Mastering Class Declaration in Java: A Complete Guide
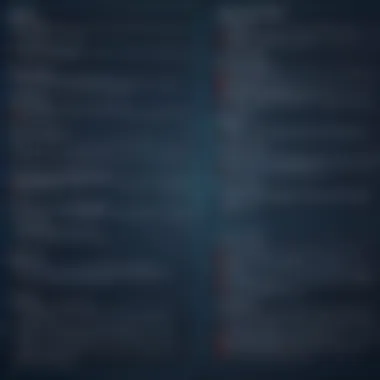
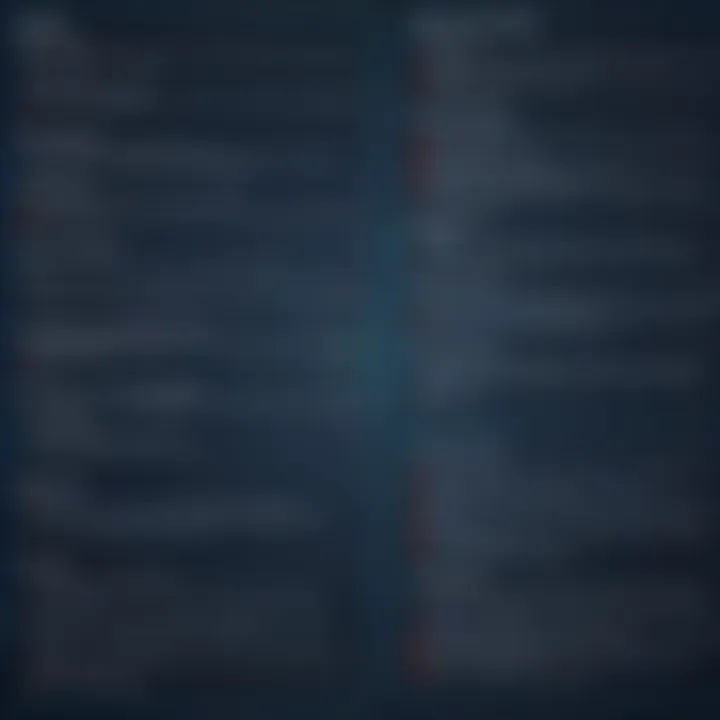
Intro
Class declaration is an essential concept in Java that underpins the realm of object-oriented programming. Understanding it not only enhances coding skills but also elevates a developer’s capability to design software that is efficient and maintainable. The syntax and structure of class declarations lay the groundwork for encapsulation, inheritance, and polymorphism—the core tenets of object-oriented design.
As software development continues to evolve, grasping these principles is vital. This guide will methodically break down the intricacies of class declaration in Java, explaining its syntax, structure, and best practices. We aim to address common challenges encountered in Java programming and provide practical examples.
Overview of Class Declaration
Class declaration in Java comprises several components, including access modifiers, class name, and variable and method definitions. Each component plays a crucial role in defining how a class will interact with the rest of the program.
Definition and Importance of Class Declaration
A class in Java serves as a blueprint for creating objects. It defines the properties (fields) and behaviors (methods) of the objects created from it. Thus, proper class declaration is paramount for effective software architecture. It allows developers to encapsulate data, making systems more modular and easier to manage.
Key Features and Functionalities
The class declaration includes various features:
- Access Modifiers: Keywords like , , and determine the visibility of classes, methods, and variables.
- Static and Non-Static Members: These allow defining properties and methods specific to the class or object instance.
- Inheritance: Classes can inherit properties and methods from other classes, promoting reusability.
Use Cases and Benefits
Class declaration has numerous applications:
- Modular Programming: By structuring code into classes, developers can isolate functionality and improve maintainability.
- Code Reusability: Through inheritance, shared code can be reused, reducing redundancy.
- Encapsulation: Sensitive data can be hidden within objects, thus promoting security.
Best Practices
Implementing effective class declarations requires an understanding of industry best practices. Here are some key points to consider:
- Use Meaningful Names: Class names should be descriptive and follow naming conventions (e.g., CamelCase).
- Single Responsibility Principle: Each class should have only one reason to change, reflecting a focused purpose.
- Encapsulate Data: Use private fields and public methods to control access to data.
Tips for Maximizing Efficiency
- Avoid Unnecessary Complexity: Simple designs are easier to understand and maintain.
- Limit the Size of Classes: Large classes can become unwieldy. Refactor when necessary.
- Document Your Code: Use comments and documentation tools to clarify purpose and usage.
Common Pitfalls to Avoid
- Ignoring Access Modifiers: Not using access modifiers can lead to unintended access to class internals.
- Poor Naming Conventions: Misleading names can cause confusion and mistakes in code usage.
Closure
Understanding class declaration is fundamental for Java developers. It provides the groundwork for creating structured, maintainable, and efficient software. By adhering to best practices and avoiding common pitfalls, developers can design classes that not only facilitate programming tasks but also contribute to the overall quality of software products.
Understanding Java Classes
In the realm of object-oriented programming, comprehending Java classes holds substantial significance. Classes serve as blueprints for creating objects, allowing developers to encapsulate data and behavior within a single structure. This encapsulation not only aids in organizing code but also facilitates easier maintenance and enhances reusability. When programmers define a class in Java, they create a new data type, which can significantly simplify complex problem-solving in software development.
Definition of a Class
A class in Java is a fundamental construct that defines a data structure consisting of fields (attributes) and methods (functions). Fields represent the properties of an object, while methods define the operations that can be performed on those properties. A class can be thought of as a template; it defines what attributes an object will have and what actions it can take. Essentially, it combines data and methods that operate on that data into a single unit.
For example, a class called can have attributes such as , , and , and methods such as and . The instantiation of a class results in an object, which is a concrete instance of that class.
Importance of Classes in Java
Classes are essential in Java programming due to several reasons:
- Modularity: Classes enable developers to break down a program into smaller, manageable sections. Each class can focus on a specific functionality, making it easier to debug and maintain the code.
- Code Reusability: Once a class is defined, it can be reused across multiple programs. This reduces redundancy and the potential for errors.
- Encapsulation: Classes provide encapsulation, which hides internal states and requires all interactions to be performed through well-defined methods, thereby enhancing security and integrity of the data.
- Inheritance: Java classes support inheritance, allowing new classes to inherit attributes and methods from existing classes. This facilitates code extension and reduces code duplication.
Java Class Declaration Syntax
Java class declaration syntax serves as the cornerstone of defining the structure and behavior of objects in Java. Understanding this syntax is crucial for software developers, IT professionals, and tech enthusiasts aiming to grasp object-oriented programming principles. The declaration syntax determines how classes interact with one another and how they encompass data and functionalities. A firm understanding of this topic equips developers with the tools to create scalable and maintainable code.
Basic Syntax Structure
The basic syntax for declaring a class in Java is quite straightforward. A class is defined using the keyword , followed by the class name and a pair of curly braces. Here is an example:
Access Modifiers Explained
Access modifiers in Java play a critical role in controlling the visibility and accessibility of classes, methods, and variables. Understanding these modifiers is essential for encapsulating data.
Public
The access modifier allows a class, method, or variable to be accessible from any other class in any package. This broad visibility makes it beneficial when creating libraries or APIs where classes need to be used across different applications. The key characteristic of is its universal access level, which fosters collaboration among various parts of a program. However, excessive use of can introduce tight coupling, which may complicate future maintenance of a software project.
Private
The modifier restricts the visibility of a class member so that it can only be accessed within the class itself. This encapsulation fosters better data protection, ensuring that sensitive information is not exposed to unintended users. The primary advantage of using is to safeguard class integrity and promote better modularization. However, it can complicate testing and accessing certain features when strict encapsulation is applied too rigorously.
Protected
The access modifier is a middle ground, allowing access to class members within the same package and by subclasses, even if they are in different packages. This provides a reasonable balance between exposure and encapsulation. It is advantageous when designing class hierarchies or frameworks where certain properties or methods should be inherited. Still, it can also increase complexity in terms of understanding how classes interrelate and ensuring proper usage across packages.
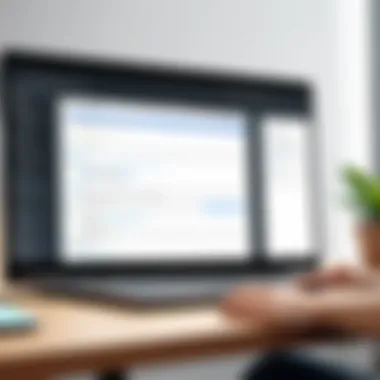
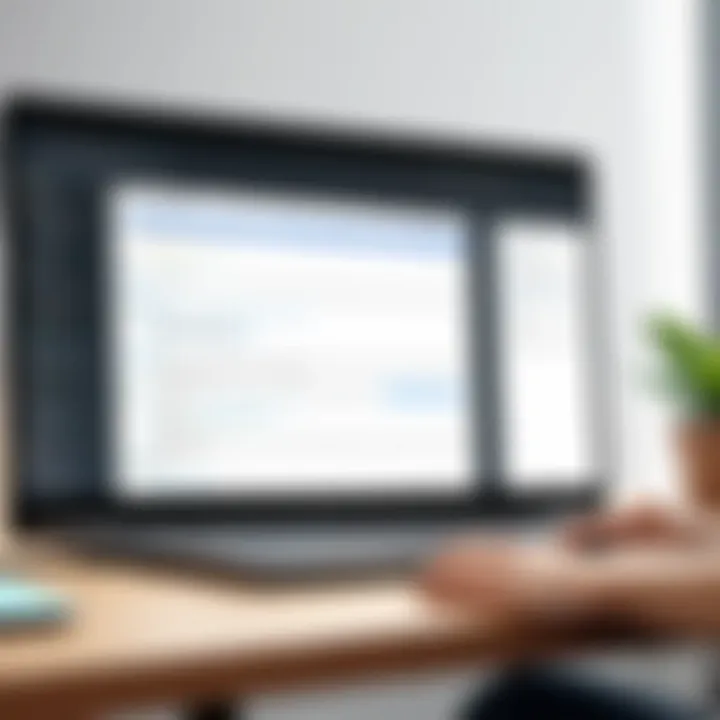
Static vs. Non-Static Classes
In Java, the distinction between static and non-static classes is significant. A static class is universally associated with the class itself rather than any instance of the class. This means that static members can be accessed without creating an instance of the class. Non-static members, however, require an instance to be accessed.
Static classes are beneficial for utility or helper classes, where methods do not depend on instance variables. However, they can lead to memory management issues if not used judiciously, as all static members live for the entire duration of the program. In contrast, non-static classes allow for greater flexibility and encapsulation of instance data but require careful management of object instances to avoid memory leaks.
Understanding the nuances of Java class declaration syntax is essential for building a strong foundation in object-oriented programming. Mastery of this topic leads to the development of cleaner, more efficient, and maintainable code.
Creating a Simple Java Class
Creating a simple Java class is a fundamental aspect of programming in Java, acting as the building block for more advanced concepts. This process not only helps developers understand object-oriented programming but also enhances their coding skills. Simplifying class creation can lead to improved code organization and readability. As developers become proficient, their ability to structure classes efficiently will result in more maintainable and scalable applications.
Step-by-Step Class Creation
To create a class in Java, it is important to follow a structured step-by-step approach. This clarity helps in avoiding common pitfalls. Here’s how to proceed:
- Define Class Name: Choose an appropriate name for your class. It should be meaningful and follow Java naming conventions, such as CamelCase.
- Declare the Class: Use the keyword followed by the class name. This starts the definition of your class. For instance:
- Add Attributes: Define attributes (fields) to hold data. Attributes should be declared with specific data types. For example:
- Create Methods: Methods define the behavior of the class. Declare them as needed. For example:
- Implement Constructors: Constructors help initialize objects. If no constructor is defined, Java provides a default constructor.
This step-by-step process ensures a clean and effective way to declare a class, helping developers grasp core Java principles more easily.
Example: A Basic Class Implementation
To illustrate the process of creating a simple Java class, consider the following example:
In the example above, the class is defined with two private attributes: and . The constructor initializes these attributes, and the method prints their values. This basic implementation showcases how simple class creation can be applied in Java, allowing even novice programmers to create functional and meaningful classes easily.
Class Attributes and Methods
In Java, attributes and methods are critical components of classes. They define the state and behavior of objects. Understanding class attributes and methods helps in crafting effective and functional classes, ultimately leading to better software design. This section dives into how to define attributes, create methods, and explore the concepts of method overloading and overriding.
Defining Class Attributes
Class attributes, often referred to as instance variables or fields, represent the properties of a class. They hold the data relevant to the object created from the class. Properly defining class attributes is essential as they impact object state and behavior.
Attributes should be declared with an appropriate access modifier (like public, private, or protected) to control visibility. Defining attributes too broadly can lead to unintentional side effects. For example, a private attribute ensures encapsulation, protecting data from unintended modifications.
Here's a typical way to define class attributes:
This example defines a class with two attributes: and . The choice of private access ensures only methods within the class can manipulate these fields, safeguarding the integrity of the object's data.
Creating Class Methods
Methods in a class encapsulate behavior. They dictate what actions can be performed on the attributes of a class. A well-designed class should exhibit a focused set of methods that manipulate its attributes appropriately, adhering to the principles of encapsulation.
To create a method, the following elements are usually defined:
- Access modifier: Determines visibility
- Return type: Specifies what the method returns
- Method name: A clear identifier for the method
- Parameters: Inputs to the method
An example of a simple method that modifies an attribute:
This method allows setting the value of the attribute while ensuring that the internal attribute is properly updated. Methods like this enhance class functionality through controlled access to attributes.
Method Overloading and Overriding
These two concepts add powerful versatility to Java methods.
Method Overloading refers to having multiple methods with the same name but different parameter lists in the same class. This provides flexibility, allowing the same operation to be performed with different types or numbers of inputs. An example is shown below:
In this case, the method can handle both integers and doubles, enhancing usability.
Method Overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. This allows subclasses to tailor inherited methods to their specific needs. For example:
By overriding a method, subclasses can modify behavior while preserving the method signature from the superclass. Overriding is essential in achieving polymorphism, a core concept in object-oriented programming.
Understanding how to define class attributes, create methods, and leverage method overloading and overriding can vastly improve your Java programming skills.
Understanding Constructors
Constructors play a pivotal role in Java programming. They are special methods that are called when an object is instantiated from a class. Their primary purpose is to initialize the object's attributes, ensuring that they hold valid values right away. Understanding how constructors work is essential for any developer looking to master Java programming. This knowledge helps in writing clear and maintainable code while preventing common pitfalls associated with uninitialized objects.
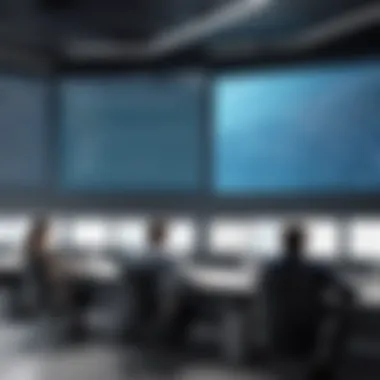
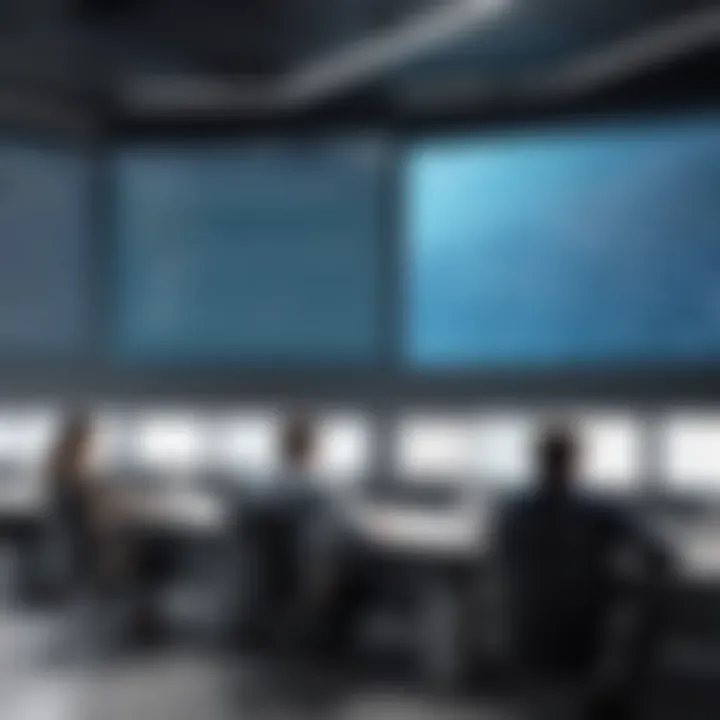
When you create an instance of a class, the constructor is invoked automatically. This process not only allows developers to set initial values but also determines how an object behaves from the start. In Java, there are two main types of constructors: default constructors and parameterized constructors. Knowing the difference between these types is critical for effective object-oriented programming.
Purpose of Constructors
Constructors serve several significant purposes in Java:
- Initialization: They allow you to set initial values for an object's attributes. This ensures the object is in a valid state when it is created.
- Overloading: Java allows method overloading for constructors, which enables you to create constructors with different parameters. This helps in creating objects easily with varying configurations.
- Enforcing Constraints: Constructors can enforce rules about how an object can be created. For example, you can implement checks to ensure that certain criteria are met during object creation.
- Clarity: They improve code readability and make it clear to other developers what values are expected during the object's creation.
A constructor always has the same name as the class it belongs to, and does not have a return type. This uniqueness helps distinguish constructors from other methods in the class.
Default Constructors vs. Parameterized Constructors
In Java, we distinguish between two types of constructors:
- Default Constructors: These are provided by Java automatically if no constructor is explicitly defined. They do not take any parameters and initialize object attributes to default values (e.g., integers to zero, booleans to false).
- Parameterized Constructors: These constructors allow the passing of parameters during object creation. They enable initialization of fields with provided values, making it flexible to create objects that are customized. This is particularly useful for cases where different objects might need different values at instantiation.
Here is a brief code example demonstrating both types:
A constructor must be defined carefully according to the needs of the class, to ensure it performs the intended initialization correctly.
In summary, constructors are foundational to object-oriented programming in Java. Understanding how they work leads to better design and overall code quality.
Inheritance and Class Declaration
Inheritance is a core concept in object-oriented programming, particularly in Java. It allows a class to inherit fields and methods from another class. This leads to a more organized and structured system of classes. The main benefit of using inheritance is the reusability of code. Instead of writing the same code again for every new class, developers can simply extend a base class. Another advantage is the creation of a relationship hierarchy, which often enhances logical organization within codebases.
However, it is also crucial to consider certain factors when using inheritance. Developers must understand how inheritance affects objects, their states, and methods. Improper use of inheritance can lead to difficult-to-maintain code and potential runtime errors. Clarity on the structure of classes is vital to avoid these pitfalls.
Concept of Inheritance
Inheritance establishes a parent-child relationship between classes. The parent class, also known as the super class, contains common characteristics which can be inherited by derived classes, known as subclasses.
This setup leverages polymorphism, allowing subclasses to override or extend behaviors defined in the superclass. When a subclass inherits from a superclass, it acquires its methods, fields, and can also introduce its own attributes and methods. This facilitates a clear approach to extend and modify behavior without altering the existing codebase unnecessarily.
There are two main types of inheritance in Java:
- Single Inheritance: Where a class inherits from one single superclass.
- Multiple Inheritance: Though Java does not support multiple inheritance directly through classes, it does allow a form of it through interfaces.
This distinction is important because multiple inheritance can lead to ambiguity, commonly referred to as the "Diamond Problem." Java avoids this issue by implementing interfaces instead.
Single vs. Multiple Inheritance
In Java, single inheritance means that a class can extend only one other class. This simplifies the design and implementation of classes. Developers can focus on a straightforward hierarchy. For example, if you have a base class called , you might create a subclass called that inherits from it. This keeps the structure simple and clear.
On the other hand, multiple inheritance allows a class to inherit properties and methods from multiple classes. While this is not directly supported in Java classes, interfaces can be used to achieve a similar effect. An interface specifies methods without implementing them, allowing diverse classes to share functionality without the complexities of direct inheritance.
For example:
To maintain clarity and to avoid confusion, developers should be cautious when structuring class hierarchies. By utilizing single inheritance and interfaces where appropriate, one can create flexible, maintainable, and efficient code.
Interfaces and Abstract Classes
Interfaces and Abstract Classes are essential concepts in Java, particularly in the realm of object-oriented programming. They facilitate a well-structured approach to software development, offering a way to achieve abstraction and encapsulation. Understanding these concepts helps software developers create flexible and maintainable code. In this section, we will explore how to define interfaces, the distinctions between abstract classes and interfaces, and the practical applications of each.
Defining Interfaces in Java
An interface in Java is a reference type, similar to a class, that can contain only constants, method signatures, default methods, static methods, and nested types. Interfaces cannot contain instance fields or constructors. They represent a contract that classes can implement, providing a way to achieve polymorphism.
When a class implements an interface, it commits to providing concrete implementations of the abstract methods declared in the interface. This enables different classes to be treated uniformly, enhancing code flexibility. For example, consider an interface named :
Here, any class that implements the interface would need to provide definitions for the and methods. Interfaces are particularly valuable in scenarios involving multiple classes that share common behavior. This encourages the use of composition over inheritance, promoting loose coupling among classes.
Abstract Classes vs. Interfaces
While both abstract classes and interfaces are used to achieve abstraction in Java, they serve different purposes and have different capabilities. An abstract class can have both abstract methods (without implementation) and concrete methods (with implementation). In contrast, an interface only allows method declarations without any method body until Java 8 introduced default methods.
Here are some key distinctions:
- Multiple Inheritance: A class can implement multiple interfaces but can only extend one abstract class. This allows for greater flexibility in design when using interfaces.
- Fields: Abstract classes can have instance variables, but interfaces cannot hold instance states (only constants).
- Constructor: Abstract classes can have constructors, enabling more complex initialization. Interfaces do not have constructors since they cannot be instantiated.
To illustrate, consider an abstract class named :
In this case, can define a common behavior, like , while leaving the specific method to subclasses.
To summarize, It is critical to choose between interfaces and abstract classes based on the requirements of your software project. If you need to define common behaviors without worrying about the state, interfaces are suitable. Conversely, if your design needs to share code and state across implementations, abstract classes are the better choice.
Common Pitfalls in Class Declaration
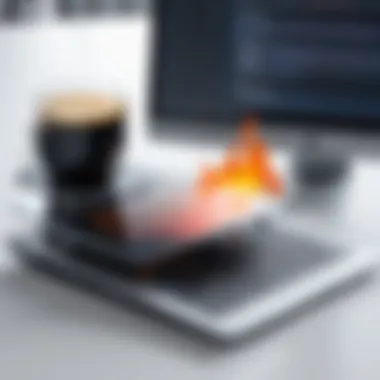
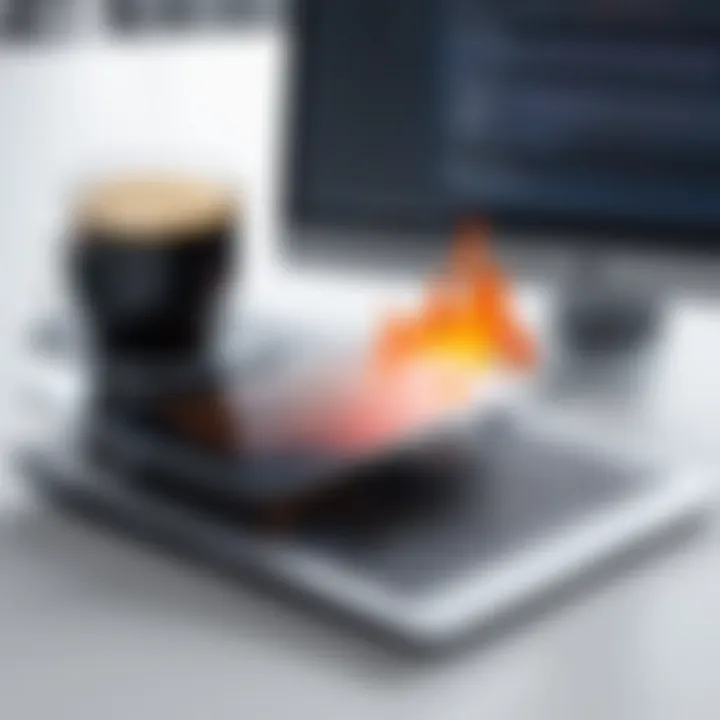
Class declarations are essential yet intricate components in Java programming. Understanding the common pitfalls involved in class declaration can significantly enhance your efficiency and effectiveness in coding. By focusing on these pitfalls, developers can prevent errors that may lead to bugs, performance issues, or misunderstandings in code behavior. Below are three crucial pitfalls to be mindful of.
Misusing Access Modifiers
Access modifiers in Java control the visibility of classes, methods, and variables. Misusing them can lead to unintentional exposure of class details or overly restrictive access that hampers usability. For instance, declaring methods as when they should be might limit their accessibility, leading to difficulties in reuse.
On the other hand, marking everything as can expose the class to unwanted modifications and dependencies. This can lead to maintenance challenges, making it hard to track how and where classes are used. Therefore, it is crucial to carefully consider the appropriate level of access for each class member based on the overall architecture of your application.
Neglecting Constructors and Initialization
Constructors play a vital role in class design, as they set initial states for class instances. Neglecting to define constructors can result in problems with object initialization. Without a proper constructor, class attributes may remain in their default state, which can lead to unexpected behavior when methods are invoked.
Additionally, using default constructors without understanding their implications may leave your class vulnerable. For example, if your class requires specific initial values for attributes to function correctly, omitting this step can cause null pointer exceptions or logic errors during runtime.
Incorrect Method Declaration
Method declaration errors are another frequent pitfall. This includes issues like incorrect return types, mismatched parameters, or misunderstandings about method overloading. For example, declaring a method that is supposed to return an but defaults to can confuse other developers and lead to runtime exceptions.
Moreover, failing to appropriately differentiate method overloads, such as using the same parameter type for different methods without adequate distinguishing features, can complicate code readability and functionality. These mistakes can lead to frustration and inefficiencies during software development.
"Mindful class design not only reduces bugs and errors but also enhances code readability and maintainability."
Best Practices for Class Design
Designing effective classes is crucial for maintainable and scalable software development. A well-thought-out class design can improve readability, enhance functionality, and reduce the occurrence of bugs. This section focuses on two key concepts in class design: encapsulation and keeping classes focused and concise.
Encapsulation and Its Importance
Encapsulation refers to the bundling of data and methods that operate on that data within a single unit or class. This practice is vital as it promotes modularity and hides the internal state of an object from the outside world, allowing for controlled interactions through specified methods. The implications of encapsulation are significant:
- It protects the integrity of an object's data by preventing unauthorized access and modification.
- It simplifies the interface for users of the class, making it easier to understand how to use it without needing to delve into the details of its implementation.
- It facilitates future enhancements and maintenance because any changes to the internal workings of a class do not affect any outside code that relies on it.
In essence, encapsulation enhances code maintainability and fosters a clearer design philosophy.
Keeping Classes Focused and Concise
Maintaining focus and conciseness within a class is also crucial. A well-designed class should have a single responsibility and should not attempt to perform multiple unrelated tasks. This principle is known as the Single Responsibility Principle.
Benefits of focused classes include:
- Improved readability, as understanding a focused class can be more intuitive.
- Easier to test and debug, since the functionality is limited and clearly defined.
- Enhanced reusability, as focused classes can often be used in various contexts without modification.
To achieve concise classes, consider the following strategies:
- Limit the number of methods: Each class should only contain methods that are directly related to its purpose.
- Avoid excessive parameters: Classes that require many parameters may be doing too much. It is better to split responsibilities.
- Use descriptive names: Clear naming conventions for classes and methods will help maintain readability and focus.
Keeping your classes focused can significantly impact the quality of your code and the efficiency of your development process.
Testing and Debugging Classes
Testing and debugging are critical components in the development process of Java classes. These processes ensure that the classes function as intended and that issues can be identified and resolved efficiently. In the realm of software development, particularly with Java, robust testing and debugging practices can significantly enhance the quality and reliability of applications. This section delves into these essential practices, emphasizing their importance and outlining practical strategies that developers can adopt.
Unit Testing Java Classes
Unit testing is a software testing technique where individual components of a program are tested in isolation. In Java, unit tests are primarily written using frameworks such as JUnit or TestNG. The benefits of unit testing include:
- Early Problem Detection: By testing classes right after their creation, developers can quickly identify issues.
- Documentation: Unit tests serve as documentation for how a class is supposed to behave. This is particularly useful for new developers who may not be familiar with existing functionality.
- Refactoring Safety: With comprehensive unit tests, developers can refactor code with confidence, knowing that they have tests in place to catch regressions.
To create effective unit tests, it is essential to follow best practices:
- Isolate Dependencies: Use mocking frameworks like Mockito to isolate the class being tested from its dependencies.
- Write Meaningful Tests: Each test should assert a specific behavior of the class, ensuring clarity in what is being tested.
- Maintain Tests: Keep your tests up to date as the code evolves. This ensures ongoing confidence in the class's functionality.
A sample unit test in Java using JUnit might look like this:
This simple test checks the functionality of an method. When executed, it confirms whether the method produces the expected result.
Common Debugging Strategies
Debugging is the process of identifying and resolving bugs within the code. Effective debugging can greatly reduce the time spent on fixing issues. Here are some common strategies:
- Log Statements: Use logging to track the flow of data and the sequence of operations. Libraries like SLF4J or Log4J can be beneficial.
- Debugging Tools: Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse provide robust debugging tools. Developers can set breakpoints, step through code, and inspect variables to understand the program's behavior.
- Code Reviews: Engaging peers in code reviews can help spot issues before the code is run. Fresh eyes often see errors or design flaws that the original developer might miss.
- Test-Driven Development (TDD): In TDD, tests are written before the code. This ensures that classes are designed with testing in mind, potentially avoiding many bugs from the start.
"Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are by definition not smart enough to debug it." – Brian Kernighan
Employing these strategies can streamline the debugging process, making it less daunting and more systematic for Java developers.
Culmination
In this article, we have explored many facets of class declaration in Java. The conclusion is essential as it encapsulates the key elements discussed and offers insights into their significance. Understanding how to effectively declare classes enhances a developer's ability to write clear and maintainable code. A well-defined class structure can lead to improved code organization and easier debugging, which is crucial in complex systems.
Recap of Key Points
- Class Basics: A class is a blueprint for creating objects. Grasping this basic principle forms the foundation for object-oriented programming in Java.
- Syntax and Structure: Clear knowledge of class declaration syntax helps prevent semantic errors during coding.
- Attributes and Methods: Defining proper attributes and methods facilitates better encapsulation, ensuring that data is managed correctly within the class.
- Constructors: Constructors are integral for initializing new objects, and recognizing the differences between default and parameterized constructors is vital.
- Inheritance: Understanding inheritance allows for code reuse, essential for maintaining clean codebases.
- Interfaces and Abstract Classes: These elements provide flexibility in design and encourage adherence to programming principles like polymorphism.
- Best Practices: Following established best practices strengthens code quality and helps maintainability over time.
- Testing and Debugging: Emphasizing the importance of testing ensures code quality before deployment. Debugging strategies also ensure smooth operation of the final product.
Future Directions in Java Class Design
As technology advances, Java continues to evolve. Developers should remain adaptive to new features and trends in class design. One significant trend is the emphasis on functional programming within Java, with the introduction of constructs like lambdas and streams. These features encourage cleaner code and innovative design patterns.
Another direction is the growing need for microservices architecture. Class design will increasingly focus on lightweight, independent classes that can act as stand-alone microservices. This aligns with the principle of keeping classes focused and flexible.
The integration of APIs and frameworks may lead to a shift towards more declarative programming styles. This approach allows developers to define behaviors without specifying the control flow, increasing productivity.
Overall, a solid grasp of class declaration principles will position developers well for future advancements and changes in the Java ecosystem.