Developing a Python Application: A Comprehensive Guide
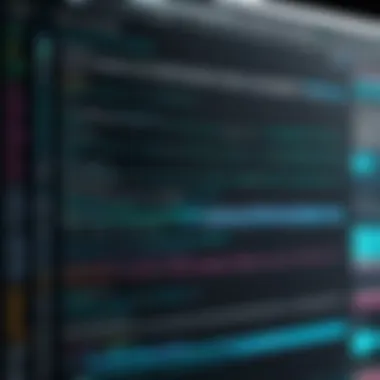
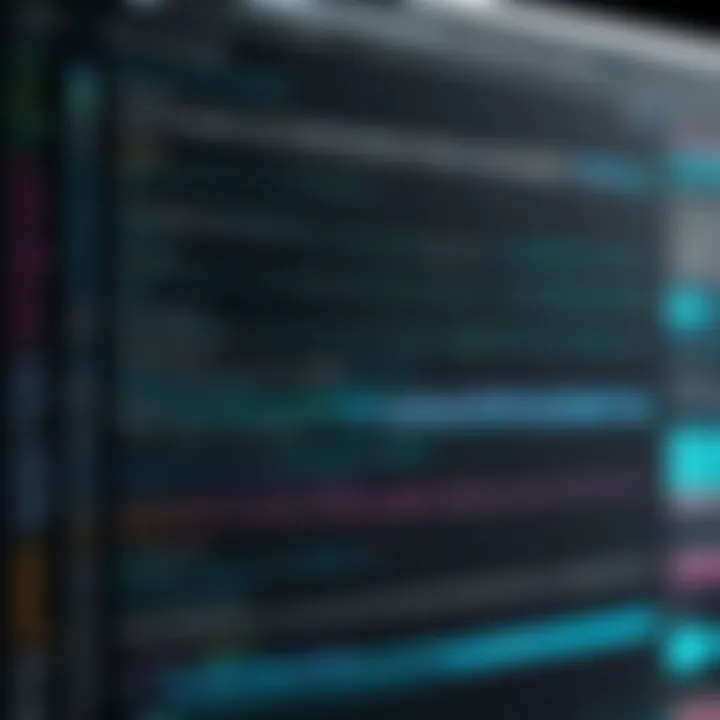
Intro
In a world increasingly reliant on technology, developing applications is vital for both personal and professional pursuits. Python, known for its readability and vast library support, has become a preferred programming language among developers. Its simplicity enables programmers to create a wide range of applications, from web services to data analysis tools, making it an attractive option.
This guide aims to provide an in-depth overview of Python application development. We will walk you through each stage of the process, emphasizing the tools and frameworks necessary for success. The focus will be on equipping developers and tech enthusiasts with the knowledge to navigate the complexities of the development lifecycle. The emphasis is placed on understanding the significance of planning, structured implementation, and effective deployment strategies.
By the end of this guide, readers will have a clearer picture of how to embark on building their own Python applications. Key concepts and practices will be examined in detail, offering substantive insights aimed at enriching your development journey.
This narrative weaves through theory and practical examples, ensuring a rounded understanding of what is involved in Python development. Let us begin with an overview that covers the essential aspects of software development, including the tools and technologies that can enhance your Python solutions.
Overview of Software Development Tools and Technologies
Software development encompasses a wide range of practices and tools that streamline the process of building applications. Various technologies serve distinct purposes in this domain. The depth of knowledge in specific tools can significantly impact the quality of applications produced.
Definition and Importance of Development Tools
Development tools refer to software programs that aid developers in creating applications efficiently. Their importance cannot be overstated. They help in automating repetitive tasks, debugging code, and enhancing productivity.
Key Features and Functionalities
Common features of development tools include:
- Code Editors: Provide syntax highlighting, code completion, and debugging capabilities.
- Version Control Systems: Essential for tracking changes in code, enabling collaboration among developers (e.g., Git).
- Testing Frameworks: Tools like PyTest or Unittest help ensure code quality through testing.
- Deployment Tools: Simplify the launch of applications on desired platforms (e.g., Heroku).
Use Cases and Benefits
The appropriate selection of development tools can lead to:
- Shorter development cycles.
- Improved code quality via built-in testing and integration.
- Better collaboration among team members.
Benefits extend beyond productivity; they enable developers to focus on designing more complex functionalities that cater to user needs.
"Choosing the right tools can be the difference between a successful project and a failed one."
Best Practices in Python Development
Knowing the right tools to use is crucial, but understanding how to use them effectively is equally important. Here are some industry best practices.
Implementing Best Practices
- Code Organization: Organizing code into modules and packages enhances readability and maintainability.
- Documentation: Thoroughly document code for clarity and future reference.
- Testing: Adopt a testing-first approach to facilitate bug detection early in the development cycle.
Tips for Maximizing Efficiency and Productivity
- Leverage integrated development environments like PyCharm or Visual Studio Code for streamlined coding.
- Use libraries and frameworks such as Flask or Django to speed up application development.
Common Pitfalls to Avoid
- Avoid prematurely optimizing code; focus on correctness before performance.
- Do not neglect version control. Consistent commits and branching can save significant hassles later.
With a solid understanding of these practices, developers can significantly enhance their effectiveness in Python application development.
Prologue to Python Application Development
The realm of Python application development is not just about writing code; it's about understanding the entire ecosystem of tools and methodologies that come into play. As more developers recognize Python's versatility, the importance of structuring this process cannot be understated. The initial planning phases set the stage for everything that follows. This article aims to provide clarity about the steps in creating a Python application, emphasizing the integration of industry best practices.
Understanding Python as a Programming Language
Python is a high-level programming language known for its simplicity and readability. This makes it an ideal choice for new programmers and seasoned developers alike. The language's syntax allows developers to express concepts in fewer lines of code compared to languages like Java or C++. This attribute alone greatly speeds up the process of development. Moreover, Python supports multiple programming paradigms, such as procedural, object-oriented, and functional programming. This flexibility expands its application suitability across various domains.
Its extensive library support is another key aspect. Python boasts a strong collection of frameworks and libraries that simplify complex tasks. Popular frameworks like Django and Flask facilitate web development, while libraries like NumPy and Pandas are fundamental to data science. This extensive support network ensures Python is equipped to handle numerous application types.
Furthermore, the language is maintained by a rich community of developers. This community contributes through forums, tutorials, and open-source projects, creating a vast repository of knowledge. For example, websites like reddit.com host lively discussions about Python development, sharing challenges and solutions.
Why Choose Python for Application Development
Python offers several compelling reasons for developers to choose it for application development. Firstly, the language’s simplicity and ease of learning allow for quicker onboarding of new team members. This is a significant advantage when scaling teams or adapting to new projects. Secondly, Python’s vast array of libraries and frameworks promotes rapid development and deployment, which can lead to reduced time-to-market for applications.
Additionally, Python is platform-independent. Code written in Python can run on various operating systems without requiring extensive modifications. This feature enhances the language's appeal for developers targeting diverse user groups.
The growing demand for Python professionals in fields like data science, machine learning, and web development highlights its relevance in today’s job market. According to sources like britannica.com, Python has become a staple in educational institutions, further solidifying its foundational role in software development.
Planning Your Python Application
Planning is a crucial phase in the development of a Python application. It allows developers to establish a clear vision, outline objectives, and identify potential challenges before diving into programming. Effective planning not only saves time during the coding process but also aligns the development efforts with the expectations of end-users or stakeholders. More specifically, this phase can significantly reduce the risk of project scope creep, ensuring the team can deliver what was agreed upon within the stipulated timeframe.
Defining Project Scope and Objectives
Defining project scope and objectives is the foundational step in planning. It involves establishing what the application will and will not encompass. A well-defined scope helps in setting realistic expectations for both the development team and stakeholders. Without it, projects can easily veer off course.
Start by gathering requirements from stakeholders. Conducting interviews or surveys can help gather insights into what users need. Once this information is collected, prioritize features based on user needs and technical feasibility. Formulate clear, measurable objectives that can guide development and provide direction throughout the project lifespan. For example, an objective could be to reduce user onboarding time by 50% through a more intuitive interface.
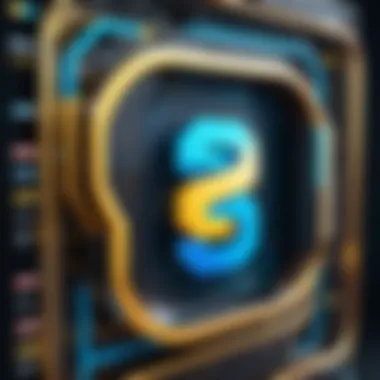
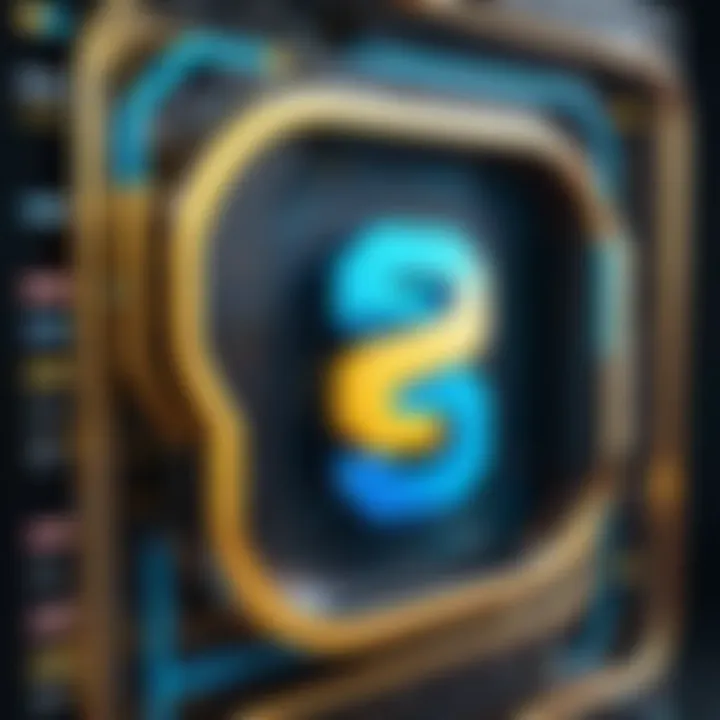
Ensure the project scope articulates boundaries. A document outlining what features will be included, and anything that will be excluded, can mitigate misunderstandings later. This scope document can act as a reference point throughout the development process, aligning the team with the project’s original intentions.
Identifying Target Users and Requirements
Identifying target users and their requirements is central to successful application development. Understanding who will ultimately use the software lays the groundwork for design and functionality decisions. Different user demographics can have varied needs, and recognizing these differences is key.
Create user personas to encapsulate the characteristics of your target demographics. Each persona should include details such as age, profession, tech proficiency, and specific goals they have for using the application. These profiles will inform design decisions—helping developers to align features with user expectations.
Moreover, during this phase, gathering requirements should be meticulous. This includes functional requirements, which describe what the application should do, and non-functional requirements, such as performance metrics and security standards. Engaging with end-users during this requirements-gathering process is invaluable. They can provide insights that are often missed by those not directly interacting with the solution on a daily basis.
This early involvement of target users can lead to a product that is more likely to meet real-world needs and avoid costly revisions later on.
Lastly, maintaining flexibility is essential. Requirements can evolve as development progresses. Using agile methodologies can assist in adapting to these changes without losing sight of the project’s overall objectives. Engaging regularly with stakeholders throughout the planning and development phases can also keep the team aligned with user needs and expectations.
Setting Up the Development Environment
Setting up the development environment is a crucial step in the process of building a Python application. A well-configured environment enhances productivity and establishes a foundation for effective development practices. This section will explore essential elements such as the selection of tools, installation of Python, and the integration of version control systems. Each of these aspects contributes significantly to overall project management and collaboration.
Choosing the Right Tools and IDEs
Choosing the right Integrated Development Environment (IDE) and tools is vital for streamlining the development process. Popular IDEs like PyCharm, Visual Studio Code, and Jupyter Notebook each offer unique features suited to different types of projects.
- PyCharm: This is an excellent choice for web development and data science due to its rich interface and powerful debugging tools.
- Visual Studio Code: Lightweight and highly extensible, making it suitable for general-purpose programming.
- Jupyter Notebook: Ideal for data analysis and visualization, favored among data scientists.
When selecting tools, consider the size of your project, the type of application you intend to build, and personal preferences. Ease of use, plugin ecosystem, and community support are also important factors. An appropriate IDE can significantly reduce the time spent on coding and debugging.
Installing Python and Necessary Libraries
The installation of Python must be done correctly to ensure that your application works smoothly. First, download the latest version from the official website. After installation, it is essential to set up a virtual environment. Using virtual environments allows you to manage dependencies effectively and avoid conflicts among packages. To create a virtual environment, use the following commands:
After activating the virtual environment, you can install necessary libraries using . Key libraries for Python application development may include Flask or Django for web applications, Pandas and NumPy for data analysis, and Requests for HTTP requests. This modularity is crucial for maintaining clean project architecture.
Version Control with Git
Implementing version control is an essential practice in any software development project, including Python applications. Git is the most widely used system for tracking changes and collaborating among teams. Setting up a Git repository allows developers to keep a history of changes, experiment with new features in branches, and revert to previous versions if necessary.
To initialize a new Git repository, follow this simple command:
Using Git effectively promotes collaboration and minimizes conflicts among team members.
Basic commands include , , and , which will be among the most frequently used as development progresses. Utilizing platforms like GitHub or Bitbucket enhances collaboration, offering an interface for managing repositories alongside powerful community tools.
Designing the Application Architecture
Designing the application architecture is a crucial step in the development process. This phase defines how the various components of an application will interact with each other. A well-structured architecture enhances scalability, maintainability, and overall performance. Without proper planning at this stage, developers may face significant challenges later, such as difficulties in implementing new features, debugging issues or managing the codebase.
Good architecture allows for a clear separation of concerns. Teams can focus on specific aspects of the application without affecting others. It can also aid in identifying the optimal tools and technologies to use. This leads to informed decisions regarding databases, libraries, and frameworks, each chosen for their compatibility with the selected architectural style.
Choosing the right architecture impacts both short-term and long-term objectives. Different use cases and expected application behavior may favor different architectural choices. The next step is critical: the decision between a monolithic structure and a microservices architecture.
Choosing Between Monolithic and Microservices Architecture
Making a choice between a monolithic architecture and microservices is an important consideration. A monolithic application is a single, unified unit where components are interconnected. This can simplify development and deployment. However, as the application grows, it can become more challenging to manage dependencies and scale.
On the other hand, microservices architecture comprises small, independent services. These services can communicate with each other through APIs. This offers flexibility in technology choices for each service and allows teams to work on services concurrently, leading to faster delivery. Yet, it introduces complexity in deployment, communication, and management.
When deciding between these two architectures, consider:
- Scalability needs: How much do you expect the application to grow?
- Team structure: Do you have the resources to manage a microservice setup?
- Development speed: What is more critical in your development cycle?
Ultimately, both approaches have their merits. A careful analysis of the project requirements will lead to a more suitable choice.
Implementing or Patterns
Many developers choose architectural patterns such as Model-View-Controller (MVC) or Model-View-ViewModel (MVVM) to streamline their applications. Using these patterns ensures a clear division of responsibilities within the codebase.
In the MVC pattern, the model manages data and business logic while the view renders the presentation. The controller acts as an intermediary. This structure is beneficial in large applications where separation helps to reduce tight coupling.
MVVM, on the other hand, is a more modern approach. It facilitates two-way data binding between the view and the view model. This reduces the amount of code needed for UI logic and provides a more straightforward framework for developing applications with complex user interactions.
Considerations for implementing these patterns include:
- Application size and complexity: Which pattern will better support your growth?
- Team familiarity: Does your team have experience with MVC or MVVM?
- Framework compatibility: Is your chosen framework optimized for either pattern?
By selecting the appropriate architecture and design patterns, developers can create maintainable and scalable applications. Proper design choices set the foundation for a successful development process.
Developing the Application
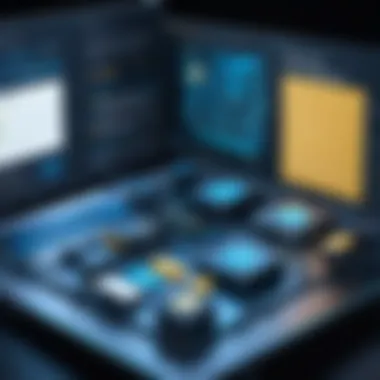
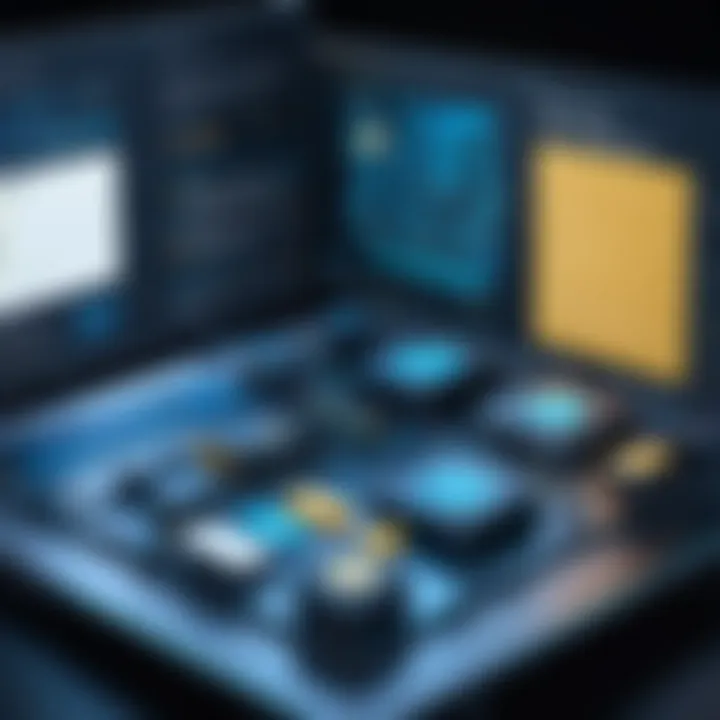
The development stage is where your initial ideas and plans begin to take form. This phase is crucial for translating requirements into functional software. By focusing on how the application actually works, developers can ensure that they adhere to design principles while keeping efficiency in their coding practices.
Good development practices are essential. They reduce both technical debt and future complications. Furthermore, writing clean code enhances the maintainability of the application, making it simpler for future team members to understand the work done. Upfront efficiency saves time in the long run. A well-structured development approach also improves collaboration among team members.
Writing Clean and Efficient Code
Writing clean and efficient code is not just a best practice; it is a necessity. Clean code improves readability, making it easier for others to follow and maintain the work. It allows for better collaboration on team projects. Efficient code runs faster and consumes fewer resources, which is particularly important in resource-constrained environments.
There are several standards that can guide you in writing clean code. Names of variables and functions should be descriptive and follow a consistent naming convention. Additionally, modular design separates concerns. This means each module should do one thing well. Commenting on your code is also important but should be done judiciously—it should clarify the intent of complex sections rather than cluttering the code.
Key Points for Clean Code:
- Use meaningful variable names
- Keep functions short and focused
- Follow conventions for formatting
- Comment where necessary, avoid over-commenting
Integrating Libraries and Frameworks
The integration of libraries and frameworks is a powerful aspect of Python application development. Python's ecosystem is rich with libraries that can significantly speed up the development process. Whether for data processing, web development, or machine learning, there is likely a library that can simplify your task.
Before selecting libraries, assess their community support. Libraries that are actively maintained are less likely to become obsolete. Do also consider compatibility with the Python version you are using.
Examples of Popular Libraries:
- Flask or Django for web development
- NumPy and Pandas for data analysis
When integrating libraries, use virtual environments to manage dependencies effectively. This prevents conflicts that may arise from different projects requiring different versions of libraries.
Error Handling and Debugging Techniques
Error handling and debugging are integral parts of development. It is vital to identify potential errors early in the development process. This can be achieved by implementing error handling techniques that enhance your application's reliability.
Python provides mechanisms such as try-except blocks for catching exceptions. This keeps your application running even if an error occurs. Moreover, logging is crucial. By logging errors, you can review what went wrong and address the underlying issues.
Debugging tools, such as Python’s pdb or IDE-specific debuggers, allow you to step through your code. They help in identifying where things might not be behaving as expected.
Error Handling Strategies:
- Use try-except blocks to catch exceptions
- Implement logging to keep track of errors
- Utilize debuggers for in-depth analysis
Good error handling anticipates issues and helps applications behave predictably.
Overall, the development stage is a critical juncture in the application lifecycle. By focusing on writing clean code, integrating the right libraries, and having effective error handling, developers set the groundwork for a robust and reliable application.
Testing Your Python Application
Testing is a critical part of developing a robust Python application. Ensuring that the application behaves as expected not only minimizes errors but also builds confidence in the code. Testing can prevent future regressions during updates, helping to maintain application integrity over its lifecycle. Furthermore, it allows for smoother integration in larger systems where multiple components interact.
Beyond just basic verification, testing reveals insights into application performance and usability. Identifying bugs early can save developers significant time and resources. Additionally, a well-tested application is easier to maintain, as adjustments or enhancements can be made with less fear of breaking existing functionality. Thus, testing should not be viewed as an ancillary step, but an integral component of the development process.
Unit Testing and Integration Testing
Unit testing focuses on verifying each individual component of the application. A unit test checks the smallest parts of the codebase in isolation. This could include functions or methods. By running unit tests, developers can identify issues at a granular level. They can adjust specific pieces of code without needing to examine the entire system.
Integration testing ensures that different components of the application work together as intended. Once individual units pass their tests, integration testing becomes necessary. It assesses how well these units interact when combined. Issues that arise from component interactions may not be evident during unit testing. Detecting these potential problems in the testing phase can significantly streamline debug processes later.
Implementing both unit testing and integration testing can provide a comprehensive safety net. It builds towards not just a functioning application, but one that also fulfills user expectations consistently.
Using Testing Frameworks
Testing frameworks can simplify the tedious process of writing and maintaining tests. In Python, frameworks like unittest, pytest, and nose2 offer structured ways to create, manage, and execute tests. These tools provide features like test discovery, assertions, and easy reporting.
For example, pytest supports fixtures which helps in setting up initial conditions for running tests, allowing for more manageable testing sessions. Here’s a simple example of using pytest for a function that adds two numbers:
In this test, the function add is defined, and then various assertions during testing validate its expected behavior. If the assertions fail, pytest will provide feedback, making it easier to troubleshoot.
Additionally, reporting features of these frameworks keep track of what tests passed or failed, contributing to better understanding the code’s stability. This feedback loop creates an environment conducive to continual improvement, essential for the longevity of an application.
"A well-tested application is not just resilient but poised for evolution."
Deployment Strategies
In the context of Python application development, deployment strategies play a crucial role in ensuring that the software reaches its end users effectively. Successful deployment aids in smooth user experience and minimizes downtime and potential issues. A well-structured deployment strategy not only encompasses the technical processes but also strategic planning that aligns with business objectives. In this section, we will explore key elements related to deployment strategies, including preparation, hosting solutions, and CI/CD practices.
Preparing for Deployment
Preparation is a vital phase in the deployment process. This stage involves validating the application's readiness and determining the necessary resources required for a successful launch. Key aspects to consider include:
- Documentation: Ensure that all necessary documentation, such as setup guides and user manuals, is clear and accessible. This helps both developers and users.
- Environment Configuration: Configure environments properly for production. This includes verifying server setup, ensuring security measures are in place, and matching production configurations with those from development and testing.
- Testing: Conduct rigorous testing before deployment. This includes not only functional testing but also performance testing and acceptance testing to ensure overall application quality.
- Backup Plans: Develop a rollback plan in case deployment encounters any issues. Having a backup enables quick recovery, minimizing disruption for users.
Choosing a Hosting Solution
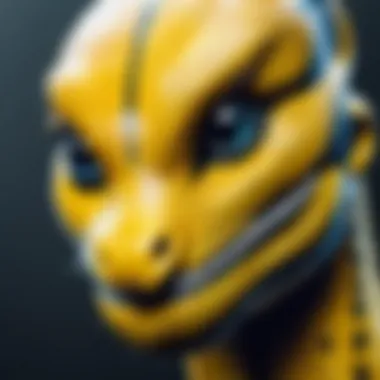
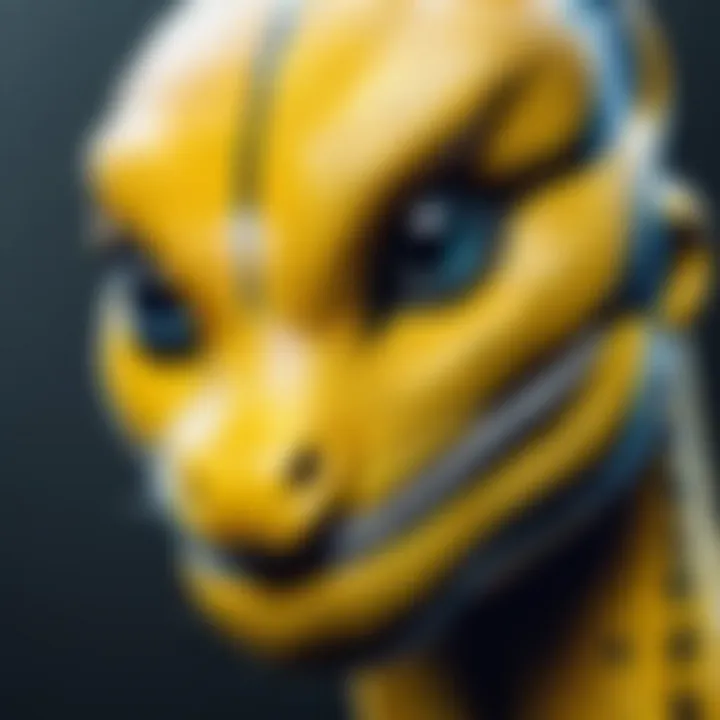
Selecting the right hosting solution can greatly influence the performance and scalability of your application. Various options are available, each with its advantages and disadvantages:
- Cloud Hosting: Offers flexibility and scalability and is suitable for applications expecting variable traffic patterns. Platforms like Amazon Web Services and Google Cloud are popular choices.
- Dedicated Hosting: Provides full control over the server environment. This option is beneficial for applications with high resource requirements but can be more expensive.
- Shared Hosting: A cost-effective solution for small applications. However, sharing resources with other applications may impact performance.
- Platform as a Service (PaaS): Such as Heroku or Azure App Service, simplifies the deployment process by managing infrastructure and scaling automatically.
Consider factors like budget, expected traffic, and necessary performance when making your choice.
Continuous Integration and Continuous Deployment (/)
Implementing CI/CD practices is essential for modern application development, as it streamlines deployment and ensures code quality. CI/CD facilitates frequent updates and reduces the risk of introducing bugs during deployment. Key points to understand include:
- Continuous Integration: This involves automatically integrating code changes into a shared repository frequently. It aids in finding and fixing bugs quickly.
- Continuous Deployment: Closely follows CI, where the latest code changes are automatically deployed to production after passing tests. This approach minimizes manual intervention and enhances release cycles.
"CI/CD allows developers to release software updates more reliably and frequently, catering to users' needs effectively."
Optimizing Application Performance
Optimizing application performance is essential for ensuring that a Python application runs efficiently and meets user expectations. A well-optimized application can reduce load times, enhance responsiveness, and ultimately deliver a better user experience. Various elements contribute to application performance, including memory usage, response time, and CPU load. Focusing on these aspects allows developers to identify areas for improvement and implement effective strategies.
For developers and IT professionals, understanding performance optimization is not just a technical requirement; it is crucial for maintaining user engagement and satisfaction. When applications lag or perform poorly, users can become frustrated, potentially leading to decreased usage or abandonment. Furthermore, search engine rankings can also be indirectly influenced by performance metrics, making optimization a multifaceted concern.
Profiling and Testing for Performance Bottlenecks
Profiling is a crucial step in identifying performance bottlenecks in your application. By using profiling tools, developers can monitor application execution and identify which parts consume the most resources. For Python, tools like cProfile and Py-Spy are popular choices that provide detailed insights into function call times and resource usage.
To effectively profile an application, follow these steps:
- Select a Profiling Tool: Choose an appropriate profiling tool that fits the project’s needs, such as cProfile for detailed statistics.
- Profile Your Code: Run your application with the profiler enabled to gather data on performance.
- Analyze the Results: Identify functions or methods that are taking more time or resources than expected.
- Optimize the Code: Refactor the identified sections for better performance.
- Re-Test: After making changes, profile again to ensure that optimizations have made an impact.
"Profiling provides the insight necessary to understand where the inefficiencies lie and enables targeted optimization efforts."
Implementing Caching Strategies
Caching is a powerful method to enhance application performance by storing previously computed results for immediate retrieval. This reduces the need for redundant processing and speeds up response times drastically. Various caching strategies can be implemented depending on the application requirements.
Some effective caching strategies include:
- In-Memory Caching: Store data in memory (e.g., using Redis or Memcached) to allow rapid access.
- Database Query Caching: Cache the results of expensive database queries to minimize redundant access to the database.
- File Caching: Store frequently requested file assets to reduce load times for static content.
When implementing caching, consider the following:
- Cache Expiry: Define how long cached data remains valid before needing an update.
- Cache Size: Monitor cache size to prevent allocation of excessive memory.
- Invalidation Strategy: Develop a strategy for cache invalidation when underlying data changes.
Employing a caching strategy can optimize performance significantly when applications face high demand or load. Assessing the system's needs and user patterns will ensure that caching yields the best results.
Maintaining and Updating the Application
Maintaining and updating a Python application is crucial for its longevity and relevance. As technology evolves, so do user expectations and security needs. Regular maintenance ensures that the application remains functional and efficient. Not addressing these aspects can lead to performance degradation and user dissatisfaction.
Key aspects that underscore the importance of maintaining and updating applications include:
- Performance Optimization: Over time, code can become less efficient due to various factors like increased data volume or user interaction. Regular updates help optimize performance, ensuring a smoother user experience.
- Security Enhancements: Cyber threats are constantly evolving. Regular updates patch vulnerabilities in the software to safeguard sensitive data and protect against attacks.
- Feature Improvement: Users may require new features or enhancements. Regular updates provide an opportunity to integrate new functionalities that keep the application competitive and aligned with user needs.
- Compliance with Standards: Changes in regulations or standards may require updates to maintain compliance. This can be especially important in sectors such as finance or healthcare.
With these points in mind, it is vital for developers to adopt a systematic approach to maintain and update their applications.
Version Management and Updates
Version management is a structured approach to managing changes and ensuring the application evolves without loss of functionality or data integrity. It plays an essential role in maintaining a clear development timeline while facilitating rollback capabilities in case new updates need to be reverted.
Using tools like Git is essential for effective version control. Git allows for the tracking of changes in code, making collaboration easier among team members. It enables branching, allowing developers to work on features separately without interfering with the main codebase.
In addition to using version control systems, it is crucial to establish a clear update policy. This policy should outline the frequency of updates, types of updates (bug fixes, feature additions), and a communication plan for users regarding these changes.
"A well-structured version management process can significantly reduce the chances of introducing bugs during updates."
Monitoring Application Health and Performance
Monitoring the health and performance of an application is vital for identifying potential issues before they escalate. Continuous monitoring helps in detecting bottlenecks, broken functionalities, or anything that might hinder user experience.
To ensure effective monitoring, consider incorporating the following practices:
- Establish Metrics: Set clear performance indicators such as load times, response times, and error rates. This allows for a quantitative assessment of the application's performance.
- Automate Monitoring: Use tools like Prometheus or Grafana to automate monitoring tasks. These tools can provide real-time insights into application performance.
- User Feedback: Encourage user feedback to identify areas needing improvement. Surveys or feedback forms can play a crucial role in understanding the user experience.
- Log Management: Implement systematic logging to capture errors or critical actions within the application. Analyzing these logs can provide insights into common issues and inform future developments or updates.
Maintaining a robust monitoring system is essential for the continued success of the application, enhancing user satisfaction, and longevity of the software.
Culmination
The conclusion of this guide serves as a crucial component in summarizing the entire journey of Python application development. It synthesizes key points, reinforcing the importance of each stage covered in previous sections. Readers can gain a comprehensive outlook on how all the aspects interconnect to create a successful application.
Recapping Key Takeaways
- Holistic Understanding: Each phase, from planning to deployment, is essential. Without proper planning, one may encounter issues during development and deployment, leading to wasted time and resources.
- Choosing the Right Tools: The significance of selecting appropriate tools and frameworks cannot be overstated. A well-chosen IDE or library can streamline the development process significantly.
- Emphasis on Testing: Testing is not just an afterthought but a fundamental part of development that ensures the application performs as intended without bugs.
- Deployment Strategies: Understanding different hosting solutions and deployment strategies enables developers to choose the best options for their applications.
- Continuous Maintenance: Maintenance ensures the application remains relevant and efficient over time. Regular updates and monitoring are critical to achieving this.
Future Trends in Python Development
As Python continues to evolve, several trends are emerging. Developers need to stay informed about potential shifts in technology and application usage.
- Increased Use of AI and Machine Learning: Python is already a leading language in AI development, and this trend will likely grow. Expect more libraries and frameworks tailored for machine learning tasks.
- Focus on Web Development Frameworks: Libraries like Django and Flask are gaining popularity, leading to refined tools and methodologies that simplify web application development.
- Greater Emphasis on Security: With the increase in data breaches, security practices are becoming a necessary part of the development process. Developers will need to incorporate security protocols within their frameworks.
- Community Contributions: Open-source projects will continue to thrive, offering new modules and enhancements that developers can integrate into their applications quickly.
- Sustainable Coding Practices: There will be a push for writing code that not only functions efficiently but also minimizes resource consumption and supports sustainable computing.
The conclusion encapsulates the entire developmental cycle. It highlights the benefits the reader can reap from the information presented and points toward future trends that will shape the landscape of Python development.