Building Web Applications in Java: A Complete Guide
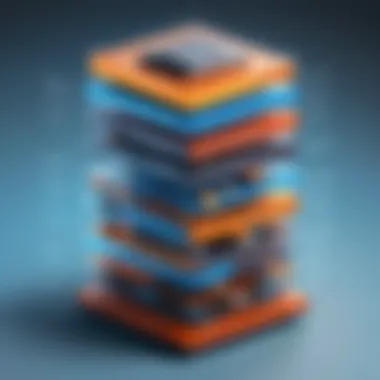
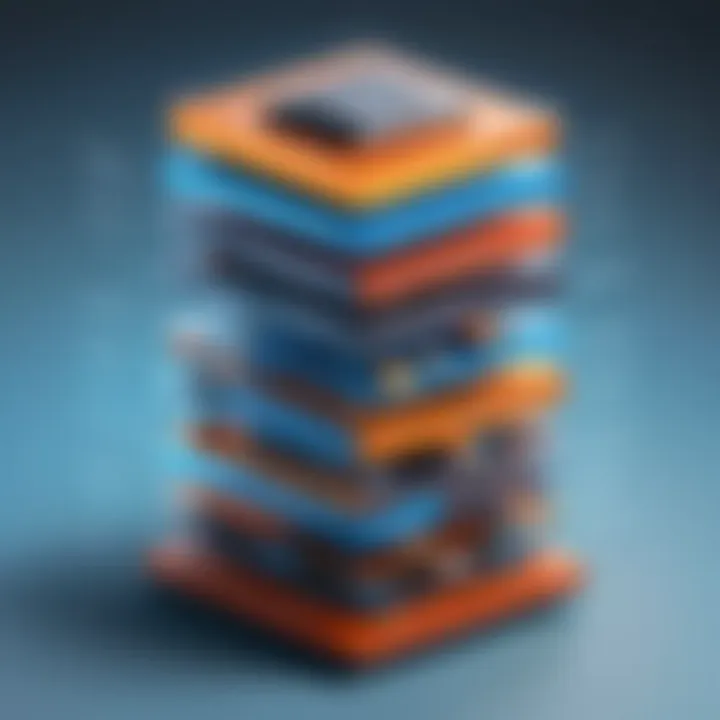
Intro
Building a web application with Java has become a significant undertaking for developers across the globe. Java's robustness and versatility make it an excellent choice, whether you're creating a small project or scaling a massive system. Understanding the fundamental roles and capabilities of Java in web development can help you navigate through the numerous frameworks and tools available today.
In this guide, we will walk through essential concepts, tools, best practices, and real-world applications in the realm of Java web application development.
Overview of Software Development
Software development is a structured process that takes ideas from conception to deployment, combining creativity with technical skills. As businesses increasingly shift towards digital solutions, software developers have become the architects of our online world.
Definition and Importance of the Technology
Web applications are programs accessed via a web browser over a network. They are gathering more users by the day due to their ease of access and powerful functionalities. Java stands out in this arena for several reasons. It’s platform-independent, meaning that you can run a Java application on various devices without needing a complete rewrite of the code. This reduces the time needed for development and makes Java exceptionally desirable in cloud-based environments.
Key Features and Functionalities
- Object-Oriented: Java allows developers to create modular programs and reusable code blocks.
- Strongly Typed Language: The strict data type requirement helps catch errors early in development.
- Multi-Threading: Supports concurrent execution of two or more threads for multitasking.
- Robust Security Features: With in-built security protections, Java applications are safer from threats.
Use Cases and Benefits
Utilizing Java leads to many advantages:
- High scalability and performance
- Extensive libraries and frameworks available such as Spring and Hibernate
- Large community support resulting in consistent updates and troubleshooting resources
Best Practices
To harness the full potential of Java for web application development, it’s vital to follow certain industry standards:
- Keep Code Simple: Complexity often leads to problems. Make your code easy to read and maintain.
- Utilize Version Control: Tools like Git can help manage changes in the codebase seamlessly.
- Regular Testing: Conducting unit tests and integration tests helps catch issues early.
By implementing sound development practices, you pave the way for efficiency and quality.
Common Pitfalls to Avoid
Even seasoned developers might stumble on common mistakes:
- Over-engineering solutions
- Neglecting user experience in favor of technical completeness
- Failing to document code and processes, which complicates future updates
Case Studies
Real-world examples often shed light on practical applications of theoretical knowledge. Netflix is a prime example of a platform built using Java. It scales efficiently, supporting millions of users while providing consistent performance.
Lessons Learned and Outcomes Achieved
Netflix’s consistent updates and scalability made it a leader in streaming services, showing the importance of adaptable software and frequent enhancement.
Latest Trends and Updates
The technology landscape is always shifting. Here’s what’s trending in Java web development:
- Microservices Architecture: Breaking applications into smaller, more manageable services.
- Containerization with Docker: Enhancing portability and efficiency in deployment.
- Integration of AI and Machine Learning: Bringing smarter functionalities to applications.
How-To Guides and Tutorials
For those looking to dive into using Java for web applications, there are plenty of resources available:
- Setting Up Your Environment: Ensure you have Java Development Kit (JDK) and an IDE like IntelliJ IDEA.
- Comprehensive Tutorials: Websites like Codecademy or Coursera offer step-by-step guides for beginners.
To be successful, it's not only about knowing the technology but also embracing the best practices and actively engaging with the community. As we delve deeper into Java's web application capabilities, the ability to remain adaptable to changes will determine one's success in this ever-evolving field.
Preamble to Java Web Applications
In the realm of software development, crafting web applications has become a vital skill. The importance of understanding Java as a programming language for building these applications cannot be overstated. Java, known for its versatility and robustness, enables developers to create scalable and high-performing applications that serve a myriad of needs across industries. In this section, we will delve into what exactly constitutes a web application and highlight the significance of using Java for development in this domain.
Defining Web Applications
Web applications are software programs that execute on a web server and are accessed through a web browser. This definition encompasses a wide range of functionalities, from simple informational pages to complex platforms that incorporate numerous features, such as e-commerce, user management, and dynamic content generation. Essentially, a web application combines various technologies to provide a seamless experience for users, enabling interactions and transactions over the Internet.
- A quintessential example of a web application would be an online shopping platform like Amazon, where users can browse products, add them to a cart, and complete purchases through a secure payment system.
- Another illustration might be Google Docs, which allows multiple users to collaborate on documents in real time, showcasing the dynamic nature of web applications.
In summary, web applications serve as a bridge between users and the underlying data and services, making them essential in today’s digital landscape.
Importance of Java in Web Development
Java occupies a prominent position in the web development ecosystem, largely due to its stability, scalability, and extensive ecosystem of libraries and frameworks. These features provide a strong foundation for building robust applications.
- One of the key advantages of Java is its platform independence, which is facilitated by the Java Virtual Machine (JVM). This allows developers to write code once and run it anywhere, significantly reducing development time and effort.
- Moreover, Java boasts a strong community support. Numerous forums, resources, and libraries are available, making troubleshooting and extending functionality easier.
Java frameworks like Spring have revolutionized the way developers build web applications by providing ready-to-use modules for various tasks, streamlining the development process even further.
"Using Java for web development is akin to having a Swiss Army knife in your toolkit—versatile and effective for a variety of tasks."
Understanding Web Application Architecture
When it comes to building robust web applications, understanding web application architecture is a fundamental piece of the puzzle. It outlines the framework upon which your application is built, casting a spotlight on the interaction between different components. An effective architecture simplifies the development process, enhances scalability, and ensures that applications can meet changing demands over time.
Having a robust architectural understanding allows developers to make informed decisions about framework selection, data flow management, and how to manage user interactions effectively. The architecture serves as a blueprint that guides the implementation phases of the development lifecycle.
Client-Server Model
In the realm of web application architecture, the client-server model reigns supreme. This structure clearly delineates the roles of both the client, which requests services, and the server, which provides them. By dividing responsibilities, this model enables efficient data handling and resource management.
The essence behind this model is to create a clear separation of concerns, which allows for a smoother exchange of information and better fault isolation. In practical terms, this means that if there’s an issue on the server-side, it doesn’t necessarily have to bring down the entire client application. Plus, this is a scalable model, making it a go-to choice for developers when building applications that expect to handle an increasing amount of users.
Layers in Web Application Architecture
Layers in web applications are like the different floors of a building, each serving its unique purpose while contributing towards the overall structure. This layered approach enhances modularity, making it easier for teams to collaborate, iterate, and maintain the application effectiveness over time.
Presentation Layer
The Presentation Layer is what the end-users interact with. Think of it as the face of the application. Its primary role is to display data well and allow user input. A well-designed presentation layer creates a seamless experience that keeps users coming back.
A key characteristic of this layer is that it focuses heavily on user experience. Technologies like HTML, CSS, and JavaScript are its backbone. Its strength lies in its ability to adapt to various devices and users, hence making it often regarded as the most crucial part of web development. Users connect with the UI; if it’s intuitive and visually appealing, it can lead to better engagement and satisfaction. However, it can also be a double-edged sword as poor design choices here can lead to high bounce rates.
Business Logic Layer
The Business Logic Layer brings structure to the application, encapsulating the core operations that process data. It's the brains behind the operation, determining how data is created, stored, and modified. This layer consolidates decisions made in one centralized location, facilitating easy updates.
This layer stands out because it is highly modular. By isolating business rules, developers can alter them without needing to rewrite the entire application. This flexibility can save time in the long run as business requirements evolve. Yet, the unique challenge is that if not adequately designed, changes in business rules can inadvertently impact the entire application.
Data Access Layer
At the heart of your application's data processing is the Data Access Layer. This layer serves as the conduit between the business logic and the database. It is essential for retrieving, storing, and modifying data securely. The focus here is on data integrity and efficiency.
The Data Access Layer is characterized by its capability to interact with various types of databases, whether they are relational or NoSQL. It’s beneficial because it promotes separation of database logic from business logic, allowing for changes to be made easily without disturbing other layers. However, if not carefully managed, it could become a bottleneck due to inefficient queries or poorly optimized data handling.
Common Architectural Patterns
Architectural patterns serve as tried-and-true templates for building your web application. Understanding these patterns enables developers to choose the right one to fulfill the specific needs of their project – and that can be the difference between a smooth process and a full-on headache.
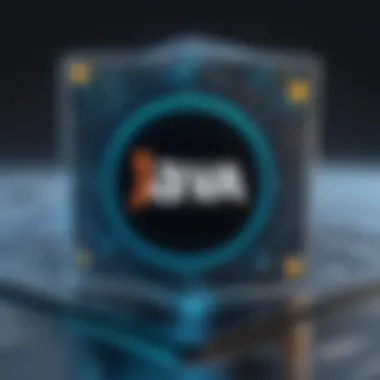
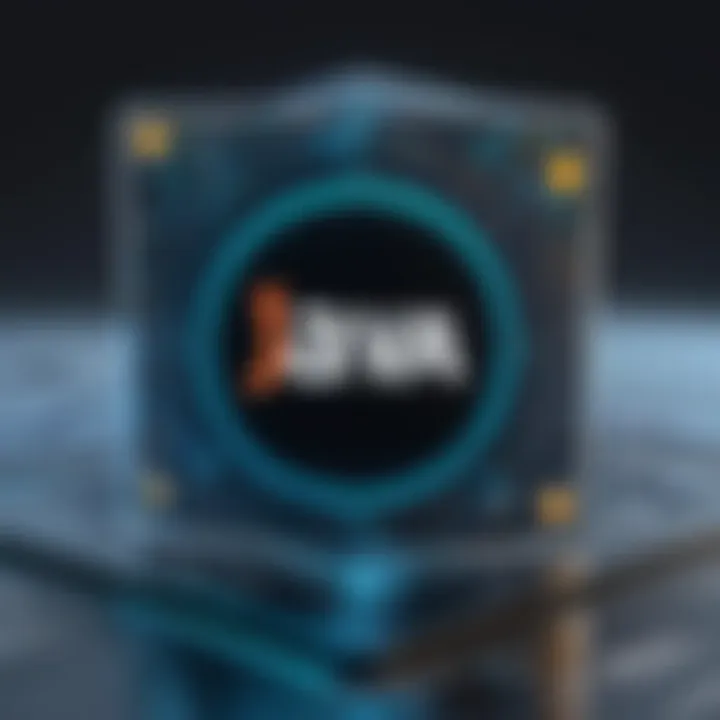
Pattern
MVC, or Model-View-Controller, is one of the most common patterns in web development. It separates the application into three interconnected components: the model, which manages the data; the view, representing the user interface; and the controller, which handles input and coordinates the interaction.
The standout feature of the MVC pattern is this clear division of concern. It allows developers to work on different aspects independently, which in turn improves collaboration and reduces the potential for errors. Its flexibility means that developers can easily switch out views or models without disrupting the entire application. Yet, it can sometimes introduce complexity, particularly in larger applications where communication between these components can become cumbersome.
Microservices Architecture
Microservices Architecture takes a different approach by breaking down applications into smaller, independently deployable services that interact through APIs. Each microservice can be developed in isolation, using various programming languages and data storage technologies that best meet its needs.
This architectural style is characterized by its focus on resilience and agility. If one service faces an issue, it won't necessarily impact the others, allowing the overall application to function smoothly. It’s an appealing choice for teams practicing agile development as it aligns well with rapid iterations. However, coordinating communication between these independent services can be challenging and, if not managed well, might lead to over-complicated systems.
In Summary: A sound understanding of web application architecture is paramount for developers. It equips them with the essential insights required to implement best practices from client-server models to recognizing architectural patterns that will shape the applications of tomorrow.
Setting Up the Development Environment
Setting up the development environment is the sturdy foundation upon which you will build your Java web applications. Good environment configuration can save hours of headaches later on. When everything is organized and functioning correctly, you can focus on what matters — writing exceptional code. In this section, we’ll discuss the key components that make up a robust development setup: tools and IDEs, version control, and dependency management.
Selecting Tools and IDEs
Java Development Kit (JDK)
The Java Development Kit (JDK) is an essential toolkit for anyone working in Java, providing the necessary tools to run and develop applications. This includes the Java Runtime Environment (JRE), development tools like the Java compiler, and additional libraries. One key characteristic that sets JDK apart is its compatibility with multiple platforms, making it a valuable choice for cross-platform development.
One significant feature of JDK is the ability to switch between various versions effortlessly. This is particularly helpful when maintaining legacy applications or experimenting with new features in recent releases. It lays the groundwork necessary for modern development practices. However, beginners might find the initial installation and configuration process a bit daunting, but mastering this step is worth it.
Integrated Development Environments (IDEs)
Integrated Development Environments, or IDEs, are invaluable for streamlining the development process. They provide an all-in-one solution, integrating code editing, debugging, and build automation into one platform. This ensures that developers can focus on their code rather than getting distracted by the tools. A standout feature of IDEs is their smart code completion, which can significantly speed up the coding process and reduce errors.
Popular choices like IntelliJ IDEA and Eclipse are particularly beneficial because they come equipped with plugins that support diverse frameworks and languages. The downside? If you're not careful, those plugins can clutter the workspace, making it tricky to navigate. Still, the enhancements they bring often outweigh the drawbacks, as they facilitate a smoother development flow.
Version Control Systems
Version control systems are the unsung heroes in the developer’s toolkit. They allow developers to track changes in their codebase, revert to previous versions, and collaborate efficiently without overwriting one another's work. Git is the most widely used version control system today, providing seamless solutions for projects, big or small.
With features like branching and merging, Git empowers developers to work in isolation on features before integrating them back to the main codebase. This significantly reduces the risk of introducing bugs into the main application. It's some serious peace of mind in a world where multiple developers often work in parallel. Not using a version control system? Well, good luck keeping chaos at bay!
Dependencies Management
Maven
Maven serves as a robust tool for managing project dependencies. Its primary characteristic is its project object model (POM), which describes how software is built and what dependencies are required. This systematic approach simplifies the process of handling libraries and frameworks crucial for Java applications.
One unique feature of Maven is its ability to manage complex dependency graphs, allowing developers to include libraries while Maven automatically handles the transitive dependencies. Though it can be complex at times, especially for newcomers, the clarity and structure it brings to dependency management make it a popular choice among developers.
Gradle
Gradle is gaining traction as a flexible build automation tool that also excels in dependency management. Unlike Maven, which relies heavily on XML for configuration, Gradle uses a Groovy-based DSL, making it more intuitive and easier to read. Its core characteristic is the build script that enables concise and expressive builds, which is a huge plus for developers.
A unique feature of Gradle is its incremental build capability, which only rebuilds what has changed since the last build. This can translate to significant time savings during development. However, it may have a steeper learning curve than Maven, particularly for those who are accustomed to the latter's structure. Yet, for those who invest the time, the benefits of using Gradle can be tremendous.
"Setting up your development environment is like laying the bricks for a sturdy wall—you can't build a great house without a solid foundation."
As you can see, every piece plays a crucial role in your journey of building a Java web application. Taking the time to set up your development environment correctly pays huge dividends in efficiency and productivity down the line.
Choosing the Right Framework
Choosing the right framework for your Java web application is a decision that can either make or break your project. It’s not just about picking the trending option; it's about understanding the needs of your application and how the framework aligns with your development goals. Whether you’re looking for rapid development, maintainability, or scalability, the right framework can provide the necessary tools and structure to achieve your objectives.
The selection can influence factors such as project complexity, team expertise, and the necessary integration with other technologies. Hence, it’s crucial to evaluate frameworks against the functionality they offer, their learning curve, and community support. A framework should simplify the development process and ultimately enhance the end-user experience.
Overview of Java Web Frameworks
When it comes to Java web frameworks, there’s a multitude of options available, which can be quite overwhelming at first glance. However, understanding a few core frameworks can narrow down the choices significantly. Frameworks can typically be categorized into full-stack frameworks like Spring and Jakarta EE, which offer comprehensive solutions, and micro-frameworks, which focus on specific tasks, thus providing flexibility and simplicity where needed.
It's important to note that each framework comes with its own set of libraries, conventions, and community best practices that guide development. Choosing one isn’t merely a technical decision; it shapes how your application will evolve over time, impacting maintainability and scalability.
Spring Framework
The Spring Framework is a stalwart in the Java ecosystem and remains a go-to choice for many developers. It offers a range of features, from dependency injection to aspect-oriented programming, which not only make applications modular but also easier to test and maintain. Spring’s powerful capabilities can be leveraged to create robust web applications with improved development speed and flexibility.
Spring Boot
Spring Boot, as a part of the Spring Framework, takes much of the complexity out of the equation. Its main draw is the ability to auto-configure applications, drastically reducing the setup time required before you can get to the actual coding. This aspect of Spring Boot is invaluable for developers who want to dive straight into building features rather than fussing over configurations.
The key characteristic of Spring Boot is its opinionated defaults, which guide developers on best practices while allowing enough flexibility for customization when necessary. Its modular approach encourages using only the functionalities you need, which can significantly enhance performance.
One unique feature is the embedded server support (like Tomcat), allowing you to run your application as a stand-alone Java application without the need for external servers. While this is an advantage for simplicity, it might also lead to difficulties in scaling for more extensive systems if not approached carefully.
Spring
Spring MVC brings a structured way of building web applications utilizing the Model-View-Controller design pattern. This division of concerns allows for clear separation in application code which promotes easier maintainability and testability. For developers familiar with web development paradigms, it feels both familiar and powerful.
What stands out about Spring MVC is its versatility; it provides flexibility in terms of views, allowing integration with various front-end technologies. Although it can be complex to configure initially, the trade-off is often worth it given its scalability and the powerful ecosystem it operates within. The framework supports RESTful services out of the box, making it an excellent choice for building modern web applications.
JavaServer Faces (JSF)
JavaServer Faces, commonly referred to as JSF, is another prominent framework that streamlines the development of user interfaces for Java web applications. This framework places emphasis on building reusable UI components that can be assembled into complex interfaces.
JSF’s integration with various Java EE technologies allows developers to leverage backend Java code seamlessly while designing the front-end. Its component-based approach alongside a rich set of libraries can make UI creation straightforward. However, some developers might find JSF less flexible than other frameworks, particularly when it comes to customizing components to meet specific needs.
Jakarta EE
Jakarta EE (formerly Java EE) is an enterprise-level framework that provides a comprehensive set of specifications for building large-scale applications. It extends Java SE beyond simple applications with components for web services, transactions, and security.
The core benefit of Jakarta EE is its robustness in handling enterprise-grade applications, making it a suitable option for applications that require high availability and scalability. Its extensive API support is beneficial, but the complexity involved can pose a challenge for newcomers.
With various frameworks available, including Spring Framework, JSF, and Jakarta EE, the journey of choosing the right one essentially boils down to understanding the unique demands of the application you want to build. By aligning the framework capabilities with your project goals and team skills, you can pave the way for a successful Java web application.
Building the Application Logic
Building application logic is a cornerstone of developing a successful web application. This segment acts as the brains behind the operations, coordinating how data flows, ensuring that it meets user needs, and effectively interacts with the user interface. By solidifying the application logic, developers build a framework that adheres to performance standards while also providing clarity and structure, which is essential for both short-term effectiveness and long-term maintenance.
Creating Data Models
Data models serve as the backbone of any application. They define how data is organized, stored, and manipulated. A well-structured data model not only reflects the business requirements but also aids in optimizing the interaction between different components of the application. To create effective data models, developers often use techniques such as ER diagrams to visualize relationships between different data entities. This process ensures that all entities are interconnected logically, minimizing redundancy and ensuring data integrity.
Defining RESTful Services
RESTful services represent a crucial aspect of modern web applications, enabling them to communicate seamlessly over the HTTP protocol. Adopting REST means that your application can easily integrate with others, making it more versatile and scalable. The architecture revolves around stateless operations, ensuring that the server does not store any information about the client session. This not only simplifies the interactions but also enhances performance. Incorporating RESTful services leads to an intuitive and robust API design that other developers can utilize and interact with efficiently.
Integrating with Databases
Databases are essential for storing, retrieving, and managing application data. The integration process can differ significantly based on the choice of database and the specific requirements of the application. Let’s explore two major types of databases:
Relational Databases
Relational databases like PostgreSQL and MySQL are well-known for their structured data handling. The core feature of these databases is their use of tables that allow for establishing relationships through foreign keys. This organized structure makes it simple to perform complex queries, enhancing data retrieval speeds.
- Key characteristic: Their adherence to ACID properties - ensuring that transactions are processed reliably.
- Why beneficial: They offer a mature ecosystem with extensive documentation and community support, making them a popular choice for developers.
- Unique feature: The ability to perform normalization, which reduces data redundancy and improves data integrity.
- Advantages: Ensure consistency and accuracy of data, suitable for applications that require complex transactions.
- Disadvantages: The rigidity of schemas may make scaling difficult, especially in applications needing frequent schema changes.
NoSQL Databases
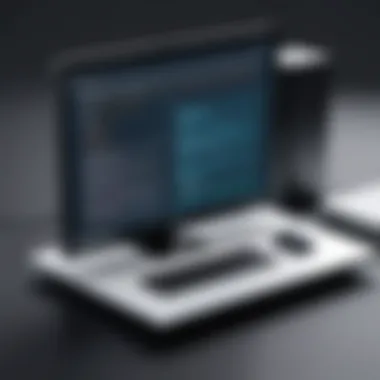
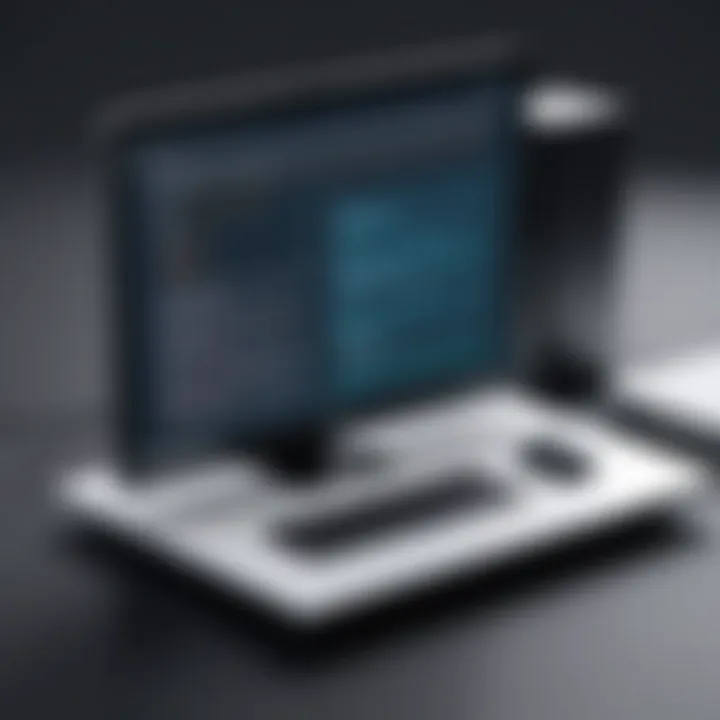
On the other hand, NoSQL databases, such as MongoDB and Cassandra, are great for applications requiring flexibility in data storage. Their architectures allow for various data formats such as key-value pairs, documents, or graph structures.
- Key characteristic: Scalability, as they can handle large volumes of data efficiently and across distributed systems.
- Why beneficial: They often perform better when handling high-velocity data or unstructured data, making them adaptable to changing business needs.
- Unique feature: Support for horizontal scaling, allowing the database to expand effortlessly across multiple servers.
- Advantages: Offer flexibility in data modeling, making them ideal for dynamic applications handling varying data structures.
- Disadvantages: Lack of standardized query languages might lead to compatibility issues, and eventual consistency models can complicate retrieval.
When choosing a database, consider the scale and nature of the data your application will handle. Both relational and NoSQL databases have their place in application development, and understanding their unique characteristics will enable informed decision-making.
By grasping the intricacies of creating data models, defining RESTful services, and integrating with databases, developers can build a solid foundation for their web applications. This ensures that not only are the applications efficient in performance but also robust enough to handle evolving user needs.
User Interface Development
The user interface (UI) plays a pivotal role in the success of any web application, acting as the bridge between the user and the underlying functionality. It’s not merely about making things look pretty; rather, it’s about ensuring that users can navigate the application seamlessly and achieve their tasks effectively. A well-designed UI enhances user satisfaction, keeps users engaged, and often directly impacts the usability and overall performance of the application. Given these points, focusing on UI development is a critical aspect in the lifecycle of building a Java web application.
Front-End Technologies Overview
The landscape of front-end technologies is diverse and ever-evolving, comprising various tools and languages—each serving its unique purpose. At the core are HTML, CSS, and JavaScript, the three musketeers of web development. Together, they lay the foundation for creating interactive and visually appealing interfaces. HTML structures the content, CSS styles it, and JavaScript brings it to life with dynamic interactions. Updates in these technologies also influence how applications are built, pushing developers to stay current with the latest standards, protocols, and best practices.
Using HTML, CSS, and JavaScript
When it comes to building engaging web interfaces, HTML, CSS, and JavaScript are indispensable.
- HTML provides the basic structure for the web pages, defining elements like headers, footers, and layout.
- CSS enhances the appearance, allowing developers to apply styles, layout designs, and responsiveness to various devices. Simply put, without proper CSS implementation, a website could look sterile, losing out on engagement.
- JavaScript is the magic wand that makes the pages interactive. From form validations to dynamic content updates, its role is to facilitate direct user involvement and a more fluid experience.
These technologies must work in harmony to create an effective UI that meets the user’s needs and demands. Therefore, understanding their interaction is crucial for any developer aiming to create intuitive interfaces.
Web Frameworks for UI
Frameworks offer structured solutions that streamline the UI development process. Two noteworthy frameworks in the Java ecosystem are Thymeleaf and Angular. Each has its unique strengths, making them suitable for different scenarios, depending on project requirements.
Thymeleaf
Thymeleaf is a modern templating engine that integrates well with Spring applications, allowing for a natural templating syntax while providing a server-side rendering model. Its key characteristic lies in its ability to generate HTML views directly from Java, making it particularly useful for traditional web applications.
- Benefits: Thymeleaf’s integration with Spring is seamless, making it a favorable option for many Java developers. It allows for natural coding without overly complicating things.
- Unique Feature: One of Thymeleaf's notable strengths is its ability to process templates in a web browser, which means that developers can view the template without requiring a server. This can speed up development and troubleshooting processes.
- Disadvantages: However, it's worth noting that Thymeleaf leans heavily on server-side rendering, which might not be the best fit for applications demanding rich client-side interactivity.
Angular
Angular, on the other hand, is a powerful framework for building dynamic single-page applications (SPAs). Its component-based architecture stands out, allowing developers to reuse components efficiently. This framework encourages modular development, which is a boon for larger projects where maintainability and scalability are crucial.
- Benefits: Angular offers two-way data binding, which simplifies how data flows between the model and the view, enhancing user experiences through real-time updates. Moreover, its rich ecosystem provides a host of tools and insights for enhancing development productivity.
- Unique Feature: The ability to create responsive and performance-centric applications is a hallmark of Angular. Its routing capability also allows smooth navigation without reloading the page, ensuring fast interactions.
- Disadvantages: Certain complexities in initial setup and steep learning curves can deter new developers, especially those who are only beginning in web app development.
Testing the Web Application
Testing is like the compass guiding a ship through stormy waters. In the realm of web application development, it is crucial. Testing ensures that everything operates smoothly, meets user expectations, and adheres to security standards. Without rigorous testing, you risk releasing a product that may lead to poor user experience, data breaches, and increased costs in the long run. This section discusses the various types of testing and automation toolsets that keep your app steered in the right direction.
Types of Testing
Unit Testing
Unit testing is the first line of defense in the testing regimen. It focuses on individual components of your application, examining them in isolation. By validating small pieces of code, unit tests ensure that each part functions correctly before being integrated with others. Its ability to identify bugs early in the development cycle makes unit testing invaluable.
A key characteristic of unit testing is its speed; running unit tests is generally quite quick, allowing developers to catch errors without significant delays. This makes it a commonly used approach in agile environments where rapid iterations are the norm.
Furthermore, unit tests serve as excellent documentation for the codebase. When new developers join a project, they can look at unit tests to understand how certain functions behave.
Advantages include:
- Early bug detection
- Improved code quality
- Simplified integration
However, unit testing has limitations. It doesn’t test interactions between components, which brings us to integration testing.
Integration Testing
As the name suggests, integration testing verifies that different pieces of the application work together as intended. This is particularly relevant when combining various modules, libraries, or resources. Integration tests look at the data flow between modules, ensuring they communicate effectively and that data remains consistent and accurate.
One significant advantage of integration testing is that it can catch issues arising from the interaction of multiple units that may not be evident during unit testing. For instance, if Modules A and B work great individually, an integration test might reveal that they struggle to handle shared data correctly.
The unique feature of integration testing is its ability to mimic real-world use cases, which often reveals flaws that unit tests overlook.
Advantages include:
- Better understanding of system behavior
- Identification of interface defects
- Flaw detection in data flows
Like unit testing, integration testing isn’t without its drawbacks. It generally takes more time to set up and execute, and it may require a more complex testing environment.
User Acceptance Testing
User acceptance testing (UAT) is the final frontier before a product goes live. It is conducted by end-users to ensure the application meets their requirements and is ready for real-world scenarios. UAT is vital because it addresses the "will this work for the user?" question. Developers may create software functioning perfectly technically, but if it doesn’t serve user needs, it won’t succeed.
The key characteristic of UAT is its focus on user experience. End-users interact with the application and provide feedback on its functionality, ease of use, and overall satisfaction.
This type of testing has its unique feature: it reveals usability issues that could have been missed in earlier test phases. It's the opportunity to catch anything that might slip through the cracks.
Advantages include:
- Direct user feedback
- Validation of business requirements
- Increased user satisfaction
However, UAT relies heavily on user availability and may not capture edge cases or scenarios not encountered in testing.
Automation Tools for Testing
As projects grow in scope and complexity, the need for automation becomes glaring. Automation tools simplify the testing process by executing test cases repeatedly, reducing the burden on developers. These tools can run unit tests, integration tests, and even UAT in some formats. Popular options include Selenium, JUnit, and TestNG.
With automation, you can save significant time and improve coverage, allowing you to test more of your application in far less time. However, setting up automated tests isn’t a walk in the park. It requires an initial investment of time and resources to create effective automated tests. Nevertheless, once in place, they often yield rich returns in efficiency.
Deployment Strategies
Deployment strategies are vital in the lifecycle of a web application. It is the bridge between coding and the live, functional site that users interact with. Understanding the dynamics of deployment can elevate your application from being just functional on a local environment to thriving in a production environment where it can handle real user traffic, which is no small feat.
A solid deployment strategy ensures that changes can be made with minimal interruptions. It helps determine how to address issues, scale resources, and deliver updates. Fumbling through deployment can lead to downtime, data loss, or miserable user experiences. Thus, planning it out becomes equally important as writing the code itself.
Environment Configuration
When it comes to environment configuration, it's essential to establish distinct settings for development, testing, and production phases. Each environment serves a unique purpose, and ideal practices recommend separating them to avoid unintended complications.
In practice, using environment variables allows developers to manage configurations without hard-coding sensitive information into the main codebase—this is critical. For example, defining database connection strings or API endpoints based on the environment. This not only keeps sensitive data secure, but it also enhances flexibility.
Here's how to approach it:
- Development Environment: This is where you throw code together, experiment, and iron out the bugs. It might look flashy with logging details, but it's not live yet.
- Testing Environment: This mirrors production to close as possible. It's where testing happens, and issues should be ironed out before meeting the real world.
- Production Environment: This is the high-stakes situation where everything needs to be buttoned up. After all, this is where real users engage with your application.
Hosting Options
Choosing the right hosting strategy is like selecting a home for your application; it sets the stage for everything that follows. Each hosting option comes laden with its own set of pros and cons that can dramatically impact performance, scalability, and maintenance efforts.
Cloud Hosting
Cloud hosting has surged in popularity, and it’s easy to see why. The concept revolves around storing your application across multiple servers that work in concert to maintain uptime and performance. One major advantage of cloud hosting is scalability; when your application sees a surge in traffic, cloud services can automatically allocate additional resources.
Key characteristics include:
- Elasticity: Adjust resources in real-time based on usage—a dream come true for applications expecting variable loads.
- Pay-As-You-Go Model: You only pay for what you use, which is friendly for startups and projects that fluctuate.
However, with great power comes responsibility. Depending on the cloud provider, you may face issues like vendor lock-in or unpredictable costs if not carefully monitored.
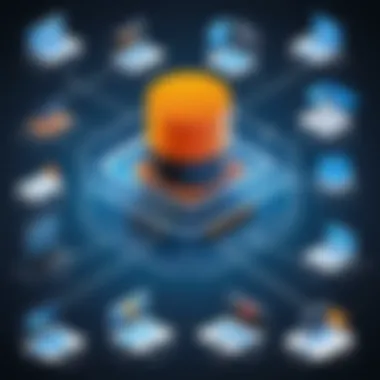
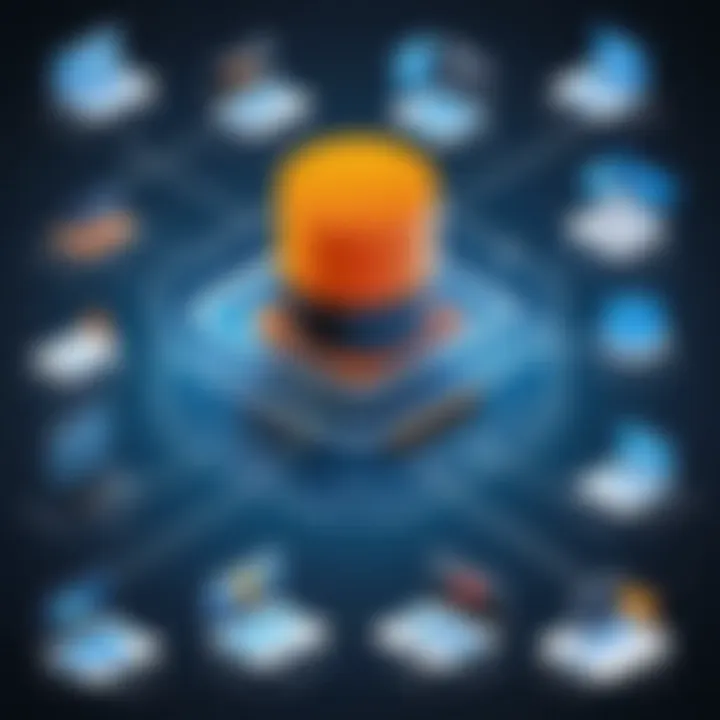
On-Premise Hosting
On the flip side, there's on-premise hosting, often favored by organizations that prioritize security and control. With on-premise hosting, you own the hardware and software, deploying them however you like. Financially, it might look like a hefty upfront cost, but it provides predictability in budgeting.
Key characteristics include:
- Complete Control: You can tailor everything to fit your needs without outside meddling.
- Enhanced Security: In-house data storage means you dictate the level of security implemented.
Nonetheless, there are drawbacks, like the necessity for in-house IT talent to manage and maintain the servers, which can seriously strain smaller teams.
Continuous Integration and Continuous Deployment (/)
CI/CD encapsulates the ongoing process of code integrations and deployments that ensure changes made to the codebase smoothly transition to production. Implementing CI/CD can dramatically enhance the reliability and speed of your development lifecycle. This practice focuses on automated testing, allowing developers to catch bugs early in the process and enabling frequent, reliable releases.
Using tools like Jenkins, Travis CI, or GitLab CI helps streamline these processes, reducing human error and accelerating feedback loops. A well-established CI/CD pipeline can be a game-changer, fostering a culture of innovation while ensuring stability—a fine balance indeed.
Post-Deployment Considerations
Once you’ve rolled out your Java web application, the job's not over. Post-deployment considerations play a crucial role in ensuring the app remains robust, performs well, and meets users' ever-evolving needs. Often, this phase is overlooked, but it can make the difference between a fleeting success and a long-standing solution. Here, we explore the essential aspects of post-deployment: monitoring and maintaining your application.
Monitoring the Application
Monitoring is like the heartbeat of your web app. Just like a watchful parent keeping an eye on a child, diligent monitoring ensures your application runs smoothly after its launch. It helps identify potential issues before they become serious breakages that could push users away.
Key metrics to keep an eye on include:
- Performance Metrics: This involves keeping tabs on response times, throughput, and load times. Nothing turns a user off quicker than a sluggish application.
- Error Rates: Regularly check for errors, exceptions, or bugs that appear post-launch. Tools like Sentry or New Relic can help you automate this process.
- User Interaction: Gauge user engagement through analytics tools to see how the app is being used. This can provide insights to fine-tune features or fix pain points.
Implementing logging mechanisms can be a game-changer here. They serve as breadcrumbs that will help you trace back to the issue if something goes awry. Logging frameworks such as Log4j or SLF4J are solid choices for Java applications.
"An ounce of prevention is worth a pound of cure". Keeping your application monitored will save you headaches down the road.
Upgrading and Maintaining the Application
Upgrading and maintaining the application is like making regular oil changes in a car. It keeps things running smoothly and can prevent larger issues from cropping up. Frequent updates not only patch security vulnerabilities but also improve performance and user satisfaction.
Some key aspects to consider:
- Regular Updates: Keep your libraries and frameworks updated to the latest versions. This can be a daunting task if you’re not using tools like Maven or Gradle to manage dependencies. Automated dependency management helps reduce the risks associated with outdated software.
- User Feedback: Post-deployment, user feedback becomes extremely valuable. Conduct surveys or monitor support requests to identify issues that need addressing.
- Automated Testing: Implement automation for testing features that have changed, ensuring new updates don't inadvertently disrupt existing functionalities. Tools like JUnit or TestNG can streamline this process.
- Documentation: Maintain clear documentation of updates and code changes. Not just for yourself but to help others who might step into the codebase later on. A well-documented application becomes easier to work with and adapt.
Security in Java Web Applications
In today's digital world, the security of web applications cannot be taken lightly. Every line of code, every framework choice, is crucial when we think about security. Java web applications, while robust and widely used, are not immune to threats. This section will delve into the strategies and practices that developers can employ to safeguard their applications against common vulnerabilities.
Identifying Common Threats
When discussing security, it’s essential to identify what the common threats are that could jeopardize a Java web application's integrity. These threats can take various forms, and understanding them is the first step in fortifying your defenses. Some of these include:
- SQL Injection: Attackers use this to inject malicious SQL statements, which leads to unauthorized access to sensitive data.
- Cross-Site Scripting (XSS): This allows attackers to inject client-side scripts into web pages viewed by other users. Malicious scripts can steal session cookies, redirect users, etc.
- Cross-Site Request Forgery (CSRF): This tricked users into unknowingly submitting harmful requests, exploiting their active session.
- Insecure Direct Object References: These occur when an application exposes internal implementation objects, leading to unauthorized file access.
- Sensitive Data Exposure: This happens when sensitive information like passwords, credit card numbers are not properly protected, as seen too often in misconfigured databases.
Awareness is key. Regularly scanning for vulnerabilities can help catch potential threats before they escalate. One effective approach is to conduct an annual security audit and make penetration testing a part of the development cycle.
Best Practices for Securing Applications
Once threats are identified, the next step is implementing best practices to counteract them. Here are some glaringly effective strategies that could protect a Java web application:
- Input Validation: Always validate inputs coming from users. Use libraries like Hibernate Validator to enforce rules on input data.
- Prepared Statements: When interacting with databases, utilize prepared statements as a means of avoiding SQL injection attacks.
- Authentication and Authorization: Implement strong authentication mechanisms, including OAut, to ensure that only authorized users can access sensitive functionalities.
- Use HTTPS: Enforcing HTTPS encrypts data in transit, reducing the risk of man-in-the-middle attacks.
- Error Handling: Be careful about error messages. They should not reveal sensitive information that could help an attacker.
- Regular Updates: Keeping your frameworks and libraries up-to-date can help mitigate known vulnerabilities. Please ensure you are using the latest version of Spring Framework, for instance.
Maintaining a top-notch security posture is not a one-time effort but an ongoing process.
- Implement Security Headers: Utilize HTTP security headers like Content Security Policy (CSP), X-Frame-Options, and others to prevent different types of attacks.
Adapting these practices into the development workflow can bolster security tremendously. The journey does not end here; ongoing vigilance in monitoring and responding to new threats is critical. Security in Java web applications is like a high-wire act. It requires constant attention, the right tools, and a seismic commitment to best practices.
Performance Optimization
Performance optimization is a cornerstone of developing any successful web application, particularly when using Java. No user wants to sit twiddling their thumbs while waiting for pages to load; thus, optimizing performance is critical not just for user satisfaction, but also for reducing server load and enhancing overall system efficiency. A well-optimized application can handle more users while delivering a seamless experience, which translates to higher engagement and retention rates.
Analyzing Performance Metrics
Before diving into optimization, it’s essential to grasp the metrics used to gauge performance. This can include factors such as response times, memory usage, and CPU load. Tools like JMeter or VisualVM can help gather detailed metrics.
- Response Time: This is the time it takes for the server to respond to a user request. Measuring this can help pinpoint bottlenecks in your application.
- Throughput: The number of transactions processed in a given timeframe. Higher throughput indicates a more capable application.
- Resource Utilization: Understanding how memory and CPU are being used can identify inefficiencies or areas that need attention.
- Error Rate: Monitoring this gives insights into potential issues that might cause more strain on the system. If a particular endpoint sees a high error rate, it could lead to performance degradation.
Analyzing these metrics regularly can lead to data-driven decisions that refine your application significantly.
Techniques for Optimization
Caching
Caching is a critical technique for optimizing performance in web applications. It involves storing the results of expensive operations so that subsequent requests can be served faster without re-executing those operations.
One key characteristic of caching is that it minimizes the need for repeated database queries or computations, which can often be the Achilles' heel of a web application. It’s a popular choice because it significantly improves response times, reduces server load, and enhances user experience. However, caching is not without its quirks. If the cache is not managed properly, it can lead to stale data being served to users, which could be detrimental depending on the application's functionalities.
- Advantages: Faster responses, reduced server load, better user satisfaction.
- Disadvantages: Potentially outdated data, complexity in cache invalidation.
Load Balancing
Load balancing is another prominent technique for optimizing web application performance. At its core, it distributes incoming network traffic across multiple servers, ensuring no single server becomes a bottleneck.
One notable characteristic of load balancing is its ability to enhance the reliability of your application. By spreading the user load, the service can continue functioning even if one or more servers go down, which is crucial for maintaining uptime. This technique is particularly beneficial for handling traffic spikes, often seen in web applications during peak hours.
- Advantages: Improved reliability, enhanced user experience during peak times, fault tolerance.
- Disadvantages: Requires additional infrastructure and careful monitoring to ensure the load is balanced effectively.
Future Trends in Java Web Development
In the rapidly evolving world of technology, understanding the future trends in Java web development is not just a necessity; it’s integral for developers aiming to stay ahead of the curve. This section explores several promising directions that indicate how Java will continue to be leveraged in creating robust and scalable applications. By taking a hard look at these trends, developers and tech enthusiasts alike can prepare themselves for the shifts in the industry, ensuring they remain not only relevant but also competitive.
Emerging Technologies
A plethora of emerging technologies shapes the landscape of web development. Java, long known for its robustness and stability, is also adapting to include innovative trends. Some key technologies to keep an eye on include:
- Artificial Intelligence (AI): Integrating AI into Java applications allows developers to create smarter applications capable of data analysis and decision-making. Libraries like Deeplearning4j facilitate this endeavor, offering extensive tools for building AI models within Java.
- Microservices Architecture: This approach segments applications into smaller, manageable components that work independently. Java frameworks, such as Spring Boot, are particularly well-suited for microservices, enhancing scalability and easing the deployment process.
- Serverless Computing: Although not solely a Java initiative, serverless architecture is emerging as a trend that Java developers will want to incorporate. Using tools such as AWS Lambda allows Java applications to execute in response to events without the overhead of managing server infrastructure.
"Emphasizing modularization through emerging technologies enables a more agile and responsive development process."
Developers should explore these technologies to see how they can be integrated into their projects to deliver more meaningful and engaging user experiences.
The Role of Java in Cloud Computing
Java’s adaptability ensures its relevance in the cloud computing realm. As organizations shift towards cloud-based infrastructure, Java developers gain an edge by understanding key elements of how Java interacts with cloud ecosystems:
- Cloud-Native Development: This environment promotes designing applications that are fully orchestrated in the cloud. Java Spring Cloud eases the process, offering tools that align Java applications with cloud-native practices.
- Scalability: Java’s performance hinges on its ability to scale efficiently in the cloud. With services such as Kubernetes, developers can employ containerization for better resource management and scalability.
- Infrastructure as Code (IaC): Utilizing tools like Terraform with Java allows for streamlined cloud resource management. Developers can write scripts to automate provisioning, making deployment faster and less error-prone.
Understanding these aspects not only enhances the efficacy of Java developers but also positions them as valuable contributors in environments that increasingly embrace cloud solutions.
Finale
In wrapping up our discussion on building web applications with Java, it's clear that mastering this powerful programming language directly links to the success of modern web solutions. The importance of a solid conclusion extends beyond summarizing; it's about re-emphasizing the core elements that lead to effective development practices. By tying things together, we can reinforce the critical aspects that have been examined throughout the guide.
Recap of Key Takeaways
Throughout this article, several crucial takeaways deserve highlighting. Firstly, understanding the architectural landscape of web applications lays the groundwork for any successful project. Recognizing the client-server model and the various layers involved — such as the presentation, business logic, and data access layers — can significantly impact how developers approach problems.
Secondly, choosing the right framework can streamline the development process. Frameworks like Spring and Jakarta EE provide robust environments that simplify many of the repetitive tasks developers face, allowing them to focus on the unique value their application can provide.
Lastly, the deployment strategies align closely with the ultimate user experience. Properly managing deployment considerations ensures that applications not only perform well but also adapt swiftly to user needs and threats in an ever-evolving digital landscape.
Final Thoughts on Java Web Application Development
The realm of Java web application development continues to evolve, bringing with it fresh challenges and opportunities. As technology advances, embracing emerging methodologies, tools, and frameworks is no longer optional; it's essential. While Java remains a steadfast choice for many developers, understanding how to leverage its capabilities fully across various platforms offers a competitive edge.
In essence, the principles discussed here—architecture, framework selection, testing, security, and deployment—coalesce into a comprehensive strategy that not only aids developers but also enhances user satisfaction and engagement. As you carve your path in the world of Java web development, remember that each application is a journey. Every line of code contributes to a larger narrative that interacts with users and meets their needs.
As they say, the proof of the pudding is in the eating. Only by putting into practice the lessons within this guide will you truly grasp the richness of what Java can offer in web application development.