Mastering Authentication Tokens in ASP.NET Core
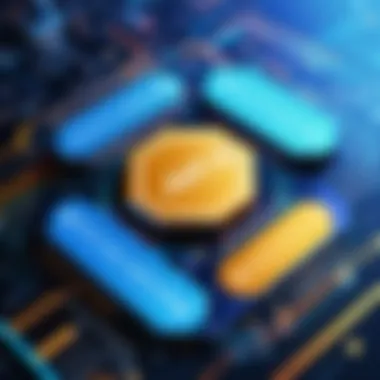
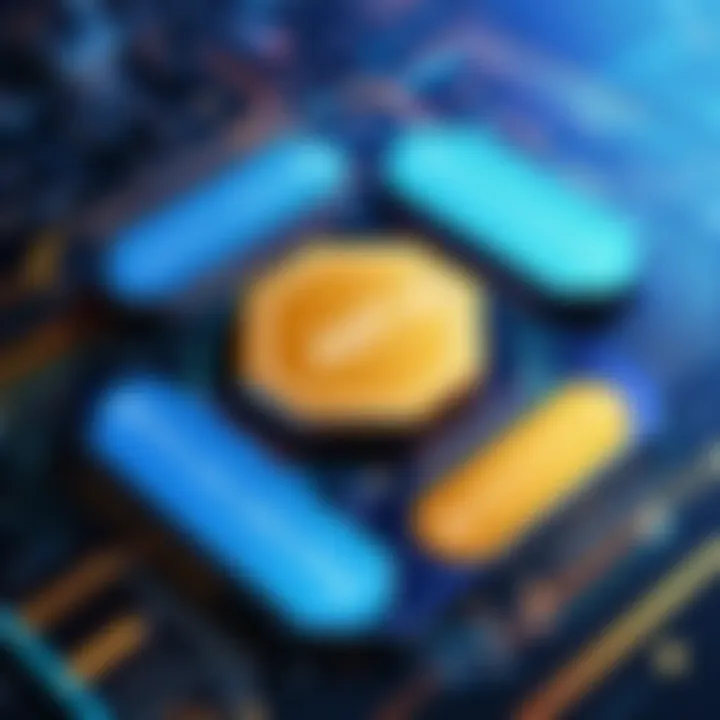
Intro
In today's digital landscape, security is paramount. As applications evolve, so does the need for robust authentication mechanisms. In the context of ASP.NET Core Web API, implementing authentication tokens is essential to safeguard user data and ensure that sensitive operations are performed by authenticated users. This guide outlines the process for effectively integrating authentication tokens, specifically focusing on JSON Web Tokens (JWT).
This approach provides a scalable and user-friendly solution for managing authentication in modern web applications. By adhering to best practices and leveraging industry standards, developers can create secure environments for their applications.
Overview of Authentication Tokens
Authentication tokens are security credentials that validate the identity of users. They serve as a means for users to access protected resources without constantly supplying their login credentials. The most commonly used token standard in the context of web APIs is JSON Web Tokens (JWT), which allows information to be securely transmitted between parties as a JSON object.
Definition and Importance of JWT
JWT is a compact, URL-safe means of representing claims to be transferred between two parties. This token is essential for stateless authentication, where the server does not need to maintain a session. The significance of JWT includes:
- Security: JWTs can be signed and optionally encrypted, ensuring that the content is protected and verified.
- Interoperability: JWTs are supported across different programming languages and platforms, promoting seamless integration.
- Scalability: With stateless nature, it scales well, making it suitable for microservices and cloud environments.
Key Features and Functionalities
The JWT structure consists of three components: Header, Payload, and Signature. Their roles include:
- Header: Contains metadata about the token, including its type and the signing algorithm used.
- Payload: Holds the claims, including user information and permissions.
- Signature: Ensures that the sender of the token is who it claims to be, and helps to validate that the message wasn’t changed.
Use Cases and Benefits
Incorporating JWT for authentication yields several benefits, such as:
- Simplified user management for single-page applications (SPAs).
- Enhanced security with reduced exposure of user credentials.
- Improved performance through stateless operations.
Best Practices
To ensure the effective implementation of authentication tokens in ASP.NET Core Web API, adhere to the following best practices:
- Use Strong Signing Algorithms: Employ algorithms like HS256 or RS256 to ensure the integrity of the token.
- Set Token Expiry: Assign short-lived tokens and refresh them as necessary to minimize the risk of misuse.
- Implement HTTPS: Ensure secure transmission of tokens to prevent interception.
- Store Tokens Securely: Use secure storage mechanisms within the client application, avoiding local storage where possible.
Tips for Maximizing Efficiency
- Leverage Middleware: Use ASP.NET Core middleware for validating tokens efficiently.
- Batch Processing: Process user requests in batches to optimize server resources.
- Monitor and Analyze: Utilize logging to keep track of token usage and identify unusual patterns.
Common Pitfalls to Avoid
- Relying on default configurations that may lack security.
- Not validating tokens properly, leading to unauthorized access.
- Allowing excessive token lifetimes without refreshing strategy.
How-To Guides and Tutorials
Implementing JWT in an ASP.NET Core Web API can be achieved through a methodical approach. Here’s a brief overview of the steps involved:
- Install Necessary Libraries: Add the NuGet package for JWT authentication.
- Configure Authentication: Adjust the file to include JWT in the authentication scheme.
- Generate Tokens: Create a method to issue tokens after a successful login.
- Validate Tokens: Implement middleware to validate token presence and integrity for incoming requests.
Here is a basic code snippet illustrating token generation:
This command generates a new Web API project named . When you open the solution, you'll see a basic structure for an API, which can be enhanced to include JWT authentication.
Installing Required Packages
Once the project is set up, you need to install the necessary packages for JWT authentication. The primary package required is . To install it, you can use the NuGet Package Manager in Visual Studio or run the following command in the command line:

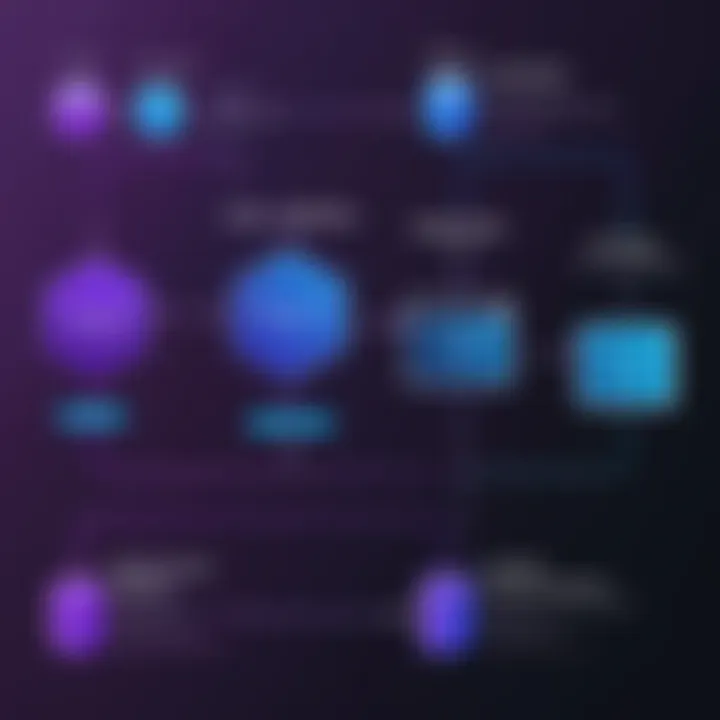
This package provides JWT bearer token authentication in ASP.NET Core applications. Optionally, you may also want to include for additional token handling capabilities.
Configuring JWT Authentication
After the packages are installed, the next step is to configure JWT authentication in the file. This involves setting up the authentication services and specifying token parameters:
- Include the necessary namespaces at the top of your file:
- In the method, add the authentication service configuration:
- Ensure to call in the method to activate authentication middleware.
By carefully setting up these configurations, you prepare your ASP.NET Core application to handle authentication tokens effectively, thus setting the foundation for a secure API.
Generating and Validating Tokens
Generating and validating tokens are crucial processes in implementing token-based authentication. They ensure that only authorized users can access specific resources or endpoints in your ASP.NET Core Web API. The tokens themselves carry information that confirms the user's identity and permissions. Therefore, understanding how to create and validate these tokens efficiently is paramount for maintaining the security and integrity of your application.
Successful token generation involves creating a unique token after user login, which can then be used for subsequent requests. When validating tokens, the server checks the token's authenticity and whether it is still valid based on predefined criteria like expiration time. The importance of these processes cannot be overstated, as without proper token management, your application could be vulnerable to unauthorized access or token reuse.
Creating a Token Generation Method
To create a token generation method in ASP.NET Core, begin by defining a service that will handle the token generation logic. This method needs to be secure and efficient to handle user requests. You can utilize JSON Web Tokens (JWT) as they provide a compact and self-contained way to transmit information. Below is an example outline for creating a token generation service:
- Define Token Options: Specify token characteristics like issuer, audience, expiration, and signing credentials.
- Claims Creation: Craft claims that encapsulate user information, such as roles and unique identifiers.
- Token Creation: Use the to generate a token with the defined claims.
- Return the Token: Finally, return the token to the user for use in their subsequent requests.
Here is a basic code example for generating a JWT:
Validating Tokens in API Requests
Validating tokens is essential in ensuring that each call made to your API is authenticated and authorized. This involves inspecting the token sent with each request and verifying its integrity. You should follow a few key steps for effective validation:
- Extract Token: Retrieve the token from the Authorization header of the incoming request.
- Validate Token Structure: Ensure the token follows the JWT format (header, payload, signature).
- Use Token Handler: Leverage the to validate the token against your signing key and check its expiration date.
- Claims Extraction: Once validated, extract the claims to ascertain what roles and permissions the user has.
An example implementation of token validation can be structured as follows:
Middleware and Authentication Pipeline
Middleware serves as a crucial component in the architecture of ASP.NET Core applications. It acts as a glue between components, processing requests and responses as they pass through the pipeline. In the context of authentication tokens, middleware plays an indispensable role in validating tokens and enforcing security policies. Proper understanding and integration of middleware can significantly enhance the security posture of your Web API.
Key features of middleware include:
- Request processing: Middleware can inspect, modify, or short-circuit HTTP requests.
- Contextual information: Middleware has access to requests and can set contextual information useful for later components in the pipeline.
- Delegated processing: Middleware can trigger the next component in the pipeline or halt further processing, depending on the authentication requirements.
With these benefits, middleware is essential for support of the authentication pipeline, ensuring that only authorized requests proceed through the application.
Understanding Middleware in ASP.NET Core
ASP.NET Core’s middleware is fundamentally different from that of classic ASP.NET. Middleware in ASP.NET Core is component-based and creates a pipeline through which requests travel. Each middleware component has the capability to look at incoming requests and decide how to process them before passing them along. Each stage can add logic, perform checks, and even interrupt or end the request.
Middleware runs as a series of delegates, functioning within a high degree of flexibility. This flexibility allows developers to layer functionalities such as authentication and authorization systematically. Each middleware is defined in the class, and developers control the order of execution.
Some commonly used middleware components include:
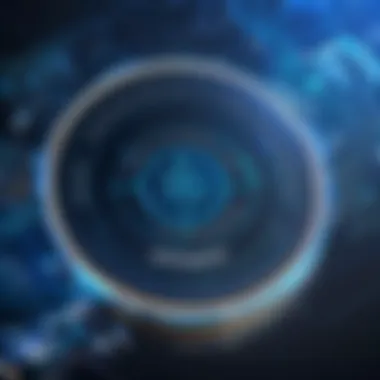
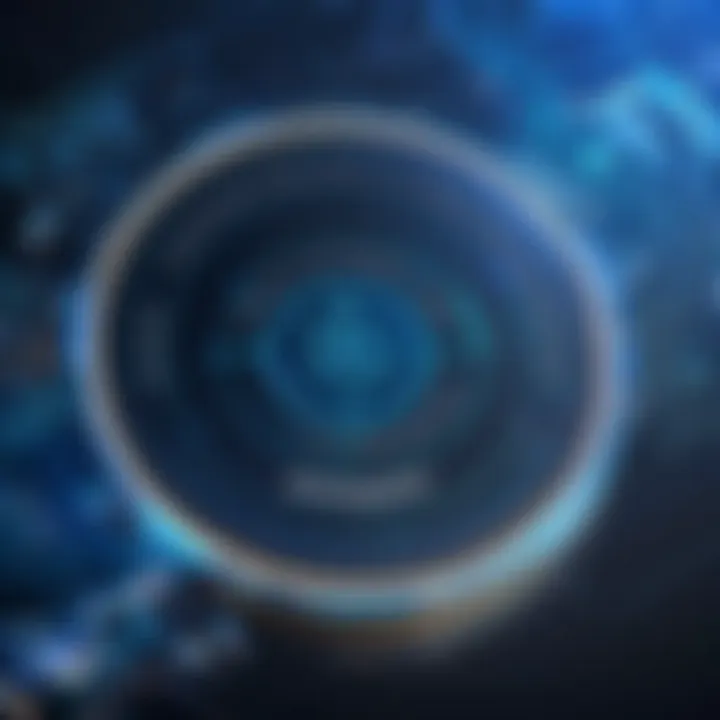
- Static Files: Serves static files like HTML, CSS, or JavaScript.
- Routing: Determines how URLs correlate to application endpoints.
- Exception Handling: Handles any errors that might occur during request processing.
In the section of authentication, implementing a middleware component specifically targeted for authentication can ensure that each incoming request is properly evaluated against the criteria necessary for access.
Integrating Authentication Middleware
Integrating authentication middleware into your ASP.NET Core application requires careful steps to ensure all authenticated requests are rightly filtered. Once the middleware is in place, it will intercept requests to check for the presence of an authentication token, validate it, and then set the user identity if the token is valid.
To integrate authentication middleware, you typically follow these steps:
- Install authentication packages: For JWT authentication, you can set up essential NuGet packages. A common choice is Microsoft.AspNetCore.Authentication.JwtBearer.
- Configure Middleware in : You will add the authentication middleware component to the services collection and specify your authentication configurations.
- Invoke the authentication middleware in the request pipeline: Ensure you call before in the method to maintain proper order of execution.
By following these steps, the middleware will effectively handle authentication tokens for your API. During the request handling phase, if a request lacks a valid token, the middleware's mechanism ensures that a 401 Unauthorized response gets promptly returned, minimizing security risks.
Important: Proper ordering and configuration of middleware is key to achieving desired security outcomes. Misplaced configurations may lead to security vulnerabilities.
Error Handling in Token Authentication
Error handling in token authentication plays a crucial role in the overall security architecture of an ASP.NET Core Web API. It is essential not just for diagnosing issues but also for maintaining user trust and application integrity. When a client application interacts with an API, especially in the context of authentication tokens, various types of errors can arise. These may range from failed logins due to invalid credentials to expired tokens that necessitate user re-authentication. Implementing effective error handling strategies is necessary to provide informative feedback to the user and assist developers in troubleshooting.
One significant benefit of proficient error handling is that it helps prevent unauthorized access. By clarifying the nature of errors, developers can ensure that clients understand the problems without exposing sensitive information. This minimizes the risk of information leakage while still guiding users toward resolutions for their challenges. Additionally, a well-thought-out error handling process keeps users informed about their authentication status, fostering a better user experience.
Common Authentication Errors
In the realm of token authentication, several common errors can occur, which developers must anticipate and manage effectively:
- Invalid Token: This occurs when a token has been tampered or otherwise altered, leading to rejection by the API.
- Expired Token: Tokens typically have a defined lifespan. Once expired, users must request a new token.
- Revoked Token: Tokens may be revoked by the server if a security threat is detected or if the user logs out.
- Malformed Token: Sometimes, token generation might lead to incorrectly formatted tokens. Such tokens cannot be processed.
- Insufficient Permissions: Occurs when the user’s token does not grant access to a requested resource or action.
Properly logging these errors can help developers understand patterns that might indicate a larger issue with the authentication process.
Graceful Error Responses
Graceful error responses are essential for creating robust APIs that handle failures elegantly. Instead of displaying raw error messages, providing structured responses can empower user applications to respond appropriately. This often includes returning standardized error codes and messages that clarify the nature of the problem.
For instance, consider the following JSON response structure for an invalid token:
Such responses enable client applications to interpret the error and take necessary actions, such as prompting users to re-authenticate or redirecting them to the login page. Additionally, ensuring all API responses conform to a consistent format simplifies debugging for developers.
Transforming error responses into friendly, actionable messages while retaining technical accuracy can significantly improve user experience. This level of detail informs application clients how to react to particular situations, thus minimizing confusion and frustration.
As a final note, the emphasis on error handling and graceful responses not only enhances the security profile of an application but also solidifies its reliability in the eyes of end users and system administrators alike.
Best Practices for Token-Based Authentication
Token-based authentication is a crucial aspect of securing applications built on ASP.NET Core Web API. By employing the right strategies, developers can significantly enhance security, user experience, and system performance. Adhering to best practices ensures that tokens are managed effectively, reducing vulnerability to attacks and unauthorized access. This section will focus on two key areas: Token Expiration and Refreshing, and Securing Secrets in Configuration.
Token Expiration and Refreshing
Tokens must have a limited lifespan for several reasons. First, short-lived tokens reduce the window of opportunity for an attacker if a token is compromised. When a token expires, an attacker has less time to exploit it. Common practice suggests setting the expiration time to an hour or less for access tokens. In contrast, longer expiration times might be appropriate for refresh tokens, which can be used to obtain new tokens without requiring a user to re-authenticate.
To implement token expiration effectively, follow these guidelines:
- Set Token Expiry: Specify expiration times when creating tokens. This can be done in the token generation method.
- Use Refresh Tokens: Implement refresh tokens that allow users to obtain a new access token without logging in again. This minimizes disruptions for users while maintaining security.
- Handle Expiration Gracefully: Clients must be able to handle token expiration smoothly. A 401 Unauthorized response should prompt the client to retrieve a new token using the refresh token if available.
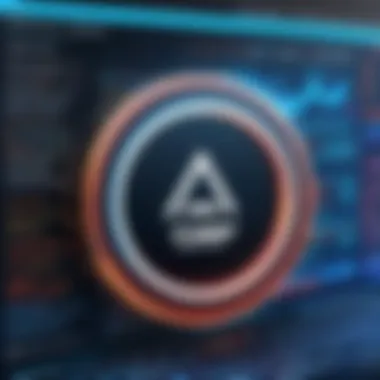
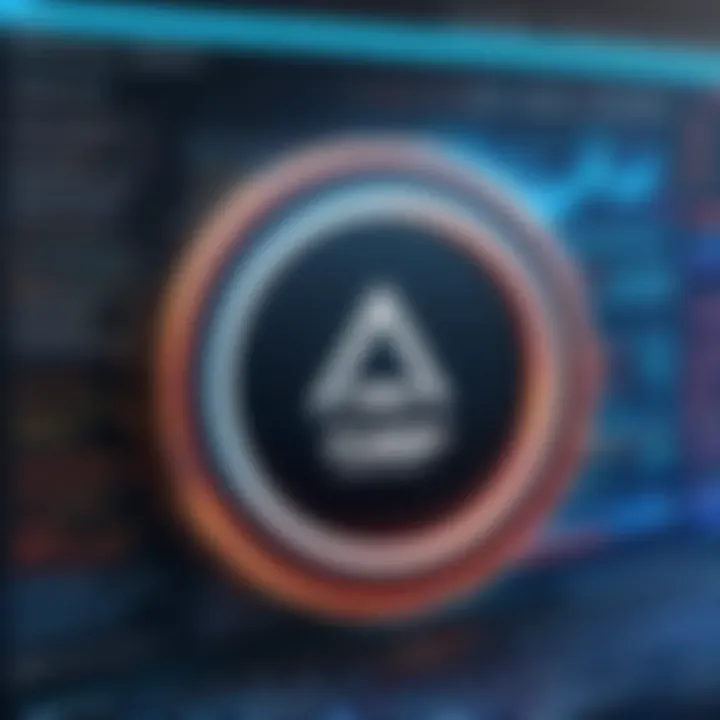
This methodology strengthens overall security. In addition, it aligns with industry standards. Using a structured approach, developers can ensure their applications remain secure while delivering a positive user experience.
Securing Secrets in Configuration
It is critical to keep sensitive information, such as signing keys and database connection strings, out of the source code. Securing these secrets helps to mitigate the risk of unintended exposure. Here are some recommended practices for securing these secrets:
- Environment Variables: Store sensitive information in environment variables. This practice isolates sensitive data from the application’s codebase, reducing potential risks.
- Azure Key Vault or AWS Secrets Manager: Utilize cloud services designed for secure storage of secrets. These platforms offer built-in security features that simplify the management and retrieval of sensitive data.
- Configuration Files: If using configuration files, ensure they are encrypted. Tools such as DotNet Core’s built-in configuration providers can help manage sensitive data securely. However, avoid hardcoding secrets directly into files that are part of your version control.
"Never put your secrets in code. Protect your credentials as you would protect your intellectual property."
Securing secrets is a fundamental practice that fundamental enhances the integrity of the authentication process. It minimizes the risk of data leaks and unauthorized access to your application.
Testing Your Authentication Implementation
Testing the authentication implementation in an ASP.NET Core Web API is crucial for ensuring that security measures are effective and do not introduce vulnerabilities. Authentication serves as the gatekeeper of your API, controlling who can access resources and how. Proper testing focuses on verifying that the authentication mechanisms function correctly, especially in various scenarios including successful authentications, failed attempts, and edge cases.
By incorporating thorough testing practices, developers can identify flaws early in the development cycle. This not only improves the reliability of the application but also enhances user trust, as users expect secure handling of their data.
Unit Testing Authentication
Unit testing is a fundamental practice in software development, particularly when it comes to authentication logic. In an ASP.NET Core application, unit tests can validate whether individual components function as intended. This includes testing token generation, validation logic, and any custom user authentication methods.
When developing unit tests for authentication, consider the following aspects:
- Testing Valid Scenarios: Ensure that valid credentials lead to the expected output, such as correct token generation and successful user identification.
- Testing Invalid Scenarios: Inputs that should fail, such as incorrect passwords, must return appropriate error messages without disclosing sensitive information.
- Mocking Dependencies: Use mocking frameworks to simulate dependencies such as database connections or external services. This isolation helps focus only on the authentication logic.
- Edge Cases: Test edge cases such as token expiration, user deactivation, and attempts to reuse tokens.
A simple example of a unit test for token generation using xUnit might look like this:
This concise test checks whether a token is generated when valid credentials are supplied.
Using Postman for API Testing
Postman is a powerful tool for testing APIs, especially when verifying the authentication components of a Web API. It allows developers to send requests to the API endpoints without writing extensive client-side code, making it a practical choice for manual testing.
In using Postman for your authentication tests, follow these steps:
- Setting Up Postman: If not already installed, download and set up Postman to begin testing.
- Creating a New Request: Open Postman and create a new request to the designated authentication endpoint.
- Configuring Authorization: Select the Authorization tab and choose the token type, such as Bearer Token. Input the token if previously generated during testing.
- Testing Various Scenarios: Send requests using both valid and invalid tokens to test the API's response. The expected response should differ based on the input:
- Examining Responses: Pay attention to the structure of the responses, focusing on status codes, error messages, and response times.
- Valid tokens should result in access to protected resources without error.
- Invalid tokens should return a 401 Unauthorized status code.
Using Postman not only simplifies the testing process but also provides a visual interface that can quickly highlight failures in the authentication flow.
By adopting both unit testing for automated checks and Postman for exploratory testing, developers can cover a broad range of scenarios. This dual approach enhances the overall security posture of the application, making it resilient against unauthorized access and potential vulnerabilities.
End
In this article, we have explored the complex realm of authentication tokens within ASP.NET Core Web API. Understanding how to effectively implement token-based authentication is crucial for securing web applications. The benefits are multifaceted, enhancing security while allowing flexible user access. Developers must recognize the importance of adopting best practices to safeguard their applications. Key aspects such as token expiration and secret management can significantly impact the security posture of an application.
Recap of Key Points
- Authentication tokens, particularly JWT, provide a robust means of securing APIs.
- The implementation process includes creating an ASP.NET Core application, managing dependencies, and configuring middleware.
- Token generation and validation are vital steps in ensuring authorized access to resources.
- Common authentication errors should be handled gracefully to improve user experience.
- Best practices emphasized include refreshing tokens regularly and securing secrets.
These elements illustrate not just the mechanics of implementation but also the strategic mindset needed for secure development.
Future Considerations in API Security
As the landscape of web development evolves, so too do the threats and methods of attack. Therefore, it is important for developers to stay informed on new vulnerabilities, authentication frameworks, and security protocols.
- Adoption of Emerging Standards: New standards, like OAuth 2.1, aim to streamline secure authentication. Keeping abreast of these changes can provide better security implementations.
- Machine Learning in Security: Utilizing machine learning to monitor authentication patterns can help quickly identify anomalies and potential incursions.
- Regular Security Audits: Commit to regularly scheduled security audits. This practice will help in identifying weaknesses before they can be exploited.
- User Education: Educate users about secure practices, such as the importance of two-factor authentication and recognizing phishing attempts.
Securing APIs is not a one-time effort; it requires ongoing vigilance and adaptability to new challenges.
By focusing on these aspects, developers can not only protect their applications but also enhance trust with users, marking them as leaders in secure application development.