The Ultimate Guide to Building a Java Application: A Developer's Handbook
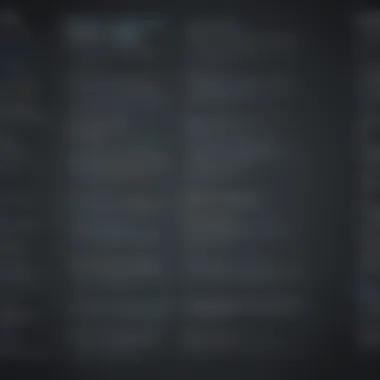
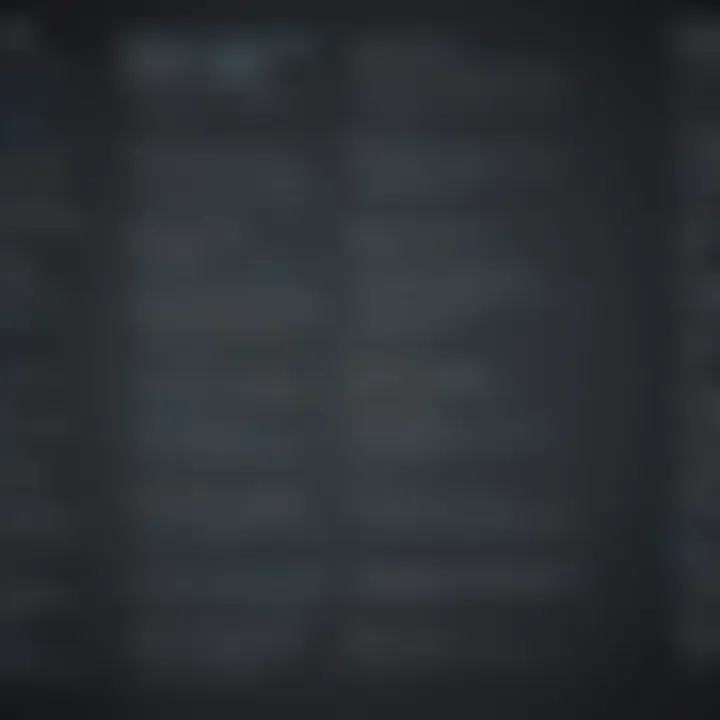
Overview of Java Development
Java development is a crucial aspect of software engineering, offering developers a versatile and robust language to create applications across various platforms. With its platform independence and object-oriented approach, Java is highly favored in the tech industry for its scalability and performance. Key features of Java include its strong community support, extensive libraries, and compatibility with different operating systems. Developers can leverage Java for web applications, enterprise software, mobile apps, and embedded systems, making it a versatile choice for diverse projects. The benefits of Java development encompass improved productivity, code reusability, and enhanced security. Its robust performance and portability ensure that Java applications run consistently across different environments, providing a seamless user experience.
Best Practices for Java Application Development
To ensure successful Java application development, industry best practices emphasize the importance of modular programming, clean code, and thorough testing. By breaking down complex functionalities into manageable modules, developers can enhance code readability, maintainability, and scalability. Tips for maximizing efficiency and productivity in Java development include adhering to coding standards, implementing design patterns, and utilizing frameworks like Spring or Hibernate. Consistent code reviews, unit testing, and continuous integration practices are vital for identifying and addressing issues early in the development cycle. Common pitfalls to avoid in Java development involve inefficient memory management, lack of code documentation, and neglecting security vulnerabilities. By following best practices and staying updated with industry trends, developers can proactively mitigate risks and deliver high-quality Java applications.
Case Studies in Java Application Development
Real-world examples showcase the successful implementation of Java applications in diverse industries, such as finance, healthcare, e-commerce, and more. Case studies highlight the challenges faced, strategies employed, and outcomes achieved through innovative Java development. Lessons learned from prominent case studies underscore the significance of agile development methodologies, user-centric design, and proactive collaboration between developers and stakeholders. Insights from industry experts shed light on emerging trends, best practices, and recommendations for optimizing Java application development processes.
Latest Trends and Innovations in Java Development
The Java ecosystem is continuously evolving, with upcoming advancements focusing on enhanced performance, modularization, and cloud-native architectures. Current industry trends reflect a growing interest in microservices, serverless computing, and artificial intelligence integrations within Java applications. Innovations such as GraalVM, Quarkus, and reactive programming frameworks demonstrate the Java community's commitment to adapt to modern software development paradigms. With a shift towards cloud computing and DevOps practices, Java developers are exploring new tools and approaches to streamline application deployment and maintenance.
How-To Guides for Java Developers
Step-by-step guides and tutorials offer practical insights for both beginners and advanced Java developers looking to enhance their skills. From setting up a Java development environment to building complex applications, these tutorials provide hands-on experience and actionable tips for effective Java development. Practical advice on optimizing code performance, debugging techniques, and integrating third-party APIs empower developers to create high-quality Java applications. By following detailed how-to guides, Java enthusiasts can explore new features, tools, and frameworks to expand their proficiency in Java development.
Introduction
Java has long been a dominant player in the realm of software development, renowned for its versatility and reliability. In this comprehensive guide to building Java applications, we delve deep into the intricate process that developers undertake to create robust and efficient software solutions. From the foundational aspects to the advanced stages of deployment, this article serves as a roadmap for developers looking to navigate the complexities of Java application development.
Understanding Java Development
Overview of Java as a Programming Language
Java's significance in the programming landscape cannot be overstated. As one of the most widely-used languages, Java offers a robust and secure platform for developing a myriad of applications. The language's cross-platform compatibility and strong community support make it a top choice for developers aiming for flexibility and versatility in their projects. Its object-oriented nature and extensive standard library further enhance its appeal, enabling developers to craft efficient and scalable solutions with relative ease.
Benefits of Using Java for Application Development
The benefits of employing Java in application development are manifold. Java's platform independence allows developers to write code once and run it on any platform, reducing the complexities associated with managing multiple code bases. Additionally, Java's emphasis on object-oriented programming promotes code reusability and modularity, enhancing the maintainability of applications over time. Its robust security features and built-in memory management contribute to the development of stable and secure software products.
Key Considerations Before Building
Defining Application Requirements
Before embarking on the development journey, it is essential to crystallize the requirements of the application. By meticulously outlining the functionality, performance benchmarks, and user experiences expected from the software, developers lay a solid foundation for the development process. Clear requirements not only streamline development but also serve as a guiding beacon throughout the project lifecycle, ensuring that the final product aligns with stakeholders' expectations.
Selecting the Right Development Tools
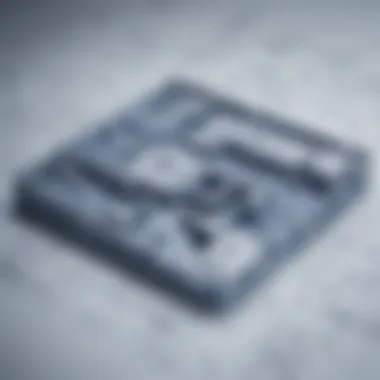
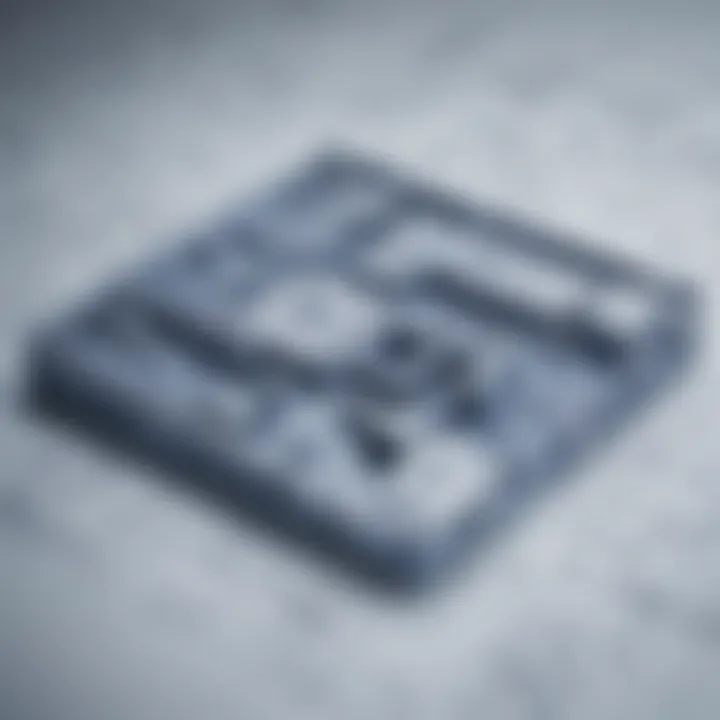
The choice of development tools can significantly impact the efficiency and outcomes of the development process. By selecting tools tailored to the project's requirements, developers enhance collaboration, streamline code integration, and facilitate seamless debugging. From integrated development environments (IDEs) to version control systems, each tool plays a crucial role in optimizing the development workflow and ensuring the successful realization of the application.
Setting Up the Development Environment
Setting up the development environment is a critical phase in Java application development. This section focuses on preparing the groundwork for efficient coding, testing, and deployment of software solutions. By establishing the right tools and configurations, developers can streamline their workflow and ensure the smooth progression of the development process. The thorough setup contributes significantly to the overall quality and performance of the Java application.
Installing JDK
Installing the Java Development Kit (JDK) is an essential step in setting up the development environment. JDK provides the necessary tools and components for compiling, executing, and debugging Java code. Downloading and configuring JDK involves obtaining the appropriate JDK version compatible with the project requirements and setting up the PATH and JAVA_HOME environment variables. This procedure ensures that the Java compiler and runtime environment are readily accessible from any directory in the system.
Downloading and Configuring JDK
Downloading and configuring JDK involve fetching the JDK distribution from the official Oracle website or alternative sources. Once downloaded, the installation process includes setting up the PATH variable to include the JDK's 'bin' directory and defining the JAVA_HOME variable to point to the JDK installation directory. These configurations enable seamless execution of Java applications and facilitate compiling source code.
Setting Environment Variables
Setting environment variables in the development environment plays a vital role in defining the system configurations for Java development. By adjusting environment variables, developers customize the runtime behavior, class paths, and system settings for the Java Virtual Machine (JVM). Properly configured environment variables ensure consistency in development environments across different systems and prevent compatibility issues during code execution.
Choosing an IDE
Selecting an Integrated Development Environment (IDE) is a crucial decision that greatly impacts the development process. IDEs provide a unified platform for code writing, testing, and debugging, enhancing developer productivity and code quality. Understanding the features and capabilities of popular Java IDEs, as well as customizing them for optimal development, is essential for building Java applications efficiently.
Overview of Popular Java IDEs
An overview of popular Java IDEs includes examining the functionalities, plugins, and user interfaces offered by platforms like IntelliJ IDEA, Eclipse, and NetBeans. Each IDE has distinct features such as code completion, syntax highlighting, and project management tools that cater to different preferences and requirements. Evaluating the strengths and weaknesses of each IDE empowers developers to make informed decisions based on their coding practices and project scope.
Customizing IDE for Optimal Development
Customizing the IDE settings to align with individual development preferences and project requirements enhances coding efficiency and performance. By configuring code templates, key bindings, themes, and plugins, developers can personalize their IDE environment for seamless workflow. Optimizing the IDE setup for collaboration, version control integration, and automated testing fosters a conducive development ecosystem that accelerates project delivery.
Designing the Application Architecture
In the intricate process of developing a Java application, one of the pivotal stages is Designing the Application Architecture. This phase lays the groundwork for the entire project, defining the structure and organization of the software. By meticulously planning the architecture, developers can create a robust foundation that supports scalability, maintainability, and efficiency throughout the application's lifecycle. Considering aspects like system components, data flow, and interaction paradigms at this early juncture can preemptively address potential challenges and streamline development.
Understanding Pattern
Model-View-Controller Architecture Overview
Within the realm of Java application development, the Model-View-Controller (MVC) architectural pattern plays a crucial role. This framework divides the application into three interconnected components - the model (data representation), view (user interface), and controller (handling user input). MVC offers a clear separation of concerns, promoting modular design and enhancing code reusability. By isolating data manipulation from presentation logic, MVC simplifies maintenance and encourages code organization, making it a favored choice for developers aiming for structured and scalable applications.
Benefits of in Java Applications
The adoption of MVC in Java applications brings forth several advantages. Firstly, MVC establishes a logical structure that simplifies development by segregating components based on their responsibilities, fostering code clarity and reducing complexity. Additionally, the division of concerns in MVC allows for parallel development, enabling teams to work on different aspects simultaneously. This parallelism enhances productivity and streamlines the overall development process. Moreover, the modular nature of MVC facilitates code reuse, making it easier to extend and modify the application without significant refactoring. Despite these benefits, it's essential to note that while MVC promotes maintainability and scalability, it may introduce some overhead in terms of initial setup and learning curve.
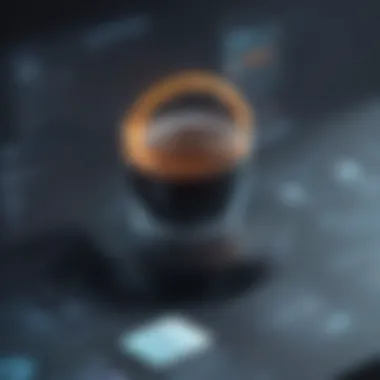
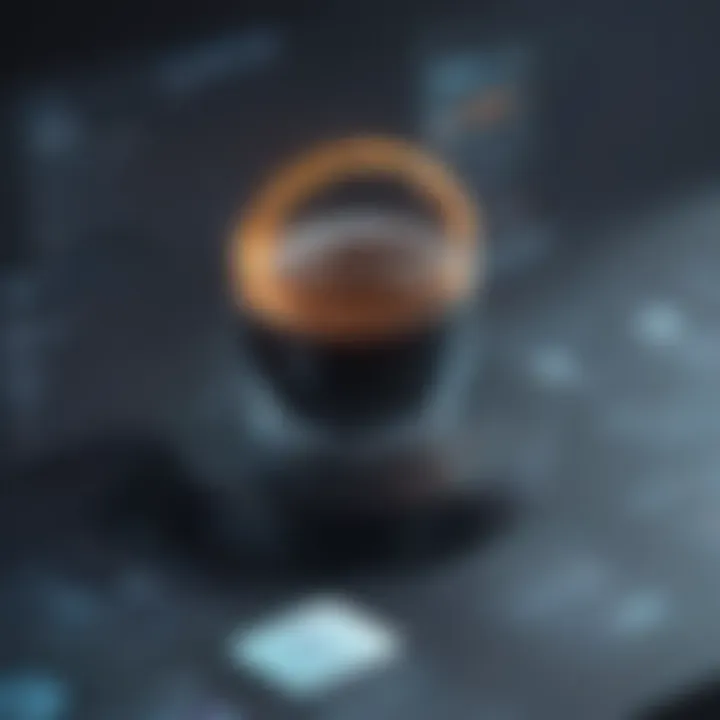
Database Design and Integration
Selecting Database Technology
When it comes to Java application development, the choice of database technology holds substantial importance. Selecting the appropriate database technology involves considering factors such as data requirements, scalability, performance, and compatibility with the application architecture. The selection process should align with the project's needs, ensuring seamless integration and optimal data management. Various database options, including relational (SQL) and non-relational (NoSQL) databases, offer distinct features and capabilities, catering to diverse application scenarios.
Connecting Java Application to a Database
Integrating a Java application with a database entails establishing a robust connection that facilitates data retrieval, storage, and manipulation. The seamless interaction between the application and the database is paramount for ensuring data consistency and reliability. By encapsulating database access within well-defined modules, developers can enforce data integrity and enhance security. Additionally, employing efficient querying techniques and transactions optimizes database performance and overall application responsiveness. While connecting a Java application to a database enhances data-driven functionality, it's crucial to address potential challenges such as network latency, data mapping discrepancies, and scalability considerations.
Implementing Functionality
In the realm of Java application development, Implementing Functionality stands as a crucial phase that bridges the gap between conceptualization and realization. This section of the comprehensive guide focuses on the intricate process of writing Java code and testing its functionality to ensure a robust and efficient software solution. By delving into Implementing Functionality, developers can unleash the full potential of their applications, incorporating essential features and leveraging Java libraries to enhance performance and usability. From laying the foundation of core functionalities to fine-tuning with testing and debugging, this stage truly shapes the functionality and effectiveness of Java applications.
Writing Java Code
Implementing Core Features
Implementing Core Features is the bedrock of any Java application, as it encompasses the essential functionalities that define its purpose and utility. By meticulously crafting and integrating core features, developers can ensure that the application aligns seamlessly with user requirements and business objectives. The key characteristic of Implementing Core Features lies in its ability to translate abstract concepts into tangible functionalities, offering a user-centric approach that enhances the overall user experience. This choice is popular among developers for its versatility and scalability, allowing for the development of complex features while maintaining code clarity and maintainability. The unique feature of Implementing Core Features is its adaptability to evolving requirements, enabling developers to iterate and enhance functionality as needed. While advantageous in enabling tailored solutions, developers must be mindful of potential complexity and performance implications when implementing extensive core features.
Utilizing Java Libraries
Utilizing Java Libraries plays a vital role in extending the functionality of Java applications by leveraging pre-built modules and components to expedite development and enhance functionality. The key characteristic of Utilizing Java Libraries lies in its ability to provide developers with a vast ecosystem of resources, ranging from data processing libraries to UI components, that can streamline development and bolster application performance. This choice is beneficial for developers looking to expedite development cycles and harness the expertise of the Java community to address common development challenges efficiently. The unique feature of Utilizing Java Libraries is the integration of specialized functionalities that would otherwise require significant development effort, empowering developers to focus on core application logic. While advantageous in accelerating development, developers should carefully evaluate library dependencies and ensure compatibility to avoid potential conflicts or security vulnerabilities.
Testing and Debugging
Unit Testing with JUnit
Unit Testing with JUnit plays a pivotal role in ensuring the reliability and stability of Java applications by validating individual units of code to detect errors and regressions early in the development process. The key characteristic of Unit Testing with JUnit lies in its automation capabilities, allowing developers to efficiently run test cases and analyze results to maintain code quality and functionality. This choice is popular among developers for its simplicity and integration with popular IDEs, enabling seamless test-driven development practices and fostering a culture of quality assurance throughout the development lifecycle. The unique feature of Unit Testing with JUnit is its ability to facilitate continuous integration workflows, enabling developers to automate test execution and swiftly identify and rectify issues, contributing to accelerated development cycles and improved software quality. While advantageous in promoting code reliability, developers must invest in comprehensive test coverage and rigorous testing scenarios to maximize the effectiveness of Unit Testing with JUnit.
Debugging Techniques
Debugging Techniques serve as a strategic toolkit for developers to identify, diagnose, and rectify errors and anomalies within Java applications to ensure seamless functionality and performance. The key characteristic of Debugging Techniques lies in its systematic approach to isolating issues, utilizing various debugging tools and methodologies to pinpoint root causes and implement precise fixes. This choice is beneficial for developers seeking to streamline troubleshooting processes and enhance code maintainability by addressing bugs and issues proactively. The unique feature of Debugging Techniques is their versatility in addressing a wide range of issues, from logical errors to performance bottlenecks, empowering developers to optimize application performance and user experience. While advantageous in fostering a proactive debugging culture, developers should prioritize code readability and documentation to streamline troubleshooting efforts and facilitate collaborative debugging endeavors.
Optimizing Performance
In the journey of building a Java application, optimizing performance holds a pivotal role. This section delves deep into enhancing the efficiency and speed of the software, ensuring seamless operation and user satisfaction. By focusing on optimizing performance, developers can elevate the overall functionality and responsiveness of the Java application, leading to improved user experience and system scalability. This aspect underscores the critical need for developers to fine-tune their code and system configurations to maximize performance capabilities.
Performance Profiling
Identifying Performance Bottlenecks
One critical aspect of performance profiling is the identification of performance bottlenecks, which are specific areas within the application where performance is suboptimal. By pinpointing these bottlenecks, developers can address the root causes that impede the software's performance. This proactive approach allows for targeted optimizations, ultimately leading to a more streamlined and efficient Java application. Identifying performance bottlenecks is instrumental in enhancing the overall speed and responsiveness of the software, ensuring that it meets the desired performance metrics and user expectations.

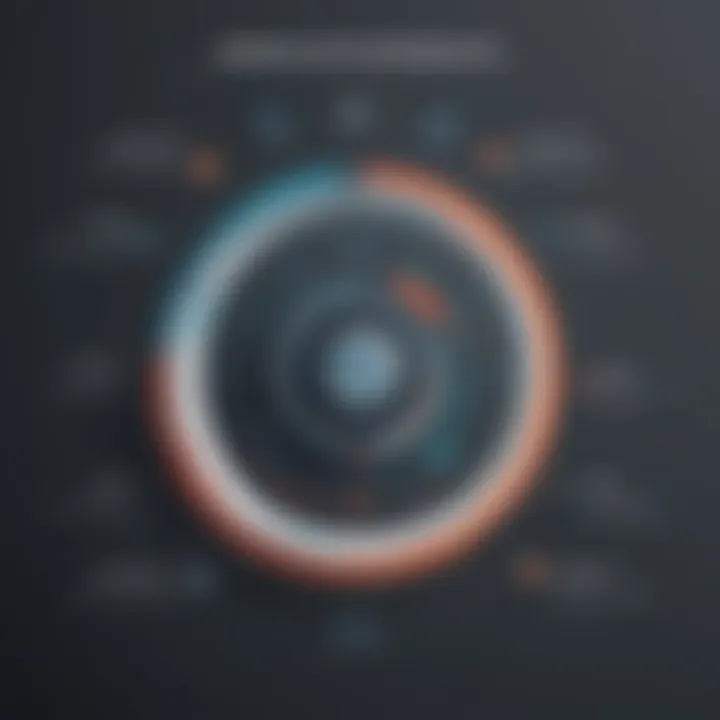
Optimization Strategies
Optimization strategies play a crucial role in maximizing the efficiency and effectiveness of a Java application. By implementing well-defined optimization strategies, developers can fine-tune the codebase, improve resource utilization, and enhance overall system performance. These strategies encompass a wide range of techniques, including algorithmic optimizations, parallel processing, and caching mechanisms, tailored to address specific performance challenges. The strategic deployment of optimization strategies empowers developers to create high-performing Java applications that deliver superior user experiences and operational excellence.
Memory Management
Memory management is a fundamental aspect of optimizing performance in Java applications, directly influencing system resource utilization and application efficiency. By effectively managing memory resources, developers can mitigate common issues such as memory leaks and inefficient garbage collection, ensuring optimal performance and stability. Implementing robust memory management practices enhances the scalability and reliability of Java applications, enabling them to handle increasing workloads and complex computations with ease.
Garbage Collection in Java
Garbage collection in Java automates the process of memory management by identifying and reclaiming unused memory for reuse. This crucial mechanism eliminates manual memory management errors and prevents memory leaks, safeguarding the application against performance degradation. The inherent advantages of garbage collection in Java include improved system reliability, reduced memory overhead, and enhanced developer productivity. By integrating efficient garbage collection strategies, developers can optimize memory usage and enhance application performance significantly.
Memory Leak Detection
Memory leak detection is a vital component of memory management that helps identify and rectify memory leaks, a prevalent issue in Java applications. By detecting memory leaks early on, developers can prevent memory exhaustion, system crashes, and performance degradation. Utilizing sophisticated memory leak detection tools and techniques, developers can systematically identify and resolve memory leakage issues, fortifying the application's stability and performance. Effective memory leak detection mechanisms are indispensable for ensuring the long-term sustainability and optimal operation of Java applications.
Deployment and Maintenance
In the realm of Java application development, Deployment and Maintenance stand as pivotal phases that can dictate the success and longevity of software products. The process of deploying an application involves packaging it into a format suitable for distribution and installation on various environments. Proper deployment ensures that the application runs smoothly and efficiently, meeting user expectations. On the other hand, maintenance is about sustaining the application's performance over time, addressing bugs, adding new features, and adapting to technological advancements. It plays a crucial role in the software development lifecycle, ensuring that the application remains relevant, secure, and optimized.
Packaging the Application
Creating JAR or WAR Files
Creating JAR (Java Archive) or WAR (Web Archive) files is a fundamental aspect of packaging a Java application. These archive formats encapsulate all the necessary files, resources, libraries, and configurations required for the application to execute correctly. JAR files are commonly used for standalone applications, libraries, or frameworks, while WAR files are tailored for web applications specifically. The packaging process simplifies deployment by providing a single file that can be easily shared and run on different platforms. JAR files, in particular, offer portability and ease of distribution due to their self-contained nature, containing all dependencies within a single file. However, managing large JAR files can sometimes pose challenges in terms of version control and conflicts.
Configuration for Deployment
Configuration for deployment involves establishing the settings and parameters necessary for the application to operate effectively in a given environment. This includes defining database connections, setting up server configurations, specifying logging mechanisms, and configuring security settings. A well-thought-out deployment configuration ensures that the application works optimally in various deployment scenarios, such as development, testing, and production environments. It facilitates scalability, performance tuning, and maintenance throughout the application's lifecycle. However, improper configuration can lead to deployment failures, performance issues, security vulnerabilities, and compatibility problems. Therefore, meticulous attention to detail and thorough testing are essential in configuring deployments for Java applications.
Continuous Integration and Delivery
Automating Build Processes
Automating build processes streamlines the development workflow by automatically compiling, testing, and packaging code changes into executable artifacts. Continuous integration tools like Jenkins, Bamboo, or GitLab CICD enable teams to integrate code changes frequently, verify the build integrity, and detect issues early in the development cycle. By automating repetitive tasks such as building, testing, and deploying applications, development teams can increase productivity, reduce manual errors, and ensure consistency across environments. Automatic build processes promote collaboration, enable faster feedback loops, and support the rapid delivery of features to end-users.
Best Practices
CICD (Continuous IntegrationContinuous Delivery) best practices encompass a set of principles and techniques aimed at enhancing software development practices. By automating testing, deployment, and release processes, CICD practices accelerate the delivery of code changes to production, increasing deployment frequency and reducing time-to-market. Implementing CICD pipelines allows for quick validation of code quality, seamless integration of new features, and efficient error detection and resolution. Continuous delivery fosters a culture of continuous improvement, collaboration, and innovation within development teams, leading to faster delivery cycles and higher quality software products.
Monitoring and Updates
Performance Monitoring Tools
Performance monitoring tools enable developers to assess the health and performance of Java applications in real-time. These tools track key metrics such as response times, CPU utilization, memory usage, and error rates, providing insights into application behavior and identifying performance bottlenecks. By analyzing performance data, developers can optimize application performance, enhance user experience, and ensure the scalability and reliability of Java applications. Performance monitoring tools play a vital role in proactively identifying issues, fine-tuning configurations, and optimizing resource utilization to deliver optimal application performance.
Rolling Out Updates Safely
Rolling out updates safely involves deploying new code changes, features, or patches to production environments without disrupting service availability or introducing regressions. This process requires meticulous planning, testing, and monitoring to ensure that updates are deployed smoothly and seamlessly. By following best practices such as feature flags, canary releases, and AB testing, development teams can mitigate risks, gather valuable feedback, and safeguard the user experience during updates. Rolling out updates safely prioritizes maintaining system stability, minimizing downtimes, and delivering consistent service quality to end-users.