CUDA Programming: Enhancing Performance in Computing
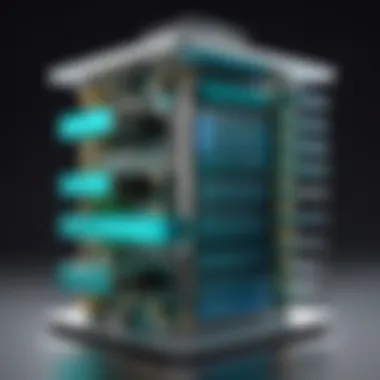
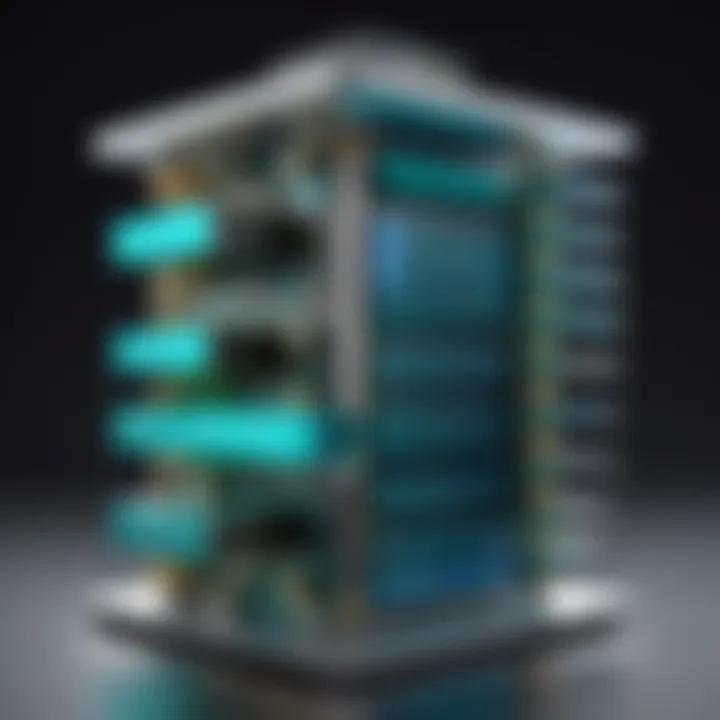
Intro
In the realm of high-performance computing, leveraging the capabilities of GPUs through CUDA programming has become increasingly vital. This article delves into the ins-and-outs of CUDA, designed to meet the needs of software developers and engineers eager to improve their applications’ performance. Understanding how to tap into the immense resources provided by modern GPUs could mean the difference between a sluggish application and one that operates at lightning speed.
CUDA, which stands for Compute Unified Device Architecture, is not merely a buzzword; it serves as NVIDIA's parallel computing platform enabling programmers to use GPU resources for general purpose processing. The power of CUDA lies in its comprehensive architecture and development techniques which are quite different from traditional CPU programming. By tapping into parallel processing, CUDA allows programmers to maximize efficiency and enhance performance across various computing tasks.
As we explore CUDA further, we will demystify its core concepts and delve into memory management, optimization strategies, and advanced programming techniques. Each of these areas plays a crucial role in developing robust applications capable of meeting modern computing demands. Alongside theoretical insights, this article will culminate in practical examples and best practices.
The target audience includes software developers, IT professionals, data scientists, and tech enthusiasts who seek to elevate their computational strategies using CUDA.
Prolusion to CUDA Programming
Understanding CUDA programming is like cracking a code that reveals the hidden potential of high-performance computing. CUDA, short for Compute Unified Device Architecture, was born from the innovative mind at NVIDIA. This platform, which allows developers to tap into the raw power of GPUs, has transformed computing practices, especially in fields that rely on extensive data processing, like scientific research, machine learning, and graphics computations.
CUDA hasn't just made programming easier for developers, it's fundamentally changed the way we approach parallel tasks. The importance of CUDA lies in its ability to enable programmers to take advantage of the parallel processing capabilities of modern GPUs. The leap from traditional CPU-based computing to a more dynamic and responsive architecture is nothing short of revolutionary. The blend of programming flexibility with the unmatched performance of GPUs opens doors to innovative applications and solutions.
What is CUDA?
CUDA is NVIDIA's parallel computing architecture that allows software developers to harness the power of GPUs for general-purpose computing. In simpler terms, CUDA provides a platform that turns a GPU into a parallel co-processor, which executes large blocks of code concurrently, thereby significantly speeding up computations. Instead of forcing time-consuming data processing tasks through CPUs, developers can offload these tasks to the GPU, allowing the CPU to handle other processes.
This ecosystem employs C, C++, and Fortran programming languages, giving developers familiar tools to work with. With CUDA, parallelization is not just an optional enhancement, but a fundamental process that can lead to performance boosts that would otherwise remain unrealized.
The Evolution of CUDA Technology
Since its inception, CUDA technology has evolved significantly. In the beginning, it was a niche product aimed primarily at gaming and graphic design industries. However, as computational needs grew in fields such as biology, physics, and data analytics, the focus shifted. The introduction of applications that could leverage GPUs - such as deep learning frameworks like TensorFlow and PyTorch - has pushed CUDA into the mainstream.
With each iteration, CUDA saw improvements in compatibility, performance, and ease of use. Innovations like Unified Memory, which simplifies memory management between CPU and GPU, reflect an ongoing commitment to address the practical challenges faced by developers. The rapid evolution isn't just about maintaining pace with technological advancements but setting the stage for new possibilities in various computational fields.
Key Features of CUDA
The standout features of CUDA make it an essential tool in the computing arsenal:
- Simplicity and Control: CUDA allows developers detailed control over hardware while providing high-level abstractions for ease of programming.
- Massive Parallelism: Unlike traditional programming models, CUDA allows the execution of thousands of threads concurrently, enabling dramatic performance enhancements for computation-heavy tasks.
- Rich Ecosystem: The CUDA software ecosystem includes a variety of libraries, compiler tools, and debugging support which facilitate the development of efficient applications.
- Interoperability: CUDA can work seamlessly with other frameworks and languages allowing for a smoother integration into existing systems.
- Optimization Capabilities: With tools for profiling and analysis, developers can optimize their applications for greater performance, identifying bottlenecks and enhancing execution speed.
Developing with CUDA is not just about enabling parallel processing; it’s about pushing the boundaries of what we thought was possible with computational power.
The CUDA Programming Model
The importance of the CUDA Programming Model is paramount as it lays the foundation for how developers can leverage the massive parallel processing power provided by GPUs. It serves as a bridge between programming techniques and the underlying GPU architecture. Understanding this model allows developers to optimize their applications effectively, tailoring them to take full advantage of the capabilities of modern GPUs. The CUDA model emphasizes the concept of parallelism, highlighting how tasks can be divided into smaller units that can be executed simultaneously. This shift in mentality is crucial for achieving high-performance computing.
Threads and Blocks in CUDA
At the heart of CUDA's innovation are threads and blocks. Each thread is akin to a tiny worker, executing instructions and handling a portion of the computation. By grouping these threads into blocks, developers create a manageable structure that reflects the hierarchical execution model of CUDA. Each block can contain up to 1024 threads, and they can cooperate to share data through shared memory, which allows for faster access than global memory.
This architecture enables a great deal of flexibility in programming. For example, in a graphical rendering application, a block of threads can be assigned to calculate the color of pixels on a screen, allowing multiple pixels to be processed in parallel. When employing CUDA, one should keep thread divergence in mind; different threads may take different execution paths, reducing performance. Thus, the design of algorithms should aim for uniformity among threads to ensure a smooth execution flow.
Kernels and Grids
Kernels are another critical component of the CUDA Programming Model. A kernel is a function that runs on the GPU and is executed by many threads in parallel. When you invoke a kernel, you are essentially launching a grid of blocks where each block, in turn, contains multiple threads that carry out computations.
This grid and block configuration allows developers to scale their computations efficiently. They can define the dimensions of the grid and blocks to best suit the computational workload. For instance, if you have a large dataset to process, defining a grid with multiple blocks can lead to a significant decrease in processing time compared to sequential execution. However, it is essential to balance between the number of threads per block and the grid size to optimize occupancy, ensuring that the GPU is well-utilized without being overburdened.
Memory Hierarchy in CUDA
The memory hierarchy in CUDA is a key consideration when programming for performance. It includes several levels ranging from the fastest but volatile registers for individual threads to the relatively slower global memory shared across all threads.
CUDA provides distinct types of memory:
- Global Memory: Accessible by all threads, but with high latency.
- Shared Memory: Faster than global memory, but only shared among threads within the same block.
- Local Memory: Private to each thread, primarily used for local variables.
Developers must thoughtfully employ these different types of memory, as improper use can lead to bottlenecks. A strategic approach could involve using shared memory for frequently accessed data by threads in the same block, while utilizing global memory for less frequently accessed data. This understanding of memory hierarchy helps in crafting applications that not only perform well but are also efficient, maximizing the speed and efficiency of operations across the GPU.
Setting Up the CUDA Environment
Setting up the CUDA environment marks the foundation of any CUDA-based development effort. It's not just about getting the right components installed, but also ensuring that everything works harmoniously. An appropriately configured environment brings about increased efficiency in coding and debugging processes. If you want to leverage the power of GPUs fully, you need to dot your i's and cross your t's in this phase.
Hardware Requirements
To embark on your CUDA programming journey, one of the first considerations is the hardware. Not any GPU will do, as CUDA has specific compatibility requirements. Generally speaking, a CUDA-capable GPU from NVIDIA is essential. Let's consider a few important pointers:
- GPU Compatibility: Look for NVIDIA GPUs that support CUDA. Popular options include the GeForce GTX and RTX series, Tesla, and Quadro variants.
- Compute Capability: Each GPU comes with a compute capability version. The higher the version, the more features are supported. Aim for at least compute capability 2.0, but models 6.0 or higher provide better performance.
- System Specifications: Beyond the GPU, ensure your machine has ample RAM and a decent CPU. A minimum of 8GB RAM and a modern multi-core processor will help.
Getting your hardware right is crucial. Remember that if your GPU is the weak link, even the best-written code won't yield desired results.
Software Installation and Configuration
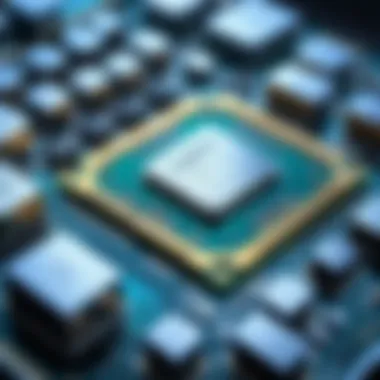
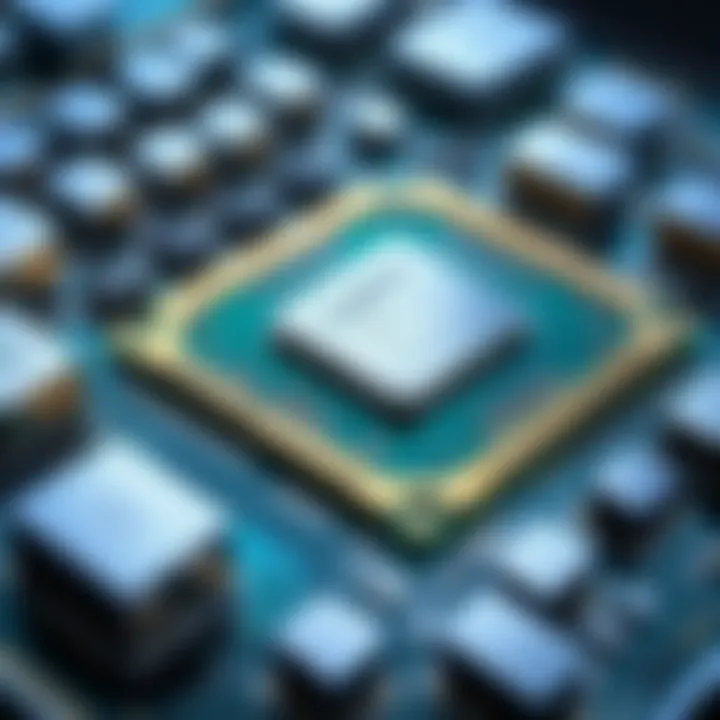
Once the hardware is sorted, it's time to turn to the software. This step may seem straightforward but can sometimes feel like you're trying to put a square peg in a round hole. Here’s what to keep an eye on:
- CUDA Toolkit: Download and install the most recent version of the NVIDIA CUDA Toolkit. This toolkit is your treasure chest, containing libraries, debugging, and optimization tools.
- Drivers: Ensure that you have the latest NVIDIA drivers installed. Outdated drivers can lead to significant performance bottlenecks.
- Configuration: After installing, don’t forget to add CUDA to the system PATH in your environment variables. This is often a step that’s overlooked yet crucial for smooth compilation and execution of CUDA applications.
"The only way to do great work is to love what you do." - Steve Jobs
The same goes for CUDA; when the setup goes right, it feels seamless.
Tools and Libraries for CUDA Development
An expansive ecosystem surrounds CUDA programming, offering various tools and libraries that enhance development efficiency. Here are some vital resources to consider:
- cuDNN: If deep learning is your game, NVIDIA’s CUDA Deep Neural Network library is indispensable. It optimizes neural network training, allowing for faster convergence.
- Thrust: This C++ template library simplifies parallel algorithms such as sort and scan, making your life easier when implementing complex algorithms.
- Nsight: The NVIDIA Nsight suite provides a set of tools for debugging, profiling, and analyzing applications.
As you get familiar with these tools, you'll notice how they can elevate your CUDA projects from decent to extraordinary. The right mix of libraries can make all the difference, enabling you to write cleaner and more efficient code.
Basic CUDA Programming Concepts
The significance of understanding Basic CUDA Programming Concepts cannot be overstated for anyone looking to utilize the power of NVIDIA GPUs. These foundations provide the groundwork necessary for developing efficient CUDA applications. Without delving into the basic concepts, developers may find themselves wrestling with complex algorithms without fully grasping the underlying mechanics of CUDA execution.
This section aims to bridge the gap between theoretical understanding and practical implementation. As we break down these concepts, we'll explore how they empower programmers to unleash greater performance from their applications. By grasping these basics, developers can not only write programs more effectively but also optimize them to reduce latency and improve throughput.
Writing a Simple CUDA Program
Writing a simple CUDA program serves as an essential first step for those venturing into GPU-based programming. Let's consider a basic kernel that adds two arrays. This will help illustrate the structure and mechanics of CUDA programming.
Here's a simple code snippet:
In this code, we allocate memory for the arrays on both the host and device, then initialize the arrays. The kernel performs the addition concurrently across multiple GPU threads, allowing each thread to operate on a different element of the arrays.
Writing this small CUDA program not only familiarizes developers with the syntax and structure but also highlights the key CUDA concepts: memory allocation, kernel invocation, and data transfer. Once the basics are grasped, programmers can gradually increase complexity by using more sophisticated algorithms and optimizing their workflows.
Error Handling in CUDA
Error handling in CUDA programming is crucial not just for ensuring the correctness of the application, but also for debugging and optimizing performance. Building robust CUDA programs requires an understanding of how to catch and manage errors that may arise during execution.
In CUDA, after every API call, you should check the return value. The typical practice is to define a macro that simplifies error checking. The following snippet can serve as a guideline:
Using this macro, you can simply add after your CUDA calls to catch errors effectively. If anything goes awry, it prints a helpful error message and exits the program properly.
The intricacies of CUDA can sometimes lead developers down the wrong path. For instance, failing to handle memory allocation, not synchronizing kernels properly, or overlooking data transfer between the host and device can cause silent failures or much harder-to-trace bugs. Addressing these errors explicitly makes for smoother, more efficient development.
"Error handling in CUDA isn't just a safety net; it's the lifeline for developing reliable GPU applications."
By integrating comprehensive error handling into your development practices, you'll secure smoother operation and pave the way for enhance efficiency and performance in your CUDA applications. \n Understanding these foundational elements lays the groundwork for deeper exploration into optimization and advanced techniques in CUDA programming.
Memory Management in CUDA
Memory management is a cornerstone of efficient CUDA programming. As the performance of a CUDA application hinges significantly on how memory resources are managed, understanding this topic is vital for those delving into the realm of high-performance computing. In CUDA, managing memory isn't just about allocating space; it encompasses a set of strategies and best practices that can optimize data access patterns, minimize latency, and maximize throughput, all of which are key to harnessing the true power of GPUs.
In this section, we will uncover two main aspects of memory management: managing global memory and utilizing shared memory. Each plays a pivotal role in how CUDA applications perform, influencing everything from speed to resource allocation.
Managing Global Memory
Global memory in CUDA is akin to a vast ocean, where developers can swim pools of data. This memory space is accessible by all threads across all blocks, making it a vital part of CUDA's architecture. However, while it provides large capacity and availability, it's important to remember that not all access to global memory is created equal.
When threads access global memory, they do so at varying speeds. Uncoalesced memory accesses can slow down your computations considerably, creating a bottleneck that derails performance. Here are some strategies to manage global memory efficiently:
- Coalescing Accesses: Grouping memory reads and writes by threads can dramatically improve efficiency. When threads in a warp read sequential addresses, transactions combine, leading to reduced bandwidth consumption.
- Memory Alignment: Aligning data structures in global memory allows for more efficient access. Ensure that data types are aligned to the size of the access patterns. For instance, structuring data in 32-byte or 64-byte sections can yield significant performance gains.
- Minimize Transfers: Keep the data in global memory as little as possible. Use shared memory or registers whenever feasible to reduce global memory traffic.
By applying these strategies, a programmer can elevate their application's performance while dodging the pitfalls of poor memory management.
Utilizing Shared Memory
Shared memory in CUDA is akin to a fast lane for data sharing among threads within the same block. This type of memory is on-chip and can be accessed much faster than global memory, allowing for rapid communication and data storage. Given its speed advantage, using shared memory can lead to significant performance boosts, especially in applications requiring frequent data sharing.
To effectively use shared memory, here are some imperative considerations:
- Data Locality: Try to keep frequently accessed data in shared memory. Doing so reduces the number of accesses to slower global memory, enhancing overall performance.
- Bank Conflicts: Beware of bank conflicts when accessing shared memory. CUDA partitions shared memory into banks; if multiple threads access the same bank simultaneously, it can slow down performance. Aim to access memory in a way that spreads access across banks.
- Efficient Use of Size: Shared memory is limited in size per block. Utilize it wisely, determining the optimal size required for your application’s needs. Allocating shared memory for large data sets means cautious planning to avoid overuse.
With a keen understanding of managing global and shared memory, CUDA developers can fine-tune their applications. Investing time into these concepts not only reaps the benefits of increased performance but also aids in streamlining the development process.
"Effective memory management in CUDA programming can be the difference between success and failure in high-performance computing."
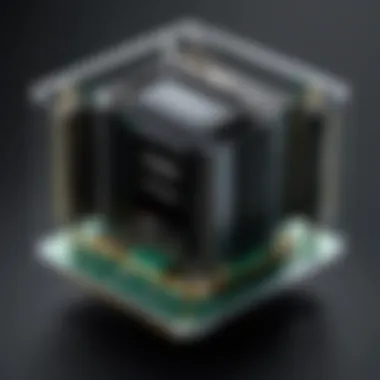
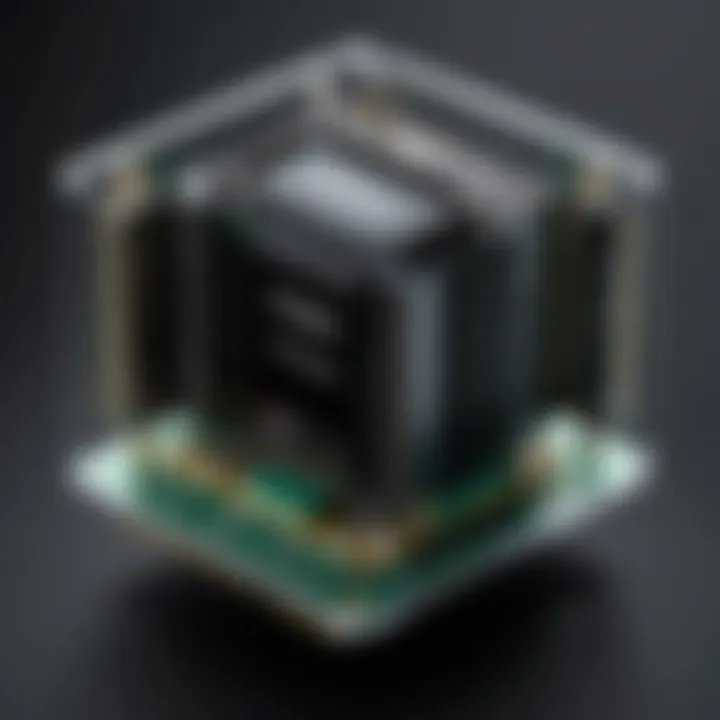
By leveraging both global and shared memory paradigms, skilled programmers can build applications ready to tackle complex computations with ease.
Optimization Techniques for CUDA Programs
Understanding optimization techniques in CUDA programming is essential for achieving peak performance in GPU applications. The right optimizations can lead to substantial improvements, making programs run faster and more efficiently. With the complex nature of parallel computing, optimizations allow developers to navigate challenges that arise from working with massive datasets and numerous threads.
Here are some critical areas to focus on when optimizing CUDA applications:
Optimizing Memory Access Patterns
Memory access can often be a bottleneck in CUDA performance. Optimizing the way threads access memory not only boosts speed but also minimizes latency. One effective strategy is ensuring that threads access memory in a coalesced manner. This means that threads in the same warp (a group of 32 threads) should access contiguous memory addresses whenever possible. Doing this reduces the number of memory transactions the GPU has to make, which can significantly increase throughput.
Furthermore, avoiding unaligned memory access is crucial. If threads access memory addresses that are not aligned to 32 or 64 bytes, it can cause the system to perform inefficiently. Developers should also leverage shared memory when possible. Shared memory is much faster than global memory and helps facilitate communication between threads within the same block.
Utilizing caching strategies can also make a difference, allowing frequently accessed data to be stored for quicker retrieval. Here are some techniques:
- Ensure proper data alignment.
- Use structure of arrays (SoA) instead of array of structures (AoS) for better memory access patterns.
- Profile memory access using tools like NVIDIA’s Nsight to find and address any issues.
Reducing Kernel Launch Overhead
Kernel launch overhead occurs each time a kernel is invoked from host code. This time can add up quickly, especially in cases where kernels are launched frequently in a loop. One way to tackle this is by minimizing the number of kernel launches. Take advantage of larger data sets per kernel execution by combining multiple operations into a single kernel.
Also, consider the use of streams for concurrent execution. By leveraging streams, developers can overlap kernel execution with memory transfers. This means, while one kernel is processing, another can simultaneously transfer data between the host and device, effectively hiding some of the launch overhead.
Another practical approach is to utilize the Unified Memory feature. This allows the developer to allocate memory accessible to both the CPU and GPU once, minimizing the need for multiple kernel launches and data transfers. By adopting such strategies, developers can ensure that kernel launches do not become a significant performance hindrance.
Strategies for Maximizing Parallelism
Maximizing parallelism is at the heart of effective CUDA programming. The challenge lies in ensuring that all available GPU cores are utilized efficiently. One fundamental approach is to increase the number of blocks per grid. If there are more active blocks than the number of streaming multiprocessors (SMs) available, the GPU can switch between blocks rapidly, keeping those cores busy.
Utilizing dynamic parallelism can also enhance the performance of some applications. This feature allows kernels to launch other kernels, enabling more flexible programming capabilities. However, it’s essential to weigh the benefits against the associated overhead, as it can become complicated very quickly.
Establishing the appropriate work per thread is another point to consider. Too little work assigned per thread can lead to underutilization, while too much can lead to long execution times per thread. Finding the right balance ensures that threads complete their tasks efficiently while fully leveraging available hardware.
Effective optimization techniques are akin to tuning a finely crafted engine; each adjustment can lead to significant gains in performance.
For further detailed discussions on CUDA optimization, you can refer to resources like Wikipedia or dedicated forums on Reddit.
Incorporating these techniques not only enhances individual applications but fosters a deeper understanding of how to navigate the intricate world of GPU computing.
Advanced CUDA Techniques
When discussing CUDA programming for high-performance computing, it becomes clear that the advanced techniques are where the magic happens. These techniques unlock the full potential of GPU architecture, allowing developers to execute complex tasks with remarkable speed and efficiency. In this section, we'll break down two pivotal areas: Multi-GPU Programming and the application of CUDA in deep learning.
Multi-GPU Programming
Utilizing multiple GPUs is a game changer, particularly in computational tasks requiring significant processing power. By harnessing the capabilities of more than one GPU, a developer can drastically improve the throughput and efficiency of applications. This approach is particularly relevant in areas like scientific simulations, 3D rendering, and financial modeling where large datasets and extensive calculations are the norm.
To implement multi-GPU programming, one first needs to consider the architecture of the hardware. For example, NVIDIA's NVLink provides high-speed connectivity between GPUs, reducing latency and increasing bandwidth. When coding for multiple GPUs, it's essential to manage how data is shared between them effectively. Strategies often used include:
- Data Parallelism: Divide the workload among the GPUs so that each one processes a subset of the data simultaneously.
- Task Parallelism: Execute different tasks on separate GPUs where independent processes can run in parallel.
A practical implementation might look something like this:
This code snippet demonstrates a simple kernel execution on a single GPU. To scale this for multiple GPUs, you would segment the data and execute the kernel separately on each GPU, managing synchronization and data transfers accordingly. It's not just about coding; it's also about optimizing the communication among GPUs, which can be a bottleneck if not handled properly.
CUDA and Deep Learning
The fusion of CUDA programming with deep learning has opened up a plethora of advancements in the field of artificial intelligence. Deep learning involves handling complex models with millions of parameters, and GPUs excel at this due to their parallel processing capabilities. This synergy allows for faster training of models and more efficient processing of data compared to traditional CPU methods.
Frameworks such as TensorFlow and PyTorch are heavily built upon CUDA programming, enabling them to leverage GPU acceleration seamlessly. With these tools, developers can easily create computations and see vast improvements in performance over standard programming implementations.
Consider the convolutional neural networks (CNNs) often used in image recognition tasks. The underlying arithmetic in CNNs is massively parallelizable, making CUDA a perfect fit. Operations such as convolutions, max-pooling, and activation functions can simultaneously process multiple inputs in the following ways:
- Batch Processing: Training on multiple data samples at once, improving the utilization of GPU resources.
- Layer Parallelism: Distributing the responsibility of different network layers across multiple GPUs.
The adaptability of CUDA in deep learning environments allows for a streamlining of workflows, ultimately enhancing model training speed and efficiency. An example of this could be:
In this short piece of code, a CNN is set up to run on a CUDA-compatible GPU, illustrating just how accessible advanced CUDA programming can be, especially in cutting-edge fields like deep learning.
The future of GPU computing lies in how effectively we can employ advanced techniques such as multi-GPU programming and deep learning integrations. These methods are not just tools but foundational elements for the next wave of technological innovation.
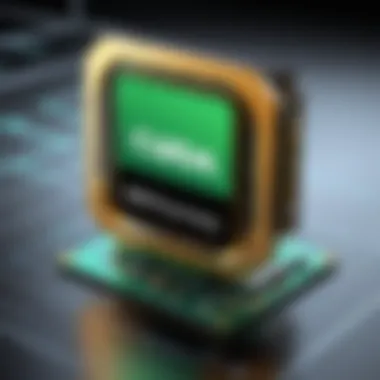
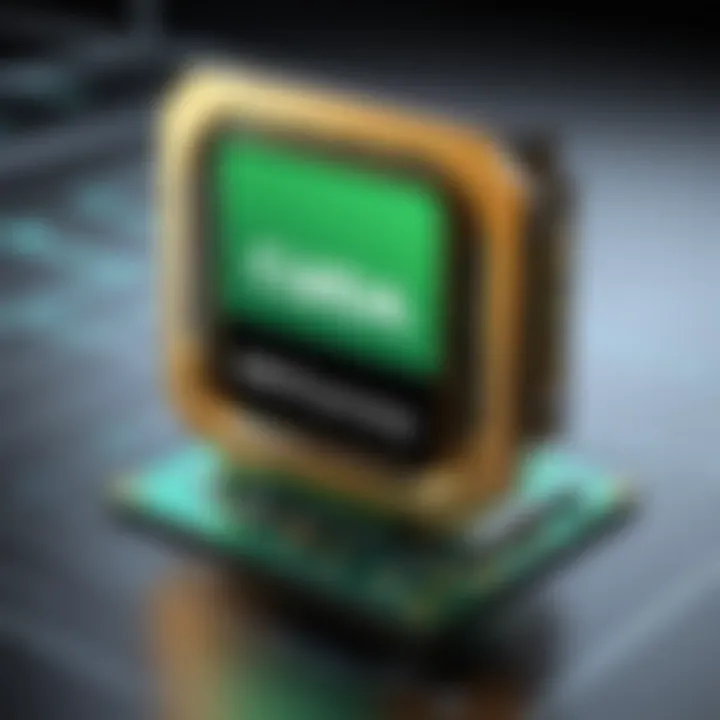
Case Studies and Real-World Applications
Case studies that utilize CUDA programming serve as a vital indication of its capabilities and effectiveness in real-world scenarios. They not only illustrate how CUDA can enhance performance but also provide practical insights into the challenges and considerations of implementation. Leveraging these case studies allows developers and engineers to grasp both theoretical concepts and practical applications seamlessly, making them essential to understanding the real impact of CUDA in high-performance computing.
In this section, we will explore two prominent domains: medical imaging processing and scientific simulations. Each of these areas demonstrates the remarkable potential of CUDA programming in tackling complex problems and enhancing computational efficiency.
Medical Imaging Processing
Medical imaging processing is an area where CUDA shines, providing significant speed improvements in the analysis of complex data sets. With the increasing demand for quicker diagnosis and treatment plans, medical imaging techniques must pivot towards more efficient processing capabilities.
CUDA allows for parallel processing which is crucial for handling large volumes of imaging data. For example, algorithms for MRI or CT scans, which may involve thousands of pixels, benefit immensely from GPU acceleration due to CUDA's capability to process many pixels simultaneously.
One specific application is in the realm of image reconstruction—transforming raw data collected from machines into usable imagery. Traditional CPU-based processes can be time-consuming, sometimes requiring hours to produce clear images. In contrast, with CUDA, reconstruction processes can be executed in minutes or even seconds. This swift turnaround can make a difference in critical care situations.
Furthermore, CUDA supports complex algorithms like deep learning image recognition, enabling systems to not only analyze but also understand images, aiding professionals in diagnostics.
Benefits of CUDA in Medical Imaging:
- Speed: Processes can be executed in a fraction of the time compared to traditional methods.
- Real-time Processing: Allows for immediate analysis during procedures.
- Scalability: Able to handle larger data sets as technology progresses.
Scientific Simulations
Scientific simulations encompass a broad range of disciplines, including physics, chemistry, and environmental science. Here, the computational intensity of simulations requires immense resources, especially when attempting to model complex systems. CUDA comes into play as it allows for distributed computations that can significantly reduce processing time.
For instance, in climate modeling, researchers often run simulations involving thousands of variables and diverse data inputs. These calculations can take weeks using conventional programming approaches. Utilizing CUDA can condense that timeframe down to days or even hours, providing quicker insights into climate behaviors and predictions.
Another specific example is seen in particle physics, where simulating particle collisions demands extraordinary computational performance. With CUDA, researchers can analyze massive amounts of collision data rapidly and efficiently, assisting in uncovering fundamental aspects of matter and energy.
"With CUDA, scientists can simulate phenomena that were once considered impractical due to computational constraints, paving the way for breakthroughs in numerous fields."
Considerations for Using CUDA in Simulations:
- Application Complexity: The inherent complexity in parallelizing certain algorithms may complicate implementation.
- Hardware Requirements: Dedicated GPUs are necessary to fully leverage CUDA's capabilities and benefits.
- Debugging Challenges: Parallel programming can lead to unique debugging challenges that standard programming does not present.
Challenges in CUDA Programming
In the realm of high-performance computing, diving into CUDA programming certainly comes with its own set of hurdles. As software developers and data scientists broaden their horizons with GPU capabilities, they often encounter challenges that demand innovative solutions and thorough understanding. This section not only sheds light on the intricacies of CUDA programming but also emphasizes the importance of overcoming these challenges to unleash the full potential of GPU computing.
When tackling CUDA programming, the first hurdle that often arises is debugging code for parallel execution. Finding and fixing bugs in a concurrent environment can be an uphill battle. Debugging when multiple threads are executing simultaneously introduces complexities that aren’t typically present in sequential programming. A rogue thread can wreak havoc without anyone being the wiser, leading to elusive bugs that take time to identify.
Moreover, profiling CUDA code is critical to understanding performance bottlenecks. With multiple executions running in tandem, pinpointing which part of your kernel is sluggish can feel like searching for a needle in a haystack. Tools like NVIDIA Nsight can help, but employing them effectively requires a solid grasp of both coding and the hardware architecture.
Debugging and Profiling CUDA Code
The importance of debugging and profiling in CUDA programming cannot be overstated. Performance profiling helps in identifying which sections of your code eat up the most resources. Without proper profiling, your program may run sub-optimally, costing you precious time and resources.
A few common techniques for profiling include:
- Using NVIDIA Visual Profiler: This tool helps visualize GPU activities, allowing developers to see where the time is spent.
- Event-based profiling: You can implement hardware performance events to understand how the GPU is handling tasks.
When it comes to debugging, a structured approach is vital. Starting with simple kernels and advancing towards complex codes can minimize debugging fatigue. Also, leveraging error checking after kernel launches can save you from the agony of ‘silent failures’ that lead to unexpected results.
In essence, a sound debugging and profiling strategy is foundational for effective CUDA programming. Focus on incremental changes, validating your logic as you go.
Managing Dependencies and Compatibility
Developing CUDA applications can also be tricky due to the reliance on various libraries and dependencies, which can change over time. Compatibility between different versions of CUDA, your programming environment, and even the hardware can lead to unexpected issues. This makes it crucial to keep all components in sync.
Key considerations include:
- Version Mismatch: Ensuring that your CUDA version aligns with the driver and libraries you are using is essential. Check compatibility matrices provided by NVIDIA.
- Third-party Libraries: Depending on libraries like cuDNN or TensorRT for deep learning can complicate things. They often have strict dependency requirements that, if overlooked, can lead to runtime errors.
Effectively managing dependencies isn’t just about avoiding pitfalls; it’s about ensuring you can leverage the tools available to you fully. Consistent environment setups using containerization tools like Docker can help isolate and manage dependencies efficiently. By controlling your entire development environment, you can mitigate compatibility headaches significantly.
Future of CUDA and GPU Computing
The future of CUDA and GPU computing is painted in vibrant strokes of opportunity and innovation. As industries across the globe strive for increased computational power, CUDA stands at the forefront, its influence growing steadily. The relevance of this topic in the broader context of high-performance computing cannot be overstated. With advancements in architecture and an ever-burgeoning demand for data processing, CUDA is set to redefine what we know about parallel computing.
One key element in this evolution is the continued enhancement of CUDA’s capabilities. The release of new versions consistently introduces optimizations that facilitate more efficient memory usage and better performance scaling across various platforms. This aspect is not merely a technical upgrade; it reflects a natural progression towards more versatile and adaptable programming paradigms. Developers who invest time in mastering these advancements position themselves advantageously in a competitive job market.
Emerging Trends in CUDA Development
As we look ahead, several emerging trends must be acknowledged. One significant trend is the integration of specialized hardware, such as Tensor Cores, which are designed to compute deep learning operations. By utilizing these advancements, CUDA can accelerate machine learning tasks significantly. Furthermore, libraries like cuDNN specifically cater to deep learning operations, allowing developers to harness GPU power fully for neural networks.
Additionally, the penetration of cloud computing introduces new opportunities for CUDA. Providers like Amazon Web Services and Google Cloud Frameworks now offer virtual machines equipped with high-performance GPUs. This shift enables smaller enterprises and individual developers to access computational resources that were once the domain of large organizations, thus democratizing access to advanced processing capabilities. This accessibility will likely spur innovation and experimentation in CUDA applications across diverse fields.
CUDA's Role in the Era of AI
CUDA's significance in the burgeoning field of Artificial Intelligence cannot be overstated. As AI algorithms become more intricate, they typically require substantial computational resources. CUDA’s parallel computing approach is not just an advantage; it is rapidly becoming a necessity for processing vast amounts of data efficiently. From image recognition to natural language processing, CUDA has positioned itself as an essential tool for developers working in AI.
The synergy between CUDA and AI is also reflected in frameworks such as TensorFlow and PyTorch, which seamlessly incorporate CUDA libraries, enhancing computational efficiency. This integration facilitates rapid prototyping and training of models, which is crucial in an industry where time is often of the essence. Observations suggest that as AI algorithms continue to evolve, so too will the role of CUDA in realizing their potential, enabling more complex scenarios to be processed within a reasonable timeframe.
"The advancements in CUDA programming are paving the way for incredible growth in computational sciences, allowing developers to create applications that leverage the power of GPUs like never before."