Mastering Advanced Node.js Concepts for Developers

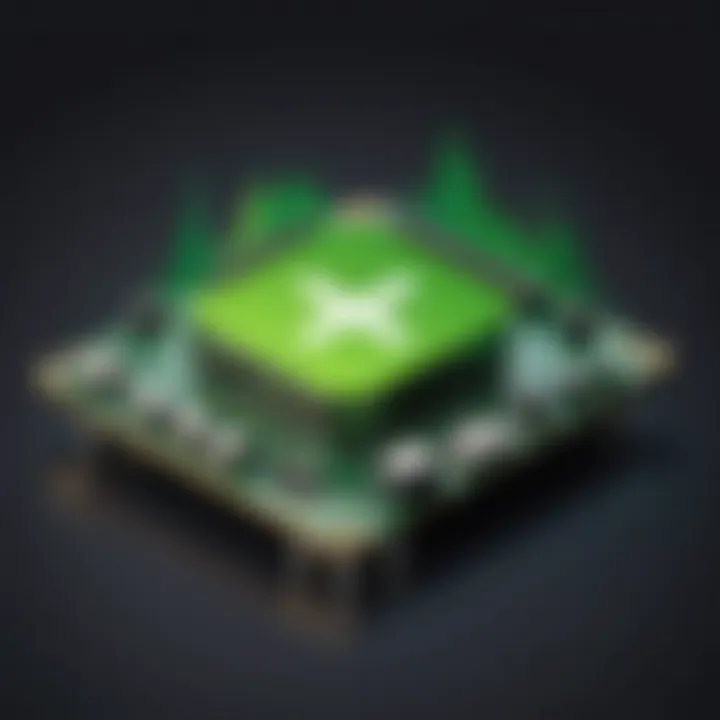
Intro
Node.js has become a significant pillar in the landscape of modern software development. Designed on top of the Chrome V8 engine, Node.js utilizes JavaScript for server-side scripting. This approach simplifes the ability for developers to build efficiently scalable network applications. Yet, the sophistication of Node.js extends far beyond these simple conveniences. This guide will deliberate on nuanced aspects of Node.js for those who seek mastery in execution and design.
In this exploration, topics like performance optimization, advanced asynchronous programming, and security considerations will be meticulously dissected. Software developers and IT professionals will find practical examples and guidelines that align with today’s industry requirements. Through this intricate yet structured discussion, we aim to go beyond mere implementation, championing the adept use of libraries and frameworks that enhance functionality.
In consolidating these discussions, valuable insights and situational applications will be highlighted to serve both the beginner and seasoned professional.
Overview of software development and Cloud Computing
Cloud computing serves a fundamental role in contemporary software development, facilitating a robust infrastructure for performance and scalability.
Definition and importance of Cloud computing
Cloud computing refers to the delivery of computing services over the Internet. These include servers, storage, databases, networking, software, analytics, and intelligence. As the flexibility offered by this technology transcends traditional models, its importance becomes apparent. Organizations can now operate without managing physical servers, a game changer for both cost and agility.
Key features and functionalities
- Scalability: Easy scaling up or down according to demand.
- Cost Efficiency: Pay for what you use, minimizing excess.
- Accessibility: Information available anywhere with internet access.
- Security: Often include robust security protocols inherent to the provider.
Use cases and benefits
- Development and Testing: Quickly create development and testing environments.
- Storage Solutions: Secure and reliable storage provided by clouds like Amazon S3.
- Big Data Processing: Clouds accommodate vast amounts of data, fitting various needs.
Security must be carefully integrated within node applications using cloud services. Thus, remaining vigilant about best practices and audits can avert potential vulnerabilities caused by the complexities of distributed environments.
Best Practices for Advanced Node.
js Development
Maximizing the benefits of Node.js requires adhering to industry standards. Here are quintessential practices:
Industry best practices
- Code Modularity: Break code into smaller modules for clarity and code reuse.
- Error Handling: Implement diligent error handling to ensure robustness.
- Asynchronous Programming: To truly harness Node's power, comprehend asynchronous functions accurately.
Tips for maximizing efficiency and productivity
- Use npm for efficient package management.
- Utilize built-in debugging tools to catch issues early in development.
- Clarify your code base - clear names and function documentation save time in the long run.
Common pitfalls to avoid
- Forgetting to handle promise rejections, reducing code quality.
- Overusing global variables, leading to undesirable side-effects.
- Relying wholly on defaults within libraries; customizing them improves efficiency.
Important: Adhering to best practices not only improves your application but also simplifies maintenance for future developments.
Case Studies
Examining real-world applications shed light on effective implementation strategies. For example:
- Trello, an application that utilizes Node.js for real-time collaborative features and efficiency.
- eBay, which employs Node.js to enhance page dynamics and performance analytics significantly.
Lessons learned and outcomes achieved
Both Trello and eBay observed marked improvements in user engagement and response time, demonstrating the solid architecture Node.js architecture can offer when used to its fullest capability. The importance of continued research and development should inform Catalyst organizations about niche adjustments needed.
Insights from industry experts
Professional opinions emphasize the critical nature of understanding both strengths and limitations. Experts suggest comprehensive testing on networks before moving applications into production environments. This knowledge ensures potential user-base booms are enjoyable and frustration-free.
Latest Trends and Updates
Remaining abreast of developments in Node.js is essential. Notable advancements include:
- Adoption of ECMAScript 2022 standards, refining syntax and performance.
- Expanding libraries and frameworks including Fastify and NestJS offering alternative server frameworks.
Current industry trends
More users are gravitating towards serverless architectures, relieving direct hardware encumberances. APIs are evolving to accommodate complex networks, paralleling the growth of front-end enhancements effectively.
Innovations and breakthroughs
Modern development now considers machine learning implications; Node.js is continually finding itself at that crossroad, being engineered to accommodate algorithmic demands.
How-To Guides and Tutorials
This segment serves kind to those seeking methods for deploying Node.js.
Step-by-step setup
Setting up a basic Node.js server can be accomplished with:
Hands-on tutorials for beginners and advanced users
Advanced users may interest in exploring WebSockets for real-time communication using frameworks like Socket.io. Basic implementations can start at listening for events and quickly delving into more complicated interactions.
Practical tips and tricks
Testing libraries, such as Jest, ensure that you build error-free applications in iterative cycles faster.
Emphasizing industry responses reflects the need for thoroughness and encodes enablement into Node.js exploration. With careful consideration and deployment of cutting-edge features, users can value from consistent function and forms that enhance experience.
Foreword to Advanced Node.
js Concepts
Understanding advanced Node.js concepts is crucial for software developers and IT professionals working with today’s web applications. Node.js, known for its scalability and efficiency, has evolved from its simple roots into a powerful runtime environment. Grasping these advanced concepts can significantly improve application performance, security, and code maintainability.
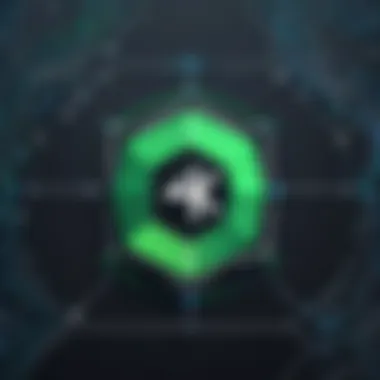
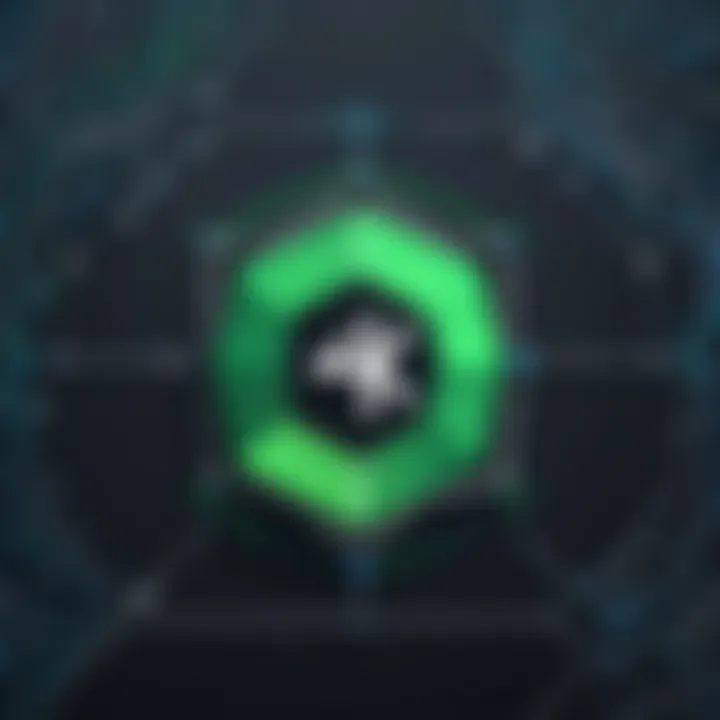
Understanding the Node.
js Event Loop
The Node.js Event Loop is at the heart of Node's non-blocking architecture. It allows Node.js to handle multiple operations simultaneously, thus enabling high throughput and low latency.
When you send an I/O request, instead of waiting for the request to complete and blocking the thread, the event loop registers the request, proceeds to execute other operations, and comes back to handle the completed request. This mechanism makes Node.js especially suitable for I/O-heavy applications, such as web servers or real-time collaboration tools.
To visualize how the event loop works, consider the following simplified structure:
- Initialization: Load all operations into memory.
- Event Queue: Place completed operations into a queue.
- Execution: Execute operations from the queue one at a time, allowing new async events to be processed.
An understanding of how the event loop operates ensures that developers can write more efficient code, leveraging these non-blocking features to their advantage.
The Importance of Non-Blocking /O
Non-blocking I/O is a pivotal feature of Node.js that significantly enhances application performance. It allows developers to manage multiple input and output processes while freeing up the main thread for other operations.
Key reasons highlighting the importance of non-blocking I/O are:
- Improved Scalability: Node.js can handle a great number of concurrent connections making it suitable for scalable applications.
- Optimized Resource Utilization: Resources such as memory and processing power are used more efficiently, minimizing waste.
- Responsive User Experience: Applications behave more responsively, as users do not need to wait for blocking tasks to complete.
In practical terms, operations can be performed using callbacks, which are passed to the I/O request. This practice ensures that code execution does not stall even for resource-heavy operations. Moreover, knowing when to use non-blocking vs blocking code is pivotal in optimizing resource consumption.
Non-blocking I/O transforms how developers think about concurrency in execution, pushing towards writing scalable and efficient applications.
By delving deeper into the advanced aspects of Node.js, you can harness the full power of its non-blocking architecture to build efficient and highly responsive applications.
Optimizing Node.
js Applications
Optimizing Node.js applications is critical for enhancing performance, improving responsiveness, and ensuring overall reliability. Given the non-blocking nature of Node.js, it is crucial to grasp how various techniques can lead to a more efficient application. Understanding these optimizations not only boosts application speed but also reduces resource consumption, which can lead to significant cost savings. To navigate the intricacies of Node.js, one must explore the avenues of profiling and monitoring, utilizing clustering, and employing caching strategies.
Profiling and Monitoring
Profiling and monitoring serve as foundational concepts in optimizing any software application, including those built on Node.js. Profiling assesses the application’s performance by collecting data during execution. It highlights key metrics, such as memory usage, CPU load, and event loop delays. That information helps developers pinpoint bottlenecks or inefficiencies.
Monitoring, on the other hand, has a proactive approach. Continuous observation of application performance can signal issues before they impact users. Tools like , , or native Node.js modules like allow developers to respond swiftly to performance dips that might otherwise go unnoticed.
By combining profiling and monitoring, developers can establish a performance baseline. This will ultimately inform future optimizations, allowing for data-driven decisions that significantly enhance user satisfaction. Importantly, neglecting these practices can lead to unforeseen downtimes or losses in revenue, underscoring their necessity.
Utilizing Clustering for Maximum Performance
Utilizing clustering is a powerful way to maximize Node.js performance. Since Node.js operates on a single-threaded model, tasks that demand extensive CPU resources can quickly choke the event loop, leading to slower response times. Clustering addresses this limitation by spawning multiple instances of a Node application, each handling different requests simultaneously.
Structured as child processes, these instances can fully utilize multi-core processors, significantly improving throughput. A common strategy entails using the built-in module to facilitate this process.
Implementing clustering effectively demands careful consideration of load balancing. The module can help automatically distribute incoming requests across multiple instances. Alternatively, external solutions, like Nginx or HAProxy, can also manage load, enhancing resilience and speed.
Benefits of clustering include:
- Improved application responsiveness
- Efficient use of available hardware resources
- Reduced latency during high traffic periods
However, clustering is not without downsides. For example, maintaining state across processes can introduce complexity. Developers need to navigate these challenges meticulously to fully leverage clustering's advantages while ensuring the application's integrity.
Caching Strategies
Caching strategies greatly improve performance in Node.js applications. By storing copies of frequently accessed data, applications can significantly reduce latency. Implementation reduces the need to repeatedly generate the same data periodically.
Different methods can be utilized, each with unique advantages. Here are some common approaches:
- Memory Caching: For a quick retrieval of static or frequently used data.
- File Caching: Uses local disk storage for larger resource allocations that do not require quick changes.
- Distributed Caching: Implements solutions like Redis to share cache data across instances, making terminations seamless across multiple processes.
- HTTP Caching: Allows servers and clients to cache content, using headers such as to manage lifetimes efficiently.
Implementation considerations may vary based on the chosen caching strategy. The focus should always remain on expiration management to prevent stale data and unnecessary resource consumption.
Security Best Practices for Node.
js
In the realm of software development, security cannot be an afterthought. As Node.js enjoys growing popularity, it becomes essential to understand and address various security considerations. Node.js applications often handle sensitive user data, making them prime targets for malicious attacks. Therefore, applying robust security best practices is critical not only for protecting valuable data but also for maintaining user trust and conforming to legal requirements.
Understanding Common Vulnerabilities
To secure your Node.js applications, it is vital to be aware of common vulnerabilities. Knowing what you are up against allows developers to proactively implement defensive measures.
- Injection Attacks: These include SQL and command injection, where an attacker fills in unexpected inputs to manipulate database queries or system commands. Using parameterized queries in datase access is one effective method of defense.
- Cross-Site Scripting (XSS): In this case, malicious scripts are injected into web pages viewed by users. Avoiding unvalidated input to client interfaces is vital to protect integrity.
- Cross-Site Request Forgery (CSRF): This triggers unauthorized actions in web applications on behalf of authenticated users. To mitigate risks, use anti-CSRF tokens to verify requests that change data.
Understanding these vulnerabilities fosters an awareness that to be used across development practices, resulting in more secure code construction.
Implementing Middleware for Security
Middleware can significantly fortify your Node.js applications against various attacks. Placing security checks early in the handling process can protect your endpoints before they are actually hit. Implementing certain middleware can bolster your security measures efficiently.
- Helmet: This is an essential library enhancing HTTP headers for vulnerabilities protection, such as clickjacking. Including in your Express app is simple:
- Rate Limiting: Implementing a rate-limiting middleware can help deter brute-force attacks. Using NPM packages like provides this functionality easily.
- Sanitize User Input: To intercept malicious input aimed at causing security breaches, integrated libraries such as and alternative validation libraries should be adopted. Sanitizing data can limit risks significantly.
Using middleware correctly fortifies security layers and sets a strong defense against many issues associated with web applications effectively. Every developer working with Node.js needs to invest in understanding and applying these best practices.
Asynchronous Programming Techniques
Asynchronous programming is a cornerstone of Node.js, allowing developers to write code that executes without blocking the main thread. This characteristic is significant because it enables applications to handle multiple operations concurrently, optimizing performance and responsiveness. The process helps prevent delays while waiting for tasks like file system access or network requests to complete. Synchronizing tasks in Node.js througheffective asynchronous methods leads to better resource utilization and enhanced user experience.
Using Promises Effectively
Promises are a powerful feature in JavaScript, introduced to make handling asynchronous operations cleaner and more manageable. They represent an operation that hasn't completed yet, allowing developers to attach success and failure handlers. When using promises, one important practice is to chain multiple promises together, providing a clearer control flow. Here’s an example:
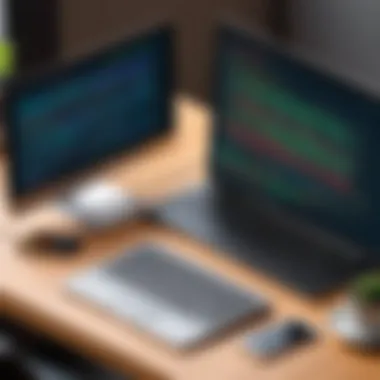
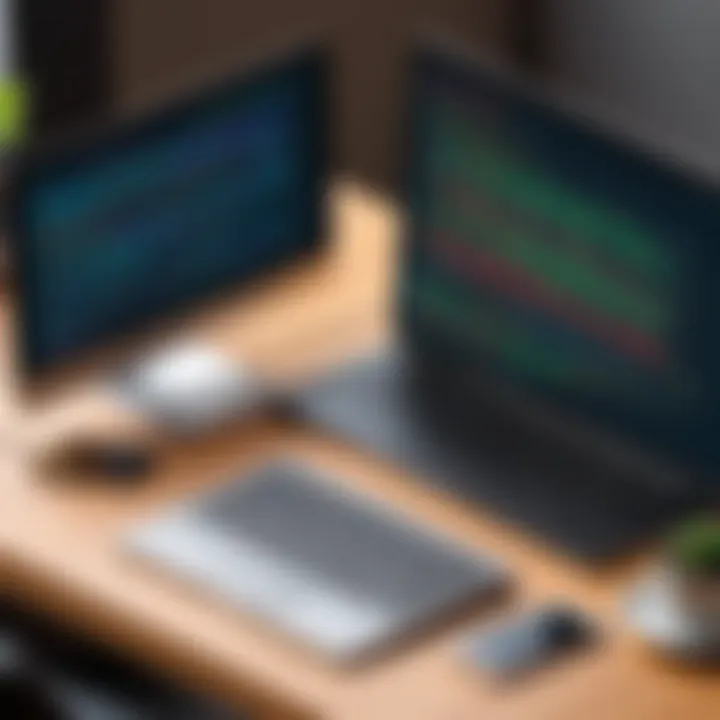
Using promises like so allows error handling to be concise and prevents the infamous callback hell, making your application more maintainable and less prone to errors. Remember that when working with promises, you should handle them correctly to avoid memory leaks or unhandled promise rejections.
Async/Await: Practical Applications
The syntax is a more recent addition to JavaScript that simplifies working with asynchronous code. This youth-extension builds on promises and leads to a more intuitive structure. When you declare a function with , the function always returns a promise. Inside this function, you can now use in front of asynchronous operations. Thus, an application can wait for a promise to resolve before proceeding.
Here's a brief example:
This code waits for the completion of the promise before logging the data. This makes the control flow much easier to read than chaining multiple statements. In practice, this can enhance code clarity and ease of debugging, leading to greater maintainability. The performance remains intact, leveraging Node.js asynchronous nature.
Error Handling in Asynchronous Code
Error handling is often overlooked in asynchronous programming but is crucial. In both promises and async/await syntax, errors can bubble up differently from synchronous code. The common strategy involves using blocks around expressions. In contrast, stake challenges involve ensuring promises catch errors correctly.
Important Considerations for Error Handling:
- Use with promises to manage errors effectively.
- Always utilize in async functions to handle potential exceptions.
- Validate user input before initiating an asynchronous operation to prevent runtime exceptions.
- Log meaningful error messages for easier debugging later.
By implementing these strategies for error handling in asynchronous code, developers can significantly enhance the stability and reliability of their applications. Proper error management not only prevents crashes but also provides insightful information about issues occurring within the application pathways.
Effective asynchronous programming is not only about making your code run faster; it’s about writing maintainable and comprehensible code that is resilient against errors.
COMLINES: Advanced Libraries and Frameworks
The role of advanced libraries and frameworks in Node.js cannot be understated. They provide the tools that enable developers to streamline their workloads, enhance productivity, and build applications more efficiently. Libraries abstract complex tasks, while frameworks offer a structured environment to develop applications. This section explores specific libraries that every advanced Node.js developer should know.
Express.
js for Building RESTful APIs
Express.js is a minimal and flexible Node.js web application framework. It provides a robust set of features to develop web and mobile applications. Express.js simplifies the process of creating RESTful APIs.
Key Features:
- Lightweight: Ideal for creating quick API setups.
- Middleware support: Extensive ability to use middleware in your app.
- Routing: Supports dynamic routes, helping to create clear URL structures.
Express.js allows handling various HTTP requests easily, which is fundamental when building RESTful APIs. Developers benefit from its simplicity and versatility. The middleware functionality enables the implementation of cross-cutting concerns like authentication and logging seamlessly.
Using Koa.
js for Modern Applications
Koa.js is a relatively newer framework compared to Express and aims to become a smaller, more expressive award-winning framework for web applications and APIs. Koa was created by the same team behind Express but is designed to leverage JavaScript's async/await functionality to offer cleaner and more intuitive code.
Advantages:
- Smaller footprint: Less boilerplate compared to other frameworks.
- Modular design: Allows for better organization of your application.
- Control over middleware flow: Developers have complete control over the request and response cycle.
With Koa.js, developers can write a more straightforward, maintainable code, as it uses modern JavaScript features. The production of clean code leads to fewer bugs during the execution of asynchronous functions.
Integrating Socket.
IO for Real-Time Applications
Socket.IO is a powerful library for real-time, bidirectional communication between web clients and servers. In a world where real-time applications are critically important, integrating Socket.IO with Node.js expands the functional capabilities of traditional web servers.
Uses:
- Chat applications: Enabling real-time communication in chat.
- Collaborative tools: Syncing sessions across various users.
- Live notifications: Updating users simultaneously across platforms.
The library provides an easy-to-use API allowing developers to build applications that require real-time updates with minimal configurations. This capability enhances user interaction significantly and accumulates value for applications that depend on real-time data flow.
Node.
js in Cloud Environments
Node.js has transformed how developers build scalable and efficient applications. With the rise of cloud computing, its importance increases. Deploying Node.js applications in cloud environments offers several advantages. These include scalability, cost-efficiency, and flexibility in infrastructure. Cloud services provide the underlying resources required to run Node.js applications without extensive server management.
Using cloud platforms for Node.js enables developers to focus on building their applications mores effectively instead of struggling with server configuration. The ability to scale applications based in fluctuating demand is particularly beneficial. Furthermore, cloud services allow for consistent performance monitoring, which can lead to better resource optimization over time.
Deploying Node.
js on AWS
Amazon Web Services (AWS) is a leading cloud service provider with robust support for Node.js. With AWS, developers can deploy their applications using various services like Elastic Beanstalk, Lambda, and EC2. Each service caters to different needs, enabling tailored solutions depending on the project requirements.
- AWS Elastic Beanstalk: This service allows for easy deployment and management of applications. Developers simply package their Node.js application, and Elastic Beanstalk handles the rest. Automatic scaling is done based on the traffic.
- AWS Lambda: For serverless applications, Lambda is an excellent option. It allows developers to run Node.js code without having own server management responsibilities. The application is executed only while active and you only pay for the computation time used.
- Amazon EC2: For those seeking complete control over the server environment, EC2 provides the necessary flexibility. Here, developers can configure servers specifically to the needs of their Node.js application, though this requires deeper infrastructure knowledge.
Key Considerations
When deploying Node.js on AWS, certain factors must be kept in mind. It's important to consider:
- Security settings: Ensure proper access controls and network configurations to protect applications.
- Cost management: Use AWS pricing calculators to estimate expenses according to usage patterns.
- Performance optimization: Introduce caching mechanisms to improve speed, placing applications in closer proximity to users can have a significant outcome.
Using Docker for Node.
js Applications
Docker greatly enhances the deployment of Node.js applications. Containers allow encapsulation of all the application dependencies, ensuring consistent behavior across any environment. This leads to fewer conflicts and more confidence in deployment.
Benefits of Using Docker
- Simplifies environment management: Developers can create a consistent environment that matches production.
- Facilitate scalability: Containers can be run in parallel, improving the application’s ability to handle concurrent requests.
- It improves continuous integration/continuous deployment (CI/CD): Docker integrates seamlessly within CI/CD pipelines, producing effective and reliable deployments.
Considerations for Usage
To derive full benefits from Docker, careful planning is required. Key considerations include:
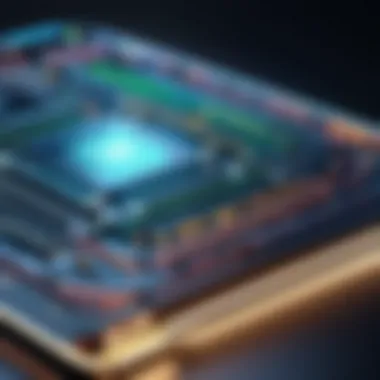
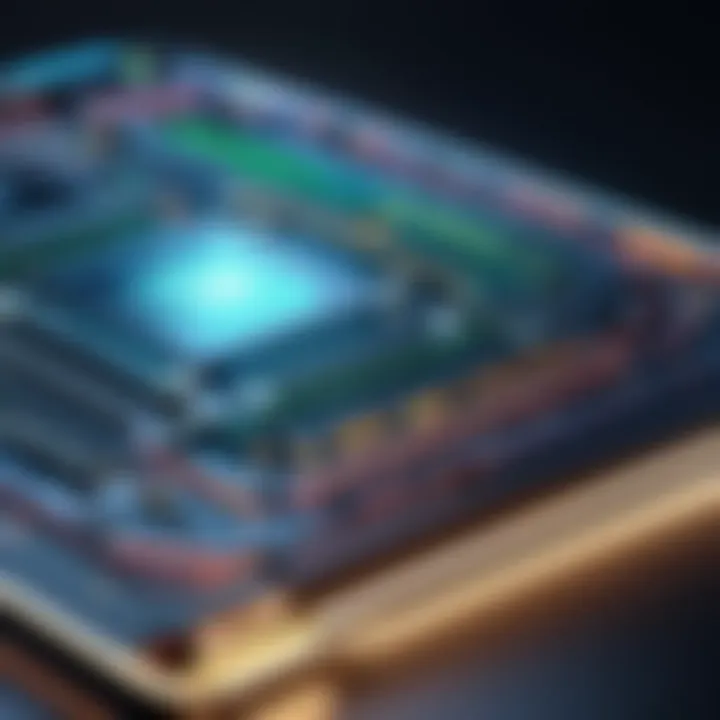
- Keeping images small: Smaller Docker images lead to faster downloads, which is crucial for deployment speed.
- Managing configurations: As the application scales, managing different configurations becomes essential. Using environment variables and config files is a practical approach.
Unit Testing in Node.
js
Unit testing is a crucial aspect of modern software development, especially in Node.js applications. Good testing practices significantly improve code quality by identifying bugs before deployment. It ensures that individual components function as intended. This process becomes vital in an environment rapidly evolving like Node.js, where changes can introduce unintended consequences.
By utilizing unit tests, developers can refactor code with confidence, as there is a safety net to verify that functionality stays intact. These tests also support future integration or scaling efforts, making them indispensable tools for maintainable code.
Choosing Testing Frameworks
Selecting the right testing framework is imperative to ensure robust unit tests for Node.js applications. There are several frameworks available, but a few stand out due to their extensive community support and feature sets.
- Jest: A popular framework with a straightforward API and built-in mocking capabilities, making it easy to test both synchronous and asynchronous code. Its zero-config setup and parallel test execution enhance speed and efficiency.
- Mocha: A flexible testing framework that allows variable reporting styles and can adapt to any testing approach preferred by the developer. It often pairs well with assertion libraries like Chai for increased versatility.
- AVA: Known for its concise syntax and focus on running tests concurrently, AVA is effective especially in larger projects with many tests that support promises and async/await method seamlessly.
Choosing a testing framework often depends on organizational requirements, team familiarity, and project size. Developers should evaluate them to find out which one aligns strictly with their needs.
Writing Effective Unit Tests
Effective unit tests should accurately reflect expected behavior and maintain clarity and comprehensibility. An approach to writing these tests consists of several strategies:
- Understand the Functionality: Always clarify what a code component should achieve. This understanding lays the groundwork for a valuable testing strategy.
- Keep tests isolated: Ensure that each test concerns only one unit of functionality. Isolated tests can easily pinpoint where problems arise.
- Use descriptive names: Naming tests appropriately helps other developers understand what each test does at a glance. Consider this while crafting the test names clearly stating its expected outcome.
This snippet showcases a basic unit test for a simple sum function. The structure comprises a descriptive context and identifies what is happening in each test, streamlining debugging efforts.
Overall, unit testing in Node.js is not just a practice, but a core methodology that contributes significantly to resiliency in applications. The adoption of thoughtful testing frameworks paired with meticulously written tests enhances the development process, leading to robust and error-resistant Node.js applications.
Microservices Architecture with Node.
js
Microservices architecture is an important concept in modern software development. It recomends splitting applications into smaller, independent components that each handle a specific concern. This approach presents multiple benefits particularly when working with Node.js, which is known for its non-blocking I/O and event-driven architecture. By utilizing Node.js in a microservices context, developers can achieve increased flexibility, scalability, and management of complex systems.
Benefits of Microservices in Node.
js
The benefits of adopting microservices architecture in Node.js frameworks are notable. Here are a few key advantages seen by developers:
- Scalability: Each microservice can scale independently according to the demand of its specific functionality. It allows developers to allocate resources more efficiently.
- Flexibility in development: Teams can develop independent services at their own pace and prefer different technologies best suited for the task.
- Improved fault isolation: A failure in one microservice does not instantly bring down the entire system, improving overall resiliency.
- Ease of deployment: Smaller services can be deployed without affecting others, promoting faster release cycles and continuous integration.
Overall, microservices promote services that are aligned with business capabilities and improve both development efficiency and application maintainability.
Communication Protocols for Microservices
In a microservices architecture using Node.js, communication between services can occur asynchronously or synchronously. Several communication protocols should be considered when designing the interactions:
- HTTP/REST: The typical HTTP protocol is widely utilized for RESTful services. It's simple and familiar for developers, making it easy to integrate with existing web technologies.
- gRPC: This modern remote procedure call (RPC) framework lets different services communicate with a higher performance standard compared to standard HTTP. It can also take advantage of different language support.
- Message Brokers: Technologies such as RabbitMQ or Apache Kafka foster decoupled communication, perfect for asynchronous interactions. It guarantees durability and scalability in message transmissions.
Using these protocols effectively is crucial. Each option has trade-offs, and careful consideration of needs and project size is vital.
Microservices architecture encourages modular design, resulting in an easier planning, coding, and testing phases throughout the software development life cycle.
Advanced Debugging Techniques
Debugging is a critical aspect of software development, particularly in environments like Node.js where asynchronous programming can lead to complex issues. By employing advanced debugging techniques, developers can resolutely identify and fix bugs, improving the overall application performance and enhancing reliability. Hence, mastering how to efficiently diagnose problems in Node.js can save time and reduce frustration.
Using Debugging Tools Efficiently
Effective debugging hinges on the strategic use of tools. Node.js provides several built-in debugging options, while external tools can also enhance the debugging process significantly.
A prominent choice is the Node.js Inspector. It allows developers to connect an IDE, like Visual Studio Code, to the Node.js process for interactive debugging. To start debugging with this tool, simply run your Node.js application with the flag. The debugging can then be performed right in the IDE, making the investigation of issues more intuitive.
Benefits of Utilizing Inspector:
- Breakpoints: Set breakpoints to pause execution and examine variables.
- Call Stack: View the current call stack for better visibility of function execution paths.
- Scope: Inspect local and global variable scopes thoroughly.
Taking advantage of logging with libraries, such as Winston or Bunyan, is also beneficial. This lets you capture detailed logs at various traces, offering insights into the application's behavior. You might find it worthwhile to implement different logging levels like error, warning, and information to segment the logs logically.
Utilize structured logging, which can integrate easily with log management systems. It provides better analyzation and tracking of issues.
Debugging Asynchronous Code
Asynchronous code can make debugging more challenging, adding layers of complexity not present in synchronous flow. Promise chains and callback functions require more focused approaches during the debugging process. Here's how to navigate this terrain effectively:
- Error Handling: Ensure that errors are caught appropriately. In promise-based code, using at the end of promise chains is crucial. For async/await having try/catch blocks neatly encapsulated can encapsulate function calls.
- Tracing Function Calls: Use tools like to output the call stack. It helps to identify how various call responses chain together.
- Async Stacks: Node.js provides capabilities to inspect asynchronous call stacks. Utilizing these eliminates guesswork about the origin of exceptions.
In summary, advanced debugging techniques revolve around fully harnessing Node.js's native debugging utilities alongside efficient practices tailored for asynchronous programming. Adopting these approaches can lead to the swift identification of issues and a smoother deployment process.
Closure and Future Directions
In the realm of software development, the conclusion serves as an important reflection on what has been learned and what steps to take moving forward. This section illustrates the significance of Conclusion and Future Directions within the context of advanced Node.js practices. Thorough understanding and exploration in these areas can benefit both individual professionals and the broader development community.
Staying Current with Node.
js Developments
The technology landscape is ever-evolving. For developers using Node.js, it is important to stay updated with the latest enhancements and versions. Each update introduces new features and fixes. Ignoring these updates can lead to security vulnerabilities and performance bottlenecks in applications. Following repositories like GitHub regularly is a good practice.
Remaining abreast of innovations involves participating in discussions. Online forums and platforms like Reddit host rich conversations on trending topics. Professional blogs, newsletters, and Node.js conference notes often are informative resources as well.
Furthermore, engaging with community proposals, codes, ontification patterns, and attending meetups helps in networking, sharing, and acquiring knowledge recently unveiled techniques or strategies.
Community Resources and Contributions
Engaging with the Node.js community fosters knowledge sharing among developers, It enhances skills when one collaborates with others in the field. The vibrant community around Node.js is one of its undeniable strengths. Understanding where to find and how to utilize this knowledge can elevate a developer’s proficiency.
Consider contributting to community-driven projects or initiatives on platforms like GitHub and even Wikipedia. Many requests for mentorship programs or code reviews are often found on social media like Facebook or specialized forums.
There are manifold community resources one can utilize. Some notable organizations include the Node.js Foundation and OpenJS Foundation. Their catalogues offer training materials. These resources help developers remain productive and informed. Through thought leadership, as expressed in articles and forum posts, one can leverage community insights as practical guideposts in real-world scenarios.
In summary, productivity in advanced Node.js is enhanced by actively engaging with current themes and community contributions. Continuous enhancement of coding techniques and insights from other technical professionals is integral for future success.