Master Full Stack Java Development: Comprehensive Guide
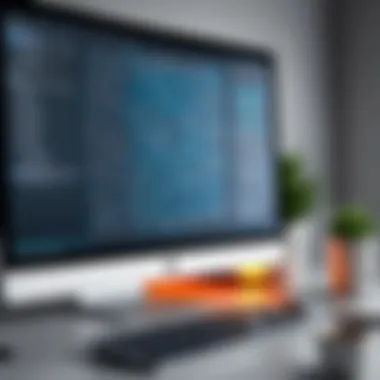
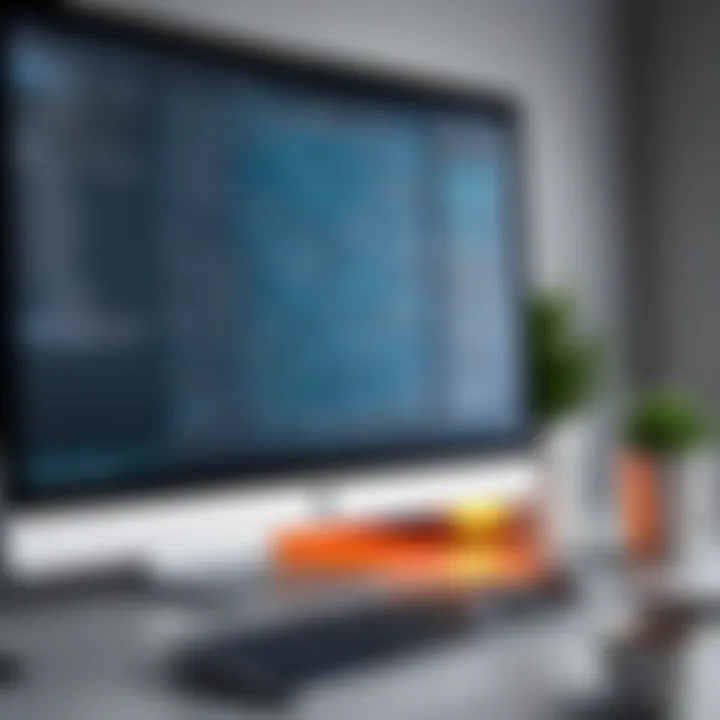
Intro
Full stack development is a comprehensive and structured approach to building applications, encompassing both the front-end and back-end. This practice is increasingly relevant in today's tech landscape, where strong integration between diverse technologies is imperative. With a focus on Java, this tutorial aims to provide an in-depth understanding of full stack methodologies that can elevate proficiency for both newcomers and experienced developers alike.
Java offers a robust framework well-suited for full stack development due to its versatility and vast ecosystem. Developers proficient in Java are capable of creating dynamic web applications that effectively respond to users' needs. By mastering the essential components of full stack development, individuals can become expansive in their ability to develop complete solutions.
Being familiar with fundamentals such as cloud computing and data management technologies is important too. These disciplines interconnect with developers’ tasks and affect the overall performance and scalability of applications. In the following sections, we will delve deeper into various aspects of full stack development using Java, and gain insights for advanced project undertakings.
Overview of software development and cloud computing
Software development denotes the systematic application of methodologies to create and maintain software. Its scope is vast, but for our focus, we examine development processes centered on Java for full stack implementations.
Software development promotes systematic methods in obtaining code that behaves correctly.
Definition and importance of the technologies
Cloud computing plays a pivotal role in modern software development. It refers to delivering hosted services over the Internet. Java frameworks are robust, and successful integration with cloud services enhances the scalability and flexibility of applications. Full stack developers can create applications that leverage cloud functionalities for storage, computation, and collaborative features.
Key features and functionalities
Utilizing Java in concert with cloud solutions enables:
- Rapid Deployment: Applications can be deployed quickly across a wide range of environments without incurring substantial costs.
- Scalability: More capacity can be provisioned as app user demand changes.
- Cost-efficiency: Pay-per-use pricing models reduce operational expenses significantly.
Use cases and benefits
Some noteworthy use cases that blend cloud technologies with full stack development include:
- Web applications that require high availability and low latency.
- Mobile backend solutions efficiently interfaced via RESTful API.
- Data analytics applications leveraging cloud data storage and processing power.
Best Practices
To navigate the complexities of full stack development using Java and cloud computing effectively, following industry best practices is essential.
Implementing best practices
- Adhere to SOLID principles: These principles guide developers towards maintaining clean and workable code.
- Monitor app components: Leverage management tools for infrastructure oversight.
- Utilize CI/CD: Continuous Integration and Continuous Deployment allow for narrowing the gap between code updates and product deadlines.
Tips for efficiency
- Opt for modular development
- Engage in regular code reviews
- Write clear documentation
Common pitfalls in development must be noted too. Frequent misunderstandings about framework nuances can lead to complex debugging sessions, a careful approach across each phase is imperative.
Case Studies
Real-world case studies serve to illustrate successful full stack implementations. For example, a notable healthcare application upgraded its infrastructure to utilize Java combined with cloud-based storage and services.
Lessons learned
- The importance of responsive design.
- Maintaining user privacy and data security was non-negotiable.
Insights sought from these examples greatly enrich the learning trajectory, ones worth analyzing for ongoing development work.
Latest Trends and Updates
Remaining current with evolving trends in software development is vital.
- Integrating AI features into applications continues to gain traction.
- Serverless architectures optimize configurations by abstracting server management tasks.
Tracking these innovations will help developers maintain competitive and cutting edge in their work.
How-To Guides and Tutorials
This section provides methodical guides tailored specifically for working with Java in full stack development.
Step-by-step implementation strategies
Begin the journey with understanding Java frameworks. Gradually assimilate advanced methodologies such as:
- Spring Boot for back-end development.
- React or Angular for front-end implementation.
By walking through either practical examples or tutorial sessions defined, it equips developers with necessary proficiencies for real-world application. These codified insights, once explored thoroughly, can escalate development skill sets in gradual, effective measure.
Finale
This journey through full stack Java development underscores the critical knowledge and frameworks necessary to succeed. Understanding not just development but real-world applications and current trends fortifies your development journey
Foreword to Full Stack Development in Java
Definition and Importance
Full stack development is a crucial aspect of modern software engineering. It includes both the front-end and back-end processes that create a cohesive web application. When using Java, a well-established programming language, developers can leverage its versatility and power in their projects. Java's robust architecture, combined with its wide array of libraries and frameworks, makes it an outstanding choice for full stack development.
Understanding full stack development in Java enables developers to engage in every aspect of the application lifecycle. This broad skill set not only enhances job flexibility but also offers career advancement opportunities. Companies often seek professionals who can navigate the Java ecosystem thoroughly, contributing to both client and server-side efforts. The integration of different technologies increases the chances of system coherence and boosts the ability to identify and fix bugs early in the application development process.
Components of Full Stack Development
Engaging with full stack Java development involves understanding various components. These essential elements include:
- Front-end Technologies: This is where user interaction happens. Knowledge of HTML, CSS, and JavaScript is needed to build dynamic user interfaces.
- Back-end Technologies: Involves server-side development using Java frameworks like Spring. This creates the logic behind how requests from the front end are processed.
- Databases: SQL and NoSQL databases play a vital role in storing and retrieving data efficiently. Java developers must be comfortable querying and integrating databases within their applications.
- DevOps Practices: Understanding Git for version control and tools for continuous integration improves teamwork and workflow efficiency.
Understanding these components holistically is crucial. Each part interacts with the others, and being proficient in all aspects results in a more coherent learning experience.
"Full stack development encompasses the full scope of application development, providing insight into every layer of the technology stack."
This empowers developers to take a project from conception to deployment, optimizing the workflow while maintaining quality.
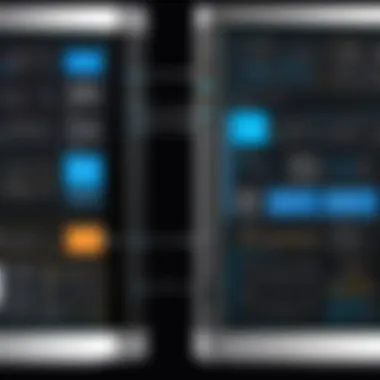
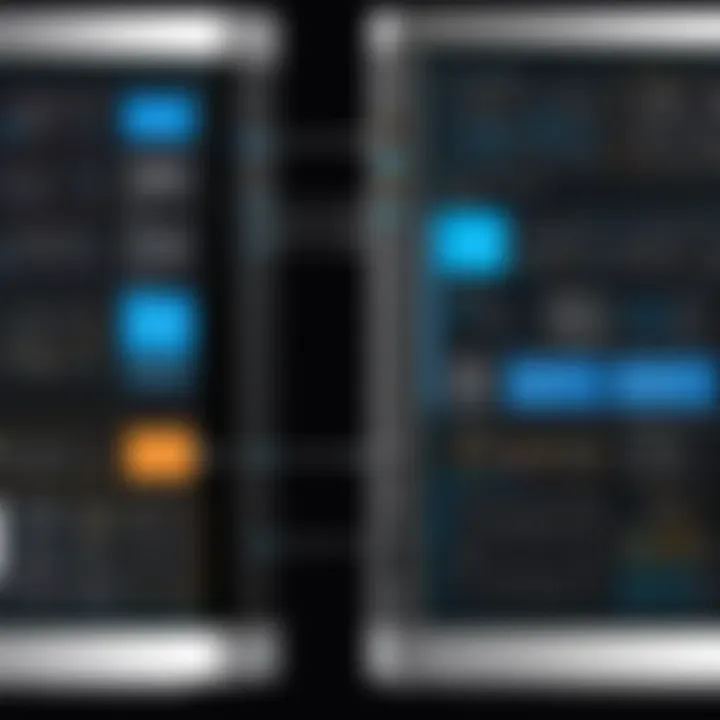
Java Fundamentals for Full Stack Development
Understanding the foundational elements of Java is crucial for anyone aspiring to be proficient in full stack development. Java lies at the heart of many enterprise applications and plays a significant role in server-side logic. This section provides insights into essential components with two key focuses: basic syntax and object-oriented programming principles. These elements are vital in building efficient and scalable applications.
Basic Syntax and Data Types
The Java programming language has specific syntactical rules that developers must learn. This basic syntax defines how different statements and expressions in Java should be structured. Having a firm grasp of these rules is essential for writing clean and understandable code.
Key Points:
- Java is case-sensitive. This means that and would be considered different names.
- Every Java program must have a definition, where the main logic resides in method definitions.
- Data types in Java are divided into two categories: primitive types and reference types.
Primitive Data Types:
- int - Single precision integers.
- boolean - Represents two possible values: true or false.
- char - Represents a single 16-bit Unicode character.
- double - Double precision floating-point numbers.
Reference Data Types:
Reference types are more complex types that point to objects. When working with such types, understanding how memory allocation works in Java is beneficial.
Coding a simple program can enhance your understanding:
This program illustrates basic syntax in action, showcasing how classes and the main method function.
Using proper syntax builds a good foundation to communicate effectively with other developers and ensures readability in larger projects.
Object-Oriented Programming Principles
Java is fundamentally an object-oriented language. This means its architecture supports an object-oriented programming (OOP) approach. OOP allows developers to model real-world scenarios, promoting reusability and organization.
Fundamental Principles of OOP:
- Encapsulation:
- Inheritance:
- Polymorphism:
- Abstraction:
- Refers to bundling the data (properties) and methods (functions) that operate on the data within one single unit.
- Example in Java:
- Establishes a hierarchical relationship between classes, which allows one class to inherit the properties of another.
- This simplifies code and promotes tree-like structures for greater abstraction.
- Allows for methods to perform different functions based on the object that calls them.
- Focuses on hiding complex implementation details and providing a simpler interface for the user.
Utilizing these principles produces cleaner and maintainable code.
The structure and principles of Object-Oriented Programming facilitate building complex applications with greater efficiency. Understanding OOP is essential in any field of software development, but particularly valuable in full stack development, where integration between multiple systems occurs.
Investing time into mastering Java fundamentals will significantly strengthen the proficiency required for exceptional full stack development. These skills form the underpinnings as we explore how Java interacts with both back-end technology and user-facing applications.
Front-End Technologies Integration
Front-End Technologies play a critical role in Full Stack Java Development, serving as the interface between users and applications. Ensuring a cohesive and efficient integration of front-end technologies enhances user experience, elevates performance, and solidifies the overall functionality of web applications. A proficient understanding of these technologies equips developers to harness their potential effectively, making them indispensable in modern web architecture.
HTML and CSS Basics
HTML (HyperText Markup Language) is the backbone of web content. It structures content on the web, allowing developers to define elements like headings, paragraphs, images, and links. A developer must grasp its syntactical elements to create well-structured pages.
CSS (Cascading Style Sheets) controls the look and feel of a web page. Styling elements such as color, font, and layout are managed through CSS. A solid path in integrating HTML and CSS is vital for creating visually appealing and functional interfaces.
Key Elements of HTML and CSS
- HTML Structure: Elements, attributes, and their roles
- CSS Styling: Selectors, properties and how to apply styles effectively
- Responsive Design: Creating fluid layouts that cater to varying device sizes
Why Understanding HTML and CSS Matters
Understanding these fundamental front-end technologies builds the foundation for any further front-end development. It leads to better communication with stakeholders, efficient problem solving, and foundational skills for learning JavaScript.
Foreword to JavaScript
JavaScript is the key scripting language for object-oriented programming within front-end development. It empowers developers to create interactive and dynamic web applications. Given its ubiquity, mastering JavaScript is essential for a robust understanding of front-end integration.
Benefits of Learning JavaScript
- Interactivity: Enhance user engagement with dynamic elements
- Asynchronous Operations: Managing data background both effectively and efficiently
- Wide Adoption: A large ecosystem of libraries and frameworks available for scaffolding projects
Using JavaScript in combination with HTML and CSS furthers the developer's capability to build sleek and performant applications, resulting in enhanced user satisfaction across various platforms.
Frameworks: React and Angular Overview
The rising need for structured, maintainable code has led to the emergence of JavaScript frameworks like React and Angular. Each has its unique strengths and weaknesses. Mastering these technologies can differentiate a proficient developer from a mediocre one.
React: Declarative Approach to UI
React, maintained by Facebook, emphasizes component-based architecture. React allows for the creation of reusable UI components, optimizing user interactions. The simplicity in handling complex UI’s gives it an edge and can dramatically enhance development speed.
Angular: Complete Framework Solution
Angular is a comprehensive framework. It offers a full suite of functionalities such as routing, state management, and form-handling mechanisms. This makes Angular suitable for larger applications where a structured approach is beneficial.
Comparison Between React and Angular
- Flexibility vs Structure: React allows more freedom in application design, whereas Angular provides more pre-structured guidance.
- Performance: React excels with reusable components, but Angular handles larger projects superbly.
- Learning Curve: React may be simpler initially, while Angular comes with a steeper learning cerebral path.
Ultimately, becoming adept in either framework enriches a developer's understanding of front-end technologies, shaping their capacity to deliver sophisticated applications in today's tech landscape.
A well-integrated front-end ensures that user perception aligns more closely with functional reality, translating to higher success rates for projects across different industries.
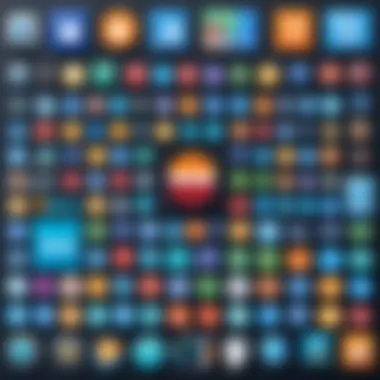
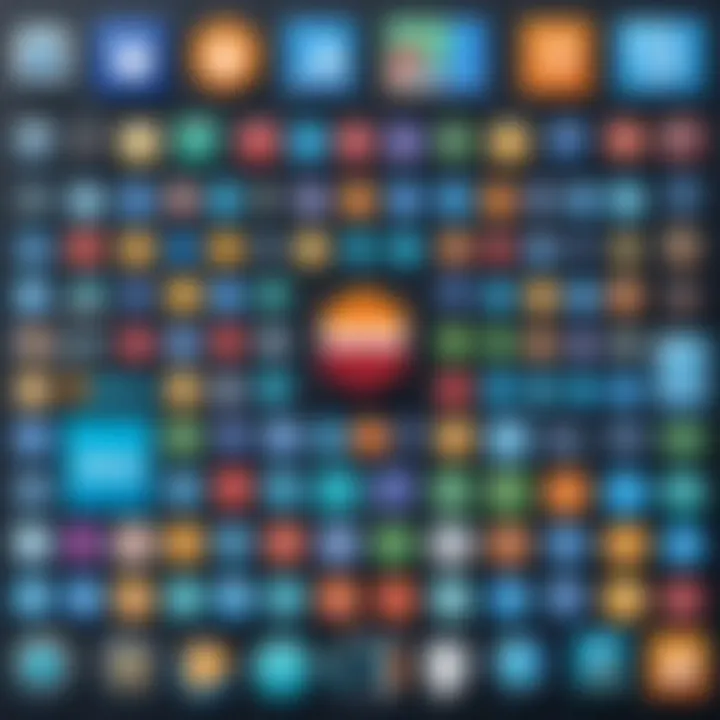
Back-End Development with Java
Back-end development is a crucial component of full stack Java development. It focuses on the server side of an application and includes everything that happens in the server, database, and application logic. Understanding back-end development is important for several reasons. Firstly, it enables the creation of functional applications that can efficiently process requests. Secondly, a solid back-end allows a system to handle various tasks like database communication and server management.
The advantages of back-end development in Java include its robustness and scalability. Java consistently outpaces other languages in portability. Its extensive ecosystem also facilitates the creation of powerful APIs and management systems. As more organizations move towards Java applications, knowledge of back-end technologies becomes increasingly relevant for software developers.
Java Web Technologies
Java Web Technologies furnish the tools developers require to create server-side applications capable of handling client requests. They consist of servlets, JavaServer Pages (JSP), and frameworks like JavaServer Faces (JSF). Understanding these components allows developers to arrange the back-end processes efficiently.
- Servlets: A servlet is a Java class that handles requests and responses in a web application. They allow for processing dynamic data and can interact with databases.
- JSP: This is a technology allowing developers to create dynamically generated web pages based on HTML, XML, or other document types. JSP combines Java code and HTML elements, which can help in content presentation.
- JSF: JavaServer Faces serve as a component-based MVC framework. It helps manage user interface components and simplifies navigation and data manipulation tasks.
Leveraging these technologies can vastly improve application performance and user interaction efficiency, allowing seamless connectivity to databases and retrieval of pertinent data.
Spring Framework Essentials
The Spring Framework stands out as one of the most widely used frameworks for Java web applications. Its modular architecture maneuvers the complexities of development for trademarks, dependency management, and integration. A significant advantage comes from its Inversion of Control (IoC) and Aspect Oriented Programming (AOP) features, which enhance code modularity and usability.
- IoC: This principle allows the framework to manage object creation, supporting the Heath of code management by delegating the responsibility of instance management.
- AOP: It provides a mechanism to separate cross-cutting concerns, such as logging and error handling, promoting better employability of code and itself modular design.
These intrinsic characteristics summarize Spring's commitment to effective developers' management and ensure that programmers can redirect focus to critical functionality, speeding up the development cycles whilst reinforcing application stability.
RESTful APIs Development
Restful APIs are at the forefront of most data exchange architectures today. These APIs not only define how different software components interact but also influence how data is transmitted efficiently with web protocols. REST, which stands for Representational State Transfer, enables communication between client and server while adhering to HTTP protocols.
In back-end development with Java, creating RESTful APIs can be simplified using the Spring Boot framework that automates boilerplate activity associated with API setups. An ideal RESTful API should implement CRUD (Create, Read, Update, Delete) operations. Thus a developer creating such APIs needs to know:
- Mapping endpoints appropriately
- Handling request validations and responses
- Incorporating security measures using token-based authentication such as OAuth.
Moreover, a deliberate consideration for documenting APIs using representations such as Swagger can greatly enhance clarity and usability by illustrating how the API can be consumed by third-party applications.
Building reliable and scalable back-end systems using Java allows developers to effectively deliver modern applications adhering to user demands and industry practices. Boosting understanding and applying these critical technologies constructs a thriving end-to-end development knowledge.
Database Management in Full Stack Java
In the realm of full stack development, database management plays a pivotal role. Efficient data handling is fundamental to building applications that are not only responsive but also robust. A well-structured database ensures that both front-end and back-end components work harmoniously. For developers and IT professionals, mastering database management is essential since data is at the heart of any application.
Prelims to SQL and NoSQL
In database management, two main types of databses commonly come to the fore: SQL and NoSQL. SQL (Structured Query Language) databases like MySQL, PostgreSQL, and Oracle are designed to handle structured data with predefined schemas. These databases provide ACID (Atomicity, Consistency, Isolation, Durability) compliance, which is crucial for transactional applications needing reliability.
On the other hand, NoSQL databases, such as MongoDB and Cassandra, cater to unstructured or semi-structured data, allowing flexibility in schema design. They are built for scalability and can handle vast volumes of distributed data across many servers. Understanding the strengths and weaknesses of both SQL and NoSQL is key to determining which solutions fit specific project needs.
Both SQL and NoSQL technologies offer unique advantages; thus, familiarity with both paradigms enhances any developer's toolkit.
Integrating Databases with Java Applications
Integrating sql or NoSQL databases into Java applications requires a clear understanding of how to communicate with these systems effectively. Java developers usually utilize Java Database Connectivity (JDBC) for SQL databases, enabling interaction through standard queries. Here's a simple example of establishing a conncetion using JDBC:
For NoSQL, libraries suited to specific databases like MongoDB’s Java Driver or Spring Data can be utilized. While writing data, using ORM (Object-Relational Mapping) tools like Hibernate simplifies the conversion of Java objects to database tables. This allows developers to focus on object management without manually handling SQL scripts each time.
Understanding variants of SQL queries enhances efficiency during data retrieval, similarly leveraging query options available in NoSQL databases supports greater flexibility. Ultimately, integrating data management seamlessly into Full Stack Java applications underpins functional effectiveness, making it a fundamental aspect of development.
DevOps Practices for Full Stack Development
In today's fast-paced software development environment, adopting agile methodologies is essential. DevOps, a combination of development and operations practices, bridges the gap between these two areas. It emphasizes collaboration, automation, and continuous improvement. By integrating these practices, modern software teams can release updates and features with high velocity and reliability.
One core aspect of DevOps is enhancing communication across team members. Breaking silos helps streamline development, testing, and deployment processes. Enhanced teamwork also leads to increased accountability and shared objectives. Moreover, employing DevOps practices results in reduced time for reaching the market, ultimately benefiting the end users.
Version Control with Git
Version control systems are crucial for any software development project. Git is one of the most popular version control systems utilized by developers worldwide. With Git, developers can save their code at various stages, facilitating easy access, collaboration, and recovery whenever necessary.
Key benefits of using Git include:
- Branching and Merging: Developers can create branches to experiment with new features without impacting the main codebase. When ready, these changes can be merged seamlessly.
- History Tracking: Git keeps a detailed history of all changes, allowing for easy identification of bugs or reverting to earlier states.
- Collaboration: Shared repositories on platforms like GitHub make it simple for teams to work together, reviewing changes and providing feedback.
Getting started with Git is straightforward. Here is a fundamental sequence of commands to initiate a repository:
This lays the foundation for effective project management throughout the development lifecycle.
Continuous Integration and Deployment Concepts
Continuous integration (CI) and continuous deployment (CD) are crucial practices within DevOps that improve developer workflow. CI focuses on regularly integrating code changes into a shared repository. This practice detects errors quickly, allowing teams to address issues before they escalate.
Key steps in continuous integration include:
- Automated Build: After commit, an automated build system compiles code, checks for errors, and prepares for testing.
- Unit Tests: Running automated tests as part of integrating code ensures immediate feedback.
- Notification System: Alerts developers of any issues that arise during the integration process.
Continuous deployment takes CI a step further. It automates the release of code changes to production environments, helping to streamline how features reach users. This method stresses stability through rigorous testing and code quality, following a strict fail-fast principle - if something goes wrong, quickly revert.
By adopting these CI/CD principles, development organizations can make software delivery more efficient and reliable, leading to better products and higher customer satisfaction.
Effective DevOps culture not only focuses on tools and automation but invests substantially in collaborative practices that can bring continuous improvement throughout the development lifecycle.
Testing and Debugging in Full Stack Java
Testing and debugging are essential processes in full stack Java development. Both serve to improve code reliability, enhance performance, and ultimately, satisfy end-users. Quality assurance, through these practices, eliminates bugs and ensures the system behaves as expected under various circumstances. The relevance of testing is amplified when developing complex applications that integrate multiple layers of technology, such as robust back-end logic and intuitive front-end interfaces.
Effective testing practices save time and resources by identifying issues before they escalate, leading to fewer runtime errors and production problems. Furthermore, debugging allows developers to pinpoint the exact source of an error when testing reveals unexpected behavior. This creates confidence in the application, promoting an iterative and incremental development process.
Unit Testing with JUnit
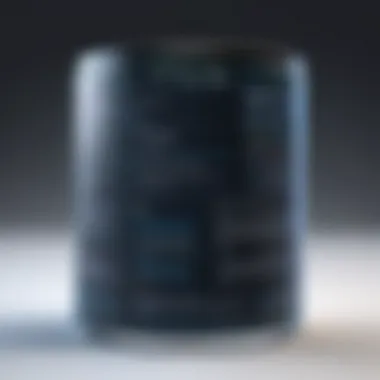
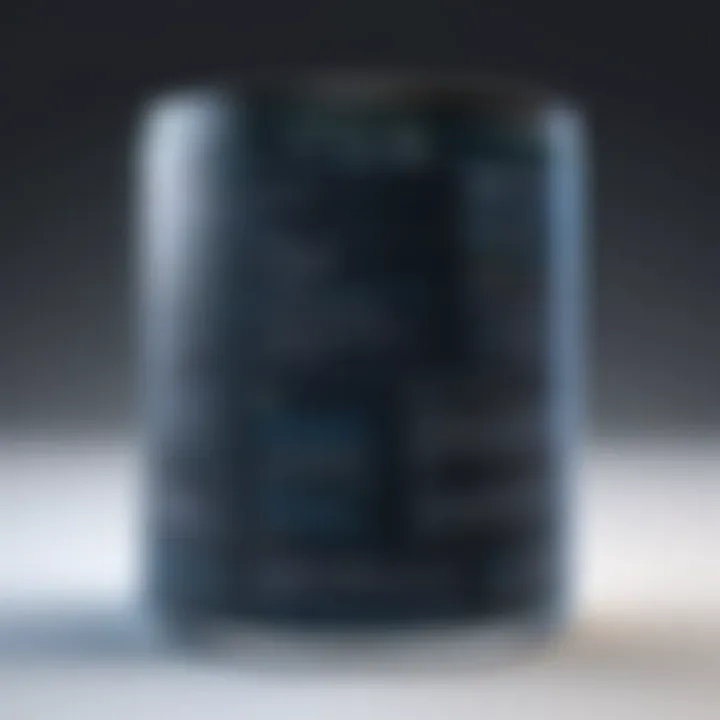
Unit testing is a key approach that focuses on validating individual components of code in isolation. JUnit is arguably the most popular framework for unit testing in Java. It provides an annotation-basedapi for defining test cases, which helps facilitate a clear structure to the testing process.
Benefits of using JUnit:
- Automates running and managing tests.
- Simple integration with build tools like Maven and Gradle.
- Provides clear reports on test results.
Using JUnit simplifies the testing process but challenging unit tests can still arise, especially concerning dependencies. It’s crucial to properly isolate units of code, which often requires adhering to proper coding principles, such as those outlined in object-oriented design.
Implementing unit tests also allows developers to track changes over time and determine where a new error was introduced within the codebase. Here’s a brief example of a unit test using JUnit:
This succinct example underscores how to assert expected outcomes, providing a basic introduction to writing tests with JUnit.
Integration Testing Strategies
Integration testing goes beyond evaluating individual components, as it scrutinizes the interactions between various modules or external systems. Successful integration involves not just verifying that parts work in isolation, but rather that they function harmoniously within an entire system configuration.
Some common strategies include:
- Top-Down Testing: This involves testing high-level modules first and progressively integrating lower-level modules.
- Bottom-Up Testing: Starting with lower-level modules, this method subsequently integrates higher-level modules or components.
- Sandwich Testing: Combining both approaches allows for greater flexibility and thorough structure.
- Big Bang Testing: Integrating all components simultaneously, although practical, poses higher risk due to the broad integration's inherent complexities.
Considerations while performing integration testing include addressing data interaction, handling edge cases, and ensuring the expected results across integrations can meet quality criteria. Enhanced collaborative communication is also key, as all teams working on integrated components must coordinate align the varying sequences properly.
In summary, it's vital to view both unit testing and integration testing as pillars that support overall design integrity. Each layer effectively serves the overarching goal: building dependable software that excels under real-world conditions.
Project Structure and Best Practices
Project structure and best practices are essential topics when diving into full stack development with Java. A coherent project structure significantly influences the ease of understanding, maintainability, and scalability of software projects. In the complex ecosystem of software development, clarity at the initial stages can prevent confusion and costly errors later on. Establishing conventions through best practices helps developers deliver consistent and high-quality code.
Over the course of this section, we will discuss key elements you should consider when structuring your Java project. From arrangement of directory files to selection of naming conventions, these details matter. Adhering to best practices not only streamlines collaboration among developers but also develops a culture of quality software delivery.
Setting Up a Full Stack Java Project
To initiate a robust full stack Java project, attention to the structure is paramount. First, define your project goals clearly. This determine how your application will be built and organized. The folder architecture typically comprises several distinct layers:
- Presentation Layer: Houses front-end components. This is where user interaction and interface design take place.
- Business Logic Layer: Contains application core functionalities and logic.
- Data Access Layer: Responsible for database interactions. This helps separate database concerns from application logic.
Additionally, it is optimal to use build tools like Maven or Gradle for your Java projects. They simplify dependency management and streamline the building process. A unified directory naming strategy, such as lower case for packages, assists in avoiding confusion.
A simple structure would resemble the following:
Following the right structures enables seamless updates. Understand that new features can be integrated without jeopardizing existing functionalities. Therefore, careful planning and adherence to a defined architecture help you scale projects with ease. Remember, failing to plan is planning to fail.
Code Quality and Documentation
Code quality is not just about writing functional code. It is a commitment to write clear, performant, and maintainable code. Following a coding standard such as Google Java Style or Clean Code can enhance your codebase significantly. Here are some good practices to observe:
- Consistency: Follow a uniform style across the project.
- Descriptive Names: Use clear and descriptive names for classes, methods, and variables. This ensures better readability.
- Write Tests: Unit testing enables you to verify that small units of code perform as expected. Incorporating tools like JUnit ensures reliability.
Equally important is documentation. It serves both as a guide for developers and as a future reference for ongoing maintenance. Key documents include:
- Project README: Provide an overview and setup instructions.
- API Documentation: This assists other developers in understanding how to utilize and integrate with your code. Javadoc is widely used for generating documentation in Java projects.
Overall, prioritizing code quality and documentation not only enriches the development process but fosters an atmosphere of professionalism. Having deliverables that are understandable and maintainable reflects much about your capabilities as a developer.
Following a structured approach to project organization cultivates an effective programming environment. Sound practices result in bolstered project longevity and quality deliverables.
Real-World Applications and Case Studies
When mastering Full Stack Development through Java, it is essential to witness how these concepts apply in actual scenarios. Real-world applications are crucial as they translate theoretical knowledge into practical skills. Understanding these applications not only demonstrates the capability of one’s skillset but also exemplifies the potential challenges and mitigation strategies encountered in various projects.
Moreover, observing real-life case studies provides perspective on the different approaches in software development. Each project brings unique requirements and constraints. Thus, learning from specific outcomes adjusts one’s perspective on optimization and efficient resource management.
Successful Full Stack Java Projects
Several projects stand out as examples of well-executed Full Stack Java development. One noteworthy project is the application developed for e-commerce platforms that provides a seamless user experience across devices. These platforms generally require careful integration of Java for the back-end processing with JavaScript frameworks for the front-end interface.
Another example is the banking software solutions that ensure secure transactions and manage robust databases. In such cases, systems leverage enterprise-level solutions, such as the Spring Framework, to build comprehensive services that handle both governmental standards and user demands. The combination of REST APIs with a secure web interface ensure safety and functionality, typical in banking software.
Projects like these demonstrate sufficient user interactions, facilitating improved engagement rates and performance tuning primarily observed through robust back-end processing.
Lessons Learned from Industry Experiences
Actual industry experiences pave the way for valuable insights. Through successful compliance procedures, one learns the significance of adhering to best practices within coding standards, solution architectures, and data protection policies.
For example:
- Effective Communication: Participation in Scrum or Agile methodologies within teams enhances collaboration and unit productivity. Enhanced teamwork leads to timely feedback, which further elevates project quality.
- Infrastructure Consciousness: Awareness of the operational environment where applications run can direct strategies for scaling solutions. Underestimation can lead to failures while deploying unconventional furniture.
- User-Centric Design: Placing emphasis on user feedback directly influences iterations in design and implementation. Techniques such as A/B testing offer environments to understand user preferences distinctly.
Future Trends in Full Stack Java Development
As the landscape of software development continues to evolve, understanding the future trends in Full Stack Java Development becomes crucial for developers and IT professionals. These trends offer insights into where the industry is heading and equip individuals with the skills needed to stay competitive. With rapid advancements in technology, adapting to new frameworks and tools is not only beneficial but necessary. This section focuses on two significant aspects:
Emerging Technologies and Frameworks
In the realm of Full Stack Java Development, new technologies constantly emerge, impacting both front-end and back-end processes. Key technologies to watch include:
- Microservices Architecture: This approach enables developers to create scalable and efficient applications. Java frameworks like Spring Boot facilitate microservices by simplifying the process of deploying independent services.
- Serverless Applications: Using platforms like AWS Lambda, developers can run code without provisioning or managing servers. This trend reduces overhead and allows easier adjustments to application needs.
- Reactive Programming: Frameworks such as Project Reactor focus on building non-blocking applications for optimizing resources. This caters to modern user demands for additional responsiveness.
- Progressive Web Apps (PWAs): Combining HTML, CSS, and JavaScript, PWAs offer a seamless experience across devices. Their popularity is rising as applications are expected to perform equally well on mobile and desktop.
- GraphQL: Allowing clients to request specific data from APIs, GraphQL streamlines communication between front-end and back-end. Tools like Apollo Client used in conjunction with Java can enhance flexibility in data-fetching.
These emerging technologies help developers stay relevant and adapt the ever-changing market需求.
The Role of Artificial Intelligence
Artificial Intelligence (AI) maintains a profound impact across various industries,and its integration into Full Stack Java Development is no exception. AI brings several advantages:
- Enhanced User Experience: Through personalization, AI can analyze user data to cater to preferences. Integrating machine learning algorithms into web applications can help organizations win over competitive edges.
- Automation of Processes: AI can automate routine tasks during development, saving time and reducing the likelihood of human error. Tools powered by AI can help automatically fix bugs in real-time, improving the overall development workflow.
- Predictive Analysis: Utilizing AI for predictive analytics allows companies to make informed decisions based on user behavior and trends within applications. This can guide businesses to optimize their software features.
- Chatbots Integration: Hosting virtual assistants using AI technologies can significantly improve user interaction. Incorporating libraries like Deep Learning for Java enables developers to integrate customer support systems easily.
It is vital for developers in the Full Stack Java ecosystem to familiarize themselves with these AI integrations. The focus towards AI represents and reinforces the demand for more intuitive and responsive solutions in software experiences.
The future might look challenging for some, yet those embracing these trends can leverage them into remarkable opportunities. By staying informed and adaptable, your value as a developer will consistently grow.
Continuing your journey in Full Stack Java means understanding these trends and their applications. Adopting new technologies and enhancing your skills with emerging frameworks will generate opportunities,or maintain relevance in this endless cycle of advancement.