Caching in Java: Concepts, Strategies, Implementations
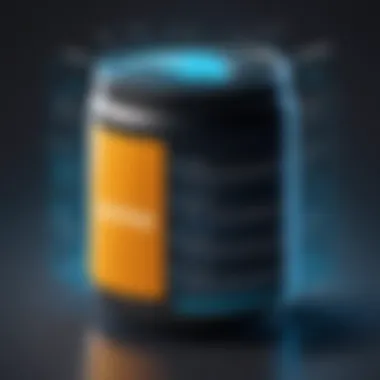
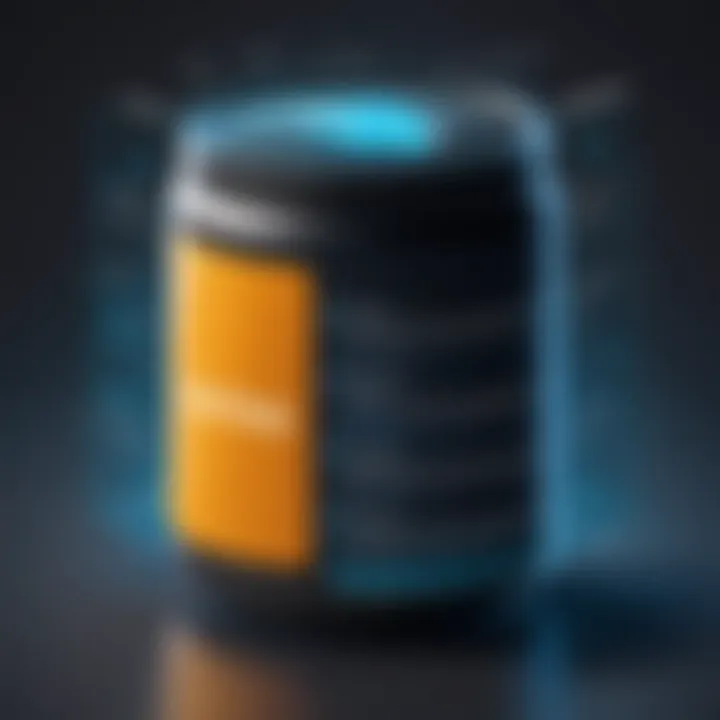
Intro
In the fast-paced world of software development, every second counts. As applications expand and user expectations rise, performance bottlenecks can quickly become the Achilles' heel of your project. Enter caching—a technique that can dramatically speed up data retrieval and enhance performance. This article dives into the nitty-gritty of caching specifically within the Java ecosystem, illuminating its pivotal role in optimizing application performance. With various caching strategies and a plethora of tools at your disposal, understanding how to implement these can be the key to unlocking your application's full potential.
Overview of Caching in Java
Caching, in essence, is the storage of frequently accessed data in a temporary location for quicker retrieval. Think of it as having your favorite snacks within arm's reach rather than in the farthest pantry. In Java, caching isn’t just a convenience; it’s often indispensable. Java applications, ranging from small web apps to enterprise-level software, can significantly benefit from caching.
Definition and Importance of Caching
Caching refers to the technique of storing data in temporary storage locations called caches, which are much faster than traditional data storage systems. When performance is crucial, caching promotes efficiency by minimizing data access times. For example, when a user requests a web page, any static resources can be fetched from the cache instead of hitting the database every time. This saves both time and resources, allowing your application to perform better and serve more users simultaneously.
Key Features and Functionalities
Some prominent features of caching include:
- Quick Access: Retrieving data from the cache is usually orders of magnitude faster than fetching it from a database.
- Reduced Load: By caching frequently requested data, the number of hits to the underlying data source decreases, alleviating strain on your databases and servers.
- Configurable Expiry: Items stored in a cache can be set to expire after a certain period, ensuring that stale data doesn’t linger.
Use Cases and Benefits
The advantages of caching stretch across various domains:
- Web Development: Speeding up page loads drastically improves user experience.
- APIs: Enhance the performance of RESTful services by caching responses.
- Machine Learning: Reduce repetitive data pre-processing by caching intermediate results.
Best Practices
While caching brings profound benefits, its implementation comes with caveats. Here are staple practices to ensure effective utilization:
- Know Your Data: Not everything needs to be cached. Focus on data that is accessed frequently but changes infrequently.
- Monitor Cache Performance: Use metrics to assess cache hit and miss ratios. It’s critical to understand if the caching strategy is effective.
- Choose the Right Strategy: Employing the appropriate caching strategy—be it in-memory caching with tools like Ehcache, or distributed caching with Hazelcast—can often make or break your application’s performance.
Common Pitfalls to Avoid
- Over-Caching: Caching too much data can negate the benefits. Aim for a lean cache that strikes a balance between speed and resource usage.
- Ignoring Cache Invalidation: A cache holding stale data can lead to frustrating application behavior. Timely invalidation is necessary to keep the data fresh.
Case Studies
Several enterprises have reaped the rewards of effective caching mechanisms.
- Netflix: Leveraging caching to handle millions of concurrent streams, Netflix has ensured that users experience seamless, buffered streaming.
- Twitter: By caching results for users' timelines, Twitter handles requests at lightning speed, ensuring users remain engaged without delays.
"Caching is like a sports strategy; you have to adjust your game plan based on real-time performance." — Industry Expert
Latest Trends and Updates
The world of caching is evolving. Here are some current trends:
- Edge Caching: With the rise of content delivery networks, edge caching is becoming mainstream. Data is cached closer to the user, enhancing speed even further.
- AI-Powered Caching: Artificial intelligence is being utilized to predict data that is likely to be accessed, optimizing cache utilization.
How-To Guides and Tutorials
For those ready to roll up their sleeves, a step-by-step guide to implementing a simple caching mechanism in a Java application is essential. Here's a quick walkthrough:
- Choose a Caching Library: Select a reliable caching framework like Guava or Caffeine.
- Set Up the Cache: Initialize the cache instance in your application.
- Add Data to Cache: Store data in the cache.
- Retrieve Data from Cache: Fetch data, checking first if it exists in the cache to avoid unnecessary database calls.
This appears simple, but the underlying principles hold the key to better performance.
By effectively applying these concepts, strategies, and practices, you can steer your Java applications toward greater efficiency and performance. Caching is not just a feature; it’s a vital component that can elevate your code from ordinary to extraordinary.
Understanding Caching
Caching is a fundamental concept in computer science, particularly in the realm of software programming, and it holds a special place in Java development. It serves as a mechanism that significantly improves application performance and responsiveness by temporarily storing copies of frequently accessed data. This section will delve into why caching matters in Java, enumerating its vital roles and the considerations involved in implementing effective caching solutions within your applications.
Definition and Importance
At its core, caching refers to the practice of storing copies of files or data in a designated area – the cache – to allow for quicker access on subsequent requests. This becomes crucial when considering today's fast-paced digital environments, where speed dictates user experience. To put it simply, imagine trying to retrieve a paper from the archives; doing so takes time. But having a copy at your desk speeds things up dramatically. Similarly, in Java applications, caching enables the retrieval of data without hitting the primary data source, which is often slower and more resource-intensive.
The significance of caching in Java can't be overstated. Here are some key reasons why it is essential:
- Performance Boost: By storing frequently accessed data, applications can reduce latency and ensure faster response times for users.
- Resource Efficiency: Caching can lower demand on databases and network requests, leading to a more efficient utilization of resources.
- Scalability: With immense data access needs, caching facilitates applications to scale up seamlessly without compromising performance.
- User Experience: A faster application translates to happier users, which is invaluable for retaining a loyal user base.
These aspects unveil just how vital caching is to modern Java applications, illustrating that it is not merely an optimization tactic, but a necessity for any serious developer aiming for efficiency and responsiveness.
How Caching Works
Caching operates on a simple principle: it stores the results of operations or data retrievals so that repeat requests can be served quickly. The mechanics hinge on two primary elements: the cache store and the cache retrieval process.
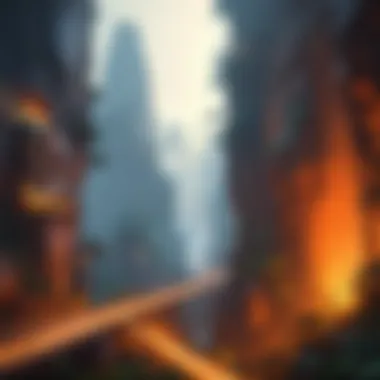
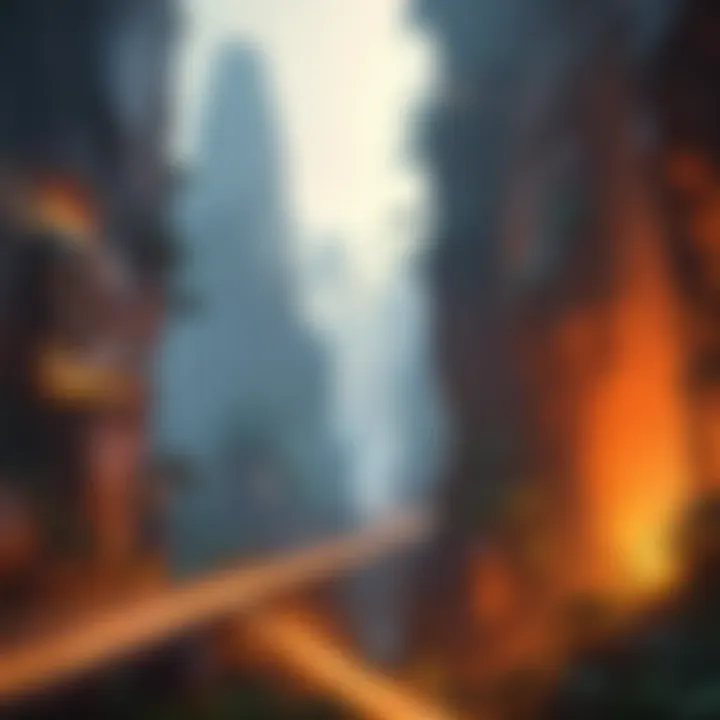
- Cache Store: This is where cached data resides. It can be in-memory (like Java's ) or external systems (like Redis). Depending on your needs, you might prefer one over the other.
- Cache Retrieval Process: When a request is made, the application checks if the needed data is already in the cache. If it is, it will retrieve from there, bypassing the slower data sources. If not, it will fetch the data, store it in the cache for future access, and then return the requested data.
This leads to a common strategy dubbed the cache hit and miss ratio. A cache hit occurs when data is found in the cache, while a miss means the data needs to be retrieved from the primary data source. The aim is to maximize hits because higher ratios contribute to improved performance and efficiency.
"Implementing caching is like setting up shortcuts to the information you need most – it makes the journey faster."
Types of Caching in Java
Caching serves a vital role in application performance, especially in Java where efficient memory management and optimization can lead to significant gains. By understanding the different types of caching available—namely In-Memory and Distributed Caching—developers can make informed decisions that enhance their applications' responsiveness and effectiveness.
In-Memory Caching
Overview of In-Memory Caching
In-memory caching is essentially keeping frequently accessed data in the system's memory, which provides lightning-fast access when needed. One key characteristic is its simplicity; it’s easy to implement and often greatly improves performance since retrieving data from memory is orders of magnitude quicker than accessing disk or database storage.
In-memory caching is particularly popular because it can vastly reduce the load on databases, therefore minimizing query times. A unique feature of this approach is that it's volatile—meaning it doesn’t persist data through crashes. This can be both a pro and a con. While developers benefit from the speed, they also have to manage security contracts and potentially handle data loss during failures. The balance is to manage the critical data that needs speed versus the staleness of any temporary data - a vital consideration in the caching strategy.
Use Cases and Examples
When it comes to use cases, in-memory caching shines in scenarios where real-time data access is paramount. Applications like social networks or financial tech platforms typically require the low-latency data retrieval that in-memory caching provides. A distinctive feature is its ability to cache session data, which offers quick retrieval for user activity within an application.
However, relying solely on in-memory caching can lead to potential pitfalls. For instance, if the system fails and all in-memory data is lost, the application's functioning can stumble. Therefore, it’s essential to carefully consider how critical the data is before deciding what to cache in memory. A constructive approach involves caching data that is read frequently but doesn’t change often, ensuring you get the speed advantage while minimizing possible downsides.
Distributed Caching
Characteristics of Distributed Caching
Distributed caching refers to your application’s ability to store cached data across multiple servers or nodes. This is advantageous because it effectively enables scalability—allowing the cache to grow as your application scales and handles increasing loads. A notable characteristic of distributed caching is its resilience; if one node goes down, others can still serve up cached data, which is crucial for high-availability systems.
This system's unique feature lies in its distributed architecture, where data is replicated and partitioned, enhancing both data availability and reliability. However, on the flip side, there are complexities involved. For example, network latency can impact performance, and keeping the cache consistent across different nodes is always a challenge. Understanding how to balance these issues is key.
Frameworks Supporting Distributed Caching
There are several frameworks that can facilitate distributed caching, each with its unique strengths—Hazelcast, Apache Ignite, and Redis come to mind. A special feature of these frameworks is their diverse configurations, enabling developers to choose the best option for their specific use case. They often come with tools for monitoring and managing the cache, providing robust solutions that cater to both beginner and experienced users.
Choosing the right framework can considerably enhance operational efficiency. However, developers should also be aware of the implications of deploying these solutions within their architectures. Factors like data consistency requirements and network design will influence which framework would serve their caching needs best. This decision can have long-lasting impacts on application performance.
Key Caching Strategies
In the realm of Java programming, adopting effective caching strategies is crucial for optimizing application performance. Caching isn’t just about storing data; it’s about ensuring quick access to frequently used information, thereby reducing database load and improving responsiveness. In this section, we will explore various caching strategies that developers can employ. Each strategy comes with its own unique characteristics, benefits, and considerations that can significantly impact application efficiency.
Cache Aside Pattern
Mechanism and Flow
The Cache Aside Pattern, also known as Lazy Loading, operates on a simple yet effective principle: the application code is in charge of fetching data from a data source only when needed. When the data is requested, the application checks the cache first. If the data is there, it gets returned immediately. If the data isn’t found, the application retrieves it from the data source (like a database), then stores a copy in the cache for future use. This approach helps in minimizing the load on databases while ensuring that frequently requested data is quickly accessible.
One of the standout features of this pattern is its on-demand nature, which avoids unnecessary data retrieval, making it a cost-effective choice. However, it does require explicit logic in the application to manage cache access.
Benefits and Limitations
The primary advantage of the Cache Aside Pattern is its simplicity and control. Developers have full discretion over what data gets cached and when to refresh it. This pattern is particularly beneficial for read-heavy applications where certain data is accessed frequently. However, this strategy has its pitfalls, too. The main drawback is that it can lead to stale data if not managed correctly, especially if the data in the source changes but the cache isn’t updated promptly. This potential for inconsistency can be a real headache in applications demanding real-time accuracy.
Read-Through and Write-Through Caching
Definitions and Process Flow
In Read-Through Caching, the application uses the cache as the main interface for retrieving data. If the requested data isn’t found in the cache, the system automatically retrieves it from the data source and populates the cache simultaneously. This approach simplifies the code since the application does not need to manage the caching layers explicitly. The Write-Through strategy complements this by ensuring that any data written to the data source is also written to the cache. This guarantees that the cache always has the most current view of the data, making it beneficial for applications where data integrity is crucial.
Application Scenarios
Read-Through and Write-Through caching shines in scenarios where data consistency is paramount, such as online retail platforms or financial applications. By maintaining a high level of data integrity, these strategies are popular in environments where users expect accurate and timely data retrieval. However, the reliance on synchronous operations in the write-through method can lead to performance bottlenecks, which needs careful consideration in high-throughput applications.
Write-Behind Caching
Workflow
Write-Behind Caching takes a different approach altogether. Instead of writing directly to the data source, data is first written to the cache. The caching system then asynchronously writes the data to the database in the background. This method provides improved performance since applications aren’t held up by slow database writes. The core of the workflow is non-blocking, allowing applications to continue functioning without delay.
However, it requires a robust system to manage asynchronous tasks and ensure that data is eventually written to the database, making it a complex solution compared to its counterparts.
Pros and Cons
The primary benefit of Write-Behind Caching is the performance boost it offers. By decoupling the write operations from the application's primary workflow, applications can handle a higher number of requests more efficiently. Still, this strategy comes with its challenges. For instance, if an application fails before the data is written to the database, there’s a risk of data loss. Furthermore, developers must account for eventual consistency, which may complicate data management.
By understanding these caching strategies, developers can make informed decisions based on their specific application needs. The right caching approach can make all the difference in creating responsive and efficient software.
Implementing Caching in Java Applications
Caching in Java applications plays a pivotal role in enhancing performance and reducing latency. This section highlights the significance of effectively implementing caching techniques to optimize application efficiency. With the myriad of data-driven applications evolving these days, proper caching not only aids in speed but also ensures that resources are utilized efficiently.
Given the diverse nature of Java applications, the implementation of caching techniques can greatly influence user experiences. It allows for quicker data retrieval and minimizes the constant query load on the backend databases. When developers grasp the importance of caching, they become better equipped to make informed decisions, balancing processing speeds and memory usage.
Additionally, understanding caching mechanisms allows developers to foresee potential pitfalls, such as stale data issues. This foresight is crucial in ensuring applications remain responsive and maintain data integrity, fulfilling user expectations.

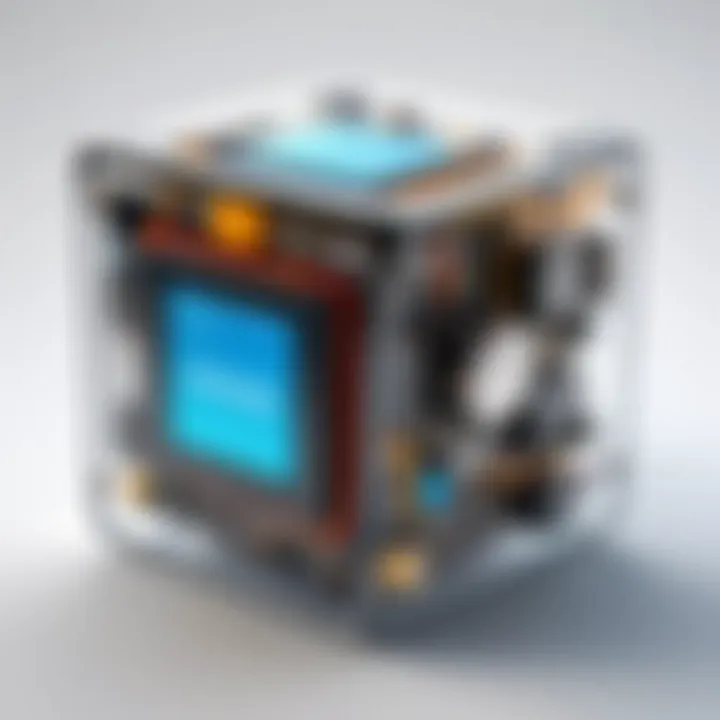
Using Java Cache API
Overview of the API
The Java Cache API offers a standardized approach to caching in Java applications. One of its standout features is its adaptability – it provides a unified interface for caching at various levels of the application. Java developers consistently choose this API due to its straightforward integration with existing Java codebases.
A notable quality of the Java Cache API is its support for various cache types, including in-memory and distributed caches. This flexibility enables developers to tailor caching solutions that fit specific application needs. Consequently, implementing the Java Cache API can lead to enhanced cache performance through various customizable configurations.
However, it's essential to be aware of what the API brings along. The API may come with a learning curve, especially for those unfamiliar with caching principles. Yet, the initial effort often pays off in spades with improved application responsiveness.
Sample Implementation
Bringing the Java Cache API to action can be straightforward. Here’s a simple implementation:
This code demonstrates how to create a cache and store a simple key-value pair. The key characteristic here is simplicity. With minimal overhead, developers can implement efficient caching mechanisms directly into their applications without significant complexity.
In practice, using the sample implementation can lead to tangible improvements in applications by cutting down the latency in data retrieval. This is particularly beneficial when handling substantial datasets, where rapid access becomes vital.
Popular Caching Libraries
Ehcache: Features and Usage
Ehcache stands tall as one of the popular caching libraries available. Its key characteristics include a robust caching mechanism that is not only versatile but also easy to deploy. Known for its ability to handle both in-memory and disk-based caches, Ehcache strikes a good balance between speed and persistence.
It’s favored by many because it seamlessly integrates with existing frameworks like Spring, offering developers a reliable option for caching. This library can be configured easily through XML or Java code, making it accessible for diverse application types.
While Ehcache is advantageous due to its flexibility, there are scenarios where care must be taken. Larger configurations, while powerful, can sometimes be cumbersome to manage efficiently, leading to potential performance overheads.
Guava Caching: Advantages and Implementation
Guava Caching is another well-regarded library that provides a fast and simple caching solution for Java developers. Its main appeal lies in its high performance and ease of use. Guava offers features such as automatic eviction policies and size-limited caches, which optimize memory use effectively.
Using Guava is advantageous for those looking to implement a no-frills caching solution without extensive configuration. Its unique ability to create different cache strategies directly through its API means developers can focus on core functionalities rather than complexity in setup.
However, it’s worth noting that Guava may not be suitable for extremely large datasets. For such cases, its in-memory nature could lead to possible limitations in scalability. Considering these trade-offs helps in making informed decisions when choosing the right caching library for your application.
Caching can be a double-edged sword in software design. Properly employed, it enhances systems significantly. Misused, it can lead to stale data and performance bottlenecks.
Performance Considerations
Performance considerations are crucial when it comes to caching in Java. They help developers understand how effectively their caching mechanisms are functioning. In a world where speed and efficiency can make or break an application, evaluating performance can lead to substantial gains. Key aspects of performance considerations include measuring cache performance, understanding trade-offs, and managing both resource allocation and data accuracy.
Understanding how well your cache performs can often indicate the overall health of your application. A cache that doesn’t perform optimally could introduce latency and degrade user experience, regardless of how efficient the algorithm or application is. Thus, this section will explore not only the metrics to track, but also the trade-offs involved in caching.
Measuring Cache Performance
Key Metrics to Track
When tracking cache performance, certain metrics stand out: hit rate, miss rate, and latency. The hit rate, which measures how often requested data is found in the cache, is often an essential figure. A high hit rate indicates that the cache is effectively serving data without needing to retrieve it from the slower backing store, like a database. This characteristic shows the caching layer's efficiency and is crucial for understanding application responsiveness.
On the other hand, the miss rate, the opposite of the hit rate, should be minimized, as frequent cache misses can lead to performance lags. The latency involved in both cache hits and misses provides insight into how long it takes to access the data. While latencies usually vary depending on how data is stored and fetched, low latency during cache hits typically translates to a better application performance.
Each metric has its unique implications in different application contexts. Tracking these metrics can directly contribute to identifying bottlenecks in your system and making informed decisions around caching strategies. Unfortunately, caching isn't a set-it-and-forget-it affair; it requires continuous monitoring and fine-tuning to truly shine.
Tools for Performance Monitoring
Performance monitoring tools like JMX (Java Management Extensions) or commercial software such as New Relic and AppDynamics provide valuable insights into cache behavior. These tools allow developers to gather crucial data such as hit/miss rates and average response times in real-time.
One key benefit of using performance monitoring solutions is their ability to help visualize data through dashboards. Not only can developers see metrics, but they can also analyze trends over time. This capability is particularly useful for identifying performance degradation and the conditions under which it occurs. However, some tools come with complexity, requiring a certain level of expertise to set up effectively, which could be a disadvantage for less experienced developers.
Trade-Offs in Caching
Memory Usage vs Speed
When you employ caching, a critical question arises: How much memory should you allocate for caching purposes? Allocating too much can lead to wasted resources and impact overall system performance. Conversely, reducing cache size often leads to increased latency due to higher miss rates. Striking that balance is vital. High memory usage doesn't always equate to better cache performance. The choice comes down to application needs, where some may necessitate high-speed data access while others focus on resource conservation.
In practice, developers might have to target specific functionality of applications to find that sweet spot—whether it be a responsive UI or an efficient background task. This consideration therefore implicates both the immediate performance of the cache and the overall resource utilization across the system, posing a delicate balancing act.
Data Freshness Challenges
Another pressing matter that surfaces with caching is maintaining data freshness. Cached data can quickly become stale, particularly in systems where data updates occur frequently. This characteristic can pose significant issues. Imagine a scenario where an application displays outdated information to users, causing confusion and mistrust. To tackle this, developers often implement strategies such as time expiration or event-based invalidation, ensuring that cached data remains relevant.
Yet, maintaining this balance creates its own set of challenges. If the data is invalidated too frequently, the cache might not deliver its intended speed benefits, while too lenient expiration can amplify the risks of serving stale information. Thus, determining the optimal freshness strategy becomes a critical consideration in performance.
"A performant cache is not just about speed, it’s about understanding the nuanced trade-offs that come with it."
Best Practices for Caching
Cache implementation isn’t merely a technical endeavor; it's an art that balances performance, resource management, and user experience. Proper caching can lead to substantial improvements in application responsiveness by storing the right data in the right manner. The effectiveness of caching systems can be heavily influenced by adhering to best practices tailored to the specific needs of your Java applications. Here, we’ll explore critical elements like what data to cache, approaches to manage cache size, and the eviction policies that help maintain optimal performance.
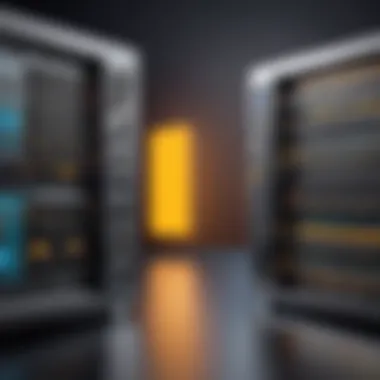
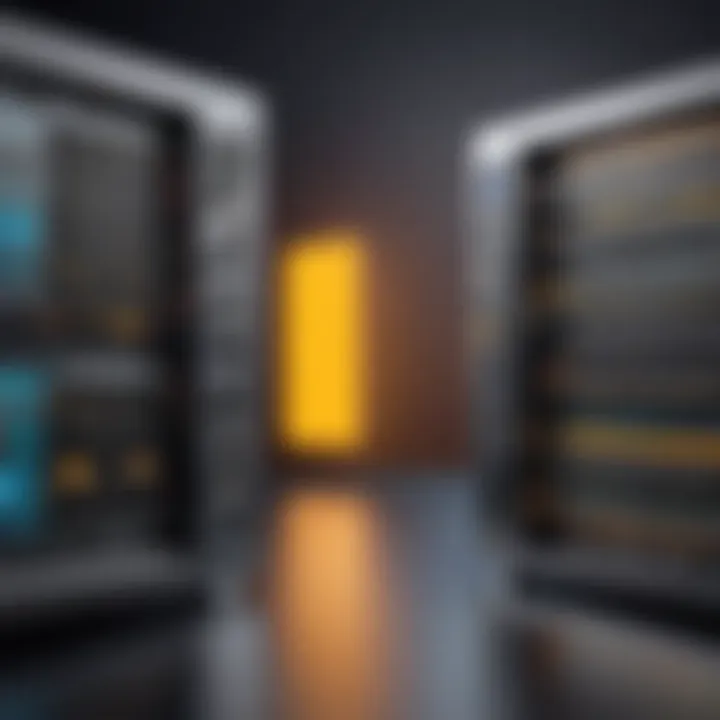
Deciding What to Cache
Identifying the right data to cache is essential. Not all data provides the same value when cached. This decision depends on several factors including:
- Frequency of Access: Data that users access frequently is prime for caching. For instance, think about user profiles on social media platforms; these are accessed often and can greatly benefit from being cached instead of querying the database repeatedly.
- Data Size: Larger data sets may strain memory resources. Instead of caching large assets, consider caching essential, smaller pieces of data that are frequently requested.
- Stability of Data: If certain pieces of data don’t change often, they make ideal candidates for caching. Stale data can lead to inconsistencies, so cache items that remain stable longer.
Taking these factors into account can lead to smarter caching strategies that promote speed and efficiency in your applications.
Cache Size Management
The amount of data stored in cache can directly affect performance. An overloaded cache can degrade application performance, so monitoring and managing its size is crucial. Here are practical tips for managing cache size:
- Set Limits: Establish maximum size limits for your cache. This keeps memory usage in check and allows for better response times.
- Analyze Usage Patterns: Keeping an eye on what gets cached and how often it’s used can inform adjustments in size.
- Periodic Review: Regularly assess your caching strategy. Just like a closet, a cache can become cluttered with old data that isn't being utilized.
Monitoring these aspects not only prevents overconsumption of memory but also ensures the cache remains effective and relevant to users' needs.
Eviction Policies
Eviction policies dictate what happens when the cache reaches its limit. How you manage this can make or break your caching strategy. Two prevalent methods are Least Recently Used (LRU) and First In, First Out (FIFO).
Least Recently Used (LRU)
This policy removes the least recently accessed items first. The primary characteristic of LRU lies in its ability to keep frequently accessed data available while discarding what's not in use. Advantages include maintaining data relevance, as older, less-used data gets cleared out first. The downside? Implementing LRU can be computationally expensive, especially in the case of very large data sets.
When employed correctly, LRU contributes significantly to optimizing cache performance, ensuring the most pertinent data is readily accessible.
First In, First Out (FIFO)
FIFO operates on a straightforward principle: the first data to enter the cache is the first to be removed. This policy is easy to implement and understand. However, its significant drawback is that it doesn’t consider how often or how recently the data has been accessed. As a result, vital data may be discarded simply because it was cached earlier, regardless of its relevance or importance.
In summary, while FIFO can suit particular use cases with predictable data usage patterns, it can also lead to inefficient use of cache resources when the data access pattern is less predictable.
Implementing effective caching strategies in Java is crucial for boosting your application's performance. By following best practices, you're not just improving speed; you're enhancing user satisfaction.
Adopting effective caching practices can significantly improve the performance of Java applications, helping developers to form a thoughtful balance between speed and data accuracy.
Challenges and Pitfalls
Caching is a double-edged sword in Java applications. While it can significantly enhance performance and speed, there are specific challenges that can derail its effectiveness. Understanding these challenges is paramount for any software developer or IT professional working on caching strategies.
One of the most critical elements of caching is knowing how to address potential pitfalls. Practical experience shows that overlooking these can lead to serious inefficiencies, or sometimes more trouble than what you'd bargained for. Hence, let’s dig deeper into two of the most prominent issues that cache implementations face: cache invalidation and stale data risks.
Cache Invalidation Issues
Cache invalidation is akin to keeping a well-stocked pantry; if you don’t know when to throw something out, you might end up with food that’s gone bad without realizing it. In caching, this means your application might continue to serve outdated data even after it has changed in the source system. This situation can be particularly troublesome for applications that deal with dynamic data.
Importance of Cache Invalidation: The challenge lies in effectively invalidating the cached data when the source data updates. Not doing so can lead to scenarios where users interact with stale or incorrect information. For example, in a typical web application, imagine a user checking stock availability in a store. If the cache doesn’t toss out its outdated info, the user might see that an item is in stock when, in reality, it’s sold out, leading to disappointment.
The crux of solving cache invalidation problems involves establishing a reliable invalidation strategy. Here are some common approaches:
- Time-Based Invalidation: Setting a time-to-live (TTL) for cached entries.
- Event-Based Invalidation: Listening for events that signal changes in the data.
- Manual Invalidation: Developers can clear or update the cache as needed, but this requires discipline.
It’s vital for developers to map out a clear strategy for cache invalidation in their applications. Otherwise, the cache can start resembling a ticking time bomb, with outdated data causing user frustration or worse, business ramifications.
Stale Data Risks
Stale data risk goes hand in hand with cache invalidation issues. It’s like driving a car with a cracked windshield—you can see just enough to get by, but the blind spots might throw you off at a critical moment. Having stale data in the application can cloud decision-making, producing results that lack accuracy.
When users encounter stale data, it erodes trust. For instance, consider an online banking application where transaction data is cached. If a user checks their balance and sees an outdated amount, they might make decisions based on incorrect financial information.
To minimize stale data risks, developers should consider the following:
- Write-Through Cache: Here, the cache updates alongside the primary data source, ensuring that the cached data is always in sync.
- Read-Through Cache: This strategy automatically populates cache entries when data is requested, so users always access up-to-date content.
- Regular Cache Refreshing: Implementing processes that periodically check for updated data entries can help reduce the likelihood of serving stale content.
Key takeaway: Stale data management is as important as the caching process itself. Software architects must proactively design their systems to mitigate this risk.
Future Trends in Caching
As technology evolves at breakneck speed, the landscape of caching in Java is no different. This section sheds light on future trends in caching that promise not only to enhance performance but also to address new challenges arising from technological advancements. Understanding these trends is vital as it leads to improved efficiency and effectiveness in application development, particularly in an era dominated by big data and cloud computing.
Caching is not just about speed; it is about adapting to new challenges and opportunities.
Emerging Technologies
In the realm of caching, emerging technologies such as artificial intelligence (AI) and machine learning (ML) are making waves. These technologies are being harnessed to optimize cache management, adapting dynamically based on usage patterns. For instance, an AI-powered caching mechanism could analyze hit rates and data access frequency to intelligently manage cache size, ensuring hot data is swiftly accessible while cold data is judiciously pruned.
Moreover, the rise of containerization, particularly with tools like Docker and Kubernetes, is reshaping how caching is implemented. These technologies facilitate microservices architectures where each service could have its own dedicated caching layer. This not only enhances scalability but also allows for tailored caching strategies, utilizing approaches like polyglot persistence without the overhead of managing centralized caches.
Blockchain technology is another player here. By using decentralized caches, applications can leverage immutability and transparency in caching operations. This can be particularly beneficial for applications that require high levels of data integrity and trust.
Integration with Cloud Services
With the growing adoption of cloud computing, integrating caching mechanisms with cloud services has become crucial. Cloud-based caching solutions, such as Amazon ElastiCache and Google Cloud Memorystore, provide scalable and managed caching capabilities. These services allow developers to offload the complexity of cache management while ensuring performance enhancements in data retrieval.
Furthermore, organizations can benefit from cost efficiency—only paying for the resources they use. This elasticity is particularly vital in fluctuating workloads, as cloud providers can automatically scale resources up or down based on demand.
The integration doesn’t stop at merely using cloud services. Understanding how to effectively leverage cloud-native caching tools allows developers to optimize performance through built-in features like automatic failover and data partitioning. This strategic approach can help maintain smooth operation during peak loads or outages, thus minimizing disruptions.
As caching continues to evolve with these technologies, developers must also stay informed about the trade-offs that come with them, balancing between immediate performance benefits and long-term sustainability.