Mastering Data Structures and Algorithms for Software Development
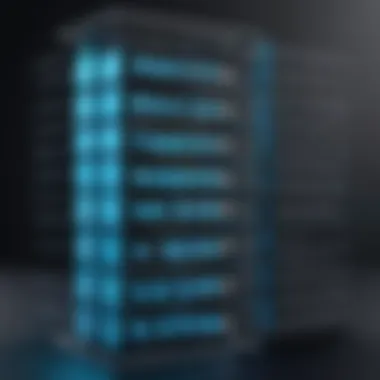
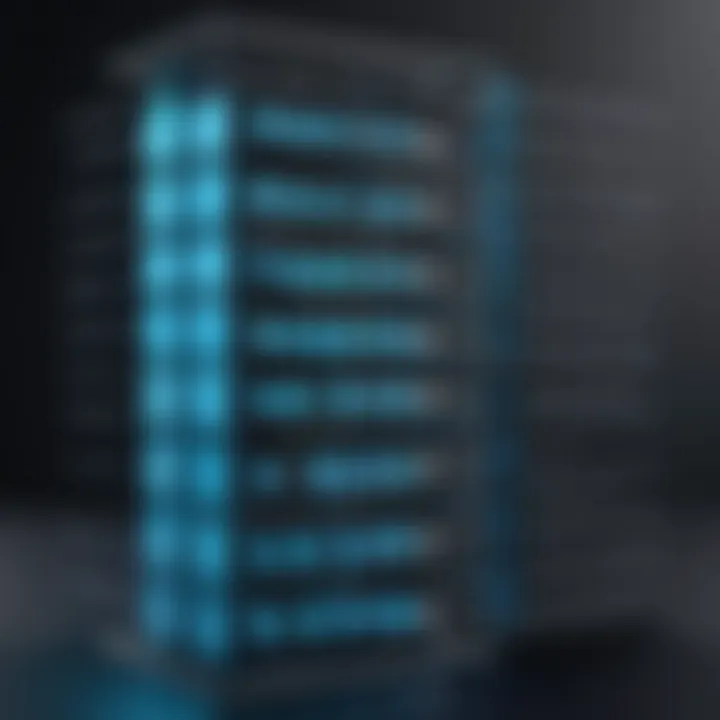
Intro
In today’s rapidly evolving technological ecosystem, understanding the core principles of software development is not just a luxury—it's a necessity. At the heart of this evolution lie data structures and algorithms, which serve as the backbone of efficient coding and problem-solving practices. They are not merely tools but the very foundation that enables developers and data scientists to create optimized solutions for complex challenges.
With the ever-increasing scope of cloud computing, data analytics, and machine learning, gaining a solid grasp of these concepts is essential. The modern software landscape demands that professionals not only know how to use various tools and technologies but also understand the underlying principles that drive them. This knowledge is crucial in a world where data is the new oil, and algorithms fuel the engines of decision-making.
Data structures determine how information is organized and accessed, affecting system performance and speed. Algorithms, on the other hand, outline the processes needed to manipulate and analyze this structured data. Together, they optimize systems, enhance user experiences, and pave the way for innovations.
As we delve deeper into this article, we will explore the importance of data structures and algorithms in contemporary software development, their specific functions, and how they align with emerging trends across different sectors. From practical applications to theoretical implications, by the end of this narrative, you will have a clearer perspective on why these concepts are indispensable in today’s tech landscape.
Intro to Data Structures and Algorithms
In the ever-evolving landscape of software development, data structures and algorithms stand out as the backbone of efficient programming. These concepts not only influence how data is organized and manipulated but also determine the efficiency with which software solutions perform. Adequate knowledge of both is not just beneficial; it is often imperative for crafting effective software that meets user needs and expectations.
Definition and Importance
To define data structures simply, they are systematic ways of organizing and storing data to enable efficient access and modification. On the other hand, algorithms are sets of rules or procedures for solving a problem. The synergy between these two elements not only enhances data handling but also streamlines computational processes.
Consider a scenario where a software developer needs to manage user information for a rising e-commerce platform. Choosing the right data structure, such as a , allows for fast data retrieval, while employing can help minimize the time taken to search for prices or historical sales data. This shows how directly linked the choice of data structures and algorithms is to software performance, influencing factors like responsiveness and scalability.
Moreover, mastering these concepts leads to better problem-solving abilities. As a developer improves their standard practice of analyzing problems through the lens of data structure choices and algorithm optimization, they inherently become better technologists, capable of creating robust solutions. In today’s tech-driven world, where the speed of information and data processing can make or break a company’s success, understanding these foundational components is vital.
Historical Context
The journey of data structures and algorithms has roots that stretch back to the dawn of computing. Initially, the focus was on simple structures like arrays, which offered straightforward methods to store data in a contiguous block of memory. However, as technology advanced, the need for more complex structures arose. For instance, in the 1960s, linked lists emerged to address situations where dynamic data sizes were more common.
Furthermore, the introduction of graph theory in the 1970s significantly impacted algorithm design, allowing for more sophisticated networking and relationship-based data handling. The rise of computational complexity theory, which classifies algorithms based on their resource consumption, brought about a profound shift in benchmarking algorithm efficiency, exemplified by the broader acceptance of Big O notation.
In sum, the historical development of data structures and algorithms reflects a growing recognition of their critical role in computational efficiency. From basic data handling to complex machine learning integrations, understanding this evolution provides a vital perspective for modern software professionals.
"The great strength of data structures and algorithms lies in their timelessness, continually guiding software development practices across decades."
Types of Data Structures
Understanding types of data structures is crucial for any software development project. They provide the scaffolding on which algorithms operate, shaping the efficiency and performance of systems. Choosing the right data structure can make or break your application's capabilities. The importance of this topic extends across the board in programming — from optimizing data access to enhancing memory management.
Linear Data Structures
Linear data structures are those where data elements are arranged in a sequential manner. This characteristic brings simplicity and clarity to operations. It impacts various algorithms, making execution straightforward and oftentimes more efficient. Let's go through some key types of linear data structures:
Arrays
Arrays are a staple in programming, characterized by their fixed size and indexed access. They contribute to performance due to their ability to store multiple items of the same type in contiguous memory locations. The ease of random access — where any element can be fetched in a constant time complexity of O(1) — makes arrays a favored choice for applications requiring quick retrieval.
However, arrays come with a unique feature of being immutable in size. Once the size is defined, it cannot be changed without allocating a new array and copying elements over, which can hinder flexibility in scenarios with dynamic data sizes. Still, their efficiency in terms of accessing elements is the reason they hold a prominent place in data structure discussions.
Linked Lists
Linked lists break the mold of fixed-size structures. This data structure comprises nodes, each containing data and a pointer to the next node. The key characteristic of linked lists is dynamic size; they can grow and shrink as needed. This flexibility is a significant benefit, especially when the number of elements is not known ahead of time.
Yet, linked lists come with trade-offs. Unlike arrays, accessing an element takes linear time — O(n) — since traversal from the head is required. This might deter their use in applications where rapid data retrieval is crucial. Still, they shine in scenarios where frequent insertion and deletion of nodes are necessary.
Stacks
Stacks embody the Last In, First Out (LIFO) principle. In this structure, the last element added is the first one to be removed. This characteristic makes stacks pivotal for managing tasks like function calls in programming or undo mechanisms in applications. The ability to access only the top element lends itself nicely to certain algorithms where maintaining a strict order is necessary.
The downside? Stacks can be limited in flexibility, as you can only interact with the top element. Operations like accessing an element in the middle become cumbersome. Even so, their efficiency in managing function calls demonstrates why they are often crucial in the development process.
Queues
Queues operate on a first come, first served basis. This FIFO (First In, First Out) structure allows elements to be added at one end and removed from the other, creating a smooth handling of tasks. This is particularly useful in scenarios like print spooling or asynchronous data processing where order matters.
A unique feature of queues is their ability to enhance task scheduling, ensuring that operations happen in the exact sequence intended. However, one downside is that like stacks, they can also introduce delays in accessing other elements. Nevertheless, their utility in intensive workloads can’t be overstated.
Non-linear Data Structures
Moving beyond linearity, non-linear data structures allow for more complexity in operations and relationships. They are pivotal for representing data that would not fit into a straight line, such as hierarchical relationships often found in databases and networks. Here's a closer look:
Trees
Trees are a hierarchical data structure. They consist of nodes that are connected by edges, with a single root node from which all other nodes branch out. The significant advantage of trees is that they allow for efficient data storage and retrieval operations, particularly in contexts that require hierarchical data representation, like file systems or databases.
One unique feature of trees is their ability to balance themselves significantly; for example, AVL trees automatically keep themselves balanced, which ensures that retrieval times remain efficient. However, the complexity in maintaining tree structures does present its challenges, such as the overhead in rotations and balancing, which can be resource-intensive.
Graphs
Graphs extend beyond trees in terms of connections. Comprising nodes (vertices) connected by edges, graphs can represent complex relationships such as social networks or city maps effectively. This structure's versatility makes it an excellent choice for representing real-world applications and solving problems involving relationships.
The unique feature of graphs becoming both directed and undirected adds another layer of complexity that can be either beneficial or detrimental — depending on the problem at hand. However, the challenge lies in their often high memory consumption and maintenance costs.
Hash Tables
Hash tables stand out for their capability to implement associative arrays, allowing for fast data retrieval based on unique keys. They employ a hash function to convert keys into indices for an array, enabling almost instantaneous access to values.
One key characteristic is their efficiency, which can be O(1) for both insertion and retrieval. However, collision handling can complicate operations when two keys hash to the same index. Even with these trade-offs, their widespread application in caching, database indexing, and various algorithms highlights their importance.
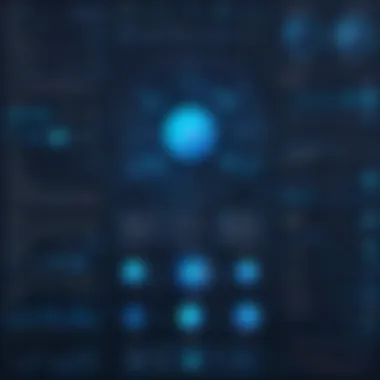
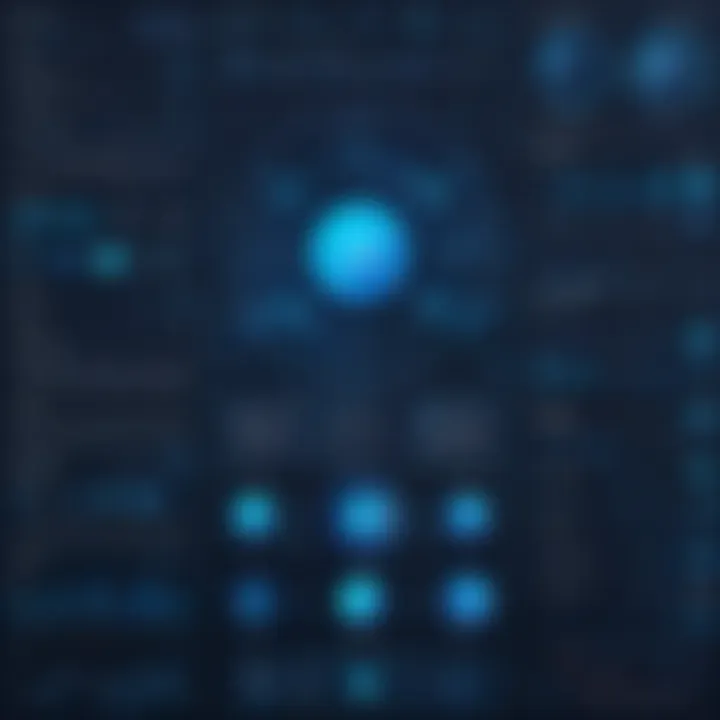
Abstract Data Types
Abstract data types (ADTs) provide a means to encapsulate data structures while defining operations on the data independently. This summary focuses on pushing beyond the basic understanding of the structures mentioned. It's important to realize that while we can architect systems around these data types, a thorough understanding of their performance implications is necessary to harness their full potential in real-world applications.
Algorithmic Foundations
Understanding algorithmic foundations is like having the blueprints for constructing a robust building; it provides you with essential structures for tackling diverse issues in software development. This section explores what an algorithm is, its complexities, and efficiency, setting a framework for everything from simple sorting tasks to complex data processing in cloud environments. This knowledge empowers developers, software engineers, and data scientists alike, effectively aiding in their problem-solving repertoire and ensuring optimal performance in their applications.
What is an Algorithm?
At its core, an algorithm is a well-defined set of rules or instructions designed to perform a task or solve a problem. Think of it as a recipe; just as a recipe lists ingredients and steps to create a dish, algorithms specify the necessary steps to achieve a desired outcome. They are crucial in computer science, acting as the foundation for data processing, calculations, and automated reasoning.
Algorithms can vary greatly in complexity and design. For instance, a straightforward algorithm might be a set of instructions to add two numbers. However, more advanced algorithms deal with complex functions like searching data in a vast database or sorting large datasets.
Algorithm Complexity
When assessing an algorithm, understanding its complexity is paramount. Complexity can be broken down primarily into two facets: time and space. Both significantly affect performance, thus being critical considerations in software development.
Big O Notation
Big O Notation provides a way to express the upper limit of an algorithm's running time or space requirement in relation to the size of the input data. It’s a fundamental concept because it allows developers to evaluate how efficient an algorithm will be as workloads scale. A key characteristic of Big O Notation is its focus on the worst-case scenario, providing a safety net for performance expectations.
For example, an algorithm that sorts data with an average complexity of O(n log n) is considered efficient for larger datasets, whereas one with O(n^2) would struggle as the data size increases. This uniqueness makes Big O Notation a favorite among developers, as it simplifies complex evaluations into understandable metrics. However, while it is very useful, it does not account for all factors affecting performance, such as constant factors and lower-order terms, which can sometimes be equally important.
Space Complexity
Space Complexity looks at the total amount of memory that an algorithm utilizes when running. Like Big O Notation, it helps in gauging algorithm efficiency, particularly when assessing algorithms that require substantial amounts of data storage during execution. A key characteristic of space complexity is its focus on memory consumption, something frequently overlooked but essential for applications running in constrained environments where resources are limited.
For instance, an algorithm that stores temporary variables during execution may have a space complexity of O(n), meaning the memory usage scales linearly with the input size. This awareness makes space complexity crucial when selecting data structures or algorithms, as it provides insight into system requirements and helps ensure that applications run smoothly without consuming excess resources, which can lead to inefficient performance or system crashes.
Algorithm Efficiency
When we talk about algorithm efficiency, we're diving into how well an algorithm performs specifically concerning time and space complexity. A well-optimized algorithm can substantially reduce execution time and resource consumption, which is invariably critical in real-world applications, particularly in contexts like software development and data analytics.
Efficient algorithms not only enhance user experience by speeding up processes but also contribute to overall cost-effectiveness, particularly in cloud computing scenarios where resource allocation translates directly to expenses.
In summary, the fundamentals of algorithms—what they are, their complexity, and their efficiency—serve as the bedrock for modern software development. By comprehending these aspects, developers can make informed decisions that will vastly improve the performance and reliability of their applications, leading to successful outcomes.
Common Algorithms and Their Applications
Understanding algorithms is key to optimizing problem-solving in software development. Common algorithms serve as the backbone for many applications, enabling efficient processing of data. From sorting data to searching through databases, algorithms help us organize and retrieve information effectively. Considered essential in both theoretical and practical aspects of programming, they streamline operations, improve reliability, and enhance user experience.
Sorting Algorithms
Sorting algorithms are crucial for organizing data in a meaningful order, thus making data retrieval faster and easier. Let's take a look at some prominent sorting algorithms.
Quick Sort
Quick Sort stands out due to its efficiency and simplicity. It employs a divide-and-conquer method by selecting a 'pivot' element and partitioning the data. This results in sorting either side of the pivot, which ultimately leads to a completely sorted list. A key characteristic of Quick Sort is its average-case time complexity of O(n log n), making it one of the fastest sorting algorithms. Its low memory usage is a distinct advantage, as it sorts in place.
However, Quick Sort can become less efficient if implemented poorly or used on already sorted data, leading to a degradation to O(n²). Yet, with a good pivot strategy, it remains a popular choice within developers’ toolkits.
Merge Sort
Merge Sort, another popular algorithm, uses a similar divide-and-conquer strategy. It divides the array into halves, sorts each half, and then merges them back together. The key feature of this algorithm is that it guarantees a time complexity of O(n log n), regardless of the input data. This predictability is a strong benefit, particularly for developers looking to ensure consistent performance.
One of the trade-offs of Merge Sort is its space complexity. It generally requires additional memory to hold the sub-arrays during the merging process. Therefore, while it is efficient and stable, the memory overhead can be a deciding factor for applications with strict memory limitations.
Bubble Sort
Bubble Sort may not be the most efficient, but it has its own charm. This simplistic algorithm repeatedly steps through the list, compares adjacent elements, and swaps them if they're in the wrong order. Its most basic trait is that it’s incredibly easy to understand, making it a reliable didactic tool for beginners.
However, with a worst-case time complexity of O(n²), Bubble Sort’s practicality diminishes for larger datasets. It’s instructional rather than practical, ideal for those just starting to learn algorithms.
Search Algorithms
Searching through data efficiently can determine the success of any application. Search algorithms find specific data points, enabling users to retrieve information swiftly.
Binary Search
Binary Search operates under the premise that the data is sorted. By repeatedly dividing the search interval in half, it quickly locates the target value. Its time complexity of O(log n) underscores its efficiency compared to linear search methods. A significant strength of Binary Search lies in its ability to handle very large datasets smoothly.
However, it relies on pre-sorted data. If the dataset isn't sorted, the performance can significantly degrade as you'll have to sort it first, a step that can add overhead.
Depth-first Search
Depth-first Search (DFS) is an algorithm used for traversing or searching through tree or graph data structures. It explores as far down one branch as possible before backtracking. This characteristic makes DFS memory efficient, requiring less space than breadth-first methods in many cases.
DFS can get caught in deep branches, which sometimes leads to inefficiencies in searching for shallow nodes. That can add a layer of complexity if the data structure is not laid out strategically.
Breadth-first Search
Breadth-first Search (BFS), contrasting with DFS, examines all neighbors before moving deeper into the structure. Its systematic approach is particularly well-suited for finding the shortest path in graph-based scenarios. The key characteristic here is that it guarantees the shortest path in unweighted graphs, making it a favorite in various applications.
However, BFS consumes more memory, as it requires tracking multiple paths at once. In cases where the graphs have large branching factors, memory limitations can arise, complicating its execution.
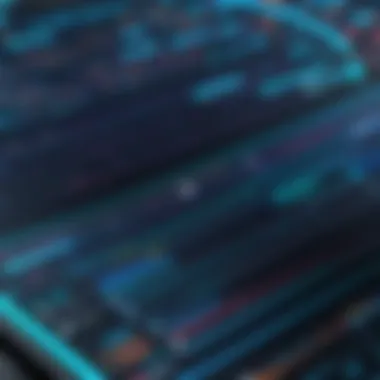
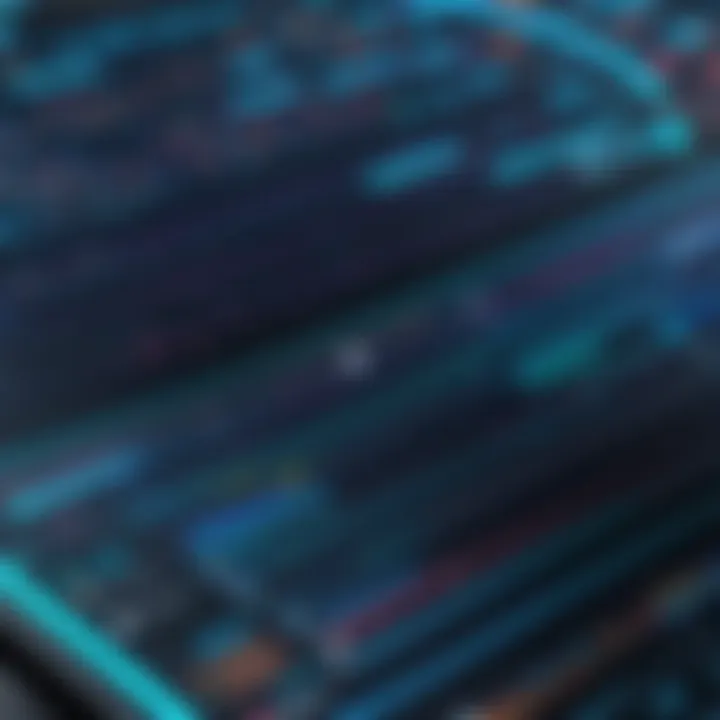
Graph Algorithms
Graph algorithms are pivotal when working with interconnected data. They form the basis of numerous applications in networking, social media, and web structure.
Dijkstra's Algorithm
Dijkstra's Algorithm is essential for finding the shortest path in a weighted graph. It evaluates all possible paths to decide the most efficient one. A notable quality is its capability to handle graphs with non-negative weights, providing optimal paths efficiently.
Despite its strengths, Dijkstra's Algorithm has drawbacks in terms of speed. It's less effective for graphs with high edge density unless paired with specific data structures, such as Fibonacci heaps, to improve time efficiency.
A Search Algorithm
The A* Search Algorithm, an extension to Dijkstra’s, incorporates heuristics to anticipate the distance to the target node. This forward-thinking characteristic can make A* significantly faster in certain scenarios by prioritizing nodes that seem to offer the most promise toward the goal.
However, the reliance on a good heuristic can sometimes backfire, resulting in less optimal paths if the heuristic does not give accurate estimates. In other words, it’s a powerful tool, but one that practitioners must wield carefully to avoid pitfalls.
Data Structures in Software Development
In the ever-evolving world of software development, the choice of data structures can act as a game changer. Data structures serve as the backbone of any software application, influencing everything from efficiency to scalability. In essence, they help in structuring data to be processed effectively and can significantly reduce execution times, which is paramount in a world demanding faster solutions. Having a firm grasp on the appropriate data structures can empower developers to write more efficient code, thus improving the overall performance of their applications.
The benefits of utilizing the right data structures include but are not limited to:
- Improved Efficiency: Well-suited data structures help minimize time complexity in algorithms. For instance, accessing data in a hash table is typically quicker than in an array.
- Optimized Memory Usage: Investigating the memory footprint of different structures can help in managing resources more effectively. Data structures like linked lists or trees save space compared to arrays.
- Enhanced Code Maintainability: Clear choices can lead to more readable and maintainable code, which in turn assists teams in collaborative settings.
However, choosing an appropriate data structure involves careful consideration. Factors such as the nature of operations (random access vs. sequential access), frequency of data modifications, and the size of data to be processed need to be explored. The landscape of software development is diverse, and the road to success is paved with strategic selections in data structures.
Choosing the Right Data Structure
Selecting the right data structure is akin to finding the right tool for a job; using a hammer for a screw will only cause frustration. Each data structure has its own strengths and weaknesses:
- Arrays are great for fixed-size collections where fast index-based access is needed, but they fall short in dynamic contexts where size changes.
- Linked Lists perform well when frequent insertions or deletions are required, making them ideal for queues or stacks. However, their sequential access capabilities can be limiting.
- Hash Tables offer constant time complexity for retrievals but require extra care for collisions. Thus, they shine in scenarios needing quick lookups but can falter if poorly implemented.
When contemplating which data structure to deploy, consider employing a decision matrix or flowchart to organize your choices based on the specifics of your application’s requirements.
Real-World Use Cases
Understanding the best practices in real-world applications makes the theoretical concept of data structures more tangible. Here are some examples:
- Web Development: In web applications, databases use various data structures for efficiency. Relational databases, for instance, often rely on B-trees for indexing, which ensures swift lookups in large datasets.
- Networking: Routing algorithms often utilize graph data structures. The shortest path problem in networks, solved using Dijkstra's algorithm, enhances network efficiency and speed.
- Machine Learning: When processing huge datasets, managing data efficiently is essential. Data frames in libraries like Pandas make use of optimized memory usage similar to arrays combined with the flexibility of linked lists.
"A solid understanding of data structures is not just an academic exercise. In practice, the lack of proper implementation could mean the difference between an application being a slick user experience and a frustrating failure."
In summary, data structures are not merely theoretical constructs; they are instrumental in the development and performance of software applications. Developers, by choosing wisely, can leverage these structures to build optimized solutions tailored to their users' needs.
Integrating Data Structures within Cloud Computing
In this age of fast-paced technology, the marriage of data structures and cloud computing stands as a pivotal element in software development. Integrating these data structures can lead to enhanced performance, better resource management, and more effective data manipulation. As applications scale and evolve in complexity, understanding how to seamlessly integrate data structures with cloud services can not only streamline processes, but also provide significant competitive advantages.
Data Storage Solutions
When considering data storage in the cloud, various solutions like Amazon S3, Google Cloud Storage, or Azure Blob Storage come into play. Each of these solutions provides different ways of storing and retrieving data, making the choice of data structure imperative to efficacy and optimization.
For instance, in a scenario where large amounts of unstructured data need to be processed, a structure like a hash table might be employed to allow faster access times. That minimizes the time spent searching through large data sets, which is often necessary when working within a cloud environment. Alternatively, if sequential access is needed frequently, using arrays could lead to better performance.
- Benefits of Choosing Appropriate Data Structures for Storage Solutions:
- Faster access and retrieval times
- Efficient memory usage
- Reduced costs in cloud service billing
The realistic application of data structures in cloud storage solutions cannot be understated. The way data is stored—whether it's a file system or a database—affects everything from read/write speeds to the costs incurred by using such services.
Scalability and Performance
Scalability refers to the ability of a cloud service to grow with user demands while ensuring consistent performance. When integrating data structures, a sound comprehension of how they behave under load is crucial. A good implementation can significantly ease the transition as user bases grow.
For example, if you're deploying a microservices architecture in a public cloud, the data structures chosen can affect how well services communicate and scale together. Utilizing trees or graphs might provide better ways of managing relationships and hierarchies in datasets, allowing services to retrieve and process data with greater efficiency.
In this regard, performance optimization becomes a necessity. Techniques such as caching, where results of expensive operations are stored for quicker access, often rely on the underlying data structures to be effective. In-memory databases, often integrated with data structures like hash tables, have shown excellent performance in read-heavy applications.
Optimizing data structures can have a snowball effect on software performance, affecting not just single applications, but entire ecosystems.
Choosing scalable, performant data structures ensures that as your application grows, it doesn't buckle under pressure. Decisions made early on impact whether an architecture can handle a larger workload, thus keeping applications responsive and efficient as demands increase.
The Role of Data Structures and Algorithms in Data Analytics
Data analytics hinges on the effective manipulation and interpretation of data. Here, data structures and algorithms become not just tools, but the very backbone that supports sophisticated analytical efforts. It’s fundamental to appreciate how the interplay between these two elements lays the groundwork for efficiently extracting insights from vast datasets. Understanding their role can enable software developers, IT professionals, and data scientists to handle data more intelligently.
Selected data structures dictate how data is organized, while algorithms provide the methods for processing that data. With the digital age bombarding us with data at a rapid clip, businesses require robust tools for analysis. Consider, for example, how a hash table can allow quick data retrieval while a tree structure may enable hierarchical data visualization. The selection of the right configuration can vastly improve not only the speed of computations but also the intuitive nature of how information is presented and understood.
"Effective data-driven decisions rely fundamentally on the efficiency of data structures and algorithms."
Data Manipulation Techniques
In the context of data analytics, manipulation techniques refer to the operations performed on data to prepare it for analysis. These operations can include filtering, aggregating, transforming, and merging datasets. Here, data structures offer various advantages:
- Arrays allow for quick access to sequential data, making them ideal for slice-and-dice operations.
- Linked lists are flexible and facilitate dynamic data structures where data can be easily inserted or deleted without restructuring the entire dataset.
- Trees organize data hierarchically and can be useful for representing relational data.
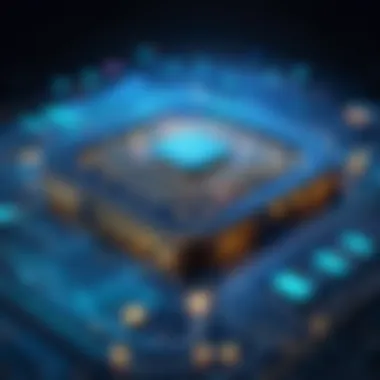
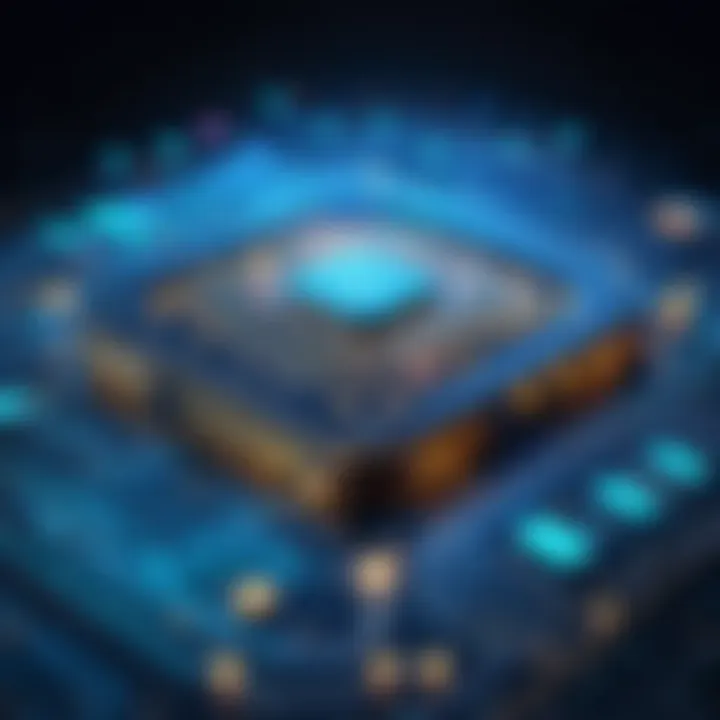
For instance, one common technique, filtering, can be efficiently implemented using a binary tree, where decision-making is accomplished by evaluating conditions at each node. This approach not only speeds up the data retrieval process but also contributes to overall performance, particularly when dealing with large datasets. Moreover, the ability to traverse data structures efficiently means analytics teams can produce reports and insights in a timely manner, which can prove invaluable in a fast-paced environment.
Optimizing Data Queries
Crucially, optimizing data queries requires an understanding of both data structures and the algorithms that operate on them. A well-constructed query leverages the underlying data structure to minimize the number of data accesses needed. For instance, using B-trees in database indexing can significantly speed up queries because they minimize disk access and allow for rapid searching and updating.
Some key considerations for optimizing queries include:
- Choosing the right indexes: Effective indexing dramatically reduces query time.
- Utilizing caching mechanisms: By implementing caching for frequently accessed data, the need for repeated data fetching is minimized, enhancing performance.
- Employing efficient joins: Understanding how to efficiently join tables can lead to considerable improvements in runtime.
In practical applications, a well-tuned SQL query that employs proper joins backed by a sound index structure can yield results in milliseconds rather than seconds, greatly benefitting data-driven decision-making processes. When executed under optimal conditions, the alignment of data structures and algorithms elevates a data analyst’s ability to extract meaningful insights, enabling organizations to stay ahead in the competitive landscape.
Emerging Trends in Data Structures and Algorithms
The rapidly evolving landscape of technology has created a need for programmers and developers to keep a vigilant eye on the emerging trends in data structures and algorithms. Understanding these trends can significantly enhance one’s ability to devise efficient solutions and maintain code quality. As software development grows more complex, grasping the relevance of new advancements in this realm is essential for maximizing both performance and ethical considerations.
Algorithmic Bias and Ethics
Algorithmic bias refers to the unintended consequences that arise when algorithms produce biased results, often leading to unfair or discriminatory outcomes. This issue occurs when the data fed into algorithms contains implicit biases, reflecting societal prejudices and existing inequalities. For instance, algorithms used in hiring processes may inadvertently favor certain demographics if trained on historical data that shows preference toward a particular group.
It's crucial to recognize that the structures housing these algorithms—be they decision trees or neural networks—carry the imprint of human decisions tied to data curation. The ethical approach involves implementing rigorous testing methods to uncover and address biases before they can affect real-world applications. The practice of transparency in algorithm development also plays an important role. Following are key considerations for addressing algorithmic bias:
- Data Quality: Ensuring diverse and comprehensive datasets can mitigate bias in initial stages.
- Regular Audits: Conducting routine evaluations of algorithms and data pipelines to identify and rectify biases whenever necessary.
- Diverse Teams: Assembling diverse teams in the development process ensures varied perspectives, leading to more rounded algorithms.
Adapting these practices can create more equitable technology, setting a standard for responsible development in the industry.
"In the development of algorithms, a stitch in time saves nine. Addressing biases early prevents larger issues later on."
Impact of Machine Learning
Machine learning (ML) has reshaped the way developers approach data structures and algorithms. With the ability to learn and adapt from vast datasets, ML introduces both opportunities and complexities in data handling. One significant trend is the rise of adaptive algorithms, which can modify their behavior based on real-time data. This adaptability can lead to enhanced user experiences in applications ranging from personalized content recommendations to dynamic pricing models.
The interplay between machine learning and data structures is particularly noteworthy. Traditional data structures may need to evolve, accommodating the nuances introduced by ML algorithms. Here are several examples:
- Specialized Data Formats: As in the case of graph databases designed for powering social networks, which differ vastly from conventional relational databases.
- Hybrid Structures: Utilizing a combination of data structures can optimize performance. For example, integrating hash tables with trees can permit accelerated search operations.
- Dynamic Resizing: The ability of certain data structures to resize themselves (like dynamic arrays) can play a crucial role in managing machine learning models that evolve over time.
Developers embracing these trends can ensure their applications remain relevant and efficient, reflecting a necessary shift toward automation and algorithmic learning in today's digital fabric.
Best Practices for Implementing Algorithms
When it comes to implementing algorithms, adhering to best practices is akin to laying a solid foundation before erecting a structure. Implementing algorithms with discipline not only enhances functionality but also paves the way for future scalability and maintainability. In the ever-evolving field of software development, juggling efficiency and clarity becomes paramount. This section will outline specific elements, benefits, and considerations surrounding the best practices in algorithm implementation.
Code Optimization Techniques
Crafting an algorithm isn't merely about getting it to work; optimizing it is where the rubber meets the road. Optimization refers to refining code to produce the quickest results while consuming the least resources. In other words, it's about making your code run like a well-oiled machine.
Several effective techniques can be utilized to achieve this:
- Choosing the Right Data Structures: The crux of effective optimization lies in selecting the right data structure tailored to the algorithm's requirements. For instance, using a hash table can reduce lookup times, while choosing arrays may speed up traversal operations.
- Reducing Redundant Calculations: Calculating the same value multiple times is like reinventing the wheel. Simple techniques such as memoization can significantly cut down processing time by storing previously computed results.
- Loop Optimization: Carefully examining loops can lead to substantial performance gains. A good practice is to minimize the operations inside loops to the minimum necessary and break them early when possible.
- Parallel Processing: Sometimes, splitting tasks among various threads or processors can yield speedier execution. This approach is particularly effective for computationally heavy operations.
The benefits of these optimization techniques extend beyond mere speed. Improved efficiency often leads to better user experiences, reduces server load, and can lead to significant cost savings in cloud computing environments.
Testing and Validation
Even the best-written algorithm may turn out to be a hot mess if not properly tested. Testing and validating algorithms ensure that they work as expected and meet defined requirements under various conditions. This involves several layers of scrutiny.
- Unit Testing: Focusing on individual components or functions within your algorithm helps to catch bugs early in the development process. Comprehensive unit tests can also serve as a form of documentation, clarifying how each part of your code behaves.
- Integration Testing: Once individual units pass muster, it's crucial to verify that they integrate well to form a cohesive system. Integration tests check for interface flaws and data flow across components.
- Functional Testing: This type of testing looks at the algorithm from an end-user perspective. It validates whether the algorithm provides the expected outputs for given inputs.
- Performance Testing: Even a perfectly functioning algorithm won't serve its purpose if it takes too long to deliver results. Measuring execution time and resource consumption is essential to ascertain performance benchmarks.
In summary, the combination of code optimization techniques and rigorous testing forms the backbone of successful algorithm implementation. Without these practices, you risk creating products that are not only inefficient but also potentially full of avoidable errors. Adopting a disciplined approach will help ensure that algorithms not only function but perform at their best, thereby propelling your software solutions into a league of their own.
To keep track, always compile a performance report post-testing. It's a helpful reference for future projects and serves as a guide for ongoing enhancements.
By emphasizing these best practices, tech professionals and software developers can elevate their coding standards, leading to superior software outputs.
Summary and Closure
In concluding our exploration of data structures and algorithms, we encapsulate the essence of what we’ve discussed throughout the article. This section serves as an opportunity to reflect on the critical role these concepts play in modern software development, data management, and the technological landscape overall. By distilling the main ideas into actionable insights, we can appreciate the implications of each topic and how they interconnect to form a cohesive understanding of the field.
The discussion begins by establishing the foundational definitions and historical context, allowing readers to grasp the evolution of data structures and algorithms. Understanding their significance is paramount for any developer or data scientist aiming to excel in their work. A robust grasp of these principles enables professionals to make informed decisions that optimize performance and enhance the efficiency of their software solutions.
One notable aspect we emphasized is the variety of data structures available, from linear to non-linear formats, each with unique attributes that influence their use. The choice of a specific data structure can make or break a solution, and thus it is imperative to align this choice with the specific needs of the application.
Furthermore, we explored common algorithms that developers encounter, such as sorting and searching techniques. These algorithms are not just theoretical constructs but practical tools that significantly influence our ability to manipulate and access data effectively.
We also touched on how these foundational elements relate to larger trends, including cloud computing and machine learning, illustrating the dynamic interplay between data management and technological advancement. An acknowledgment of these trends is key as we look to the future of software development, where the ability to manage data efficiently is more crucial than ever.
Finally, the best practices outlined present a roadmap for implementing these concepts effectively. Emphasizing techniques for code optimization and robust testing will prepare software developers to face the challenges of today and tomorrow with confidence.
Summary: Mastery of data structures and algorithms is not merely academic; it is a professional imperative for those engaging with software that needs to stand the test of both time and scale.
In essence, this article has sought to equip readers with a comprehensive toolkit—one that empowers them to tackle real-world problems with a clear understanding of the principles underlying software development.
Key Takeaways
- Foundational Knowledge: A solid understanding of basic data structures like arrays, linked lists, and trees is essential for writing efficient code.
- Algorithm Selection Matters: Choosing the right algorithm for a task can vastly improve application performance.
- Real-World Applications: Concepts discussed are not only theoretical; they have practical implications in cloud computing and data analytics.
- Stay Current: As software trends evolve, ongoing education in emerging techniques and methodologies is critical.
- Practice Makes Perfect: Regular implementation and testing of these methods lead to better coding practices and a deeper understanding.
Future Directions
As we look ahead, several promising trends in data structures and algorithms warrant attention:
- AI and Machine Learning: Emerging technologies are harnessing advanced data structures to enhance machine learning algorithms, offering the capacity for more nuanced data handling and processing.
- Data Privacy and Security: With increasing concerns around data breaches, the role of algorithms in ensuring data safety is more critical than ever.
- Distributed Systems: As cloud infrastructures grow, understanding data management in distributed contexts will become essential.
- Optimization Techniques: Ongoing research into algorithmic optimization could revolutionize how we approach complex problems, particularly in resource-constrained environments.
- Ethical Considerations: The ongoing dialogue about algorithmic bias underscores the need for ethical frameworks within software development, emphasizing fairness and accountability in algorithm design.
In summary, the landscape of data structures and algorithms continues to evolve, playing a pivotal role in shaping the future of technology. Staying abreast of these developments while honing foundational skills ensures readiness for the challenges and opportunities that lie ahead.
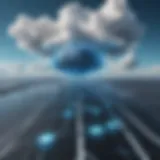
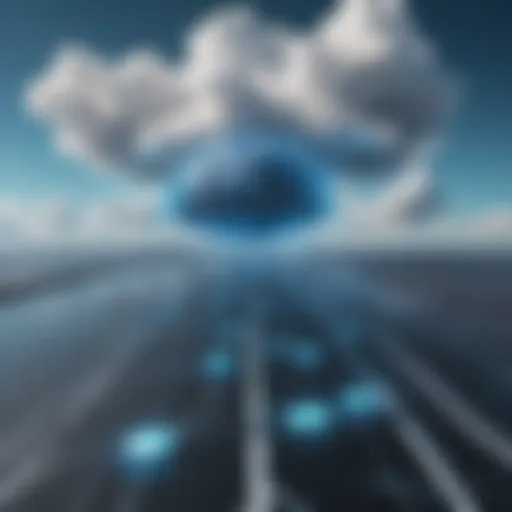