Designing Java Applications: Best Practices and Frameworks
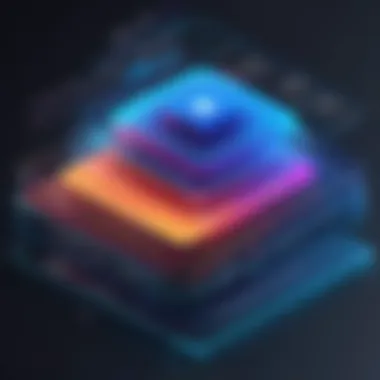
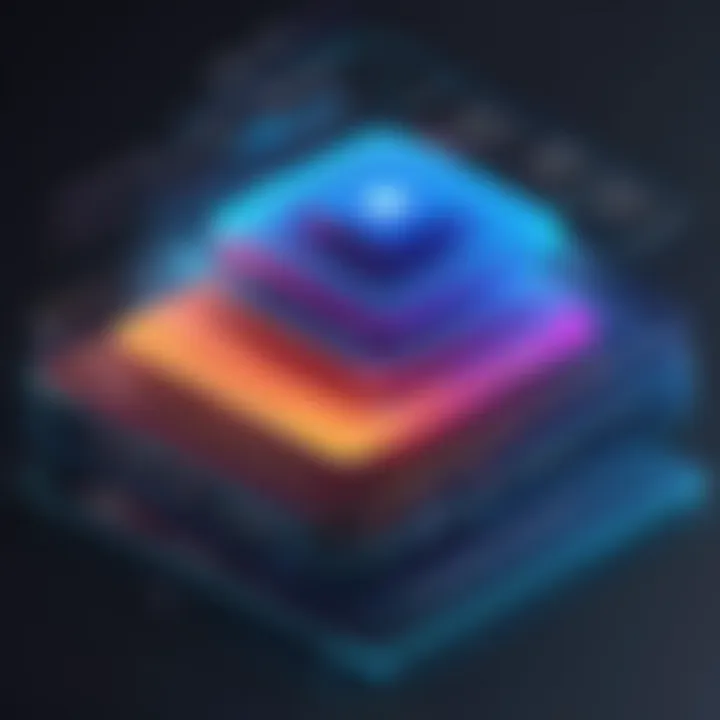
Intro
In the ever-evolving world of software development, Java remains a steadfast pillar, integral to countless applications across various sectors. The journey of designing applications through Java requires not only an understanding of the language but also of the underlying methodologies and architectural principles that can make or break a project. This article seeks to illuminate the terrain of Java application design, focusing on strategies that promote scalability, maintainability, and robustness. By examining industry best practices, common pitfalls, and performance optimization techniques, developers and IT professionals can deepen their mastery of Java, honing their skills to create top-notch applications.
While there are many languages vying for attention, Java's versatile nature, backed by a rich ecosystem of tools and libraries, ensures it remains relevant. It’s robust, platform-independent, and features a vibrant community that continually contributes to its growth. Whether you’re stepping into the world of Java for the first time or looking to refine your expertise, understanding how to design an application effectively sets the foundation for success.
Next, we will explore the expansive overview of software development as it relates to Java, diving into its significance in the modern tech landscape.
Overview of Software Development
At its core, software development is about transforming ideas into functional pieces of software that solve real-world problems. It encompasses a series of methodological steps, from initial concept through to deployment, often defined by specific frameworks that guide the process. Understanding these frameworks and their impact on various aspects of development can immensely improve the output quality.
Definition and Importance
Software development is the art and science of building computer programs. Its importance can’t be overstated; without it, our digital world would simply not exist. In the context of Java, successful software development allows the creation of powerful applications that are safe, efficient, and tailored to user needs.
Key Features and Functionalities
- Cross-Platform Capability - Java's mantra, "write once, run anywhere," exemplifies its ability to operate seamlessly across diverse environments, reducing testing time and enhancing user experience.
- Robust Security Measures - Built with security in mind, Java applications leverage a rich set of APIs that protect users and data, making it a favorite in enterprise settings.
- Rich Ecosystem - Libraries and frameworks, such as Spring and Hibernate, facilitate the development process, providing pre-built components that address common development challenges.
Use Cases and Benefits
Java applications span a gamut of industries. Here’s a glimpse at some common use cases:
- Enterprise Applications - Large corporations utilize Java for managing their complex systems, ensuring stability and reliability.
- Mobile Development - Android's application framework is built on Java, promoting the rapid growth of mobile apps.
- Big Data Technologies - Many big data frameworks, like Apache Hadoop, utilize Java for their core components.
The benefits are clear: robust performance, a comprehensive library framework, and a large talent pool of Java developers.
Best Practices
When embarking on Java application design, adhering to industry-best practices can position developers for success. Here are several tips to enhance efficiency and productivity:
- Code Reusability - This is crucial for maintaining manageable codebases; always aim to write modular and reusable code.
- Continuous Integration - Implement CI/CD pipelines to automate testing and deployment — it streamlines processes and uncovers issues early.
- Design Patterns - Familiarize yourself with design patterns like Model-View-Controller (MVC) or Singleton to provide proven solutions to common problems.
However, there are pitfalls that should be avoided:
- Neglecting Documentation - Proper docs can save hours of confusion and improve team collaboration.
- Hardcoding Values - Keep configurations separate to avoid bottlenecks when adjustments are necessary.
Finale
As we delve further into the realm of designing Java applications, there’s a wealth of knowledge to explore. There’s much to gain from understanding both foundational principles and emerging trends in this dynamic field. From tackling comprehensive case studies to revealing the latest innovations, each aspect enriches our perspective and enhances our capabilities in Java development. Stay tuned for more insights and expertise as we navigate through the landscape of software design.
Understanding Java Application Design
Java application design is a cornerstone of successful software development that can't be overlooked. It's not just about writing code; it's about how the code interacts, functions, and evolves over time. This section dives into the essence of what designing Java applications entails. It’s crucial for developers and IT professionals to grasp the core principles and practices that guide effective Java application design. This understanding ultimately enables the creation of software that is not only functional but also efficient and easy to maintain.
Defining Java Application Design
Java application design encompasses the strategies, practices, and methodologies employed when creating software applications using the Java programming language. It includes determining the structure of the application, choosing the right architectural style, and creating a seamless user experience. A well-defined design process lays a solid foundation, ensuring that the application will perform reliably under various conditions.
Some specific aspects of Java application design include:
- Architecture: Understanding how various layers interact, like presentation, business logic, and data access layers.
- Scalability: Designing applications that can grow with user demand.
- Maintainability: Structuring code in a way that makes future modifications straightforward and less error-prone.
In this light, the significance of a thoughtful design process becomes evident. It reduces the risk of encountering problems down the line and fosters a more organized approach to development.
Importance of Effective Design
Effective design in Java applications is not merely a luxury; it’s a necessity. When developers get it right, the benefits are numerous. Understanding the necessity of good design comes with various considerations such as long-term maintainability, ease of collaboration, and performance efficiency.
"A stitch in time saves nine; it’s better to invest time in design now than to face complex refactoring later."
Some notable advantages of effective Java application design include:
- Improved Code Quality: A structured approach leads to cleaner, more understandable code.
- Enhanced Performance: Thoughtful design decisions can significantly improve application speed and responsiveness.
- Ease of Maintenance: Applications designed with maintenance in mind are simpler to update, thus prolonging their lifecycle.
- User Satisfaction: A well-designed application meets user expectations more effectively, which leads to positive feedback and higher adoption rates.
In summary, understanding Java application design is the bedrock of developing robust and efficient software. Targeted attention to architectural choices and the design approach lays the groundwork for successful project outcomes, resonating well with both users and developers alike.
Key Principles of Software Design
In the realm of Java application development, certain guiding principles stand as the bedrock upon which effective software is constructed. Understanding these principles is vital for any developer looking to enhance their craft. They do not merely serve as rules; rather, they act as a compass that directs the development process towards clean, maintainable code. Adhering to these principles can yield significant benefits—not only in the quality of the software but also in the team dynamics and efficiency during the project lifecycle.
When it comes to software design, a few principles rise to the top of the heap. These principles help structure code in a way that promotes flexibility and reusability, two characteristics integral to successful applications.
SOLID Principles
The SOLID principles consist of five design concepts that facilitate the development of maintainable and understandable software. The acronym SOLID comes from:
- S – Single Responsibility Principle (SRP): A class should have only one reason to change. This principle emphasizes that each class should focus on a single task, leading to clearer and more understandable code.
- O – Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification. By adhering to this principle, developers can introduce new functionalities without altering existing code, which increases stability.
- L – Liskov Substitution Principle (LSP): If a program is using a base class, it must be able to substitute any derived class without affecting the correctness of the program. It reinforces the ideas of inheritance and polymorphism, ensuring that subclasses can stand in for their superclass seamlessly.
- I – Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use. This helps avoid bloated interfaces by breaking them into smaller, more specific ones, which improves usability.
- D – Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules; both should depend on abstractions. This principle encourages the use of interfaces rather than concrete implementations, reducing coupling and promoting flexibility in code.
These SOLID principles, when applied appropriately, create a harmonious ecosystem within the codebase. They make it feasible for developers to understand code written by others and adapt it as needed, thus easing teamwork and reducing the learning curve for new team members.
The discipline of following SOLID principles transforms chaotic code into a well-orchestrated symphony of classes, with each playing its part skillfully.
DRY and KISS Principles
In addition to SOLID, two more principles deserve special mention: the DRY (Don't Repeat Yourself) and KISS (Keep It Simple, Stupid) principles.
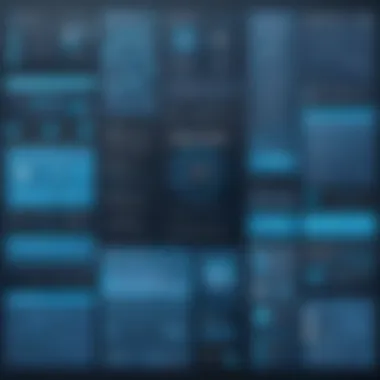
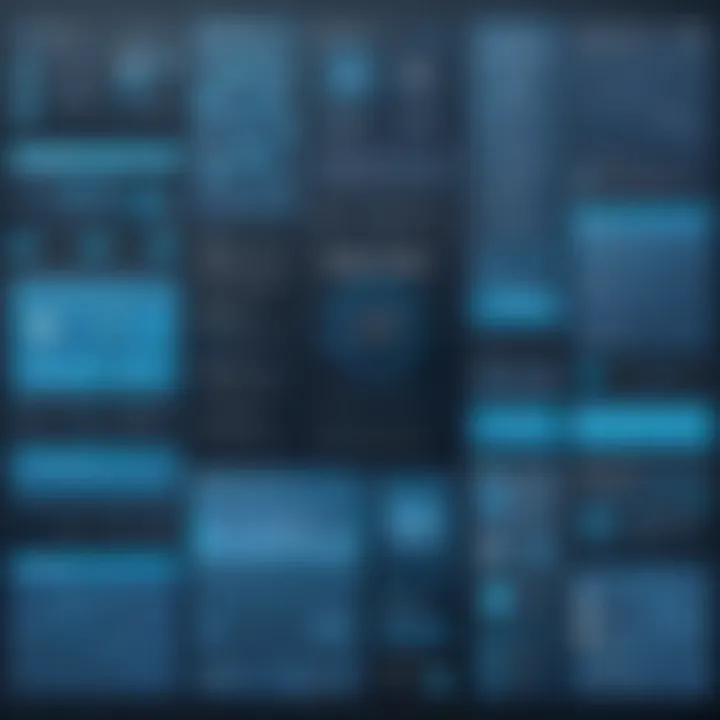
- DRY Principle: The essence of the DRY principle is simple: duplication is the enemy of a solid application. By ensuring that any piece of knowledge is represented within the system in a single, unambiguous way, developers can avoid errors and inconsistencies. It also fosters a clean codebase. If there's a need to change a method or a class, having it centralized means changing one spot instead of multiple ones.
- KISS Principle: Often hailed as a lifeline for developers, the KISS principle reminds us not to overthink our designs. Complexity can lead to errors and increased maintenance costs, so keeping designs intuitive and straightforward is key. This principle advocates for writing code in a way that even someone new to the project can grasp its purpose and function quickly. At its core, KISS promotes simplicity over complexity, ensuring that the code serves its purpose without unnecessary fluff.
In summary, the key principles of software design—SOLID, DRY, and KISS—serve not just as mnemonic devices but as foundational tenets that can lead to cleaner, more maintainable, and effective Java applications. Integrating these principles into daily coding practices will undoubtedly result in better software and a more proficient team.
Architectural Patterns in Java
Architectural patterns are fundamental blueprints that guide the structural organization of software systems. In the realm of Java applications, these patterns lay the groundwork for how components interact, promoting efficiency and scalability. By leveraging architectural patterns, developers can address complexity, improve code manageability, and enhance overall system performance.
When it comes to designing Java applications, embracing architectural patterns can result in many benefits:
- Separation of Concerns: By clearly defining roles and responsibilities within the application layers, developers can streamline maintenance and upgrades.
- Enhanced Scalability: Architectural patterns allow applications to be built in a way that supports growth seamlessly. As requirements change, patterns like microservices enable teams to scale individual components without a complete overhaul.
- Improved Team Collaboration: Utilizing standardized design patterns fosters a language among team members, reducing miscommunication and increasing overall productivity.
Overall, architectural patterns serve as a roadmap for developers, guiding the design process towards greater robustness and effectiveness. In this section, we’ll explore three prominent architectural patterns that hold particular relevance in Java: layered architecture, microservices architecture, and event-driven architecture.
Layered Architecture
Layered architecture is one of the most traditional designs in software engineering. This pattern organizes code into distinct layers that interact with each other according to well-defined rules. Typical layers include the presentation layer, business logic layer, and data access layer. Each layer has its own responsibilities and knowledge requirements.
The benefits of a layered architecture are numerous:
- Modularity: Each layer can be modified without affecting others, which aids in isolating changes, debugging, and testing. This modularity makes the overall application more manageable.
- Clear Division of Responsibilities: By compartmentalizing different functionalities, layered architecture provides clarity. Developers can focus on a specific layer without worrying about cross-layer dependencies.
An example implementation could look like this in pseudo-code:
In this example, you can see how responsibilities are neatly divided across the layers. The presentation layer takes care of UI logic, while the business logic and data access layers handle processing and data interactions, respectively.
Microservices Architecture
In contrast to traditional layered architecture, microservices architecture structures applications as a collection of loosely coupled services. Each service focuses on a specific business function and communicates with others through APIs. This approach is incredibly advantageous for developing large-scale applications that require frequent updates.
Some key advantages of microservices include:
- Independent Deployments: Teams can deploy different services independently of one another. This capability significantly reduces downtime and speeds up the release process.
- Technology Diversity: Each microservice can utilize different programming languages or technologies, permitting developers to choose the best fit for specific tasks.
Consider a company that handles e-commerce. They might break their application into specialized microservices like:
- User Management Service
- Product Catalog Service
- Order Processing Service
This granularity means that if the order processing service needs updates, the entire application won’t go down; only that specific service will be affected. Overall, microservices architectural patterns can lead to enhanced agility and robustness in Java applications.
Event-Driven Architecture
Event-driven architecture (EDA) stands out as a highly flexible design paradigm that reacts to events or changes in state. In an event-driven setup, components communicate via event notifications rather than direct calls. This decoupling allows for more responsiveness and adaptability in complex systems.
Here’s why event-driven architecture is gaining traction in Java application design:
- Asynchronous Communication: With events, different parts of the system can operate independently, enhancing speed and efficiency when doing tasks.
- Better Scalability: When the demand increases, systems can easily ingest and process a higher volume of events without overstressing on specific components.
With effective use of event-driven architecture, an application can respond to a surge in user activity without breaking a sweat.
Java frameworks such as Spring Cloud Stream provide the tools necessary to establish event-driven solutions in Java. The application reacts to events efficiently, which lends well to scenarios like dynamic user interactions or extensive data processing.
Common Design Patterns in Java
In the realm of software development, design patterns are fundamental. They offer tried and true solutions to recurring problems that developers encounter. The topic of common design patterns in Java merits a thorough exploration because these structures not only foster cleaner, more efficient code, but they also enable the team to collaborate seamlessly across a variety of projects.
Design patterns serve as blueprints for developing scalable applications. They enhance code reusability and maintainability, making it easier to implement changes without unraveling the entire application. In Java, where its object-oriented nature allows for encapsulation, inheritance, and polymorphism, understanding these patterns can mean the difference between a hastily constructed application and a well-architected software solution.
Let's delve deeper into the three main types of design patterns: creational, structural, and behavioral.
Creational Patterns
Creational patterns deal primarily with object creation mechanisms. They help ensure that your code has the right objects at the right time, avoiding needless instantiation and memory consumption. One well-known creational pattern is the Singleton pattern. This pattern guarantees that a class has only one instance and provides a global point of access to it. Suppose you're building a logging framework; employing the Singleton pattern would streamline this. With only a single logger for the entire application, you can control logging in a more organized way.
Key Advantages of Creational Patterns:
- Controlled Instantiation: Manage object creation.
- Flexibility: Change your architecture without major changes to your code.
- Configuration: Create objects in a centralized manner.
Structural Patterns
Structural patterns are concerned with how objects and classes are composed to form larger structures. These patterns facilitate the organization of classes and objects into a flexible structure that's easy to manage. Take the Adapter pattern for example. It allows incompatible interfaces to work together. Imagine you’re integrating a new service that requires a specific interface but you have an existing codebase. Instead of rewriting the entire code, an adapter can bridge the gap.
Key Structural Patterns:
- Facade: Abstracts a complex system behind a simpler interface.
- Decorator: Adds behavior to individual objects without affecting other instances of the same class.
Some Benefits of Using Structural Patterns:
- Simplified Interfaces: Ease user interactions with complex systems.
- Enhanced Flexibility: Makes it easier to extend functionality.
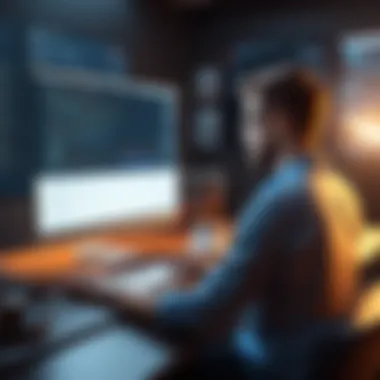
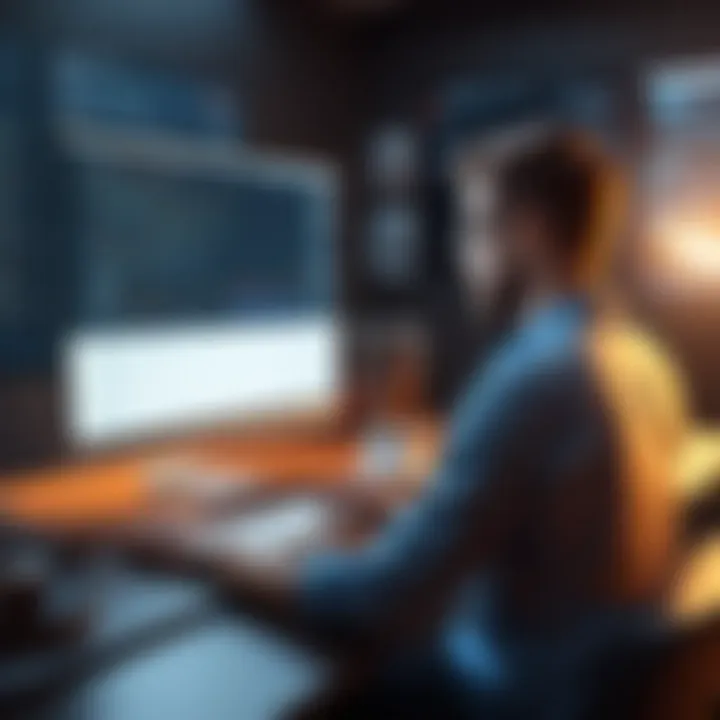
Behavioral Patterns
Behavioral patterns focus on communication between objects, emphasizing how they interact and operate together. The Observer pattern exemplifies this well. It allows one object to notify a list of dependents of changes without needing to know who those observers are. This is particularly advantageous in event-driven systems, such as monitoring user actions in a mobile application.
Benefits of Behavioral Patterns include:
- Loose Coupling: Reduces dependencies between objects.
- Improved Communication: Facilitates complex workflows.
In summary, understanding these common design patterns can elevate a developer's ability to create robust and maintainable Java applications. They pave the way for software design that is both efficient and adaptable, essential for tackling modern technological challenges.
Overall, incorporating design patterns not only enhances your programming toolbox but significantly improves the architecture of your software solutions.
Best Practices for Java Application Design
Designing Java applications with finesse goes beyond merely getting the code to run; it's about crafting an ecosystem where code can evolve effortlessly over time. Best practices in Java application design provide a framework that can guide software developers and IT professionals through the convoluted landscape of application development. When these practices are implemented effectively, they echo not just in the current codebase but can reverberate throughout the entire lifecycle of the application, ensuring that it remains maintainable, scalable, and, most importantly, intelligible.
The advantages of adhering to best practices are manifold:
- Improved Readability: When code is easily readable, it becomes simpler for team members to contribute. Clear code reduces the learning curve for new developers joining a project.
- Ease of Maintenance: Following established protocols makes fixing bugs and implementing new features more straightforward, leading to a more robust application.
- Consistency: A uniform approach to coding helps in maintaining a predictable structure across the application. It fosters team collaboration, whereby every developer speaks the same language, so to say.
- Time Efficiency: By eliminating rework and confusion, fluent application design practices can save teams valuable time, allowing them to focus on meaningful features rather than wrestling with technical debt.
Reflecting on these benefits, it's evident that the implementation of best practices isn't just about writing good code; it's about ensuring longevity and interdisciplinary collaboration across the software development lifecycle.
Code Readability and Maintenance
Code readability isn’t just a fancy term tossed around by instructors; it's a crucial aspect that can make or break a project. If the code resembles a foreign language to the developer tasked with keeping it alive, the risk of pitfalls increases significantly. To improve readability, developers can employ several strategies:
- Meaningful Variable Names: Clear naming conventions go a long way. For example, is more descriptive than .
- Consistent Formatting: Following a consistent code style improves readability. Javadoc comments should be utilized to document public methods and classes adequately. A simple line break can clarify logical blocks.
- Avoidance of Complex Logic: When logic becomes convoluted, consider splitting it into smaller, manageable methods. A method should ideally do one thing and do it well.
Keeping code readable greatly enhances its maintainability, which is no small feat. Maintenance mainly involves bug fixes, feature enhancements, and ensuring Code adheres to current best practices. A maintainable codebase ensures efficient iterations without substantial overhead.
Version Control and Collaboration
Modern Java development environments would be unthinkable without version control systems. Tools like Git enable teams to collaborate without stepping on each other's toes. Version control provides a plethora of benefits:
- History Tracking: Keeping a record of changes can be invaluable. If a bug slips through due to a change, it’s easier to trace back to the origin of the issue.
- Branching and Merging: Branching allows developers to work on features in isolation, facilitating smoother integration when the feature is complete.
- Collaboration: With version control, team members can work concurrently, irrespective of geographical boundaries. It fosters a collaborative spirit that is essential for agile development.
However, effective version control goes beyond these tools; it involves establishing clear protocols. Regular check-ins, code reviews, and proper documentation are integral to maximizing the benefits of version control strategies. In doing so, teams can reduce friction during collaborations and streamline the development process.
The practice of continual improvement forms the backbone of successful Java application design, encapsulating both state-of-the-art technology use and a profound respect for code history.
By adhering to best practices for Java application design, developers are not merely enhancing the functionality of their projects; they are embedding a mindset geared towards ongoing growth and excellence. The practices outlined here foster an environment conducive to innovation and rigor, ensuring that Java applications thrive in a fast-evolving technological landscape.
Performance Considerations
In the realm of software development, performance can often make or break a Java application. The nuances in how an application performs can dictate user satisfaction and system responsiveness. Thus, performance considerations are not merely optional; they lay the groundwork for building efficient, scalable, and responsive applications. Examining performance aspects can help identify bottlenecks early in the development process, ensuring that the overall experience is smooth for end-users.
When it comes to performance, several key elements stand out, including profiling, memory management, and optimization strategies. By honing in on these areas, developers eyeing top-tier performance can systematically resolve potential issues and maximize the effectiveness of their Java applications.
Profiling Java Applications
Profiling is the art of measuring how an application consumes resources while it's executed. In this process, developers can gather insights on CPU usage, memory allocations, and execution times for various methods—information that can be pivotal in understanding where performance hitches may arise. There are a variety of profiling tools available for Java applications, such as VisualVM and YourKit. These tools help developers identify hot spots within their code, providing a granular view of system behavior under different workloads.
The benefits of profiling include:
- Enhanced Performance: By identifying slow methods and tight loops, developers can refactor them as needed.
- Resource Usage Insights: Understanding resource consumption leads to better resource allocation.
- Guided Optimization: Using data-driven approaches allows developers to address performance issues based on concrete evidence instead of guesswork.
Profiling, in effect, transforms performance tuning from an abstract theory into a grounded practice.
Memory Management Techniques
Memory management is a crucial aspect of ensuring that a Java application runs efficiently over time. Java’s automatic garbage collection does much of the heavy lifting, but developers must still be aware of memory allocation patterns and garbage collection cycles, as improper management can lead to memory leaks and unintended application slowdowns.
To sharpen memory management techniques, consider the following:
- Use Appropriate Data Structures: Opt for data structures that are optimal for your use case. For example, using an when a is more suitable can incur unnecessary overhead.
- Implement Object Pooling: This strategy involves reusing instances rather than constantly creating and disposing of objects. For high-frequency object use, it can significantly cut down on time and memory fragmentation.
- Analyze Memory Usage: Leverage tools like the Eclipse Memory Analyzer (MAT) to scrutinize heap dumps, helping pinpoint memory leaks.
"Effective memory management is not just about freeing up space; it's also about maximizing performance over time."
By prioritizing memory considerations early in the design and development stages, developers become equipped to create applications that do not just run better but also stand the test of time in the fast-paced tech landscape.
Testing Java Applications
Testing represents a cornerstone of the software development lifecycle, especially in the realm of Java application design. During the development of any application, ensuring its reliability, functionality, and performance is crucial. This guarantees that users experience fewer bugs, leading to a smoother interaction with the software. Fundamentally, testing serves to identify areas of risk in your applications and provides feedback that can be pivotal for future design iterations.
One of the primary benefits of rigorous testing is that it fosters greater confidence among developers. It allows them to make changes and updates to the codebase while knowing that they can rely on automated tests to signal any regressions or failures that may arise from their work. This reduces the risk of introducing new issues into the production environment, which can damage user trust and cost organizations dearly in terms of reputation and resources.
"A working application is not just a matter of looks; it’s about proving its worth through consistent testing and validation."
When structuring tests, it’s important to consider both unit tests and integration tests. Each plays a unique role within the software testing framework. The emphasis should be placed not just on code coverage, but also on the comprehensiveness of testing scenarios to better simulate real-world usage.
Unit Testing Practices
Unit testing focuses on the smallest testable parts of an application, known as units. In Java, this means writing tests for individual methods or classes to ensure each performs as expected. The key advantage of unit testing lies in its ability to isolate individual components, allowing developers to pinpoint issues quickly, which can lead to faster debugging and problem resolution.
Java provides several frameworks for unit testing, with JUnit and TestNG being among the most commonly used. Using these frameworks, developers can structure their tests clearly and consistently.
- Setup and Teardown: Both JUnit and TestNG allow for setup and teardown methods, facilitating repeatable tests. They help initialize conditions before tests and clean up afterward.
- Assertions: Unit tests often include assertions to verify the correctness of the output against expected results. Utilizing assertions correctly is crucial as they explicitly highlight the success or failure of tests.
- Mock Objects: In unit testing, it’s also essential to minimize dependencies. Using mock objects to simulate the behavior of complex components can streamline testing and enhance isolation, making tests more robust.
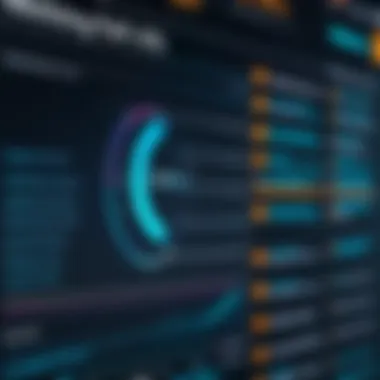
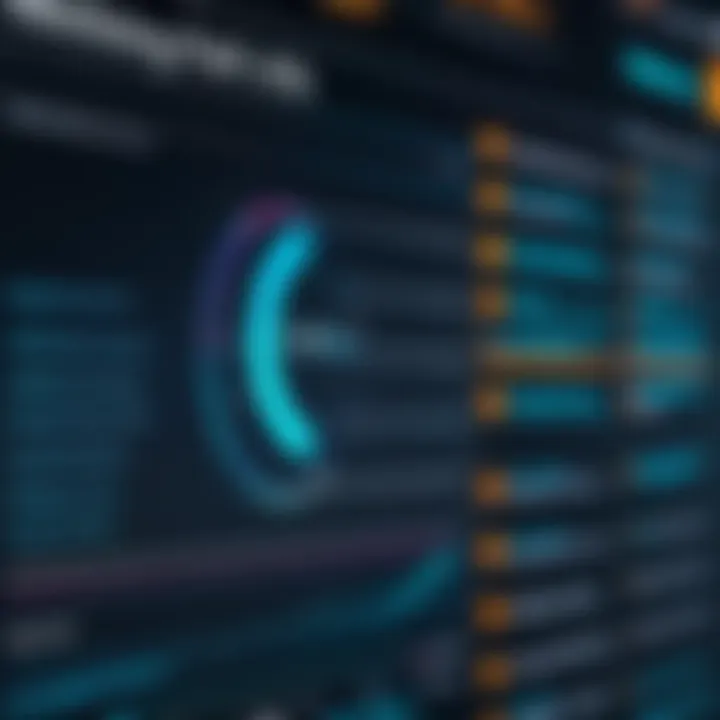
Example of a simple unit test using JUnit:
Integration Testing Strategies
While unit tests investigate the functionality of individual components, integration tests evaluate how those components operate together. It’s not uncommon for the interactions between modules to introduce unexplained errors, making integration testing a vital step in ensuring application integrity.
When structuring your integration testing strategy, several considerations come into play:
- Testing Environment: Ensure that the environment matches production as closely as possible. This simulates real-world interaction between components accurately.
- Data Management: Use a test database or mock services to rigorously test data flow and transactions between integrated modules without compromising live data.
- Tools: Tools like Postman, or frameworks such as Spring Test can significantly ease the process of writing and executing integration tests. These tools allow you to set up endpoint tests and run integrations across distributed systems seamlessly.
- Continuous Integration (CI): Implementing CI systems ensures that your integration tests run automatically whenever new code is pushed, helping catch any issues that crop up early.
An effective integration test can encompass multiple modules, forming a more nuanced picture of overall functionality even when certain individual components may not exhibit errors in isolation.
Deployment Strategies for Java Applications
When it comes to Java application design, discussing deployment strategies may seem a bit like discussing the icing on a cake. However, these strategies hold significant importance in ensuring that applications run smoothly and efficiently in a production environment. A solid deployment strategy can reduce downtime, improve user experience, and allow for rapid iteration. This section dives into pivotal aspects ranging from continuous deployment practices to the exploration of cloud-based deployment options.
Continuous Deployment Practices
Continuous deployment, often referred to as CD, is a practice that allows development teams to release changes to software applications automatically after they pass a series of tests. This means that as soon as developers write a piece of code and it has been validated through testing, it goes straight into production without manual intervention. In essence, it creates a robust feedback loop between developers and end-users.
Some key elements of continuous deployment include:
- Automation: Streamlined processes with automated testing and deployment reduce human error.
- Immediate User Feedback: Quick deployment allows developers to receive real-time feedback, enabling quick pivots based on user requirements.
- Increased Responsiveness: By minimizing the barriers to deployment, teams can react to market changes or fix bugs faster than ever before.
However, there are considerations that come into play:
- Testing Needs: Rigorous automated tests are critical because once code is pushed to production, flaws can easily reach the end user.
- Monitoring Post-Deployment: Continuous monitoring is necessary to catch issues that slip through the testing phase.
- Cultural Shift: Teams must embrace a culture of continuous improvement and responsibility, which may require training and adjustment.
Implementing continuous deployment can feel like a daunting task, but many tools and practices exist to help streamline the journey. From Jenkins to CircleCI, these can ease the transition.
Cloud-based Deployment Options
Cloud technology has redefined how Java applications can be deployed, allowing solutions to scale effortlessly while utilizing resources more efficiently. Deploying Java applications in cloud environments like Amazon Web Services, Google Cloud Platform, or Microsoft Azure provides several benefits while also raising unique considerations.
Benefits:
- Scalability: Cloud services offer the ability to scale resources up or down based on demand. For example, during high traffic periods, an application can utilize more servers and revert once demand decreases.
- Cost Efficiency: Pay-per-use models allow organizations to avoid costly investments in physical hardware.
- Geographic Flexibility: With multiple global data centers, applications can be delivered closer to users, enhancing performance and loading speeds.
Considerations:
- Vendor Lock-in: Dependence on a single cloud provider can lead to challenges if a business decides to migrate its applications in the future.
- Security Concerns: Storing data in the cloud necessitates strong security practices to prevent unauthorized access.
- Configuration Complexity: Navigating through various cloud services can be bewildering, requiring teams to stay up to date on best practices and offerings.
"In the era of technology, your deployment strategy is as critical as your application design itself."
Both continuous deployment and cloud-based options bring their own set of advantages and obstacles, making it essential to choose a strategy that aligns with the project requirements and team capabilities. For teams tackling Java applications, these deployment strategies are not just technical decisions but strategic ones that shape the future of their product.
Handling Technical Debt
In the context of software development, technical debt isn't just some abstract concept that gets tossed around during meetings. It's a very real phenomenon that can hinder progress and complicate future enhancements. Technical debt arises not from oversight but rather from design decisions made for the sake of expediency. Recognizing and managing this debt is paramount for Java application developers who aspire to create sustainable, robust applications.
Identifying Technical Debt
Spotting technical debt can be a bit like looking for a needle in a haystack, especially in large codebases. However, there are telltale signs that can signal its presence:
- Code Smells: Look out for overly complex classes or methods. If you find something that makes you scratch your head, there's a good chance it's a code smell pointing towards deeper issues.
- Inconsistent Patterns: When different parts of the application follow divergent patterns, it can lead to confusion and inconsistency in development and maintenance.
- Frequent Workarounds: If developers must regularly employ hacky fixes or quick workarounds, that’s a red flag indicating underlying structural problems.
- Test Failures: When automated tests frequently fail or require significant adjustments to accommodate new features, it often indicates a fragile or convoluted code structure.
Recognizing these signs early can prevent the accumulation of technical debt, steering the development process toward a cleaner and more manageable codebase.
Strategies to Mitigate Technical Debt
Mitigating technical debt requires a proactive approach, much like regular maintenance on a vehicle. Here are strategies that can help keep your Java applications running smoothly:
- Refactoring: Regularly revisiting and refactoring code can significantly reduce technical debt. This practice involves rewriting parts of the code to improve its structure without changing its external behavior. Aim for smaller, incremental changes over massive overhauls.
- Documentation: Proper documentation can go a long way in reducing misunderstandings and clarifying design decisions. When developers know the rationale behind certain choices, it lowers the likelihood of repeating mistakes. Ensure your code, decisions, and thought processes are well documented.
- Automated Testing: Implementing a comprehensive suite of unit and integration tests can help catch issues early. Tests serve as a safety net, allowing developers to refactor with confidence, thus minimizing sloppy short-term fixes that lead to debt.
- Regular Review Cycles: Conduct code reviews regularly. Peer feedback can often reveal hidden flaws and practices that lead to technical debt. It encourages a culture of accountability and awareness among developers.
- Prioritization: Not all debt is created equal. Focus on the debt that poses the greatest risk to your software's functionality and future enhancements. This can involve product managers and developers working in tandem to evaluate the cost-benefit of addressing various debts.
"Technical debt is like any other debt; it must be managed wisely. Ignoring it will only amplify its problems in the long run."
Tackling technical debt should never be viewed as a one-time task, but rather an ongoing journey. By embedding these strategies into your workflow, you can craft more durable, maintainable, and efficient Java applications. Remember, the goal is to gradually shift the balance from incurring debt to paying it down, ensuring your projects can sustain long-term growth without being shackled by past decisions.
Future Trends in Java Application Design
The landscape of software development is continuously evolving, and staying abreast of future trends in Java application design is vital for developers and IT professionals alike. As we move deeper into an era dominated by digital transformation, these trends shape how applications are built, deployed, and maintained. The importance of this topic lies not just in understanding current methodologies but also in preparing for the next wave of technologies that will undoubtedly impact Java development.
In embracing these trends, developers can harness the potential benefits such as improved performance, increased scalability, and enhanced user experience. It's crucial to consider factors like adaptability and resource efficiency when looking at future trends. Organizations that proactively integrate emerging technologies will likely find themselves at a competitive advantage, reaping benefits from more effective and streamlined processes.
Emerging Technologies and Frameworks
The surge of advancements in technology presents exciting opportunities for Java developers. Frameworks like Spring Boot and Micronaut are paving new roads for microservices architectures, allowing for rapid application development. These frameworks simplify the complexities involved, enabling developers to build robust applications that can handle high traffic loads. Utilizing containerization technologies, such as Docker, further enhances deployment options, fostering better resource management and environmental consistency.
A few of the noteworthy trends include:
- Serverless Architecture: With tools like AWS Lambda, developers can run code without provisioning or managing servers. This trend reduces upfront costs and allows developers to focus on writing code rather than worrying about infrastructure.
- Reactive Programming: Technologies like RxJava allow for event-driven applications that can handle a large number of concurrent users without blocking threads, enhancing responsiveness and performance.
- GraphQL: An alternative to REST, GraphQL allows clients to request exactly the data they need, which can lead to more efficient data fetching and better application performance.
Adapting to these emerging technologies and frameworks is fundamental. They inform the design and operation of modern applications, allowing for more flexible, maintainable, and efficient software solutions.
Impact of Artificial Intelligence on Design
Artificial Intelligence is not merely a buzzword; it's reshaping how applications are developed and designed. The impact of AI on Java application design can be seen in areas such as predictive analytics, automation, and improved decision-making processes. By integrating AI and machine learning capabilities into Java applications, developers can create systems that learn from data and improve over time.
Consider the following aspects of AI's influence:
- Enhanced User Experience (UX): AI can analyze user behavior, enabling personalized experiences. Java applications can also employ chatbots for improved customer support, streamlining communication and increasing efficiency.
- Automated Code Generation: Tools that utilize AI can assist in writing code faster and with fewer errors. For example, tools like Codex by OpenAI can help generate code snippets based on natural language descriptions.
- Predictive Maintenance: AI algorithms can forecast system failures by analyzing historical data trends and patterns. Java developers can integrate these analytics to enhance application performance and reliability.