Mastering the Intricacies of Database Programming in Java: A Comprehensive Guide
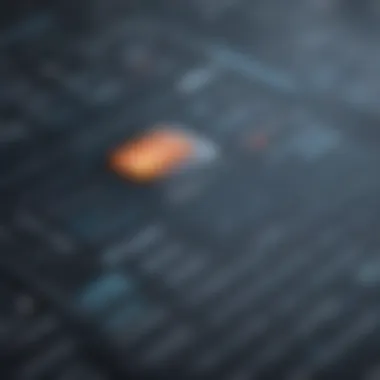
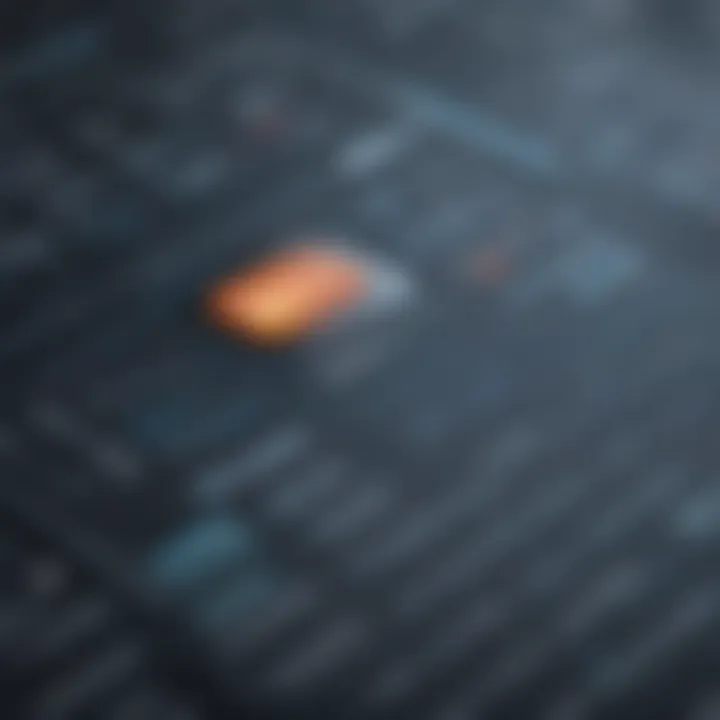
Overview of Database Programming in Java
In the domain of software development, database programming in Java holds significant importance. Java is widely utilized in designing robust and efficient applications that interact with various databases. The key features of database programming in Java include its compatibility with multiple database management systems, such as MySQL, Oracle, and SQLite. This versatility enables developers to seamlessly integrate Java applications with diverse database environments. The use cases of Java database programming range from developing data-driven web applications to creating enterprise-level systems that require secure and reliable data management.
Best Practices for Java Database Programming
When delving into database programming in Java, implementing industry best practices is essential for ensuring optimized performance and scalability. Developers should adhere to efficient query optimization techniques to enhance database access speed. Additionally, utilizing connection pooling mechanisms can significantly boost application responsiveness and resource utilization. To maximize efficiency and productivity in Java database programming, developers should adopt object-relational mapping (ORM) frameworks like Hibernate or JPA to streamline data manipulation and persisting objects in databases. Common pitfalls to avoid in Java database programming include inefficient data retrieval methods, inadequate error handling, and suboptimal database schema designs.
Case Studies in Java Database Programming
Real-world examples illustrate the successful implementation of Java database programming concepts in varied scenarios. For instance, a renowned e-commerce platform enhanced its order processing system using Java database programming, resulting in faster transaction processing and improved customer satisfaction. Lessons learned from such implementations highlight the importance of data indexing for query optimization, incorporating data caching mechanisms for performance enhancement, and ensuring robust transaction management for data consistency. Insights from industry experts emphasize the significance of continuous performance monitoring, database normalization, and proactive data security measures in Java database programming.
Latest Trends and Updates in Java Database Programming
The field of database programming in Java is witnessing notable advancements, including the integration of cloud-native database services, such as Amazon RDS and Google Cloud SQL, to facilitate seamless scalability and data durability. Current industry trends focus on the adoption of microservices architecture for decentralized data management and the emergence of blockchain-based databases for enhanced data transparency and security. Innovations like graph databases in Java applications offer enhanced data visualization capabilities and sophisticated data querying functionalities, catering to the evolving needs of modern software development.
How-To Guides and Tutorials for Java Database Programming
For beginners and advanced users alike, step-by-step guides and hands-on tutorials play a vital role in mastering Java database programming. Beginners can benefit from tutorials on setting up JDBC connections, executing SQL queries, and implementing basic CRUD operations in Java applications. Advanced users can explore in-depth tutorials on integrating ORM frameworks, implementing database transactions, and optimizing database performance through indexing and query tuning techniques. Practical tips and tricks, such as connection pool configuration, entity mapping strategies, and batch processing optimizations, empower Java developers to harness the full potential of database programming in their applications.
Introduction to Database Programming in Java
In the realm of software development, understanding database programming in Java holds paramount importance. As technology advances, the significance of effectively utilizing databases within Java applications cannot be overstated. Java, known for its robustness and versatility, serves as a key player in facilitating seamless interaction with databases. By delving into this topic, developers gain insight into optimizing their application's performance, scalability, and security.
Understanding the Significance of Databases
Evolution of Databases
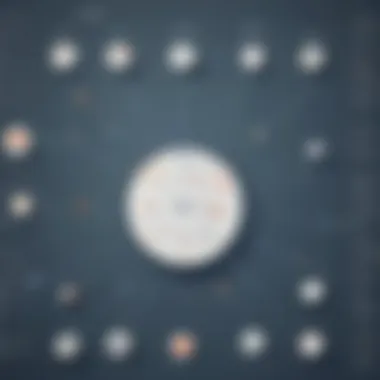
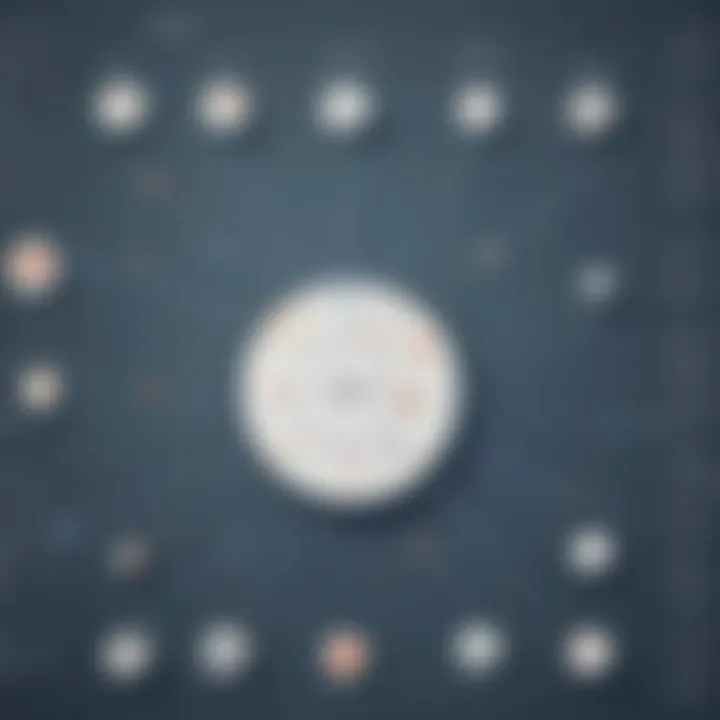
The evolution of databases remains a pivotal aspect in the landscape of technology. Over time, databases have transitioned from traditional hierarchical models to sophisticated relational databases, ultimately contributing to the efficiency and effectiveness of data storage and retrieval mechanisms. The evolution of databases plays a crucial role in shaping the way modern applications handle and manage vast amounts of data, offering structured and organized storage solutions that enhance the overall functionality of applications.
Role of Databases in Modern Applications
Databases serve as the backbone of modern applications, playing a transformative role in how data is stored, accessed, and manipulated. In today's dynamic digital environment, databases are instrumental in ensuring data integrity, enabling seamless data transactions, and supporting complex querying operations. Their importance lies in providing a reliable and scalable infrastructure that empowers applications to deliver optimal performance and responsiveness in handling diverse user interactions.
Java as a Robust Programming Language for Databases
Advantages of Using Java for Database Operations
Java's suitability for database operations stems from its high portability, multi-threading capabilities, and extensive community support. The language's platform independence allows developers to write code once and run it on any Java-enabled platform, streamlining the development process. Furthermore, Java's robust multi-threading features enable applications to execute multiple database operations concurrently, enhancing performance and efficiency.
Impact of Java on Data Management
Java's impact on data management is profound, providing developers with a versatile set of tools, libraries, and frameworks to streamline database interactions. With Java, developers can leverage powerful object-oriented concepts to model data effectively, leading to more manageable and scalable database solutions. Java's influence on data management extends to enhancing data security, transaction management, and overall system stability, making it a preferred choice for database-centric applications.
Core Concepts of Database Programming in Java
In this segment of the article, we delve into the fundamental principles of database programming in Java. Understanding the core concepts is crucial for anyone looking to harness the power of databases within Java applications. By exploring Java Database Connectivity (JDBC) and Object-Relational Mapping (ORM) frameworks, developers can streamline data management and improve application efficiency. These concepts serve as the building blocks for database operations in Java, laying the groundwork for advanced techniques and best practices.
Java Database Connectivity (JDBC)
Working with JDBC Drivers
When it comes to working with JDBC drivers in Java, developers benefit from a direct interface to interact with various databases. JDBC drivers facilitate seamless communication between Java code and the database management system, enabling the execution of SQL queries and manipulation of data. The key characteristic of JDBC drivers lies in their platform independence, allowing developers to write database-agnostic code that can be deployed across different environments. This flexibility makes JDBC drivers a popular choice for database operations in Java, offering high performance and reliability. However, developers must be mindful of the driver version compatibility and configuration nuances to ensure optimal connectivity and data access.
Establishing Database Connections in Java
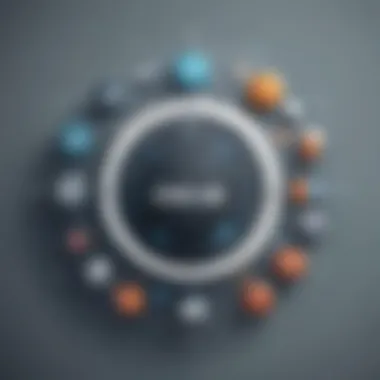
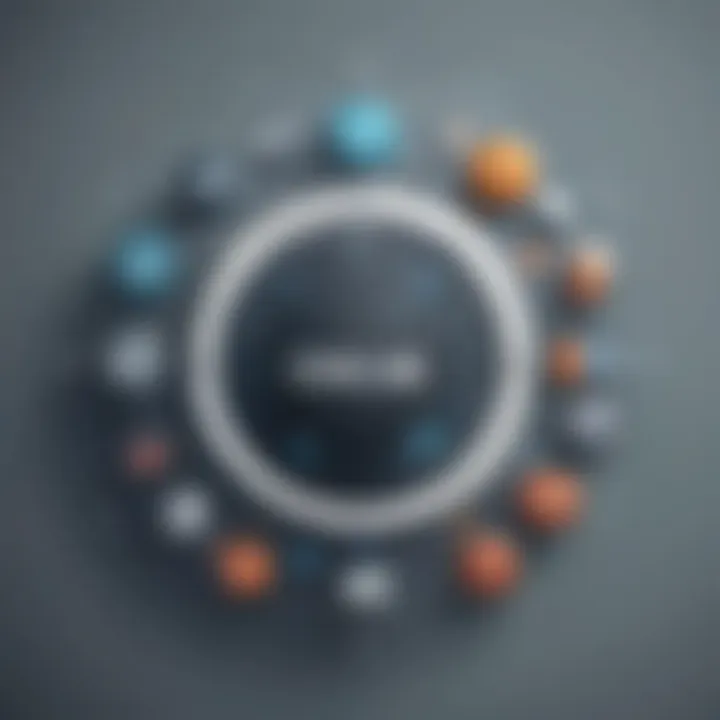
Establishing database connections in Java is a critical aspect of database programming, as it lays the foundation for executing SQL queries and transmitting data between the application and the database. The key characteristic of database connections is the ability to create a session that bridges the gap between Java code and the database server, facilitating data retrieval, updates, and transactions. This feature is beneficial for real-time data processing and seamless integration of database functionalities within Java applications. However, developers should handle connection pooling and resource management efficiently to prevent memory leaks and performance issues.
Executing SQL Queries
Executing SQL queries in Java is essential for retrieving, updating, or deleting data from a database using structured query language. The key characteristic of executing SQL queries is the dynamic generation of database commands within Java code, allowing developers to interact with the database programmatically. This process enables data manipulation, transaction management, and result set handling, ensuring accurate and efficient database operations. However, developers should optimize query performance, handle parameterized queries securely, and implement error handling mechanisms to enhance the overall stability and security of the application.
Object-Relational Mapping (ORM) in Java
In the realm of database programming, Object-Relational Mapping (ORM) frameworks play a pivotal role in bridging the gap between object-oriented programming in Java and relational databases. By providing a seamless mapping between classes and database tables, ORM frameworks simplify data persistence and retrieval, eliminating the need for complex SQL queries. The overview of ORM frameworks highlights their ability to enhance developer productivity, maintain code consistency, and reduce boilerplate code in database interactions. However, developers should consider the performance overhead of ORM frameworks, design efficient entity relationships, and optimize object-to-database mappings to maximize application performance and database scalability.
Advanced Techniques in Database Programming
The section of Advanced Techniques in Database Programming within this comprehensive guide on Exploring Database Programming in Java serves a pivotal role. To grasp the essence of this complex subject is vital for software developers and IT professionals seeking to optimize database operations. Unlike basic concepts, advanced techniques delve deeper, unraveling intricacies that can elevate database performance to new heights. Within this section, we will navigate through essential elements such as Transaction Management, Isolation Levels, ACID Properties, Performance Optimization Strategies, Caching Mechanisms, and Query Optimization.
Transaction Management in Java
Transaction Management in Java represents a crucial aspect of database programming efficiency. A transaction ensures the integrity of data operations within a database, facilitating smooth and accurate processing of queries. Within this domain, Isolation Levels play a pivotal role in determining the degree of isolation between concurrent transactions. Let's delve into the specifics of Isolation Levels and understand their significance within the context of database operations in Java.
Isolation Levels
Isolation Levels dictate the degree to which concurrent transactions are isolated from one another. The four standard Isolation Levels - Read Uncommitted, Read Committed, Repeatable Read, and Serializable - offer varying layers of isolation, catering to different data consistency requirements. Understanding and implementing the appropriate Isolation Level is paramount for safeguarding data integrity and minimizing resource contention within database environments. Despite their benefits, each Isolation Level entails distinct advantages and considerations that need careful evaluation depending on the application's specific requirements.
ACID Properties
ACID Properties, comprising Atomicity, Consistency, Isolation, and Durability, form the cornerstone of reliable database transactions. These properties ensure that database operations are executed reliably and revert to a consistent state in case of failures. The meticulous adherence to ACID Properties guarantees the integrity of transactions, preventing data anomalies and ensuring robustness in data management. Despite their importance, ACID Properties also introduce certain trade-offs and considerations that developers need to weigh while designing database systems.
Performance Optimization Strategies
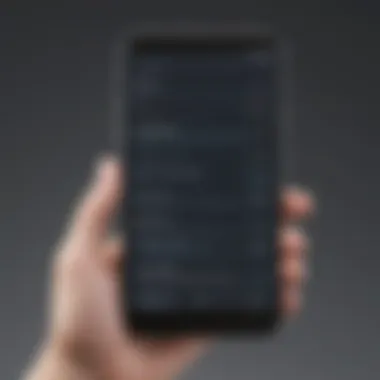
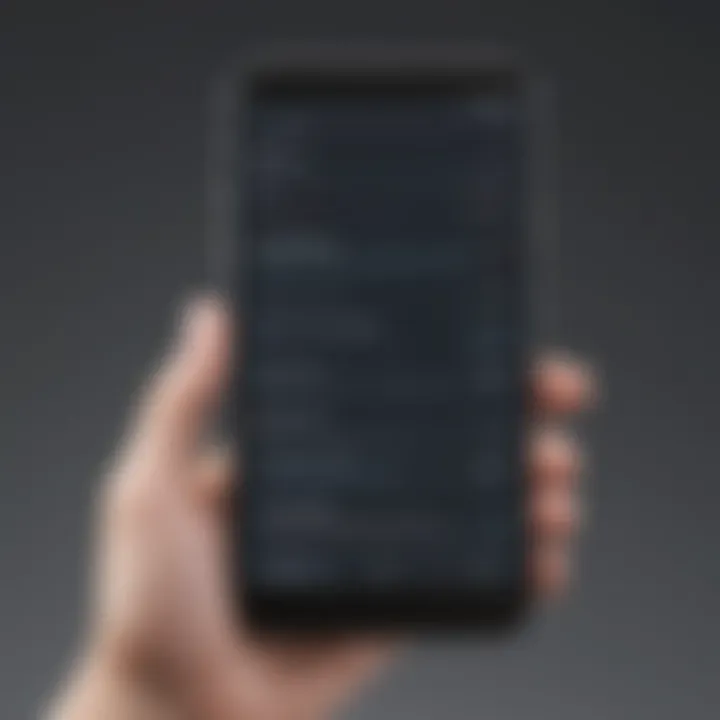
In the realm of database programming, Performance Optimization Strategies are fundamental for enhancing the efficiency and speed of database operations. Caching Mechanisms and Query Optimization stand out as indispensable tools in the arsenal of database developers, aiming to minimize query execution times and optimize system throughput.
Caching Mechanisms
Caching Mechanisms cache frequently accessed data in memory, reducing the need for repetitive database queries and enhancing overall system performance. By storing data closer to the application layer, caching mechanisms significantly reduce latency and improve response times. However, the implementation of caching mechanisms requires thoughtful consideration of data consistency and cache invalidation strategies to strike a balance between performance gains and data integrity.
Query Optimization
Query Optimization focuses on enhancing the efficiency of SQL queries by analyzing query execution plans, indexing strategies, and database statistics. By fine-tuning queries to leverage indexes and minimize resource-intensive operations, query optimization plays a pivotal role in improving database performance. However, developers need to be cautious of potential pitfalls such as over-optimization and query plan instability, ensuring a balanced approach towards optimizing query performance.
This section elucidates the critical role of Advanced Techniques in Database Programming within the Java ecosystem, offering insights into transaction management, performance optimization, and vital strategies for elevating database systems to peak efficiency.
Best Practices and Security Measures
In this section, we delve into the crucial aspect of Best Practices and Security Measures within the realm of database programming in Java. Understanding the importance of adhering to best practices and implementing robust security measures is imperative for ensuring the integrity and confidentiality of data in Java applications. By following industry-recognized best practices, developers can mitigate potential vulnerabilities and safeguard sensitive information from unauthorized access, ensuring a robust and secure database environment.
Ensuring Data Integrity and Security
Prepared Statements
Prepared Statements play a pivotal role in enhancing the security of database operations in Java. These precompiled SQL statements offer numerous advantages, including optimized query execution, prevention of SQL injection attacks, and efficient reuse of query plans. The key characteristic of Prepared Statements lies in their ability to parameterize queries, thereby reducing the risk of malicious injection of SQL code by external sources. This feature is particularly beneficial in scenarios where user input is involved, ensuring that data manipulation is controlled and secure within the context of this article.
Input Validation
Effective Input Validation serves as a crucial line of defense against various forms of cyber threats and data inconsistencies. By validating user input against predefined criteria and data formats, developers can prevent unauthorized data alterations, buffer overflows, and other common security vulnerabilities. The key characteristic of Input Validation is its ability to enforce data integrity rules at the point of entry, thereby mitigating the risk of malformed data compromising the database integrity. While Input Validation enhances overall data security, developers must be cautious of over-validation, which can lead to usability issues and performance overhead within the purview of this article.
Implementing Scalable Database Solutions
In this section, we explore the nuances of implementing scalable database solutions within Java applications. Database Connection Pooling emerges as a critical aspect of scalability, allowing for efficient management of database connections and optimized resource utilization. The key characteristic of Database Connection Pooling lies in its ability to reuse existing connections, minimizing connection overhead and enhancing performance in high-traffic scenarios. By managing connection pooling effectively, developers can achieve enhanced scalability and improved responsiveness in database operations.
Vertical vs. Horizontal Scaling
The debate between Vertical and Horizontal Scaling is pivotal in determining the scalability strategy for database systems in Java applications. Vertical Scaling involves increasing the capacity of existing hardware, while Horizontal Scaling focuses on distributing workload across multiple machines. The unique feature of Vertical Scaling lies in its simplicity and cost-effectiveness for smaller databases, whereas Horizontal Scaling offers unmatched scalability and fault tolerance for large-scale applications. Understanding the advantages and disadvantages of each scaling approach is essential for crafting a scalable and resilient database architecture within the context of this article.