Mastering Buffer Utilization: A Comprehensive Guide for Software Development Excellence
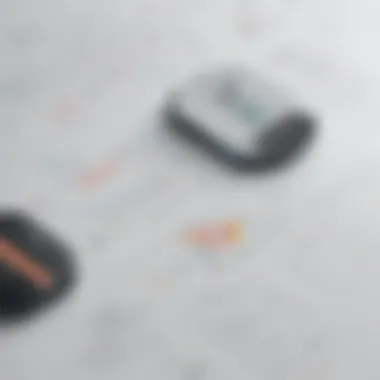
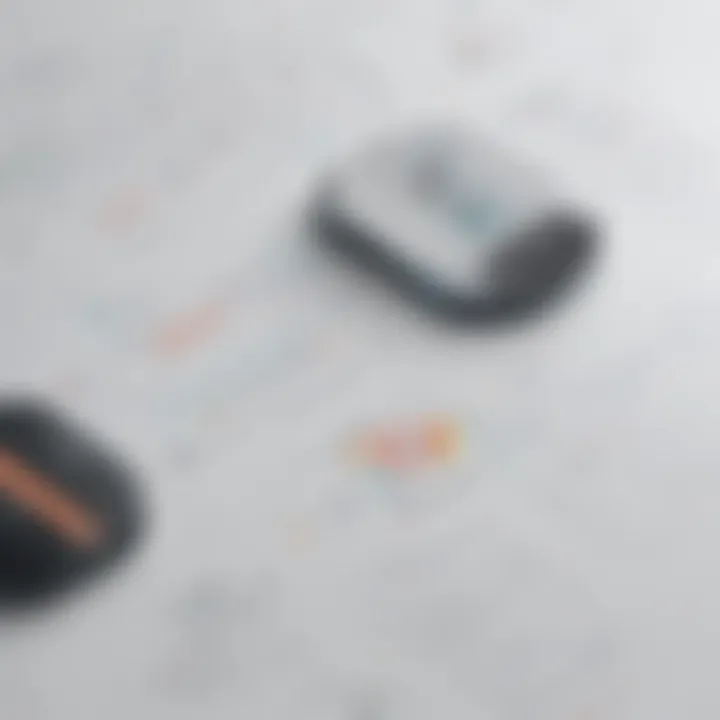
Overview of Buffer Utilization in Software Development
In the realm of software development, the efficient use of buffers plays a crucial role in optimizing performance. Buffers act as temporary storage areas in memory that hold data while it is being transferred between locations or processes. They help in smoothing out discrepancies in data transfer speeds, ultimately enhancing the overall efficiency of the software. Understanding the concept of buffers and how to utilize them effectively is essential for developers aiming to create high-performing applications.
Best Practices for Buffer Utilization
When it comes to maximizing buffer utilization in software development, following industry best practices is key. Developers should pay attention to buffer size allocation, ensuring it is neither too small to handle the required data nor excessively large, leading to inefficiencies. Implementing efficient data transfer algorithms and error handling mechanisms is crucial for optimal buffer management. It is essential to avoid common pitfalls such as buffer overflows, which can result in security vulnerabilities and system crashes.
Case Studies on Buffer Optimization
Examining real-world examples of successful buffer utilization can provide valuable insights for developers. Case studies showcasing how companies have effectively managed buffers to improve application performance can offer practical learnings. Lessons learned from these implementations, including challenges faced and outcomes achieved, can guide developers in enhancing their own buffer utilization strategies. Industry experts can share valuable insights on best practices and innovative approaches to buffer management.
Latest Trends and Updates in Buffer Management
Staying informed about the latest trends and updates in buffer management is crucial for developers seeking to stay ahead of the curve. Emerging advancements in buffer optimization techniques, such as adaptive buffer resizing and advanced caching strategies, are transforming the way buffers are utilized in software development. Keeping up with current industry trends and forecasts enables developers to leverage cutting-edge buffer management solutions and drive innovation in their projects.
How-To Guides and Tutorials for Effective Buffer Utilization
For developers looking to enhance their buffer utilization skills, comprehensive how-to guides and tutorials can be invaluable resources. Step-by-step walkthroughs on setting up and managing buffers, along with hands-on tutorials for both beginners and advanced users, offer practical insights into optimizing buffer performance. Additionally, practical tips and tricks for effective buffer utilization, such as cache-friendly programming techniques and memory management strategies, empower developers to elevate their software development capabilities.
Introduction to Buffers
Buffer utilization plays a pivotal role in software development, ensuring efficient data processing and resource management. Buffers are essential components that aid in optimizing data transmission and enhancing overall system performance. Understanding the various aspects of buffers is crucial for developers aiming to streamline their code and boost operational efficiency.
What are Buffers?
Buffer Definition:
Buffers in computing serve as temporary storage areas that hold data during the transfer process. They act as intermediary spaces between different components, facilitating smooth data flow and preventing bottlenecks. The buffer definition highlights the significance of these structures in managing data effectively within a software system. By efficiently handling incoming and outgoing data streams, buffers contribute to improved system responsiveness and streamlined operations.
Role of Buffers in Computing:
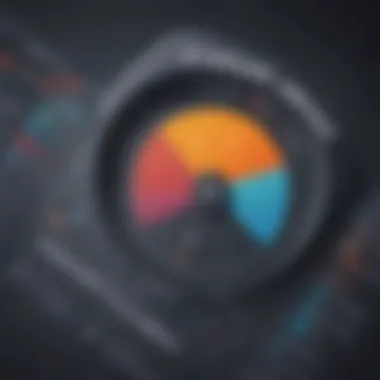
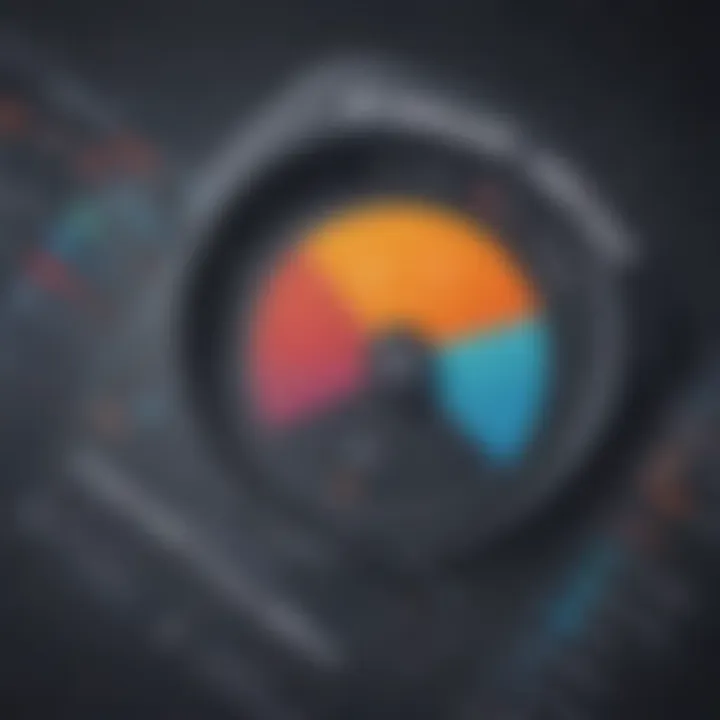
Buffers in computing are instrumental in mitigating latency issues and optimizing resource utilization. They enable efficient data processing by temporarily storing information and regulating the flow of data between system components. The role of buffers in computing is crucial for maintaining data integrity and enhancing the overall performance of software applications. By reducing data transmission delays and optimizing resource allocation, buffers play a vital role in ensuring smooth and reliable operation of software systems.
Types of Buffers
Input Buffers:
Input buffers are designated memory spaces that store incoming data before processing. These buffers help in handling data streams efficiently by temporarily storing input information until it is ready for consumption. The key characteristic of input buffers lies in their ability to manage high volumes of data while ensuring seamless data transfer within the system. While input buffers enhance data processing speed, they may also impact system performance if not managed effectively.
Output Buffers:
Output buffers serve as temporary storage areas for processed data awaiting transmission or further processing. By holding output information until it is ready to be transmitted, these buffers regulate the flow of data and prevent data loss during transfer. Output buffers play a vital role in optimizing data transmission efficiency and ensuring the orderly delivery of information to external systems or users.
Circular Buffers:
Circular buffers, also known as ring buffers, are specialized data structures that facilitate continuous data processing by recycling storage space. These buffers offer a seamless way to manage data streams by overwriting old data with new information once the buffer capacity is reached. The unique feature of circular buffers lies in their ability to provide a constant stream of data without the need for resizing or extensive memory management. However, careful handling is required to prevent data overwrite and ensure data integrity.
Importance of Buffers in Software Development
Data Transmission Efficiency:
Efficient data transmission is a key advantage of utilizing buffers in software development. By optimizing data flow and reducing bottlenecks, buffers enhance the speed and reliability of data transfer processes. The data transmission efficiency supported by buffers ensures quick and seamless communication between system components, leading to improved overall system performance.
Resource Management:
Effective resource management is another critical aspect of buffer utilization in software development. Buffers help in allocating and managing system resources efficiently, preventing resource conflicts and enhancing system stability. By ensuring optimal resource utilization and preventing resource exhaustion, buffers contribute to the seamless operation of software applications and mitigate potential performance issues.
Optimizing Buffer Performance
Buffer Size Considerations
Choosing the Right Buffer Size
Choosing the Right Buffer Size plays a pivotal role in determining the effectiveness of buffer utilization. The size of a buffer directly impacts how efficiently data is processed and transmitted within a software system. Selecting an appropriate buffer size requires striking a balance between accommodating data volume and avoiding memory wastage. The optimal buffer size is influenced by factors such as the type of data being handled, the system's memory constraints, and the desired performance outcomes. A carefully chosen buffer size can enhance system responsiveness and throughput by ensuring that the right amount of data is buffered for processing at any given time.
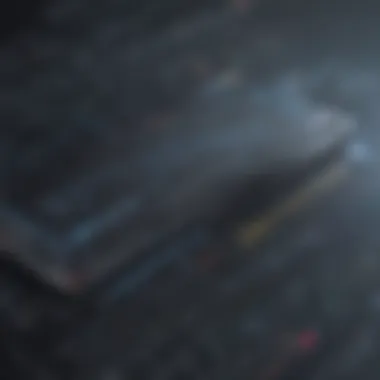
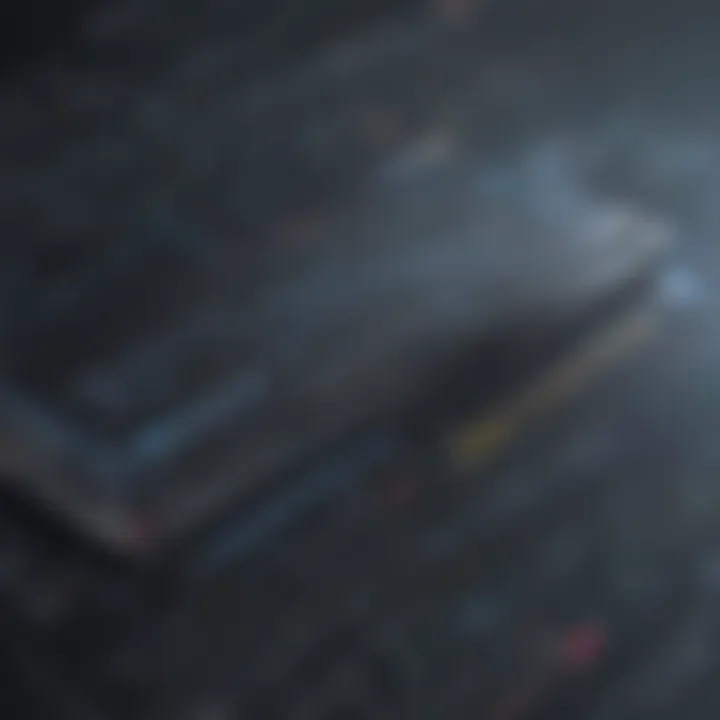
Impact on Memory Management
The Impact on Memory Management resulting from buffer size decisions is profound. Inefficient buffer sizing can lead to memory inefficiencies, such as excessive memory allocations or underutilization of available memory. Choosing an oversized buffer can strain system resources, while a too-small buffer may result in frequent data overflows or throughput bottlenecks. Properly managing buffer size is essential for optimizing memory usage and ensuring optimal software performance. By understanding the impact of buffer size on memory management, developers can make informed decisions to enhance system stability and efficiency.
Buffer Overflow Prevention
Boundary Checking Techniques
Implementing effective Boundary Checking Techniques is imperative for preventing buffer overflows. Boundary checking involves validating input sizes and ensuring that data written to buffers does not exceed their allocated memory space. By enforcing strict boundary checks, developers can mitigate the risk of buffer overflows and potential security vulnerabilities. Utilizing techniques such as bounds checking and buffer size validation helps safeguard software systems against data corruption and memory-related issues.
Error Handling Strategies
Error Handling Strategies play a key role in managing buffer overflows and addressing errors that may arise during data processing. When buffer overflows occur, having robust error handling mechanisms in place can help maintain system integrity and prevent crashes or malfunctions. Effective error handling strategies include logging error messages, implementing data validation routines, and gracefully handling exceptions to prevent cascade failures. By incorporating reliable error handling mechanisms, developers can enhance the resilience of their software applications and promote overall system stability.
Buffer Utilization Best Practices
Efficient Data Handling
Efficient Data Handling practices are essential for maximizing buffer utilization and improving software performance. Efficient data handling involves optimizing data read and write operations within buffer structures to minimize processing overhead and maximize throughput. By adopting efficient data handling techniques, such as batch processing and data compression, developers can enhance data transmission efficiency and reduce latency in software systems.
Optimized O Operations
Optimized IO Operations are crucial for streamlining data transfer processes and minimizing bottlenecks in software applications. By optimizing inputoutput operations, developers can enhance data throughput, reduce latency, and improve overall system responsiveness. Utilizing techniques like asynchronous IO, prefetching, and buffering can significantly enhance IO performance and enhance the overall efficiency of software systems. Optimized IO operations play a critical role in ensuring smooth data flow and enhancing the user experience in software applications.
Buffer Management Strategies
Buffer management strategies play a critical role in optimizing buffer utilization in software development. Proper management of buffers ensures efficient data handling and optimized IO operations. By implementing effective buffer management strategies, software developers can significantly enhance system performance and resource utilization. Without a robust buffer management approach, systems may encounter inefficiencies, memory leaks, or data corruption issues. Given the vast amount of data processed in software applications, buffer management becomes indispensable for maintaining system stability and performance.
Dynamic Memory Allocation
Malloc and Calloc Functions
Dynamic memory allocation using functions like Malloc and Calloc is pivotal in buffer management strategies. Malloc allocates memory blocks of a specified size, while Calloc initializes the allocated memory to zero. These functions offer flexibility in managing memory dynamically during runtime, allowing for efficient memory utilization and deallocation. Malloc is popular for its ability to allocate memory based on the specific requirements of the program, enabling flexible memory management. In contrast, Calloc provides the advantage of initializing memory blocks, reducing the risk of accessing uninitialized memory. While Malloc and Calloc enhance the dynamic memory allocation process, they may lead to memory fragmentation and potential memory leaks if not used judiciously.
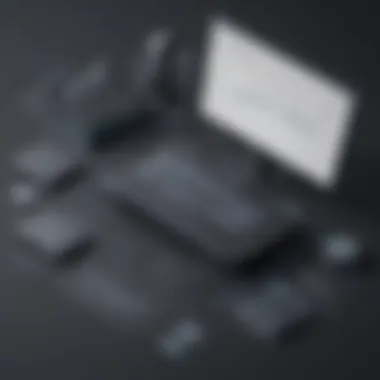
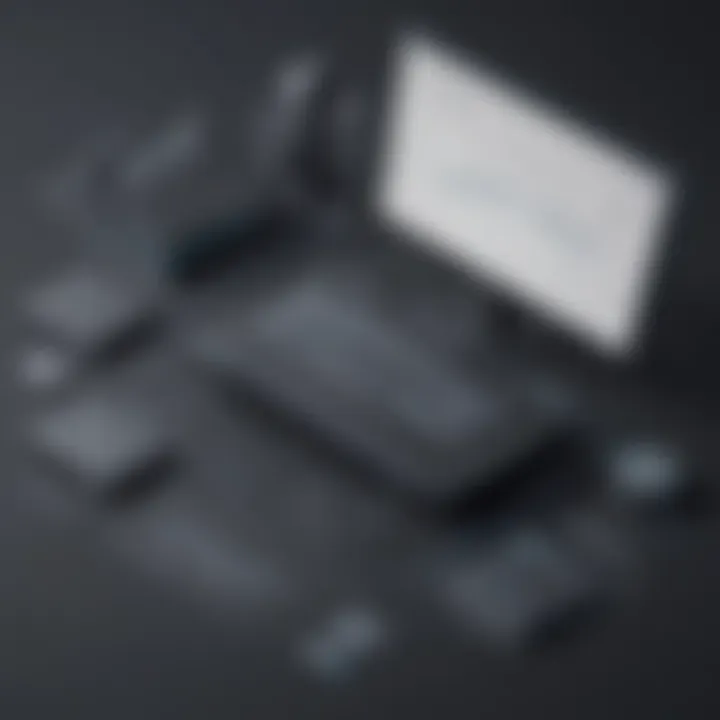
Memory Deallocation
Memory deallocation is a crucial aspect of dynamic memory management in buffer utilization. Proper deallocation of memory allocated using functions like Malloc and Calloc prevents memory leakage and ensures optimal memory usage. Memory deallocation, often done through the Free function, releases allocated memory back to the system for reassignment. However, improper memory deallocation can result in memory leaks, where allocated memory remains inaccessible, leading to inefficient memory usage and potential system crashes. Effective memory deallocation is essential for maintaining system integrity and preventing memory-related issues in software applications.
Buffer Recycling Techniques
Buffer Pooling
Buffer pooling is a key technique in buffer management strategies aimed at reusing buffer memory efficiently. By maintaining a pool of pre-allocated buffers, applications can avoid the overhead of continuous memory allocation and deallocation. Buffer pooling enhances system performance by reducing memory fragmentation and overhead associated with dynamic memory management. The reuse of pre-allocated buffers in buffer pooling leads to optimized memory utilization and improved memory access speeds, contributing to faster data processing and enhanced system efficiency.
Object Pool Pattern
The Object Pool pattern is another valuable technique in buffer recycling, focused on managing a pool of reusable objects. By preallocating objects and managing their lifecycle, the Object Pool pattern optimizes resource usage and performance. This pattern allows for efficient object creation and destruction, reducing the overhead of frequent object instantiation. The Object Pool pattern enhances system scalability and performance by minimizing the overhead of object creation and destruction, making it a preferred choice for optimizing buffer utilization in software development.
Multi-Threaded Buffer Handling
Synchronization Mechanisms
Synchronization mechanisms are essential for managing buffers in multi-threaded environments, ensuring thread safety and data consistency. Techniques like mutexes, semaphores, and spin locks facilitate synchronized access to shared buffers among multiple threads. Synchronization mechanisms prevent race conditions and data corruption issues that may arise from concurrent buffer access. By implementing effective synchronization mechanisms, software developers can maintain data integrity and coherence in multi-threaded buffer handling scenarios.
Thread-Safe Buffer Access
Thread-safe buffer access is critical for preventing data corruption in multi-threaded applications. Establishing thread-safe buffer access involves implementing synchronization mechanisms like mutex locks or atomic operations to safeguard shared buffers from concurrent write or read accesses. Thread-safe buffer access guarantees data consistency and prevents data races in multi-threaded environments, ensuring that critical data in buffers remains intact and correctly updated. By prioritizing thread-safe buffer access, developers can mitigate the risks associated with concurrent data access and maintain system reliability in multi-threaded software applications.
Buffer Optimization Tools
In the realm of software development, Buffer Optimization Tools play a crucial role in enhancing performance and efficiency. These tools are designed to analyze and fine-tune buffer usage to prevent bottlenecks and optimize data handling processes. By utilizing Buffer Optimization Tools, developers can improve memory management, data transmission speed, and overall system stability. One major benefit of incorporating these tools is the ability to identify and rectify potential buffer overflow issues before they impact system functionality.
Profiling and Monitoring Tools
- Valgrind: Valgrind is an essential tool for detecting memory leaks, potential errors, and managing memory efficiently in software. Its key characteristic lies in its ability to provide detailed reports on memory allocation and deallocation, helping developers pinpoint memory-related issues swiftly. Valgrind's popularity stems from its accurate and reliable analysis, making it a preferred choice for optimizing buffer utilization in software development.
- GNU gprof: GNU gprof is a powerful profiling tool that aids in identifying code segments consuming disproportionate amounts of processing time. By highlighting performance bottlenecks, GNU gprof enables developers to streamline their code and enhance overall program efficiency. Its unique feature lies in its ability to generate comprehensive reports on code execution, allowing for targeted optimizations. While GNU gprof offers valuable insights, it may have slight overhead in terms of runtime performance.
Debugging Utilities for Buffer Analysis
- GDB Debugger: The GDB Debugger is a versatile utility for debugging and analyzing code behavior, making it a valuable asset in buffer analysis. Its key characteristic is the ability to trace program execution step by step, facilitating the identification and resolution of buffer-related issues effectively. GDB Debugger's interactive nature and wide array of features make it a go-to choice for software developers seeking robust debugging capabilities.
- AddressSanitizer: AddressSanitizer is a dynamic tool that detects memory errors, including buffer overflows and accesses to deallocated memory regions. Its unique feature lies in its instrumentation approach, which adds runtime checks to the compiled code for real-time error detection. While AddressSanitizer offers comprehensive memory error detection, it may result in increased memory consumption and execution overhead.
Performance Tuning Techniques
- Cache Optimization: Cache Optimization plays a vital role in enhancing buffer performance by leveraging system caches effectively. Its key characteristic is the ability to minimize memory latency and improve data access speeds by storing frequently accessed data closer to the processor. The unique feature of Cache Optimization lies in its capacity to boost overall system performance by reducing the time taken to retrieve data from main memory. However, improper cache configurations may lead to cache thrashing and hinder performance.
- IO System Tweaks: IO System Tweaks focus on optimizing inputoutput operations to maximize buffer efficiency. These tweaks enhance data transfer speeds and reduce latency by fine-tuning how data is read from and written to storage devices. The key characteristic of IO System Tweaks is their impact on overall system responsiveness and data processing capabilities. However, implementing aggressive tweaks without proper testing may result in data corruption or system instability.