Optimizing Java for Peak Performance and Efficiency
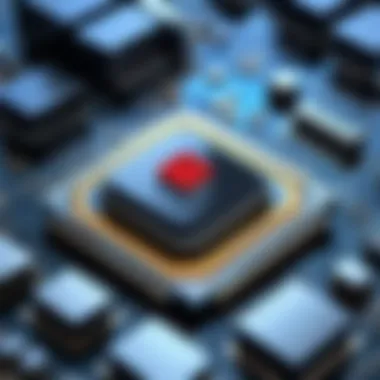
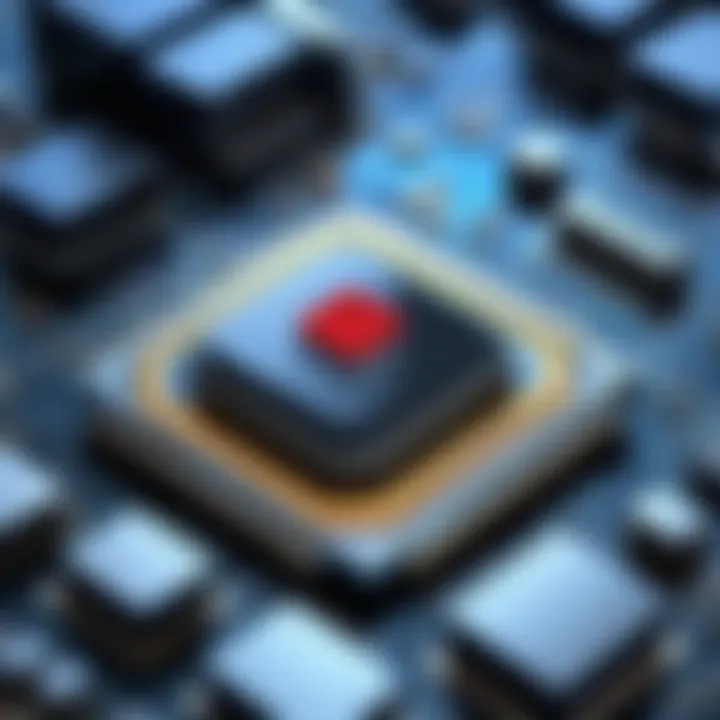
Intro
Optimizing Java applications is a crucial aspect of software development that directly impacts performance and efficiency. As Java remains a widely used programming language across various industries, understanding how to enhance its capabilities can lead to significant improvements. The aim of this article is to delve into specific optimization techniques, exploring garbage collection, memory management, multithreading, and effective coding practices. By addressing these elements, Java developers will gain valuable insights into strategies that can optimize their applications, yielding better user experiences and resource utilization.
Overview of Optimization Techniques
Java is often praised for its portability and scalability, yet it can face challenges in performance under certain conditions. Common issues arise from inefficient garbage collection, excessive memory consumption, and suboptimal multithreading practices. Recognizing how to effectively address these challenges is essential for developers seeking high-performance applications.
Definition and importance of optimization
Optimization in Java refers to the process of improving application performance and resource management. This is particularly important in environments where speed and resource conservation are paramount, such as cloud computing and big data applications. Every millisecond of response time and byte of memory matters when it comes to building responsive and robust systems.
Key features of optimization
Some key areas of focus for optimization include:
- Garbage Collection Tuning: Understanding how to appropriately tune garbage collection settings can lead to lower pause times and reduced memory consumption.
- Memory Management: Efficient management of memory usage helps prevent memory leaks and excessive garbage collection cycles.
- Multithreading: Proper implementation of multithreading can improve throughput and responsiveness in applications.
- Code Optimization: Reviewing and refining algorithm efficiency ensures that only necessary operations are executed.
Use cases and benefits
The benefits of optimization are numerous. Applications that utilize optimized Java code experience reduced latency, increased throughput, and better resource utilization. For example, large-scale enterprise applications that handle significant user loads require optimizations to maintain responsiveness. By implementing effective strategies, developers can enhance performance without sacrificing functionality.
Best Practices
Adopting best practices for optimization is vital for achieving consistent performance improvements.
Industry best practices
- Regularly profile your application using tools like Java Flight Recorder and VisualVM. Profiling helps identify bottlenecks and memory issues.
- Apply proper garbage collection algorithms for the application's unique requirements. For instance, the G1 garbage collector is often suitable for large heap sizes.
Tips for maximizing efficiency
- Employ data structures that best match your data access patterns. For example, using a HashMap for frequent lookups ensures faster access times.
- Review and refactor long-running operations to prevent blocking other threads. Using asynchronous methods can improve performance.
Common pitfalls to avoid
- Avoid premature optimization; first, identify critical areas needing improvement.
- Do not ignore the importance of clean and maintainable code. Complex optimizations can lead to increased technical debt and hinder future iterations.
Case Studies
Real-world examples can exemplify effective optimization strategies.
Successful implementation
Consider a financial services application. After identifying memory bottlenecks, the team optimized the garbage collection settings and implemented efficient data structures, resulting in improved transaction processing times by over 25%.
Lessons learned
One key takeaway was the importance of thorough testing after optimization. Performance improvements must be balanced against stability to ensure no new issues arise.
Insights from industry experts
Industry analysts emphasize that optimization should not be a one-time activity but an integral part of the development lifecycle. Continuous evaluation and adjustment yield lasting benefits.
Latest Trends and Updates
As technology evolves, so do optimization techniques and best practices.
Upcoming advancements
New garbage collection algorithms, such as Z Garbage Collector, promise low-latency performance. These advancements allow developers to handle larger heaps effectively.
Current industry trends
Trends show a growing importance of reactive programming to improve responsiveness and scaling capabilities in Java applications. This shift encourages developers to adopt asynchronous and event-driven architectures.
Innovations and breakthroughs
The rise of machine learning models directly in Java applications is influencing optimization strategies. Developers need to focus on optimizing AI alongside traditional functionalities to handle complex use cases efficiently.
How-To Guides and Tutorials
For those who seek practical advice, step-by-step guides can be invaluable.
Step-by-step guides for using optimization tools
- Install Java Profiling Tools: Use tools like VisualVM or YourKit.
- Profile Your Application: Run tests to benchmark performance.
- Analyze Results: Identify areas for improvement.
- Implement Changes: Apply adjustments and tune settings.
- Re-test: Always validate changes to ensure performance gains.
Hands-on tutorials for beginners and advanced users
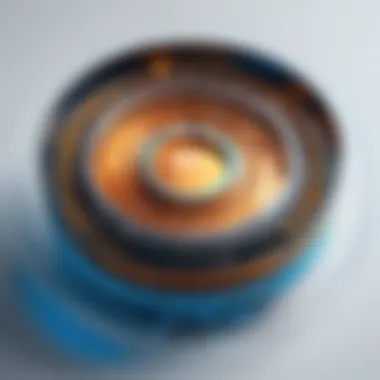
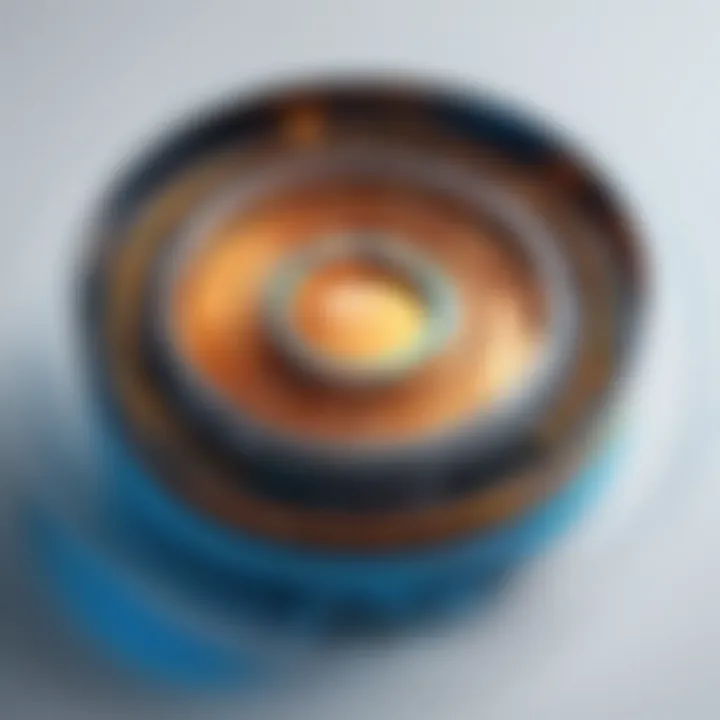
Beginner developers can start with basic profiling. Advanced users might explore intricate settings of the JVM and experiment with different garbage collectors. Each level of understanding provides an opportunity to enhance application performance effectively.
By focusing on these topics, the article will serve as a comprehensive guide for Java developers, equipping them with the knowledge to improve their application's performance while navigating challenges in the fast-evolving tech landscape.
Prelude to Java Optimization
Optimizing Java is an essential consideration for developers aiming to enhance the performance of their applications. Java, while versatile and widely used, can be demanding on resources if not managed properly. Developers must understand the various components that influence Java's performance, including memory management, threading, and code efficiency. This article aims to shed light on these dimensions and provide actionable strategies to tackle performance issues.
Understanding Java Performance
Java performance is influenced by multiple factors, such as execution speed, memory usage, and responsiveness. It is crucial for developers to monitor and analyze these aspects to achieve an efficient application. Some key aspects include:
- Execution Speed: The time taken to execute a Java application can significantly affect user experience. A slow application can frustrate users and increase abandonment rates.
- Memory Usage: Java applications can consume a considerable amount of memory, especially when managing large data sets or running multiple processes. Efficiency in memory management is vital to prevent leaks and optimize performance.
- Responsiveness: Applications that react quickly improve user satisfaction. Achieving responsiveness often requires effective thread management and asynchronous programming.
By understanding these performance metrics, developers can identify areas for improvement and ultimately enhance the user experience.
Importance of Optimization in Java Development
Optimization in Java development is not merely about improving speed; it encompasses a range of practices that lead to longevity and scalability of applications. The benefits are clear:
- Cost Efficiency: Optimizing code and resource usage can lead to lower operational costs. Efficient applications consume less hardware and network resources.
- Scalability: As applications grow, an optimized codebase can handle increased loads better. Efficient algorithms and data management practices allow systems to scale without significant rewrites.
- User Retention: Performance directly correlates with user satisfaction. Optimized applications retain users better and are more likely to generate positive reviews.
In summary, the optimization process is a strategic investment that pays off in terms of performance, cost, and user satisfaction. Emphasizing the importance of this topic is vital for every Java developer aiming for success in modern software development.
Profiling Java Applications
Profiling Java applications is a crucial step in understanding their performance characteristics and behavior during execution. Profiling involves the measurement of various aspects of program performance, including CPU usage, memory consumption, and execution time. This information allows developers to identify inefficient code paths, assess system performance, and improve overall application efficiency. The insights derived from profiling assist in both proactive optimizations and reactive troubleshooting, ultimately contributing to higher-quality Java applications.
Furthermore, profiling is not a one-time task; it is part of an ongoing process of refinement within Java development. It ensures that changes in the code base do not negatively affect performance. Developers who implement consistent profiling practices often benefit from enhanced code quality and more resilient applications.
Types of Profiling Tools
When it comes to profiling Java applications, various tools exist to meet different development needs. Commonly used profiling tools include:
- JVisualVM: This is an official monitoring tool that comes bundled with the JDK. It provides real-time data on application memory consumption, CPU usage, and thread activity.
- YourKit Java Profiler: This commercial solution offers advanced profiling capabilities focused on both memory and CPU. It helps identify memory leaks and optimize the application.
- Eclipse Memory Analyzer: Often used for analyzing heap dumps, this tool helps identify memory leaks and inefficient memory usage.
- JProfiler: Similar to YourKit, this tool provides a comprehensive profiling experience, including CPU, memory, and thread profiling.
Each tool has its own strengths and weaknesses, and selecting the appropriate tool largely depends on the specific profiling needs of the application. Developers should assess their requirements, complexity of the application, and lifecycle stage when choosing a profiling tool.
Identifying Bottlenecks
Identifying bottlenecks in a Java application is a key objective of profiling. A bottleneck occurs when a particular resource limit restricts system performance, ultimately causing unnecessary delays. Profiling helps locate these bottlenecks by pinpointing areas in the code or resource allocation that require optimization.
To effectively identify bottlenecks, developers should focus on:
- Analyzing CPU Usage: Monitor methods and processes that consume excessive CPU time. This can highlight methods that are inefficiently coded or could benefit from algorithm changes.
- Memory Analysis: Investigating memory leaks or unexpected memory usage can substantially enhance performance. Tools like YourKit and Eclipse Memory Analyzer can assist in finding and fixing memory-related issues.
- Thread Monitoring: Examine thread states and synchronization points. Excessive blocking or contention can dramatically slow down performance, leading to sluggish applications.
By recognizing and addressing bottlenecks, developers can significantly improve performance metrics, leading to faster response times and better user experiences.
Memory Management Strategies
Memory management is a critical aspect of Java performance optimization. Proper handling of memory can significantly impact application speed, responsiveness, and scalability. Techniques in this domain not only prevent memory leaks and excessive garbage collection but also enhance the overall efficiency of applications. Understanding memory management strategies is essential for any developer aiming to achieve high-performance Java applications.
Understanding the Java Memory Model
The Java Memory Model (JMM) defines how threads interact through memory and what behaviors are allowed when they access shared variables. It plays a fundamental role in determining how memory is allocated and managed in Java.
Java applications generally operate within a memory structure divided into several regions: the heap, stack, and method areas. The heap is used for dynamic memory allocation, where objects and their instance variables reside. The stack stores local variables and method call information.
The JMM ensures thread safety by providing rules about visibility and ordering of actions in concurrent programming. Each time a thread reads or writes to a variable, it must adhere to these defined behaviors. Understanding JMM helps in making informed decisions about memory sharing and optimization techniques that avoid pitfalls like data inconsistency.
Garbage Collection Optimization
Garbage collection (GC) in Java automates memory management by reclaiming objects that are no longer in use. While beneficial, it can also introduce overhead and affect performance. Optimizing garbage collection is a vital step towards enhancing Java application performance.
Java provides several collectors, such as the G1, CMS, and ParNew, each with unique characteristics. It's crucial to select the most suitable garbage collector based on your application’s requirements. One can monitor GC performance through various tools such as jVisualVM and GC logs, helping identify excessive pause times or frequent collections.
Some optimization techniques include:
- Adjusting Heap Size: Properly sizing the heap can help in reducing the frequency of GC pauses.
- Minimizing Tenuring: Aim to minimize the number of objects that stay long in the young generation to limit expensive promotions to old generation space.
- Selecting the Right Collector: Match the garbage collector to the application type, whether it is low-latency or throughput focused.
Effective GC tuning can lead to better resource utilization and a smoother user experience.
Effective Use of Memory Pools
Memory pools in Java allow for structured memory allocation within the heap. They enable efficient management and use of memory resources by partitioning the heap into different areas.
When utilizing memory pools, developers can control how memory is allocated, utilized, and released. By strategically employing pools, objects created during program execution can be reused, reducing the costs associated with frequent allocations and deallocations.
Consider these strategies for memory pool management:
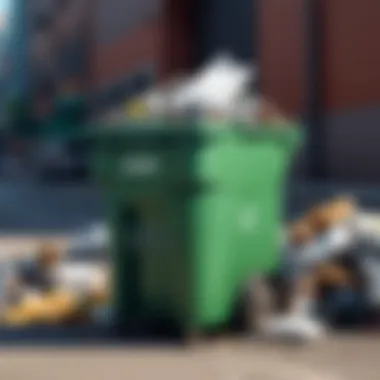
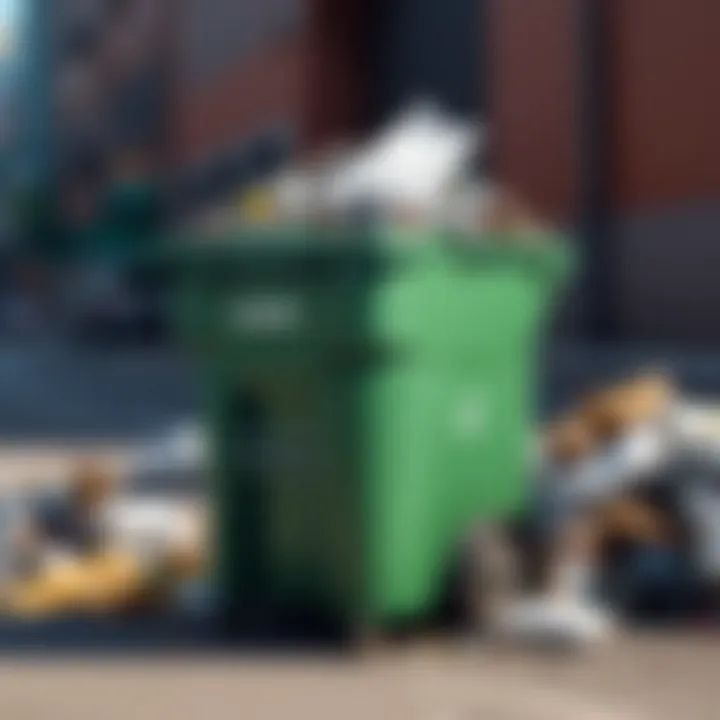
- Pooling similar objects: Create pools for frequently instantiated objects, such as database connections or thread resources.
- Optimizing pool sizes: Analyze application behavior to determine ideal pool sizes, balancing performance and resource use.
- Recycling pools: Instead of releasing objects back to the GC, recycle them for reuse, which can reduce GC pressure and improve performance.
Proper use of memory pools can lead to more responsive applications and a reduction in overall memory consumption.
Code Optimization Techniques
Code optimization is a critical aspect of Java development focused on improving the performance of Java applications. Efficient code not only enhances runtime speed but also optimizes resource use, leading to better overall performance. Here, we will explore vital techniques that can be implemented to optimize code effectively. Each technique addresses specific performance challenges, providing tangible benefits.
Efficient Data Structures and Algorithms
Selecting the right data structures and algorithms plays a crucial role in performance. Java offers various data structures like ArrayList, HashMap, and LinkedList, each with its own strengths and weaknesses. For example, using a HashMap for frequent search operations is far more efficient than using an ArrayList when dealing with large data sets due to its average constant time complexity for insertions and lookups.
When choosing algorithms, consider complexity. Prefer algorithms with lower time complexity to minimize processing time. A classic example is implementing a sorting algorithm. While Bubble Sort may be easier to understand, its average time complexity is O(n²). Using Quick Sort or Merge Sort, which average O(n log n), is usually much better for large datasets. By carefully choosing data structures and algorithms, developers can eliminate bottlenecks and dramatically improve application responsiveness.
Minimizing Object Creation
Java’s garbage collection mechanism is efficient but not without costs. Frequent object creation can lead to increased garbage collection cycles, which can decrease application performance. Optimizing object creation involves reusing objects where possible, leveraging design patterns like Singleton for unique instances, and using object pools for frequently used objects.
Also, consider using primitive types over their corresponding wrapper classes whenever feasible. For instance, using instead of can save significant memory overhead. By paying attention to how and when objects are created, developers can effectively reduce the performance impact caused by excessive garbage collection.
Inlining Methods and Constants
Inlining is a powerful optimization technique that involves replacing a method call with the method's actual code. This reduces the overhead of a method call and can significantly improve performance for small, frequently called methods. However, developers should judiciously apply this strategy. Over-inlining can lead to larger bytecodes and increased memory consumption. Therefore, it's vital to balance performance gains against possible size increases.
In addition, consider using constants, especially when their values are fixed. Instead of recalculating values, define them as constants, allowing for better readability and optimization by the Java compiler. This can enhance performance as the compiler can streamline the code during compilation.
"Optimizing code early in the development process can lead to significant improvements down the line, preventing technical debt and performance issues."
In summary, focusing on code optimization techniques empowers developers to write efficient, high-performance Java applications. Utilizing effective data structures, minimizing unnecessary object creation, and employing inlining intelligently can bring noticeable performance enhancements. As Java continues to evolve, staying updated on these optimization strategies remains essential for developers aiming for excellence.
Multithreading and Concurrency
Java's capability to handle multithreading and concurrency is fundamental for developing responsive and efficient applications. In a world where applications often need to perform multiple tasks at the same time, understanding this topic becomes crucial. It allows developers to optimize resource usage, leading to faster execution and improved performance.
Concurrency enables the execution of several threads simultaneously. Each thread runs independently, allowing tasks to be processed in parallel. This is particularly beneficial in environments where tasks are I/O-bound or require significant processing power. However, managing concurrency effectively requires an understanding of various aspects of thread management and synchronization techniques.
Understanding Thread Management
Thread management in Java involves creating, controlling, and terminating threads. Managing threads properly is essential for effective concurrency. Java provides several classes and methods for thread management through the package.
When creating threads, developers can choose between extending the class or implementing the interface. Both methods have their pros and cons: the interface is generally more flexible as it allows a class to extend another class as well. Once threads are created, they can be started using the method, which invokes the method in the new thread.
Additionally, controlling the lifecycle of threads is important. Threads can be in various states such as new, runnable, blocked, waiting, and terminated. Understanding these states can help developers anticipate which thread might need more resources or when to possibly terminate a thread that is no longer necessary, thus saving system resources.
Using Executors for Better Performance
Executors provide a higher level of abstraction for managing threads. This feature is part of the package. Using executors allows for better control over thread lifecycle and resource allocation. Rather than manually creating and managing threads, developers can use an executor to handle tasks more efficiently.
One of the key advantages of using executors is the ability to pool threads, which helps in reducing the overhead of thread creation. A thread pool recycles a fixed number of threads for executing multiple tasks, thus improving resource utilization.
For instance, the is a common executor suitable for situations where the number of tasks doesn’t exceed the number of available threads.
Here is an example of how to use a fixed thread pool:
This example demonstrates submitting ten tasks to a pool of five threads, ensuring effective resource use while executing the tasks concurrently.
Synchronization Techniques
Synchronization in Java is critical to prevent data inconsistency when multiple threads access shared resources. Without synchronization, threads can modify data simultaneously, leading to unpredictable behavior.
Java provides synchronized methods and synchronized blocks to manage access to shared resources. By marking a method or block as synchronized, developers can ensure that only one thread can access that section of code at any given time.
Another technique is using , which offers more flexibility compared to synchronized blocks. It allows developers to attempt to acquire the lock without blocking and provides more sophisticated features like conditional waiting.
"Effective synchronization ensures data integrity and stability in multithreaded applications."
Best Practices for High-Performance Java
Optimizing Java applications goes beyond choosing the right algorithms or managing memory appropriately. It also involves adopting best practices that ensure long-term performance improvements. Best practices help developers maintain a balance between code readability and performance efficiency while leveraging the built-in capabilities of the Java ecosystem. In this section, we will delve into two key aspects: the trade-offs between code readability and performance and the advantages of utilizing Java’s Standard Libraries.
Code Readability vs Performance
Code readability is an essential quality in software development. When developers write clear and concise code, it becomes easier for others to understand, maintain, and extend it. However, optimizing for performance can sometimes lead to complex and less readable code. The challenge lies in finding a harmonious balance.
Some developers may prioritize performance at the cost of clarity, using intricate operations that boost speed but confuse anyone who later needs to work with the code. On the other hand, overly simplistic code can lead to inefficiencies in processing.
In practice, it is necessary to evaluate performance impacts carefully. A good approach is to write clean and readable code first, ensuring it accomplishes the required functionality. After achieving this, profiling the code can identify bottlenecks where optimization is necessary. Remember, optimization should come only when the performance gains are clear and justified.
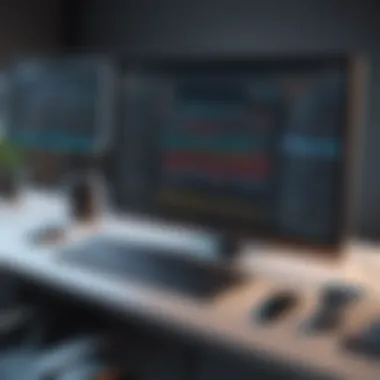
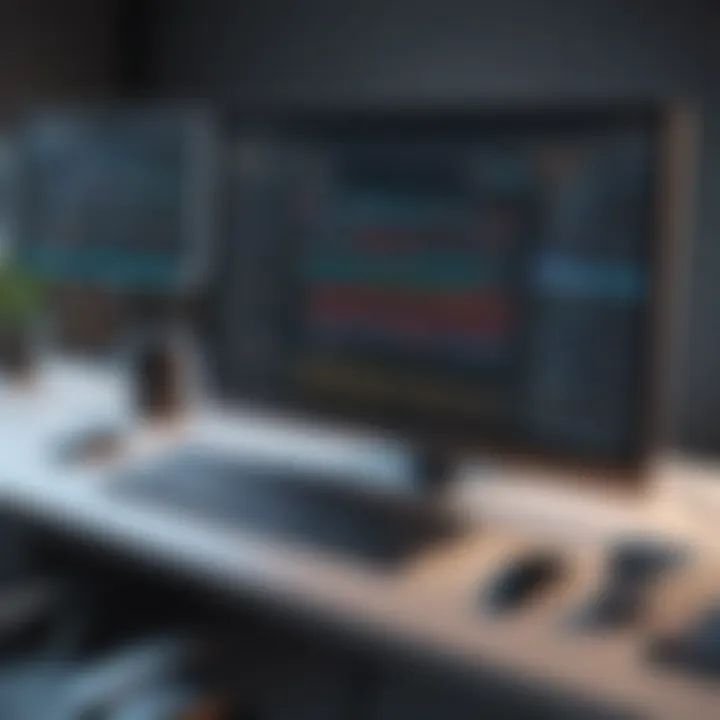
"Writing readable code is a long-term investment that facilitates collaboration and innovation."
Utilizing Java Standard Libraries
Java provides a rich set of built-in libraries that offer optimized functions for many common performance-related tasks. Utilizing these libraries is beneficial for several reasons.
- Efficient Implementations: The Java Standard Libraries are built with performance in mind. They often provide optimized implementations which can save developers time and effort.
- Well-Tested: These libraries undergo rigorous testing and refinement. Consequently, their reliability is often superior to custom solutions devised on the fly.
- Time Savings: By utilizing existing libraries, developers can focus on the unique aspects of their applications rather than reinventing the wheel.
- Ease of Maintenance: Relying on standard libraries reduces the complexity of codebases. Maintenance becomes easier, as fewer custom implementations mean less technical debt.
When employing Java Standard Libraries, developers should explore options such as the Java Collections Framework, which offers various data structures effective for specific use cases. Additionally, the Stream API can help in processing sequences of elements, providing significant performance improvements over traditional for-loops.
Benchmarking Java Applications
Benchmarking is a critical aspect of optimizing any application, including those developed in Java. It serves to measure the performance of the application under various conditions and workloads. By understanding performance metrics, Java developers can identify inefficiencies and optimize their code accordingly. A robust benchmarking process reveals how applications respond to changes, assisting developers in understanding the impact of their optimizations.
One of the primary benefits of benchmarking is its ability to provide objective data. Metrics collected during benchmarks help in making informed decisions on performance improvements. This data can also be crucial when justifying the need for refactoring or other optimizations to stakeholders.
However, when considering benchmarking, it is vital to acknowledge certain considerations. It is easy to focus solely on numbers without considering the context in which they arise. Conditions such as system load, underlying hardware, and even Java Virtual Machine (JVM) settings can significantly influence the results. Therefore, establishing a consistent environment for benchmarks is important. Moreover, benchmarks should be designed to simulate realistic usage patterns rather than artificial scenarios, which might not provide a true picture of performance.
Consequently, establishing best practices for benchmarking Java applications can offer a structured approach. This includes defining key performance indicators (KPIs), regularly reviewing results, and integrating benchmarking into the development cycles.
Tools for Benchmarking
There are many tools available for benchmarking Java applications, each offering distinct features that cater to varying requirements. Here are some notable choices:
- JMH (Java Microbenchmark Harness): Designed specifically for benchmarking Java code, JMH provides accurate and reliable metrics. It is particularly useful for micro-level benchmarks and allows developers to create benchmarks with various configurations.
- Apache JMeter: Although primarily used for load testing, JMeter can also be employed to benchmark Java applications. It measures the performance of various components, enabling testing under simulated load conditions.
- YourKit Profiler: This commercial tool provides comprehensive profiling and benchmarking features. It helps identify memory leaks and performance bottlenecks in real-time, which can be incredibly useful during the optimization process.
Using the right tool fosters confidence that the data gathered will be useful in guiding optimization efforts. Each tool comes with its strengths, and developers should choose one that best matches their specific needs.
Interpreting Benchmark Results
Once benchmarks are executed, the next critical step is interpreting the results. This process involves analyzing the data collected, understanding what it signifies, and determining actionable insights. Performance metrics such as response time, throughput, and resource utilization help in evaluating the effectiveness of code.
Understanding the context in which these metrics are derived is fundamental. For instance, a low response time under minimal load may not be an accurate indicator of performance under heavy usage. Thus, careful consideration of different workloads is crucial for a comprehensive assessment.
It is also important to compare current results with baseline measurements. This comparison can highlight trends over time and assess the impact of recent code changes.
"The value of benchmarking lies not only in gathering data but in the insights that lead to performance improvements."
After evaluating results, developers should take the necessary steps to refine their code further. This may involve profiling hot spots, revisiting algorithms, or adjusting configurations. Documenting findings ensures continuity and helps in establishing a robust optimization process.
This continual cycle of benchmarking and refinement is essential. A systematic approach reinforces performance as a priority, ensuring applications are not only functional but also performant under varying conditions.
Continuous Optimization Process
Continuous optimization is a crucial aspect of Java application development. This process involves systematically refining performance at every stage of the software lifecycle. It recognizes that optimization is not a one-time effort but an ongoing journey. Every new feature, bug fix, or enhancement can introduce changes that impact performance. Thus, adopting a mindset of continuous optimization leads to consistently high-performing applications.
Implementing Agile Development Practices
Agile development practices tightly align with the philosophy of continuous optimization. Agile methods emphasize incremental changes and rapid iterations. By breaking down the development process into smaller, manageable parts, teams can quickly respond to performance-related issues. For example, regular sprints allow developers to analyze performance metrics and make adjustments before issues escalate. This approach fosters collaboration and allows for testing and feedback in real-time.
Incorporating Agile practices also ensures that performance considerations are embedded within the development cycle rather than treated as an afterthought. For instance:
- Daily stand-ups can include discussions about optimization goals.
- Sprint reviews can focus on the performance impact of new features implemented.
- Retrospectives can help teams identify optimization strategies that worked well or areas needing improvement.
These measures keep performance at the forefront, encouraging a culture where optimization is part of daily discussions.
Feedback Loops and Iterative Improvement
Feedback loops are fundamental to the continuous optimization process. They provide developers with immediate insights into how their code is performing in real-world conditions. By setting up effective feedback mechanisms, teams can collect data on application performance, user experience, and system resource usage.
For example, integrating monitoring tools can help identify performance bottlenecks, which in turn guides developers toward areas requiring improvement. Iterative improvement means that developers do not wait for a major release to address performance issues. Instead, they continuously refine their code based on feedback and real-time analytics.
"Continuous feedback is the key to unlocking application performance. It turns data into actionable insights, allowing for proactive measures rather than reactive fixes."
A few effective strategies for implementing feedback into the optimization process include:
- Automated Performance Testing: Regularly run performance tests during development to catch issues early.
- Utilize A/B Testing: Experiment with different versions of features to determine which performs better with actual users.
- Post-Release Monitoring: Keep an eye on application performance metrics after deployment to catch any regressions.
Closure and Future Directions
In the realm of Java optimization, arriving at a conclusion entails more than just summarizing key points addressed throughout the article. It represents an ongoing commitment to enhance performance, efficiency, and adaptability of Java applications. Java continues to evolve, just as the technologies surrounding it do. It is pivotal for developers to keep abreast of these developments to maintain and improve application performance.
Moreover, future directions in Java optimization emphasize the need for a proactive approach toward integrating emerging technologies. Tools and methodologies that were formerly adequate may become obsolete. Continuous learning and adaptation are vital. One of the substantial benefits of recognizing these future directions lies in their potential to provide competitive advantages in developing high-performance applications.
As we delve into the specific elements of what’s next for Java optimization, several key areas warrant attention. Adapting to advancements in hardware, such as multi-core processors, demands an updated understanding of concurrency and threading. Similarly, shifts in software architecture, like microservices and serverless models, bring new challenges and opportunities for optimizing Java applications. Testing and profiling tools will also need to evolve alongside these changes to ensure robust performance validation.
Continuous optimization is not merely a phase; instead, it should be regarded as a core practice in the software development lifecycle.
Emerging Trends in Java Optimization
The landscape of Java optimization is experiencing notable shifts. Developers must remain vigilant with emerging trends to harness the full potential of their applications. One such trend is the increasing reliance on machine learning and artificial intelligence in performance tuning. Integrating these technologies can offer dynamic, data-driven optimization approaches that adapt based on real-time performance metrics.
Furthermore, performance enhancing tools are evolving at a rapid pace. GraalVM, for instance, is gaining traction as it allows polyglot programming and offers ahead-of-time compilation for Java. This innovation has strong implications for reducing startup time and improving application efficiency.
On the other hand, containerization and orchestration tools like Kubernetes significantly impact how developers deploy Java applications. These systems help manage resources more effectively, allowing for smoother scalability and increased performance under load.
Amid these shifts, it remains critical to uphold the fundamentals of Java performance such as optimizing algorithms, managing memory efficiently, and ensuring minimal object creation. The integration of traditional best practices with modern methodologies forms a solid foundation for robust application performance.