far more efficient.
Here's a quick code comparison:
In this example, the NumPy operation is not only more concise but likely executes faster as well, thanks to NumPy's optimizations.
Pandas for Data Analysis
As Python developers dig deeper into data management and analysis, Pandas emerges as yet another vital library. It's specifically designed to handle and analyze complex datasets with ease. With its powerful DataFrame structure, it allows for efficient data manipulation, cleansing, and aggregation.
One of the benefits of using Pandas is its ability to integrate seamlessly with other data-focused libraries. When dealing with larger datasets, traditional methods might fall short in performance and readability. Applying Pandas enables chunk processing and efficient data filtering.
Consider the scenario of reading a large CSV file. Using Pandas, this is straightforward and optimal:
Such operations can be cumbersome with standard Python lists and dictionaries. By using Pandas, we save time and resources while maintaining clarity in the code.
Using Cython for Compilation
Cython presents another level of enhancement for those seeking improved execution time. This programming language aims to gain C-like performance with code that is written mostly in Python. With Cython, developers convert their Python code into compiled code, translating high-level syntax into low-level language that the machine can execute much faster.
Through simple type declarations and compiling code ahead of time, Cython can lead to performance gains that are significant, especially in execution-intensive applications. For instance, a basic Cython function can dramatically reduce the time complexity of nested loops. This makes Cython a worthy consideration when maximum speed is a priority.
Here’s how you might typically define a function in Cython:
By compiling this function, the performance can surpass that of pure Python—in turn, speeding up applications that rely heavily on iterative processes.
Leveraging built-in libraries like NumPy, Pandas, and Cython empowers programmers to optimize Python code efficiently, cutting down run times significantly.
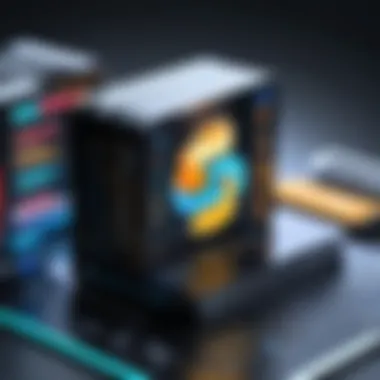
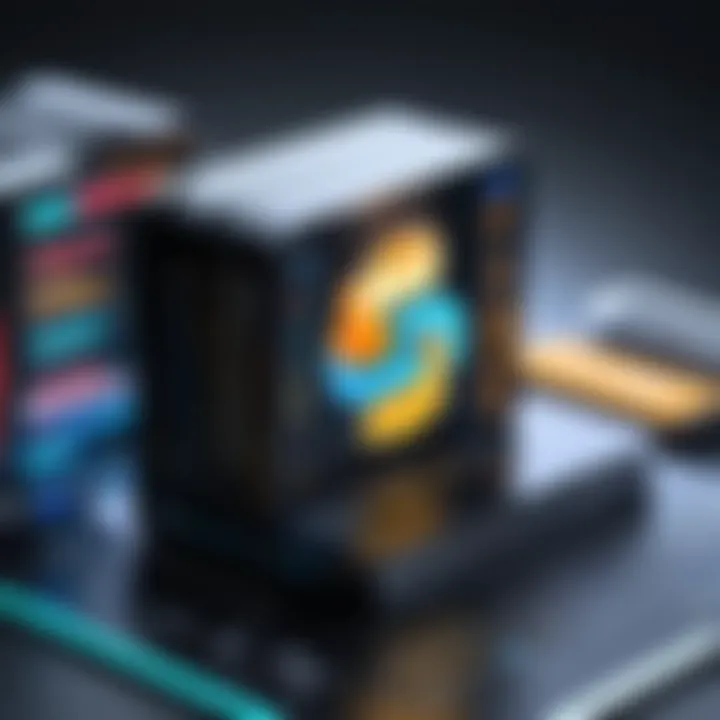
In summary, the integration of these powerful libraries not only enhances the performance of Python applications but also enriches the coding experience by allowing developers to focus on higher-level programming constructs.
Concurrency and Parallelism
In the realm of optimizing Python performance, concurrency and parallelism are critical concepts that enable developers to maximize efficiency and speed. In simple terms, concurrency allows multiple tasks to progress without necessarily being executed simultaneously, while parallelism involves executing multiple tasks at the same time. Grasping these concepts is essential for building responsive applications, especially when dealing with I/O-bound and CPU-bound tasks.
Understanding how tasks can be executed concurrently can significantly improve the responsiveness of applications. For instance, when waiting on a network response or file read, it's possible to kick off other tasks instead of idling. Having the ability to utilize the full capacity of multi-core processors through parallel processing can lead to performance gains that are noticeable in more intensive applications – from scientific computing to image processing.
Using Threads
Threads are lightweight processes that share memory space within a program. This means they can communicate with each other more easily compared to separate processes. Utilizing threads can improve the efficiency of a program, particularly in scenarios where the program often waits for input/output operations.
Here's a practical example: Imagine you have a web scraper that fetches news articles. Using threads, the scraper can send out multiple requests simultaneously instead of one by one, resulting in faster completion overall. Python's module simplifies this by allowing developers to create threads with relative ease. However, there are important considerations. The Global Interpreter Lock (GIL) in Python means that only one thread can execute Python bytecodes at a time. This can turn off some developers when working with CPU-bound tasks, as the performance may not improve as they'd hope. But don't be too quick to dismiss threads; they're still useful for I/O-bound tasks.
Remember, when using threads:
- Avoid shared state when possible to prevent race conditions.
- Be wary of thread overheads; sometimes simplicity with a single thread may outperform complex threading schemes.
- Use higher-level abstractions when applicable, such as concurrent.futures for easier thread management.
Multiprocessing Advantages
Unlike threading, multiprocessing circumvents the GIL by using separate memory spaces for each process. This means that each process can run completely independently, harnessing the power of multiple cores effectively. Python provides the module, which allows developers to create processes that can run concurrently and even share data when necessary.
Consider a computation-heavy task, such as simulating a physics model or performing data analysis. With multiprocessing, you can distribute these computational loads across multiple CPU cores, speeding up calculations significantly.
Here are some advantages of using multiprocessing:
- True parallelism: Since each process runs in its own memory space, they can operate independently without being hindered by the GIL.
- Scalability: Applications can take advantage of multiple cores without much modification to the code structure.
- Better stability: If one process crashes, it doesn't affect other processes, allowing the main application to continue running.
However, multiprocessing isn't without its downsides. The overhead of creating processes can sometimes be more considerable than the gain in speed. It also comes with its own set of challenges in terms of data sharing and inter-process communication, which may require careful design.
A balance between threading and multiprocessing is often key. It is essential to evaluate the demands of your application and choose the right strategy based on whether it is I/O-bound or CPU-bound.
Memory Management Techniques
Optimizing memory management in Python is a critical aspect that significantly influences the overall performance of applications. When code consumes more memory than necessary, it tends to slow down execution and may lead to inefficient resource use. Good memory management helps in freeing up resources, thus ensuring that your application runs seamlessly. Here, we’ll explore key insights into garbage collection and the use of memory-efficient libraries that can enhance your coding practice.
Garbage Collection Insights
Garbage collection plays a pivotal role in automated memory management within Python. It essentially refers to the process of identifying and disposing of objects that are no longer needed by a program. Since Python uses reference counting to track objects, it automatically frees memory allocated to objects once there are no references to them. However, circular references can complicate matters, potentially leaving memory unused.
To mitigate these issues, Python employs a cyclic garbage collector which periodically scans for unreachable objects – those that are circularly referenced. Understanding these mechanics can help pythonistas refine their code. For example:
- Be cautious with object references. Keeping unnecessary references can lead to memory not being freed.
- Use weak references when suitable. This technique allows for more aggressive memory cleanup without disrupting object lifecycle.
Using Memory Efficient Libraries
Employing specialized libraries designed for memory efficiency can vastly improve performance in Python applications. Let’s delve into two notable libraries: Struct and Array.
Struct
The library is particularly advantageous for dealing with binary data. It enables packing and unpacking of C-style data structures into byte strings. One key characteristic of is its ability to minimize memory usage by converting Python types into byte representations, which often occupies less space than traditional Python data types.
- Benefits: It offers a structured way to handle data, especially when interfacing with C APIs or handling large datasets where memory overhead is a concern.
- Considerations: While is powerful, its syntax and requirements can be a bit complex for beginners, which may lead to unnecessary overhead if used inappropriately.
Array
Similar in intent, the library offers a type-specific implementation of arrays in Python, allowing for the effective storage of homogeneous data. This library is invaluable for large datasets, requiring less memory than lists because it stores data more compactly in contiguous memory allocations.
- Benefits: The array holds elements of the same type, providing speed improvements during iteration and operations. It’s particularly favored in applications needing high-performance numerical calculations.
- Considerations: However, whereas are efficient, they are also less flexible than lists, which could pose a challenge when dealing with varying data types.
Incorporating these libraries into your coding practices not only paves the way for enhanced performance but also helps you manage your application’s memory footprint effectively.
"Effective memory management is the unsung hero of optimized code. It quietly ensures that applications run faster and more efficiently, all while enhancing the user experience."
By better understanding memory management techniques, such as garbage collection and leveraging memory-efficient libraries, developers can significantly improve their code's execution speed and responsiveness.
Database Interaction Optimization
Optimizing how your Python code interacts with databases is crucial in improving performance, especially in applications that rely heavily on data storage and retrieval. A well-optimized database interaction can be the make or break when it comes to the speed of your application. When databases aren't structured or queried effectively, it can lead to significant slowdowns and resource waste, which is the last thing any developer wants.
By focusing on this aspect, developers not only ensure faster execution of queries but also enhance the scalability and responsiveness of the application. Specifically, this section will cover efficient query structuring and connection pooling strategies, two fundamental elements in achieving optimal database interactions.
Efficient Query Structuring
Query structuring plays a vital role in how quickly and efficiently a database can retrieve and process data. Poorly written queries can create bottlenecks that slow down an entire application. Here are a few thoughts on how to optimize your query structuring:
- Select Only Needed Columns: Instead of using , always specify the columns you need. This cuts down on data transfer time and reduces the load on the database.
- Use Indexing Wisely: Proper indexing can dramatically speed up data retrieval. However, over-indexing can harm performance during write operations. Think carefully about which columns need indexes.
- Database Normalization: Normalize your database to eliminate redundant data. It might feel tempting to have denormalized structures for faster reads, but the trade-off could be higher maintenance complexity. A balanced approach is necessary.
"The efficiency of your database interactions is often hidden beneath the surface, yet it can define the overall performance of your application."
- Avoid complex joins when simpler alternatives exist: Sometimes, restructuring the database or queries can lead to a more straightforward approach that enhances performance without needing deep joins.
- Batch processing: Instead of sending single queries, consider batching them. This can lead to considerable reductions in the number of database round trips and subsequently improve the application's performance.
Connection Pooling Strategies
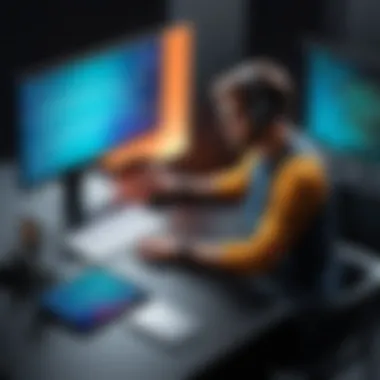
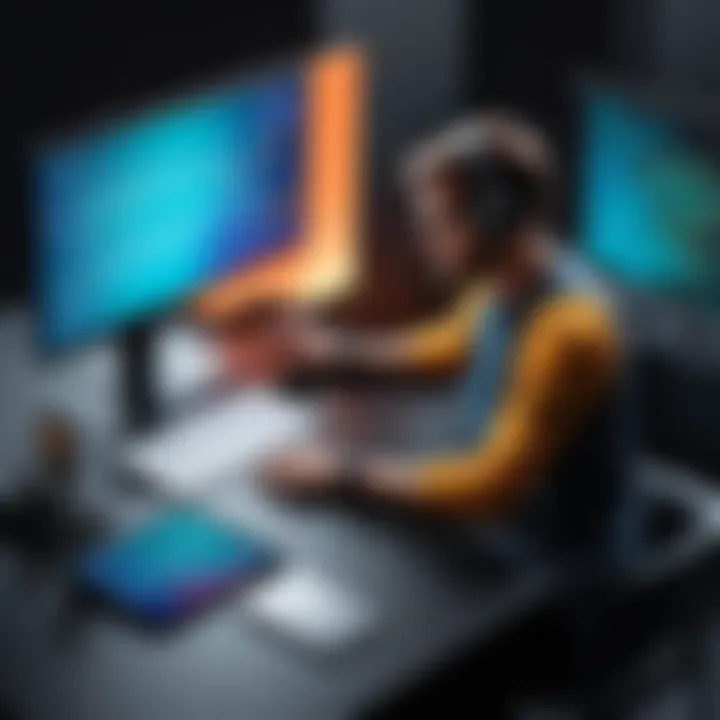
Connection pooling is an essential technique that helps manage database connections, minimizing the overhead associated with establishing new connections. Below are some considerations regarding connection pooling:
- Reuse Connections: A connection pool allows multiple requests to share a limited number of active database connections, which reduces the overhead associated with creating new connections. This can significantly improve response times in applications with heavy database traffic.
- Define Connection Limits: Set sensible limits for the maximum number of connections that your application can open concurrently. This helps in not overloading the database server and maintains stability.
- Connection Timeout Settings: Adjust timeout settings to ensure that connections that are no longer needed get closed swiftly, freeing up resources for other requests.
By implementing effective connection pooling strategies, applications can handle numerous requests efficiently, keeping performance steady even under heavy loads.
Testing and Benchmarking
In the realm of Python performance optimization, testing and benchmarking stand as cornerstones of effective practice. Understanding how your code behaves in real-world scenarios allows you to identify not only where your strengths lie but also where pitfalls might linger. When developers push their applications to the limit without proper benchmarks, they often miss critical performance insights. Without a doubt, effective benchmarking provides clarity and drives improvement.
Setting Up Benchmark Tests
Setting up benchmark tests is essential for gaining a transparent view of your code’s execution time and resource consumption. These tests help isolate specific functions or sections of your program, allowing you to measure execution speed under various scenarios. The following steps can guide you in establishing an effective benchmark:
- Choose the Right Framework: Selecting an appropriate benchmarking framework is crucial. Libraries like or are great tools that can help measure execution time accurately by repeating the specified code several times to get a reliable average.
- Isolate the Code: Create a controlled environment by isolating the piece of code you wish to analyze. This helps eliminate external factors such as concurrent processes that may skew results.
- Conduct Multiple Trials: Run your tests multiple times. This repetition helps smooth out any anomalies and leads to more reliable results. For precise averages, inscribe loops into your test.
- Analyze Results Methodically: After you obtain results, analyze them thoroughly. Look for outliers or patterns amongst your data. Sometimes, seemingly minor discrepancies can shed light on hidden inefficiencies within your code.
Here is a simple example of how to set up a benchmark test using the module:
Evaluating Performance Improvements
Once you have your benchmark tests in place, the next logical step is evaluating the performance improvements that result from your optimizations. This involves comparing the performance metrics before and after changes have been made. Here are key considerations to keep in mind:
- Result Comparisons: Establish a direct comparison of the metrics you’ve gathered. For instance, how much did the execution time reduce after applying a specific optimization? Establishing a quantifiable metric can reveal valuable insights.
- Assess Memory Usage: Alongside speed, remember to assess memory consumption. An optimized code may also consume less memory, which can be beneficial for performance, particularly for resource-heavy applications.
- Utilize Visual Tools: Consider using visual tools or software for deeper analysis. Tools like Line Profiler can show you which lines of code are consuming the most time. It can be an eye-opener.
- Continuous Regression Testing: Finally, make it a habit to perform these evaluations regularly as you refactor or enhance your codebase over time. Keeping a continuous testing approach ensures that performance degradation doesn't sneak up on you.
Benchmarking is not a one-time scenario; it’s an ongoing part of maintaining performance efficiency.
All these insights come together to help optimize not just for speed, but for an overall more efficient execution environment. Whether you are a software developer, data scientist, or tech enthusiast, understanding the depths of testing and benchmarking can significantly uplift your programming game.
Advanced Techniques
Exploring advanced techniques can be a game changer when it comes to enhancing Python's performance. While the basics are important, they only scratch the surface. Advanced strategies take a deeper dive into optimization, often speaking directly to the needs and requirements of developers wanting to squeeze every bit of performance out of their code. These techniques can lead to significant improvements in execution speed and resource utilization, which is crucial in today’s performance-oriented environment.
Here, we will discuss two key advanced techniques: just-in-time (JIT) compilation with Numba and utilizing AsyncIO for I/O operations. Both of these approaches have their unique advantages but also come with certain considerations that developers must keep in mind to fully leverage their capabilities.
JIT Compilation with Numba
Numba is a powerful tool that can drastically optimize the performance of numerical computations in Python by converting specific Python functions into machine code at runtime. This technique, known as Just-In-Time (JIT) compilation, allows functions to run at speeds comparable to, or even faster than, equivalent code written in lower-level languages such as C.
Why Use JIT Compilation?
- Speed Boost: Functions decorated with can achieve massive speed improvements, especially in loops and mathematical functions, reducing execution time significantly.
- Ease of Use: Integrating Numba into existing code is relatively straightforward. You can often see performance gains by simply adding a decorator above your function.
- Compatible with NumPy: Numba works particularly well with NumPy arrays, making it ideal for projects that rely heavily on numerical calculations.
Before employing Numba, it’s essential to consider:
- Type Stability: The function should ideally have a consistent input type to fully benefit from JIT.
- Code Complexity: Some complex Python constructs may not be supported by Numba, so profiling your code to see what can be optimized becomes important.
For example, here's a simple demonstration:
Here, wrapping the sum_of_squares function with allows Numba to optimize the loop execution, speeding up the computation significantly.
Utilizing AsyncIO for /O Operations
When it comes to managing I/O-bound tasks, AsyncIO stands tall as a vital framework within the asynchronous programming paradigm. It allows developers to write single-threaded concurrent code using coroutines, offering a fresh approach to handling operations like file reading, network requests, or database queries without blocking.
Benefits of AsyncIO:
- Concurrency Without Threads: It allows concurrency without the overhead of thread management. By using coroutines, you can handle many operations more efficiently.
- Enhanced Responsiveness: Especially important in web applications, using AsyncIO can lead to a more responsive user experience since it allows other tasks to run while waiting for I/O tasks to complete.
- Scalability: Applications built on AsyncIO can easily scale under high loads, managing numerous simultaneous connections or requests efficiently.
That said, there are considerations:
- Learning Curve: For many developers accustomed to traditional Python scripts, moving to an asynchronous model can be a paradigm shift.
- Not Always Faster: For CPU-bound tasks, AsyncIO won’t necessarily offer performance enhancements. It shines primarily in I/O-bound scenarios.
An example of using AsyncIO can be seen below:
In this example, the fetch_data function retrieves data from a URL without blocking the main thread, showcasing how AsyncIO can streamline workflows involving I/O tasks.
Culmination
In this concluding section, we reflect on the importance of employing effective strategies for optimizing Python performance. Performance optimization isn't just an optional enhancement; it's a fundamental aspect of coding that can determine whether a program is a sleek, efficient machine or an overworked mule struggling to get the job done. By understanding the principles discussed throughout this article, developers can harness the capabilities of Python without falling victim to its default speed limitations.
Recap of Key Strategies
Throughout our exploration, we have identified several significant strategies that stand to elevate the performance of Python code. These range from identifying bottlenecks using profiling tools like cProfile and line_profiler to employing advanced techniques such as JIT compilation with Numba. The importance of efficient coding practices cannot be overstated. Here’s a quick recap of the key strategies:
- Identify Bottlenecks: Use various profiling tools to analyze your code and pinpoint where the slowdowns are occurring.
- Optimize Data Structures: Choose the most suitable data structures, such as using tuples instead of lists when immutability is required, or opting for dictionaries for fast lookups.
- Leverage Built-in Libraries: Make use of libraries like NumPy for numerical computations and Pandas for data analysis, as they are optimized for performance.
- Explore Concurrency and Parallelism: Depending on your application’s needs, decisions on whether to use threading or multiprocessing can yield significant speed boosts.
- Memory Management: Keep an eye on how your code allocates memory. Utilizing memory-efficient libraries and understanding how Python’s garbage collector works can help manage this.
By integrating these strategies, software developers, data scientists, and tech enthusiasts will find themselves better equipped to tackle performance issues head-on.
Encouraging Continued Learning
Final thoughts lead us to an encouragement of continuous learning in the field of Python performance optimization. Technology and best practices evolve rapidly, and staying updated can be challenging. Here’s what can help keep the learning process alive:
- Engage with Community: Platforms like Reddit or specialized forums are ideal for learning from peers and sharing experiences.
- Experiment with Projects: Hands-on practice is invaluable. Implementing small projects with a focus on performance can lead to insights that theoretical knowledge alone may not provide.
- Read and Explore Resources: Periodical updates on Python enhancements and optimizations can be found on websites like Wikipedia or Britannica. Always look for new insights and upcoming libraries that may complement your toolkit.
In summary, the realm of Python performance optimization is vast and requires a proactive approach to keep up with its nuances. Whether you're tweaking a legacy codebase or diving into a fresh project, the commitment to optimize will independently influence the user experience and, ultimately, the success of your software applications.